Understanding If/Else statements
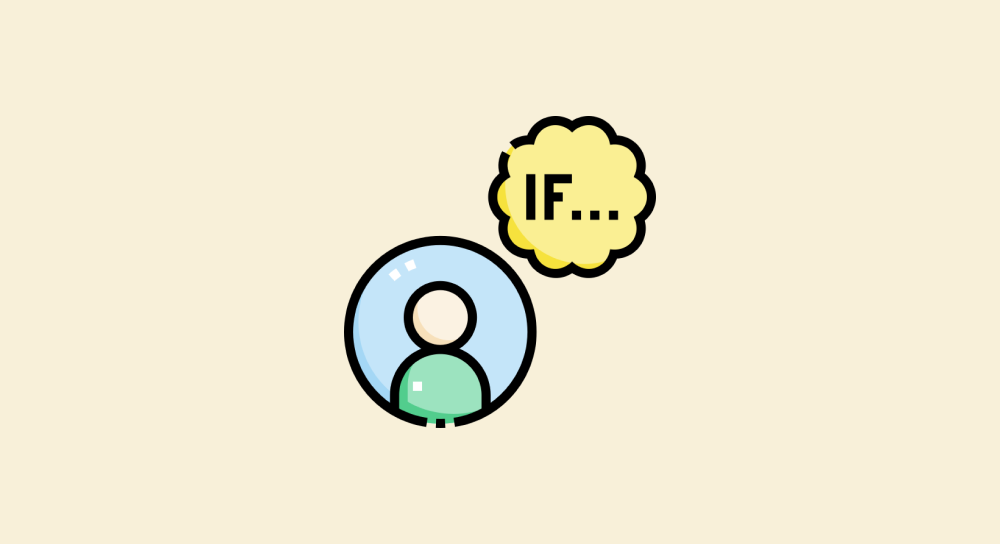
In the real world, we always take decisions based on some condition.
For example, let's just say we are driving on a highway.
- If we are driving during the night, we turn on a car's headlights.
- Else, If we are driving during the day, we don't turn on the headlights.
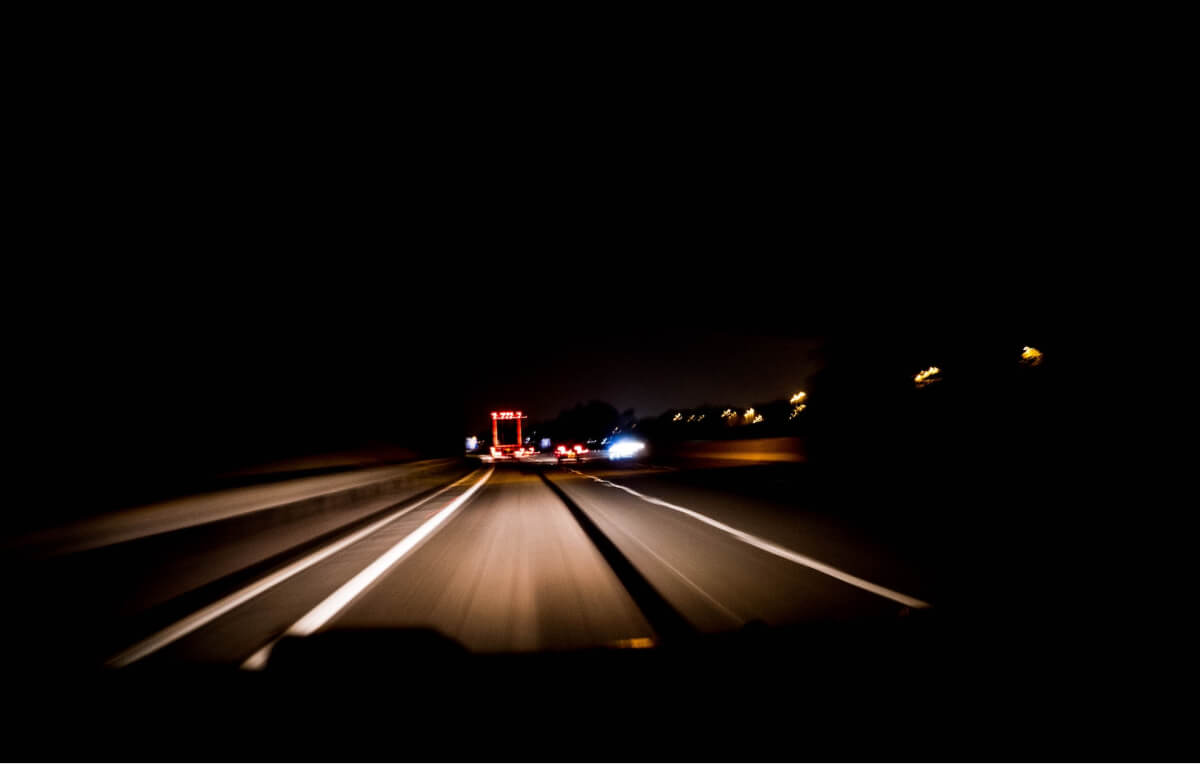
In other words:
- If the answer to your question is "yes" (it's dark), you do one thing.
- But if the answer is "no" (it's not dark), you do something else.
The same logic is applicable to the programs you code as well.
As a programmer, sometimes, you need to take decisions based on a condition.
For example, when it comes to the "Show/Hide password" functionality:
- If the "Show password" field is checked, then the password should be revealed
- Else the password should be concealed (hidden)
The "Show password" field being checked is the condition for showing or hiding the password.
This sounds simple theoretically.
But programmatically, how do we check if a particular condition is met?
We know the answer now. We can use boolean expressions to check for a particular condition.
The important question here is, how do we do something if a particular condition is met?
This is where If/Else statements come in Javascript.
The definition
The If/Else conditional statement helps you take decisions in your code based on a condition.
The main purpose of the If/Else conditional statement is to :
- Let you check for a condition
- And if the condition is met, it allows to you do something
- Else, it allows you to do something else.
In other words, checking for a condition is like asking a question and then doing different things depending on the answer.
Here is the syntax:
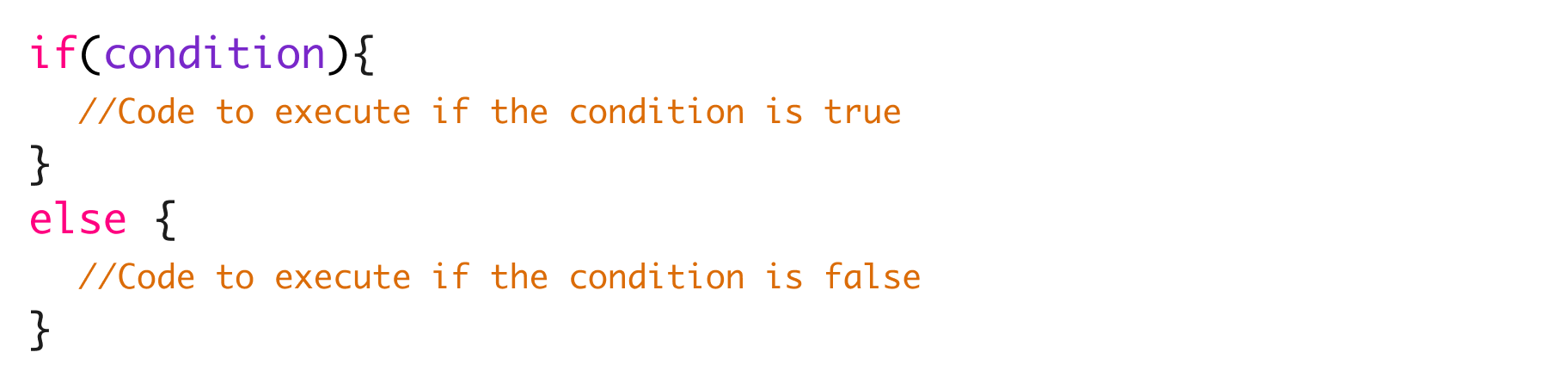
If you notice, there are three parts to an If/Else statement:
- The condition - an expression (preferably a boolean expression)
- The "if" block
- The "else" block
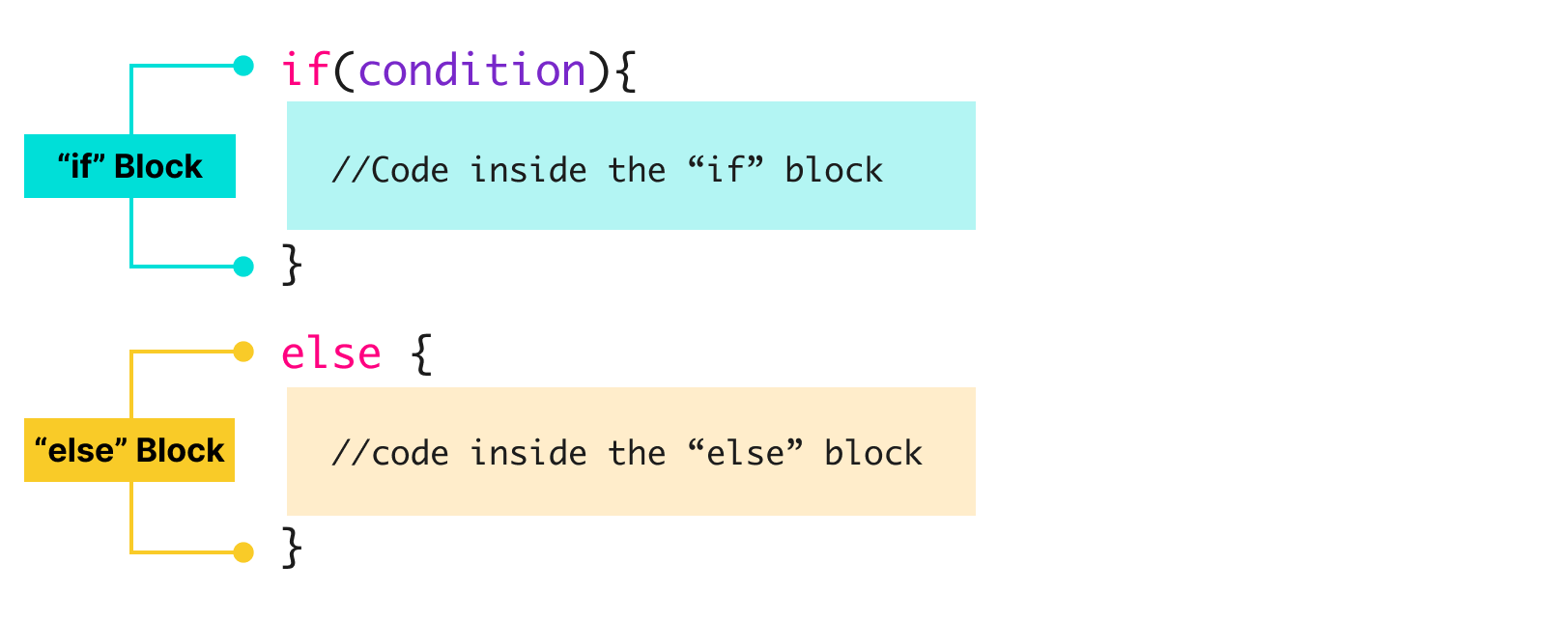
Introducing the condition
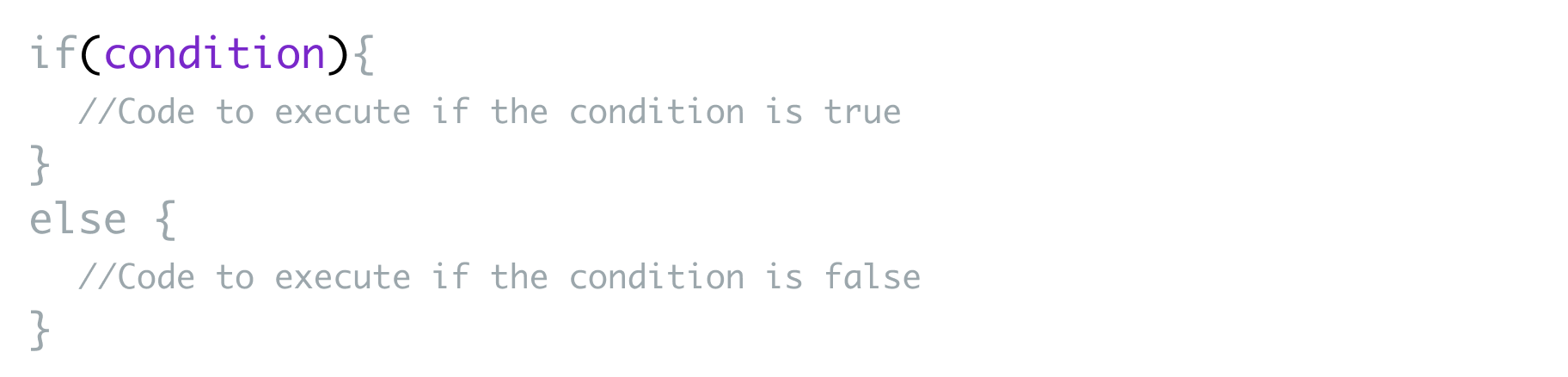
For an If/else statement, it is mandatory to provide a condition.
Ideally, the condition provided must be an expression that resolves to either true or false.
If the condition resolves to true, the code inside the "if" block will get executed and the code inside the "Else" block is skipped from the execution.
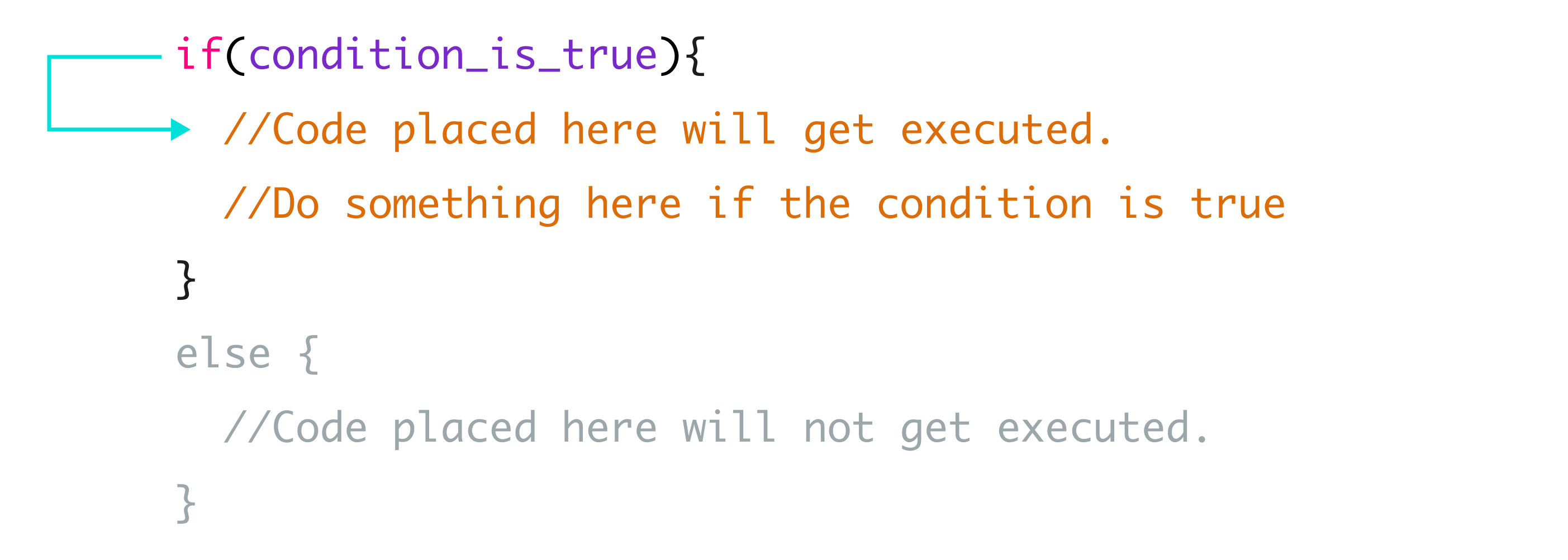
But if the condition is not true, then we will execute the code inside the "else" block directly by skipping the code inside the "If" block.
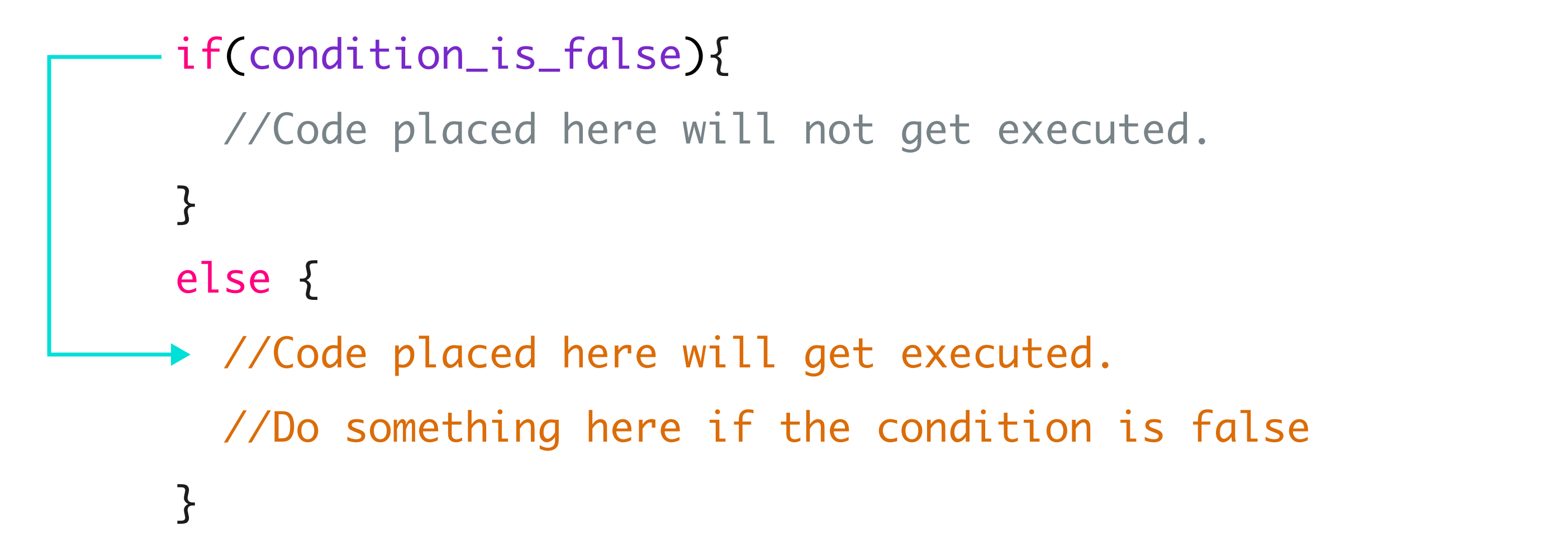
As simple as that.
This is better asked than explained
Come on, open up a new tab in the browser, and type the following code in the console but don't hit enter:
if(10 > 5){
console.log("The condition is true");
} else {
console.log("The condition is false");
}
In the above code, we have provided the expression 10 > 5
as the expression.
Now tell me.
Which message would get logged to the console and why?
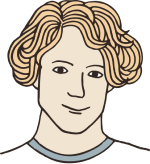
Bro, "The condition is true" will get printed because 10 is greater number than 5.
Correct!
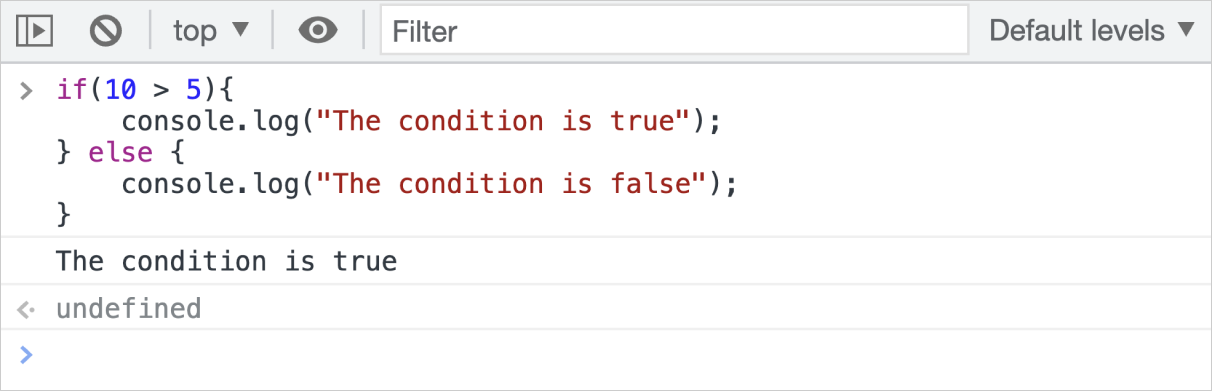
When the condition is true
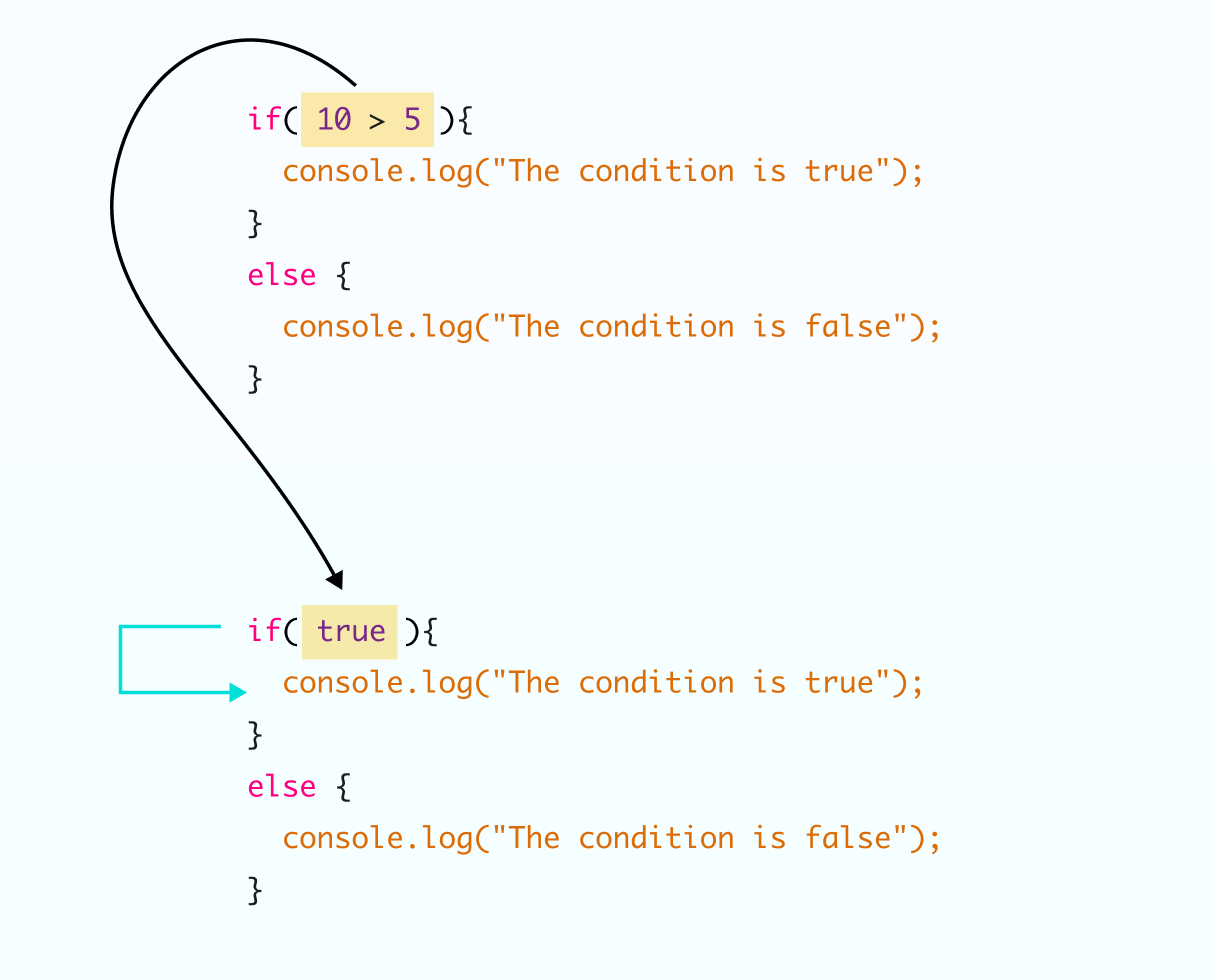
Basically:
- When javascript comes across the If statement, it first evaluates the condition (expression).
- In our case, when the condition
10 > 5
is executed, it produces the valuetrue
internally. - Because the condition turned out to be true, the javascript now executes the code inside the "If" block.
- As a result, the message "The condition is true" is getting logged inside the console.
When the condition is false
Now let's just say the condition is 22 < 5
.
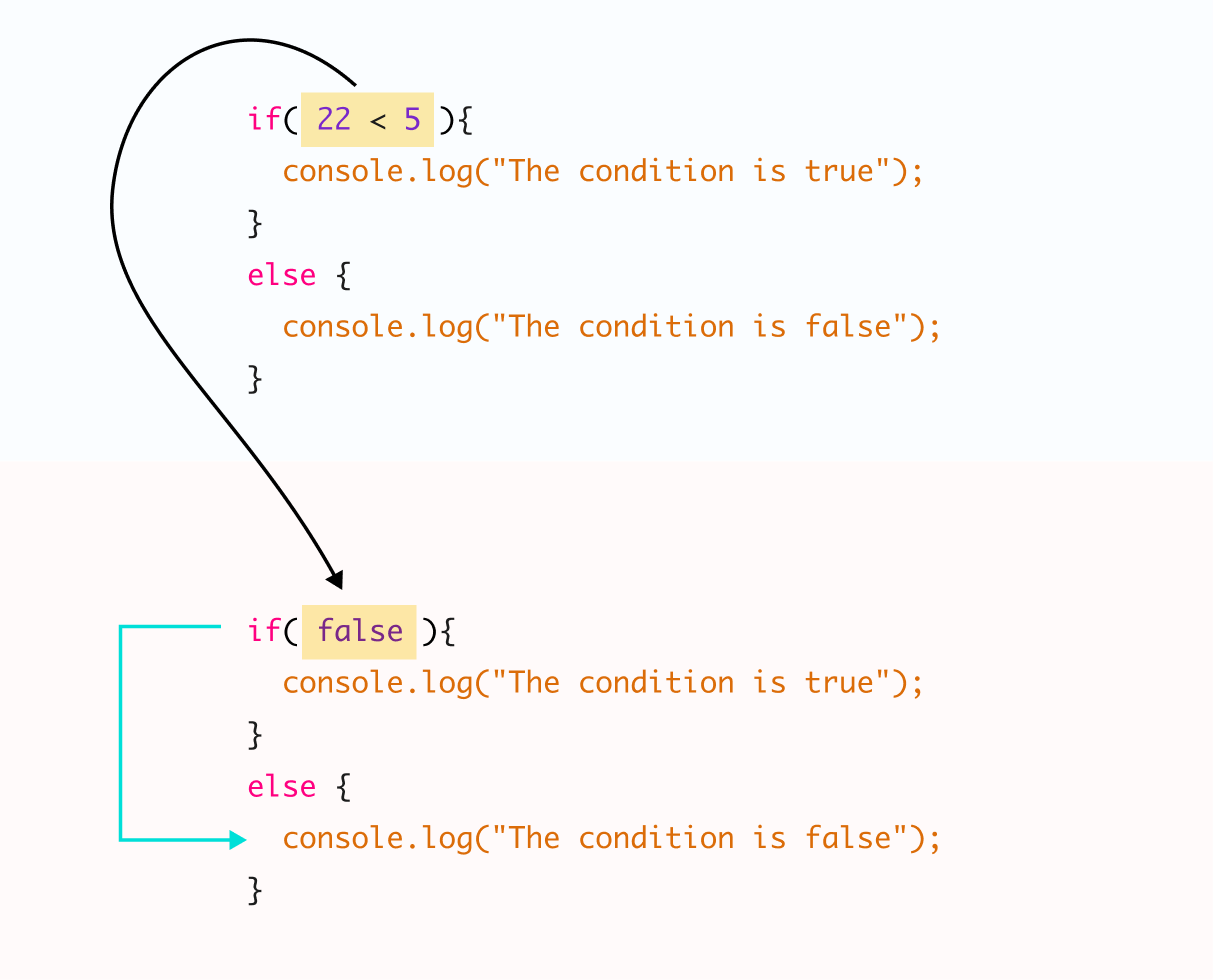
- When javascript comes across the If statement, it first evaluates the condition.
- So, when the condition
22 < 5
is executed, it produces a value offalse
because the number 22 is not smaller than 5. - Because the condition turned out to be false, the code inside the "Else" block is executed.
- As a result, the message "The condition is false" is getting logged inside the console.
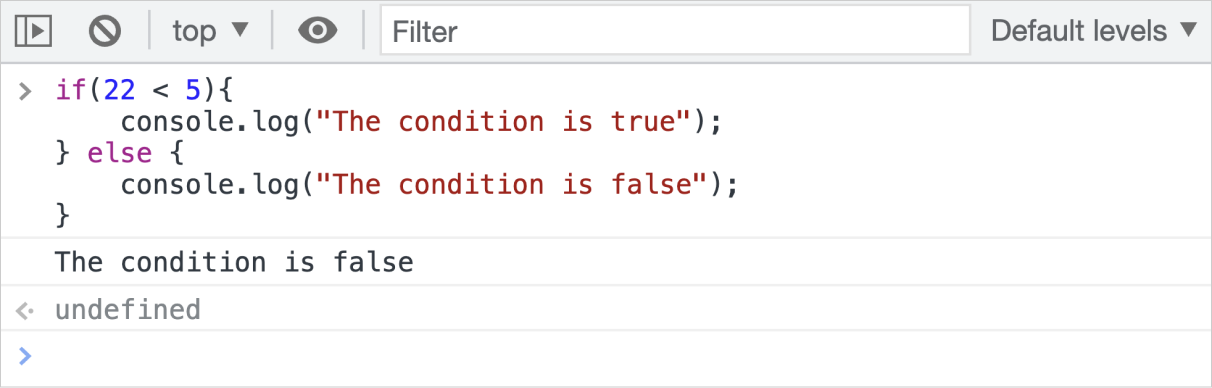
Anyway, if you don't understand the If/else statements yet, don't worry :)
It's okay. I felt the same way when I was getting started.
But I promise that things will get more clear when we start working on real-world projects and we will do that starting from the next lesson.