Writing your first loop: Console log Hello 10 times
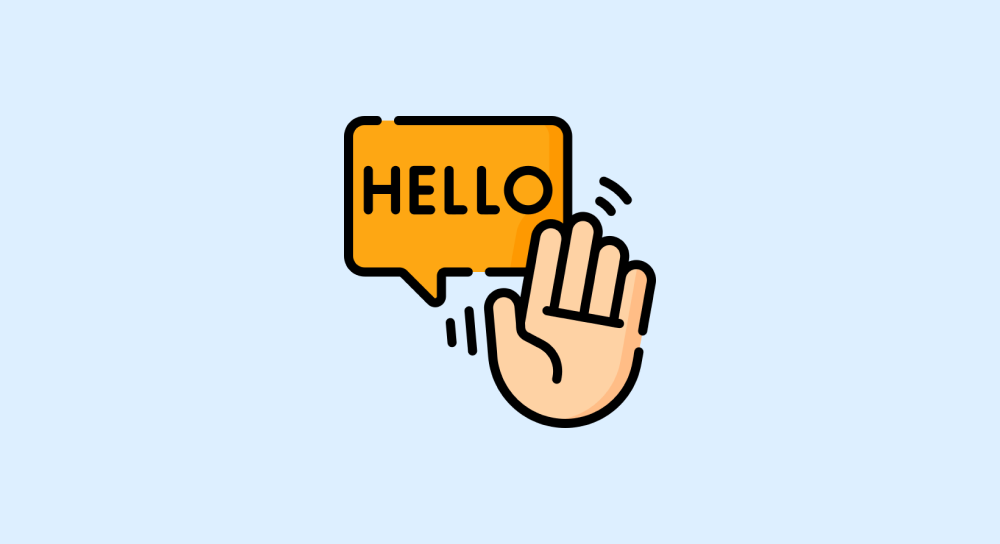
I know repeatedly printing "hello" on a browser console is not a practical example.
But when learning to program loops, it is important that you take baby steps by crawling before you walk.
So, let's start with simple and impractical exercises and then move on to practical exercises.
Anyway, In order to write a loop in programming, we need to use a loop statement.
There are different types of loop statements in Javascript:
- "for" Loop
- "while" loop
- "do...while" loop
- "for...in" loop
- "for...each" Loop
- Few other kinds of loops
Each of these loop statements has different mechanisms and syntax.
So, certain loop statements are a better choice compared to others in specific situations.
In other words, choosing the right loop statement depends on the task at hand, and your judgment for choosing the right loop statement will improve as you keep gaining experience in Javascript.
So, let's understand the task for this lesson and then decide the correct loop statement to use.
The task at hand: Log "hello" to the browser console 10 times
If loop statements don't exist in programming, we could have achieved the task like this:
console.log("Hello");
console.log("Hello");
console.log("Hello");
console.log("Hello");
console.log("Hello");
console.log("Hello");
console.log("Hello");
console.log("Hello");
console.log("Hello");
console.log("Hello");
But because loop statements do exist.
So, we shouldn't type the console.log
method ten times, as shown in the above code.
Instead, we should automate it using a loop statement so that console.log
method is only typed once but gets executed ten times:
for(let counter = 1; counter <= 10; counter++){
//execute the below line of code ten times
console.log("hello");
}
Now comes an important question: What would be the correct loop statement to use to achieve this task?
The task clearly mentions that we must log the word "Hello" 10 times to the browser console.
If we revisit the definition of a loop, it means repeating an action a certain number of times or until a condition is met.
In our case, the action is logging the word "Hello" to the browser console, and we want to repeat it 10 times.
Whenever we want to repeat an action a certain number of times, let's say 10 times, the best loop statement for the job is a "for" loop statement.
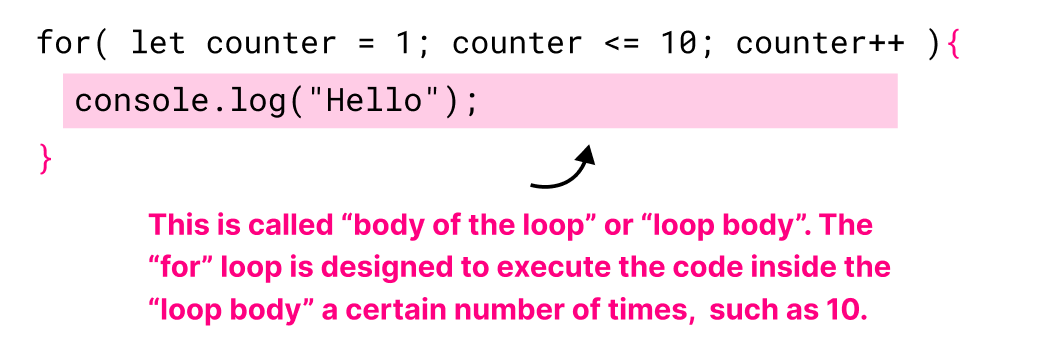
Another definition: A "for" loop statement is helpful only if we know how many times we must repeat an action.
This is because the "for" loop statement provides us with a mechanism to set up and manage a counter.
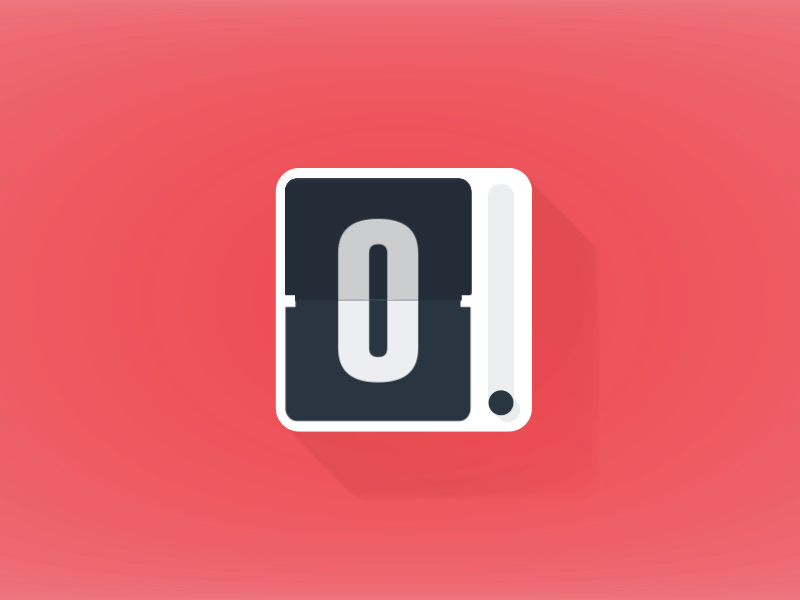
A counter becomes everything when we want to perform an action only a certain number of times.
Here is the syntax of the "for" loop with its "counter" mechanism:
for(setUpCounter; conditionalStatementToTrackCounter; updateCounter){
//Loop Body: the code for performing the action goes here
//Loop body can accommodate any number of lines of code inside it
//This loop body is entered only if the conditional statement returns true
}
As you can see, the "for" loop has four parts:
- Setting up a counter
- A conditional statement to track how many times an action has been performed
- Loop Body to perform the action
- Updating the counter for repeating the action
1) Setting up a counter
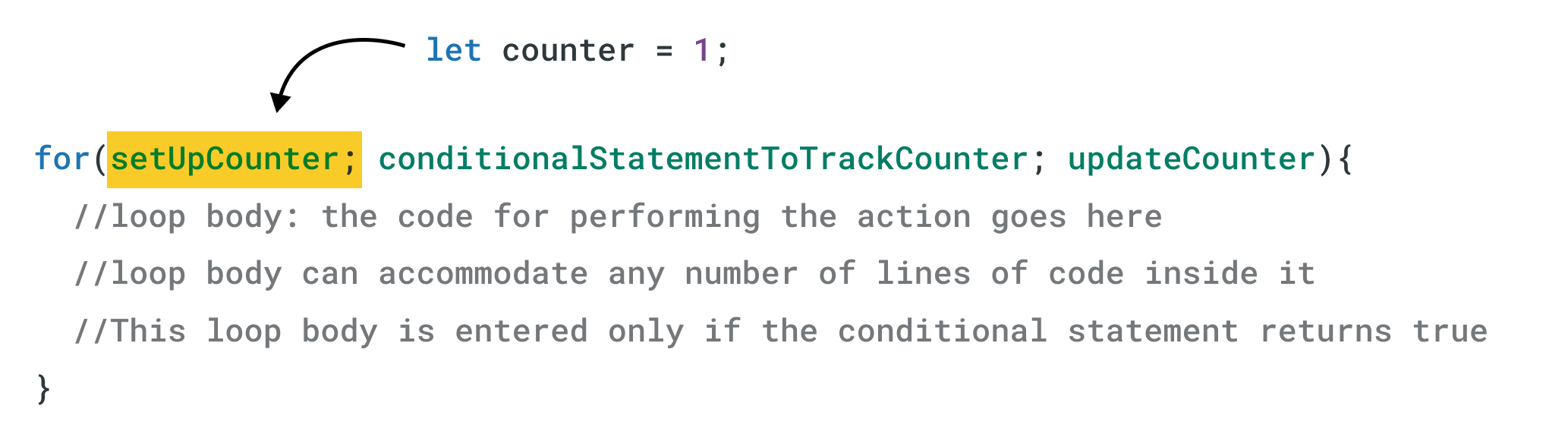
When I say "set up a counter", it just means creating a variable where you can store the initial value of a counter:
let counter = 1;
//or
let i = 1;
//or
let adkasjdkl = 1; // :D
Whenever we start counting for some reason, we usually start counting as 1, 2, 3, 4, 5, etc.
So, the initial value of a counter is usually 1
.
2) A conditional statement to track how many times an action has been performed and then terminate the loop
The next part of a "for" loop is providing a conditional statement.
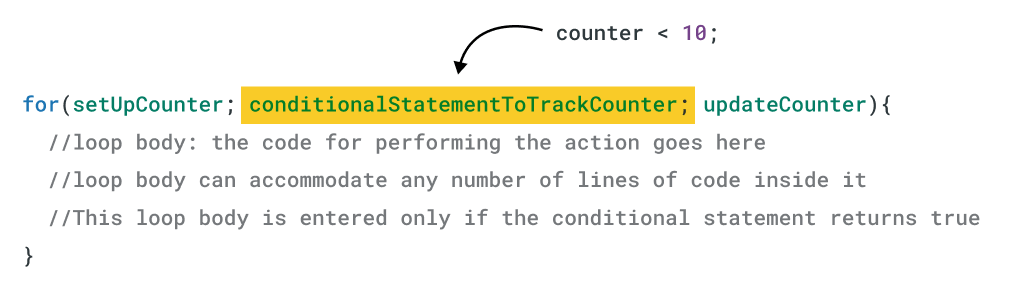
The conditional statement you provide decides how many times a loop should run (how many times an action must be performed).
It is also responsible for terminating the loop once it has run for a certain number of times.
3) "Loop Body" to perform the action
The loop body is the place where you write code for performing an action repeatedly.
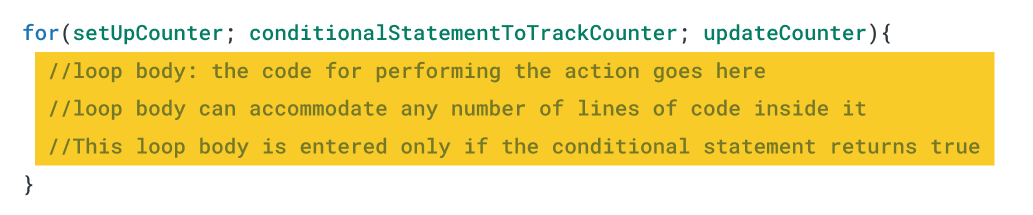
Depending on the conditional statement, the code inside the loop body will be executed a certain number of times.
4) Updating the counter to take the loop forward or exiting the loop
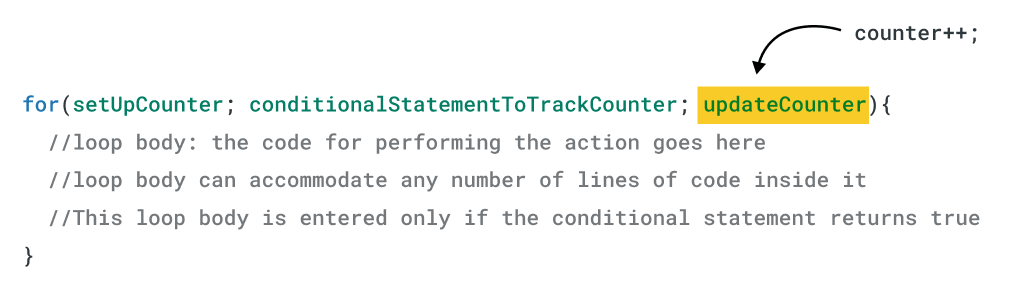
And when I say updating the counter, it technically means:
- Incrementing the value stored inside the counter variable (incrementing counter)
- Or, decrementing it
Updating the counter will help us answer the question: How many times have we performed the action so far?
Once we have implemented the action a certain number of times, the counter will cross the limit set, and the "for" loop will stop performing the action (exits the loop).
The "for" loop knows that the counter has crossed its limit by using a conditional statement to check the current value of the counter.
This is better shown than explained by implementing our task...
Log "hello" to the browser console 10 times
Here is the solution:
for(let counter = 1; counter <= 10; counter++){
//execute the below line of code ten times
console.log("hello");
}
//Javascript execution comes here after it exits out of the loop flow
console.log("Finished logging 10 messages to the browser console");
The above "for" loop is designed to run the code inside the "loop body" only 10
times because:
- We have set up the initial counter value as
1
. This means we want to start counting from1
. - Next, we have provided a conditional statement
counter <= 10
. This says that we should run the loop 10 times only. - We are updating the counter after every cycle of the loop so that the code inside the loop body will be executed 10 times.
Here is the final output of executing the above code inside the browser console:
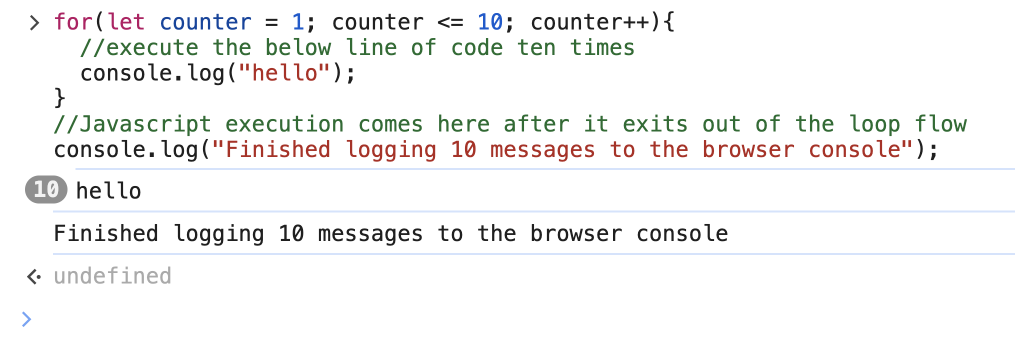
Now, let's go through it step by step in ultra detail.
Iteration 1, Step 1: Set up the counter
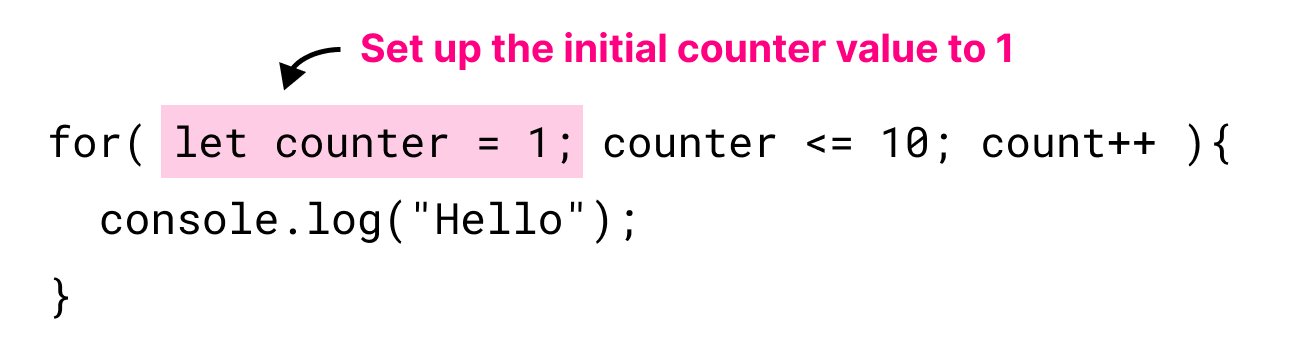
The first iteration of the loop mechanism begins by setting up the initial counter value as 1
.
This is achieved by declaring a variable called counter
and storing the value of 1
inside it.
This means the current value of the counter is 1
.
counter
variable anything you want. For example, most people use i
as the name of the counter variable. Also, you can start the counter at any value, such as 0, 2, 3, etc. You'll understand the reason behind this in the upcoming lessons.Iteration 1, Step 2: Check if the current value of the counter is less than or equal to 10
Next, the loop mechanism will shift its focus to the condition statement.
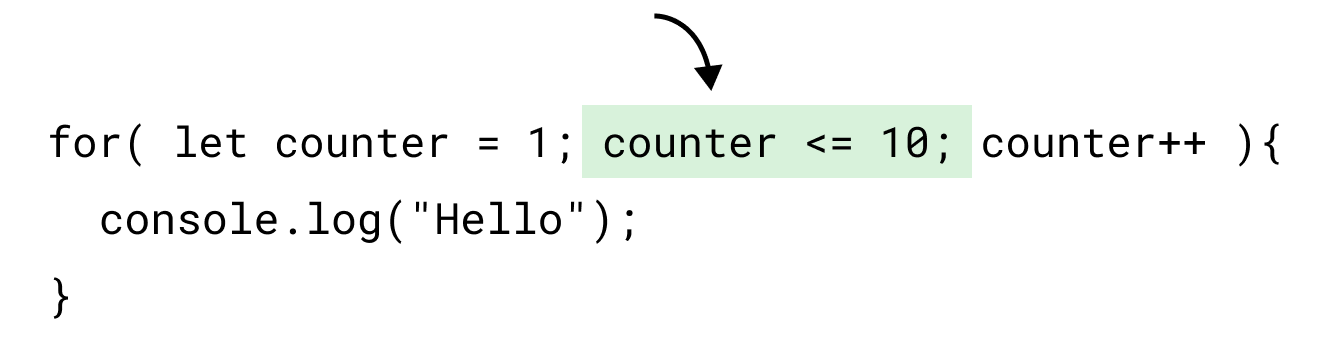
As a programmer who wrote the code, we know that the loop has just begun, and the current counter value is still 1
.
But the Javascript engine doesn't work like that because it doesn't know how to analyze a situation like humans.
So, even if the loop is running for the first time, the "for" loop mechanism still checks whether the current value of the counter is less than or equal to 10
:
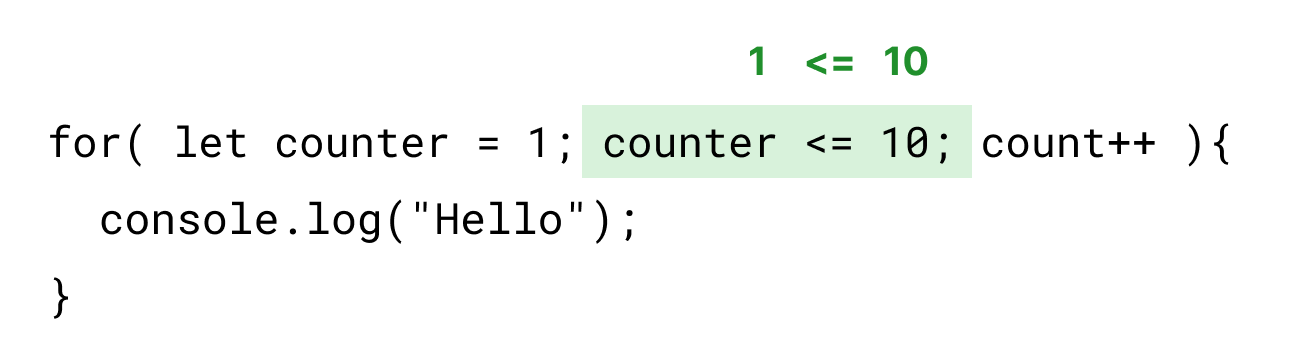
If it is, only then it will execute the code inside the loop body.
In our case, because this is the first iteration of our "for" loop, the current value of the counter is 1
.
Is 1
less than or equal to 10
?
Yes, it is.
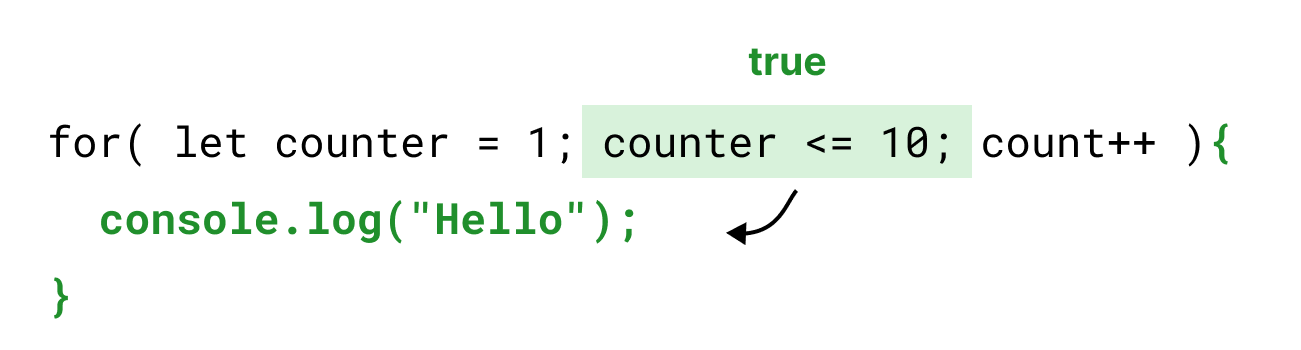
So, the conditional statement counter <= 10
resolves to true, and the code inside the loop body will be executed.
This results in the first "hello" message being logged to the browser console.
counter < 11
because it still checks whether the current counter value is less than or equal to 10
. Simply put, mathematically speaking, counter < 11
conveys the same meaning as counter <= 10
.Iteration 1, Step 3: Update the counter
Once all the code inside the loop body is executed, it technically means the first iteration is over.
So, the loop mechanism shifts its focus to updating the counter:
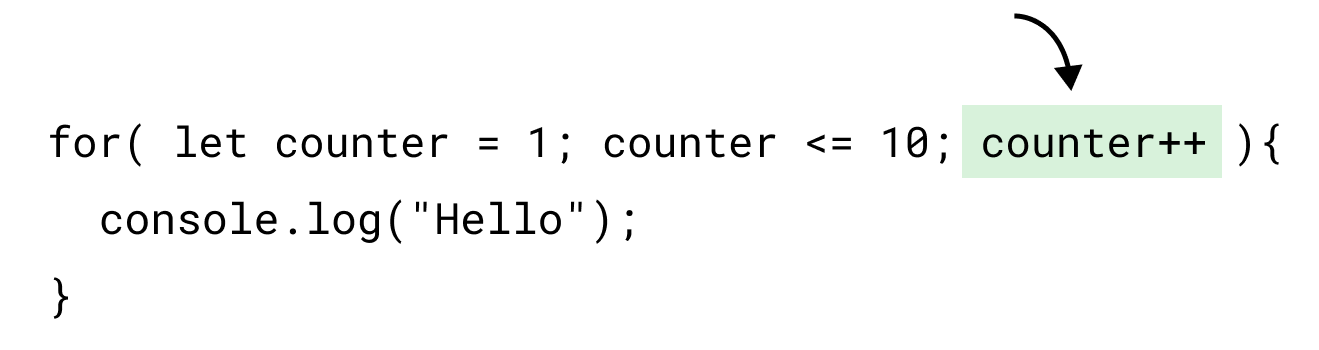
If you notice, we are incrementing the value stored inside the counter
variable using the increment operator (++
).
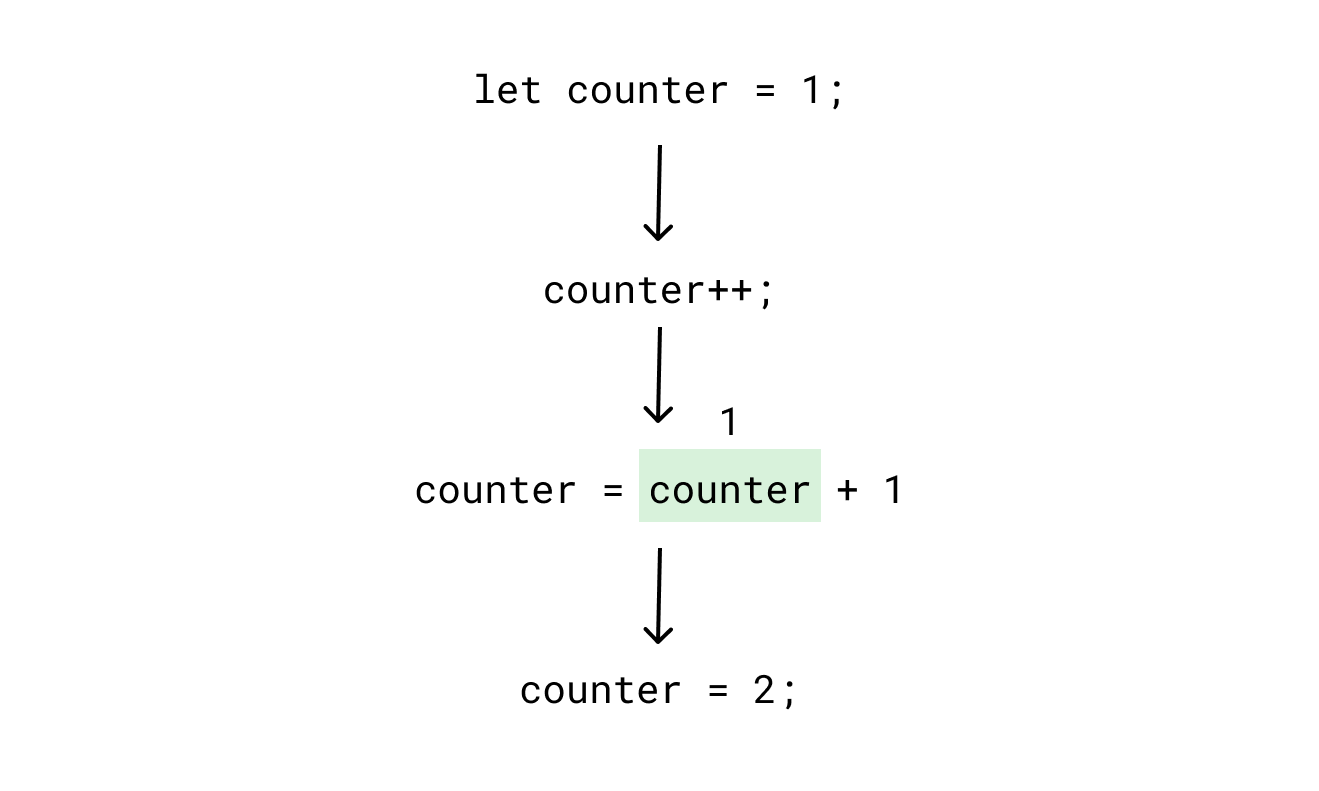
The increment operator (++
) takes the current value of the variable it is being applied to and increments its value by 1
.
In our case, the current value stored inside counter
variable is 1
right?
So, executing counter++
will update the current counter value to 2
.
In other words, executing counter++
is the same as:
counter = counter + 1;
So, the value stored inside the counter
variable is now 2
.
This also means our loop counter is now updated to 2
.
The loop now moves on to its second iteration.
Iteration 2, Step 1: Check if the current value of the counter is less than or equal to 10
During the second iteration, the loop mechanism will ignore the counter set-up part because it was done during the first iteration.
In fact, it will be ignored for the rest of the iterations because the counter
variable declaration only happens during the first iteration:
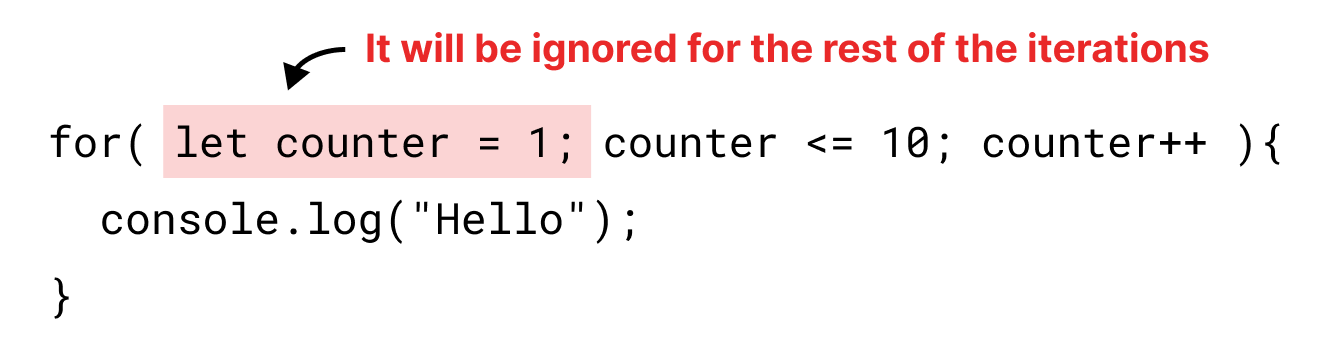
And the loop mechanism directly jumps to the conditional statement again:
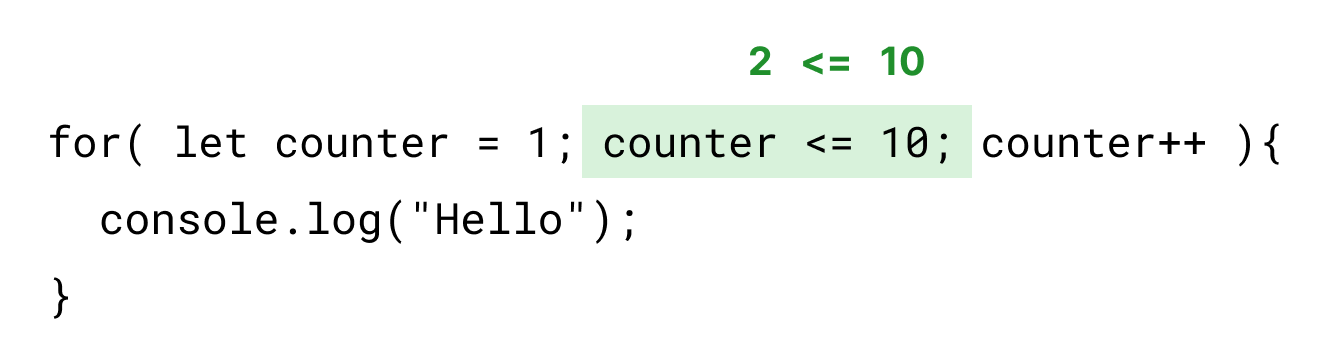
Because we have incremented the counter during the previous iteration, the current value of the counter is 2
, right?
Is 2
less than or equal to 10
?
True, it is.
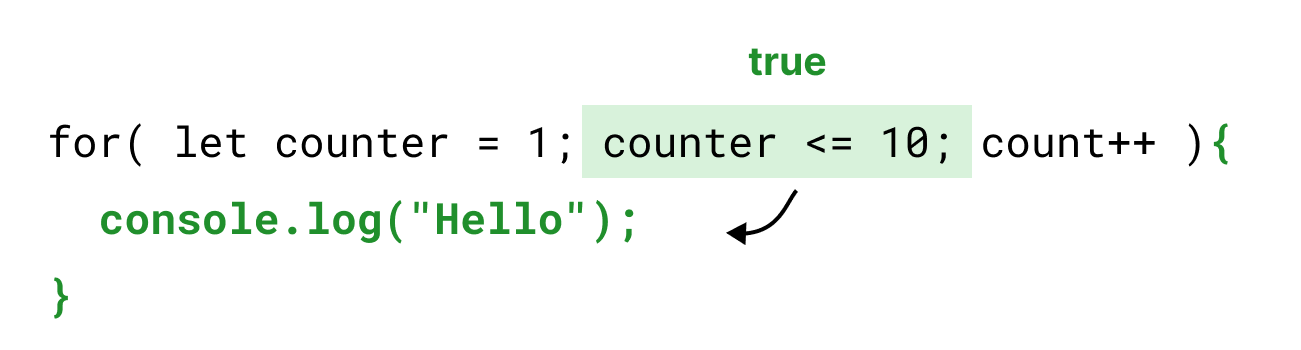
So the condition statement resolves to true, and the code inside the loop body will get executed for the second time, and this results in the second "hello" message being logged to the browser console.
Iteration 2, Step 2: Update the counter
Once all the code inside the loop body is executed, the loop mechanism shifts its focus to updating the counter again:
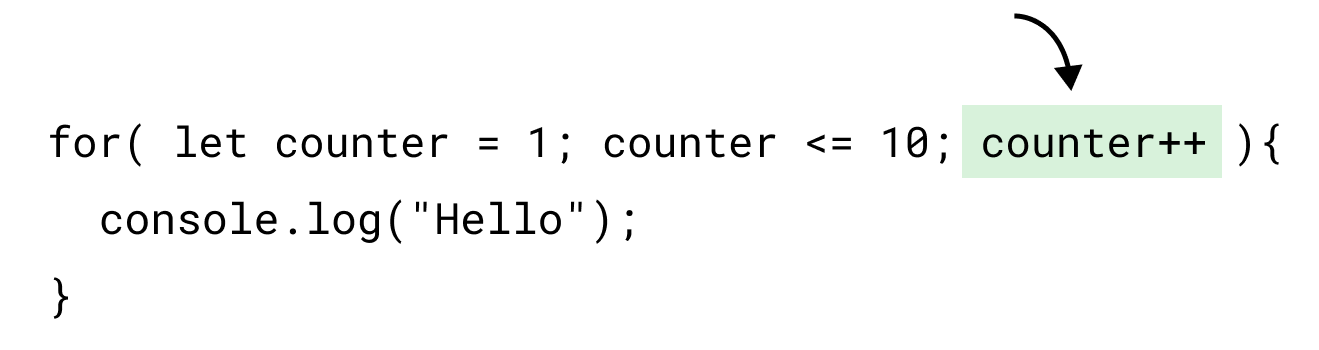
The current value of the counter is 2
, right?
So, executing counter++
will update the counter value to 3
:
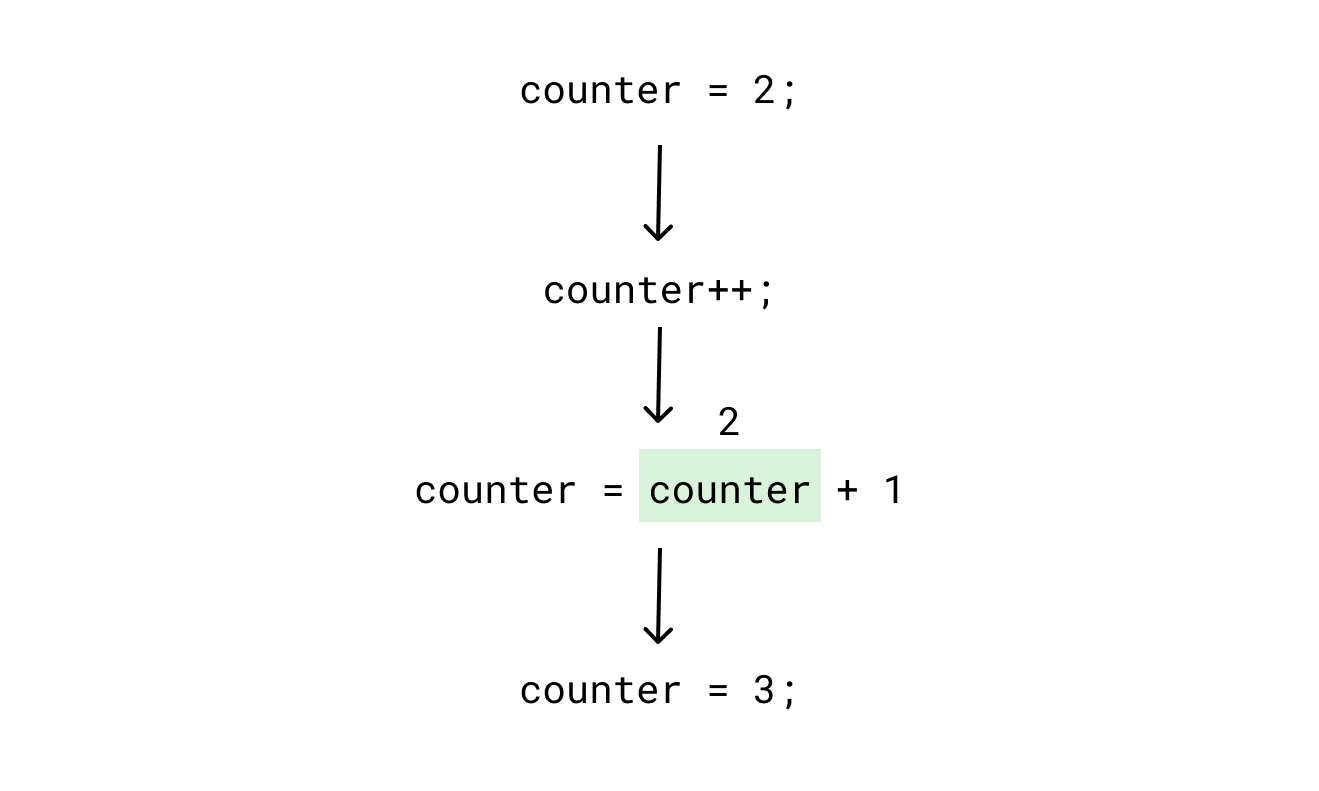
This also means our loop counter is now updated to 3
.
And as soon as the counter is updated, iteration 2 (second loop cycle) is now completed.
The loop now moves on to its third iteration and checks whether the current counter value is less than or equal to 10.
Iteration 3, Step 1: Check if the current value of the counter is less than or equal to 10
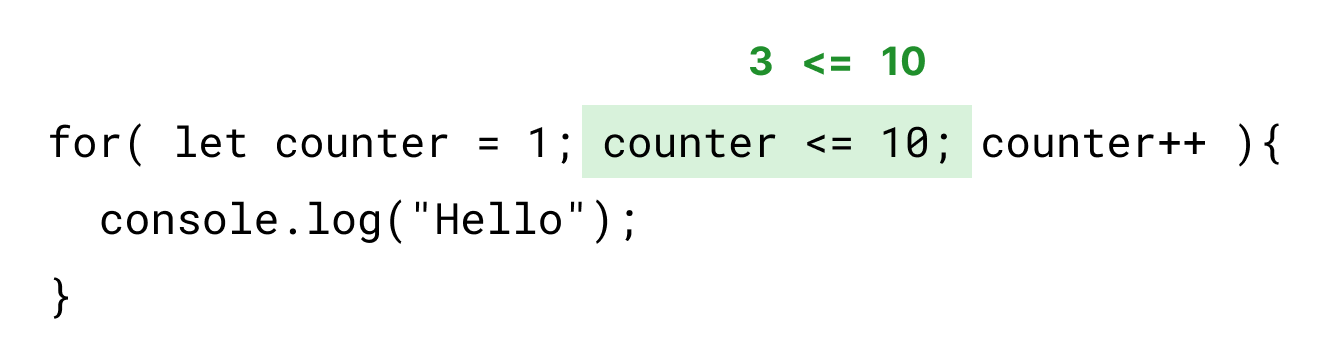
Because we have incremented the counter during the previous iteration, the current value of the counter is 3
, right?
Is 3
less than or equal to 10
?
True, it is.
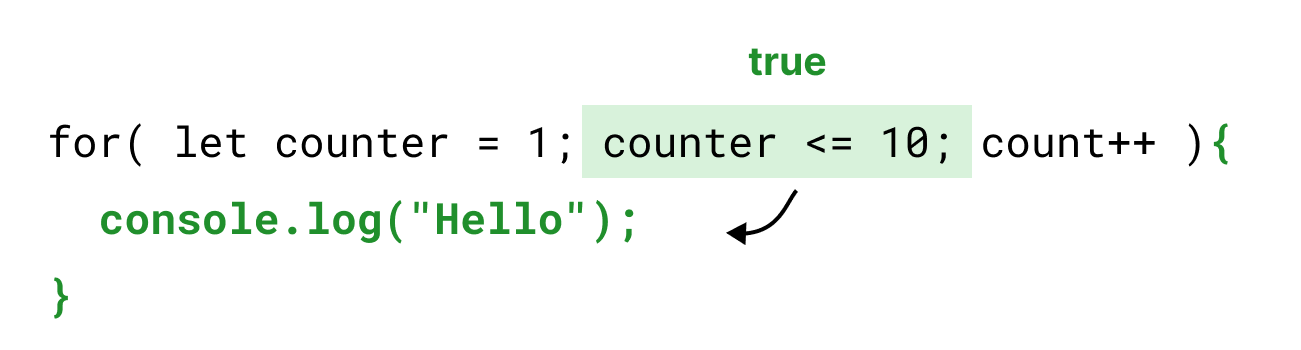
So the condition statement resolves to true, and the code inside the loop body will get executed for the third time, and this results in the third "hello" message being logged to the browser console.
You get the idea, right?
The same process repeats for the rest of the iterations
So, let's fast forward to iteration 10 (last iteration).
Iteration 10, Step 1: Check if the current value of the counter is less than or equal to 10
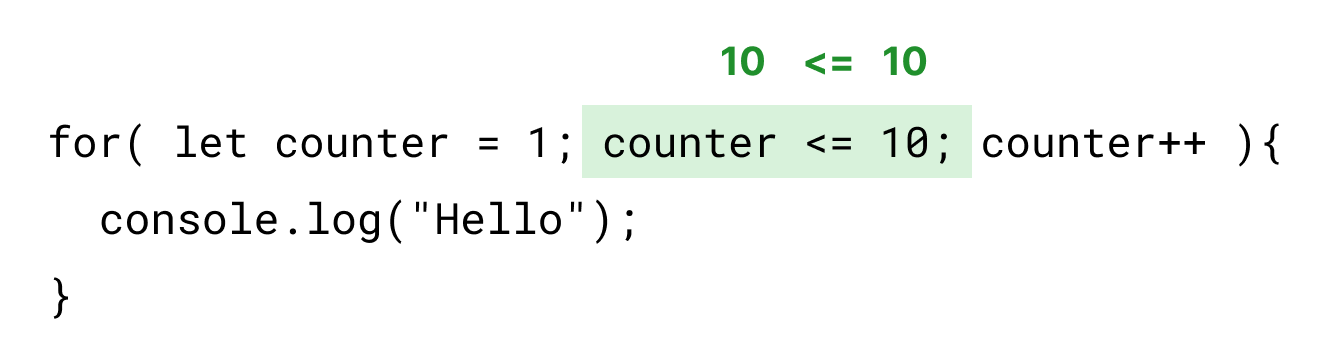
Because we have incremented the counter during all previous iterations (4,5,6,7,8,9), the current value of the counter would be 10
, right?
The counter got incremented to 10
after the end of the 9th iteration.
Is 10
less than or equal to 10
?
True, it is.
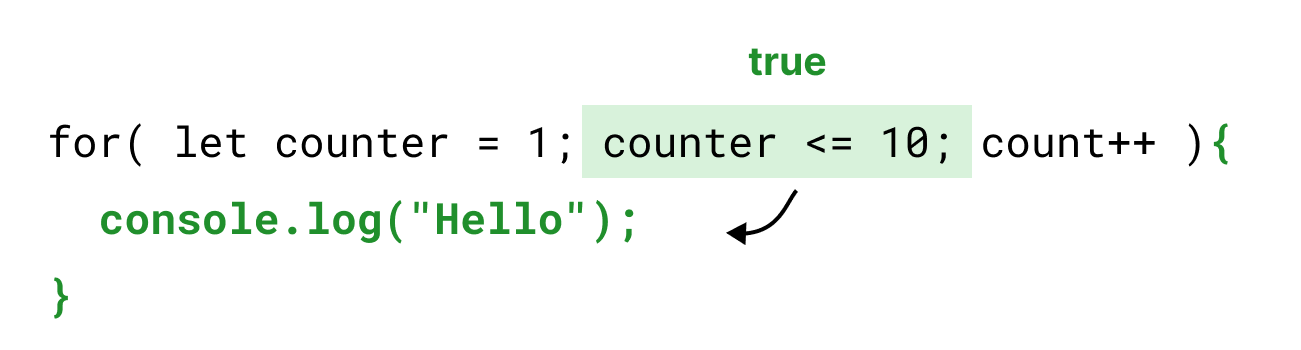
So the condition statement resolves to true, and the code inside the loop body will get executed for the tenth time (last time), and this results in the tenth "hello" message (last message) being logged to the browser console.
Iteration 10, Step 2: Update the counter
Once all the code inside the loop body is executed, the loop mechanism shifts its focus to updating the counter again:
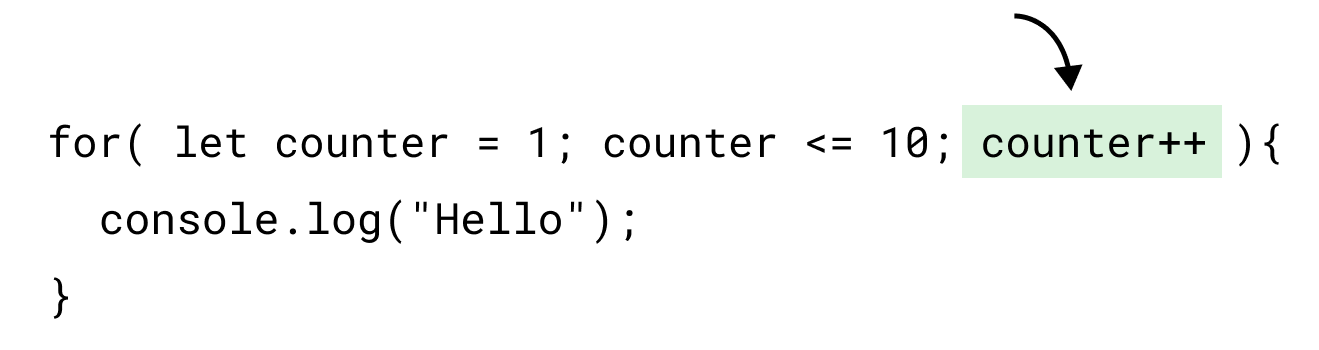
The current value of the counter is 10
, right?
So, executing counter++
will update the counter value to 11
:
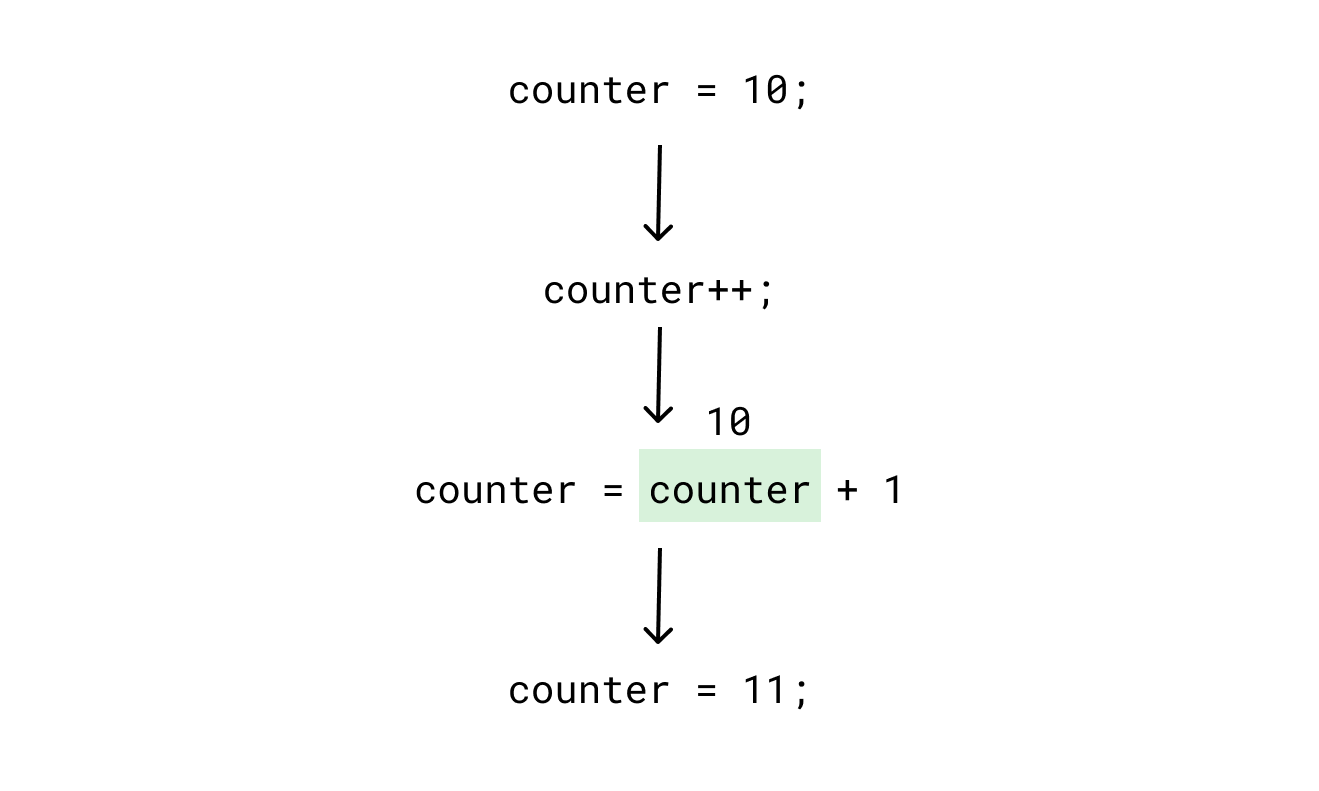
This also means our loop counter is now updated to 11
.
And as soon as the counter is updated, iteration 10 (last loop cycle) is now completed.
The loop now tries to move on to its eleventh iteration and checks whether the current counter value is less than or equal to 10.
Iteration 11, Step 1: Checking the condition after the loop ends
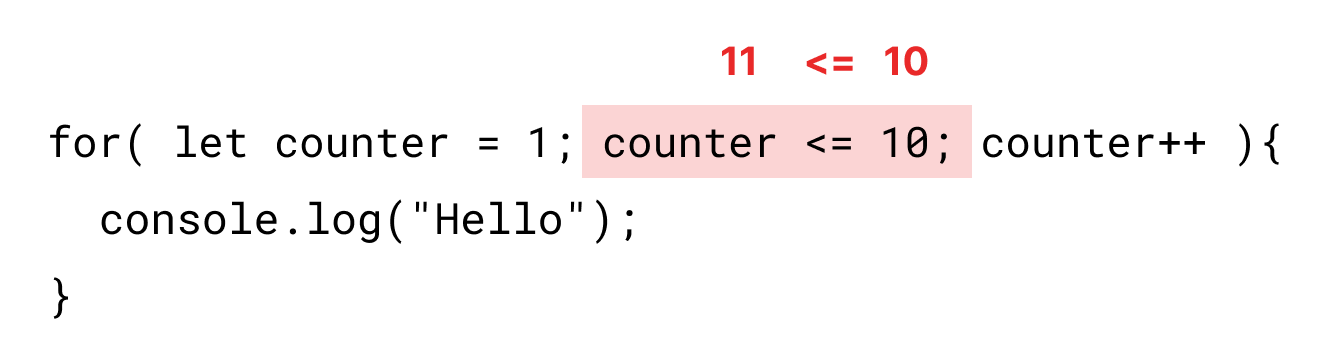
Because we have incremented the counter during the previous iteration, the current value of the counter is 11
, right?
Is 11
less than or equal to 10
?
Nope! False!
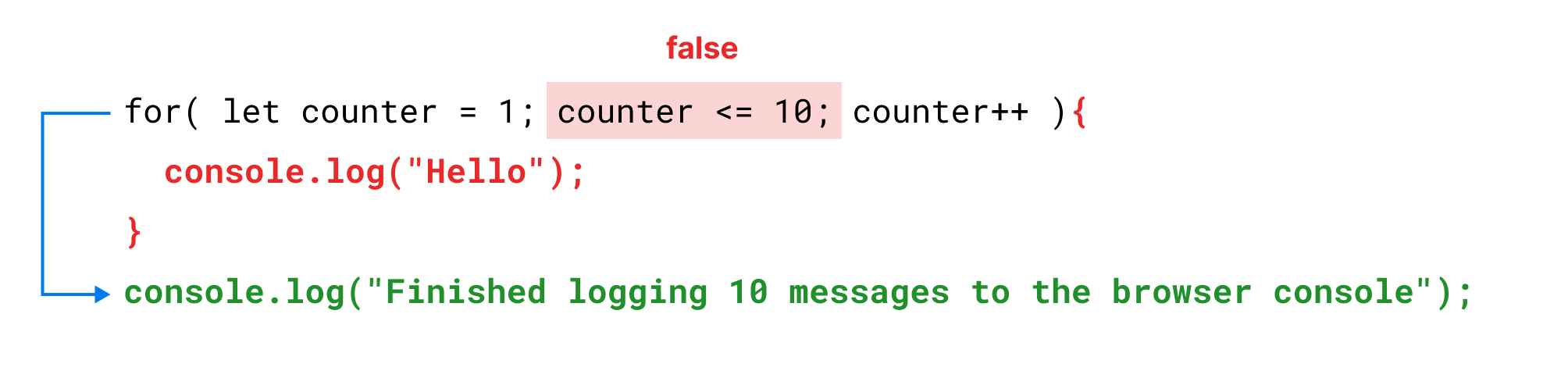
As soon as the counter value exceeds 10, the condition statement resolves to false, and the loop body will not get executed.
This is because the code flow exits the "for" loop altogether when the condition statement returns false and starts executing the remaining code that comes after the for loop.
Simply put, the loop was terminated, and the Javascript engine moved on to the code that came after the loop.
Currently, there is only one console.log
call after coming out of the loop:
console.log("Finished logging 10 messages to the browser console");
So it will get executed.
Because of this, in the browser console, once the 10 "hello" messages have been logged, you'll see the message "Finished logging 10 messages to the browser console":
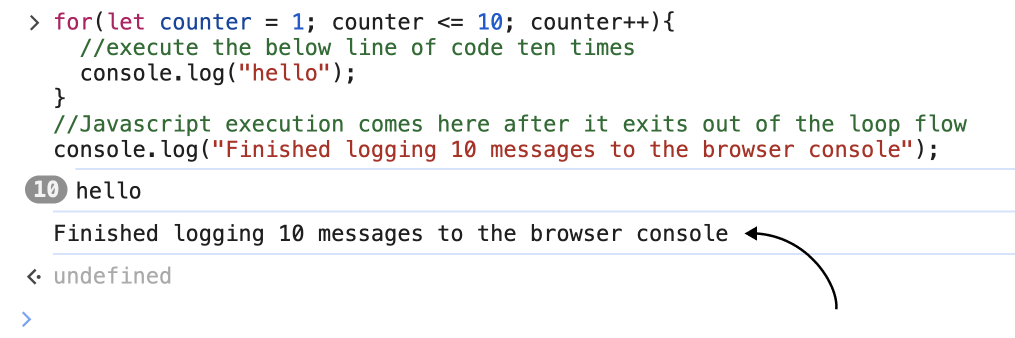
That's all.
That's how the "for" loop works.
In the next lesson, we will increase the complexity by outputting the counter
value inside the console and accessing items of an array using the same counter variable.