Understanding Function parameters (inputs)
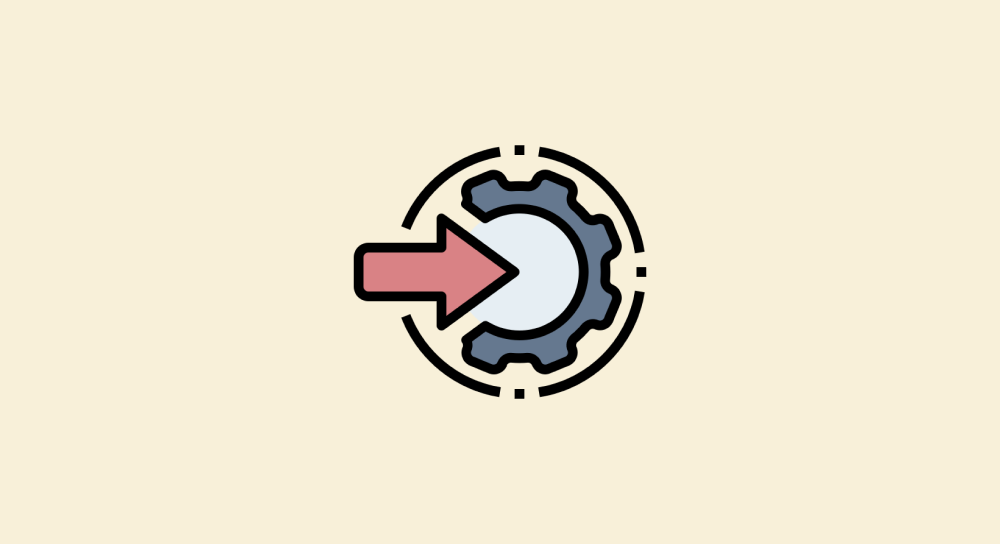
In the last lesson, we understood that:
- A Function is responsible for performing one task and one task only
- A Function has a name so that we can use it
- A Function can be triggered with
()
to make use of it
But the most important feature of a function
is the ability to accept inputs.
Function inputs will help us customize the behavior and, therefore, the output of the function
totally.
These inputs are also called "Parameters" in the programming world (not just Javascript).
Function parameters ( inputs )
A coffee vending machine is nothing without coffee beans, milk, and water, right?
They are needed to produce the final output ( coffee ), and we should provide them to the machine.
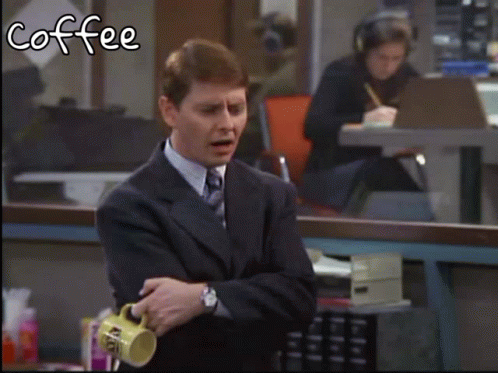
Similarly, A Function needs to be supplied with the necessary inputs to be helpful.
An input is nothing but a piece of data that the Function can use to produce some meaningful output.
Again, the best example is the alert
Function.
If you just call the alert()
Function with nothing inside the curly braces will trigger an empty alert box.
//This will trigger an empty alert box
alert();

An alert box with no message is not that useful, right?
So, it only makes sense if the alert
Function will allow us to provide a message to it somehow, right?
And the alert
function does just that. It accepts an input called message
.
alert(message);
As you can see, the input goes right in between the parentheses of the Function.
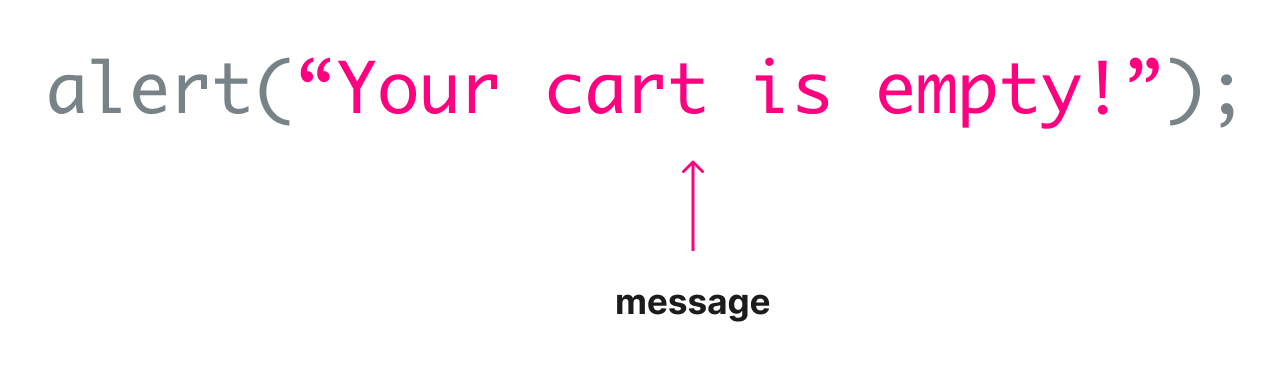
//A function being supplied with an input. In the case of the "alert" function, it's the message.
alert("Your cart is empty!");
Now, the browser will display the message "Your cart is empty!"
when it pops up the alert box.

Simple enough, right?
BuzzWord: Parameter
A parameter is nothing but an input that you provide to a function.
A function can have multiple parameters
In the case of alert
it accepts only one parameter.
And it makes sense because the alert
Function only cares about alerting the user with one message. Not multiple messages.
However, some functions need to be provided with multiple parameters to get the task done.
For example, let's just say we have a custom function that multiples two numbers:
//We created a custom function that multiples provided numbers
function multiplyTwoNumbers(number1, number2
// We are performing the multiplication and returning the result.
return number1 * number2;
}
//This call will not produce any meaningful output
multiplyTwoNumbers(10);
Don't worry about this code yet! This is a custom function
To the multiplyTwoNumbers
function, If we don't input at least two numbers we want to multiply, it produces no meaningful result.
So, you have to provide at least two numbers you want to multiply:
//We created a custom function that multiples provided numbers
function multiplyTwoNumbers(number1, number2
// We are performing the multiplication and returning the result.
return number1 * number2;
}
//This call will return back 20 as the result
multiplyTwoNumbers(2, 10);
Similarly, some functions might need three or more parameters to be useful.
Some functions accept no parameters at all
For example, the print
Function accepts no parameters.
It doesn't need any input because the only responsibility of the print
Function is to open up the printing options of the web page.
It doesn't do anything else.
Come on, try it out. Here is a quick exercise for you.
print()
in it and hit enter.You get the point, right?
But here is the actual question.
How do I know that the alert
Function accepts only one parameter?
How do I know that the print
Function doesn't accept any parameters at all?
We will figure out the answer in the next lesson.