Understanding and using Dot Notation Chaining a.k.a Object chaining
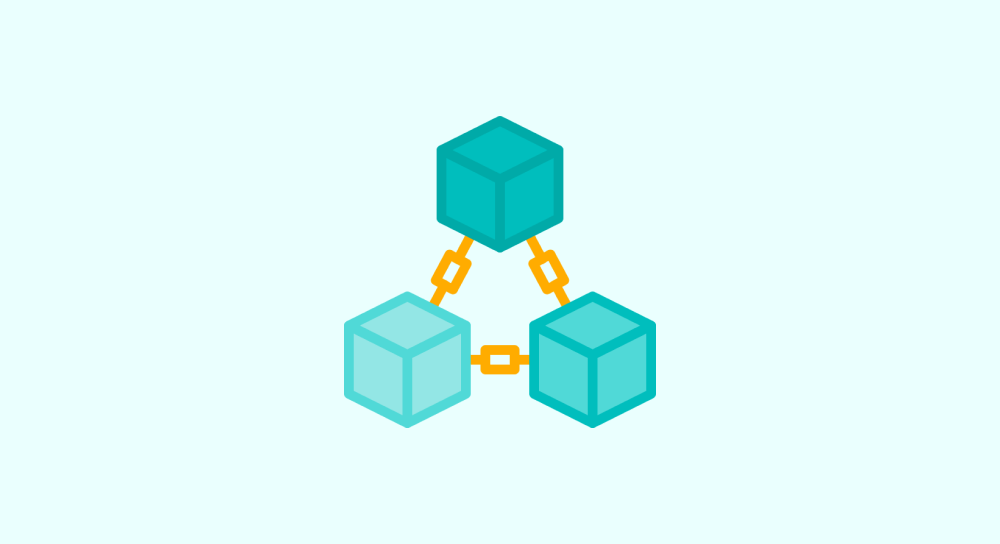
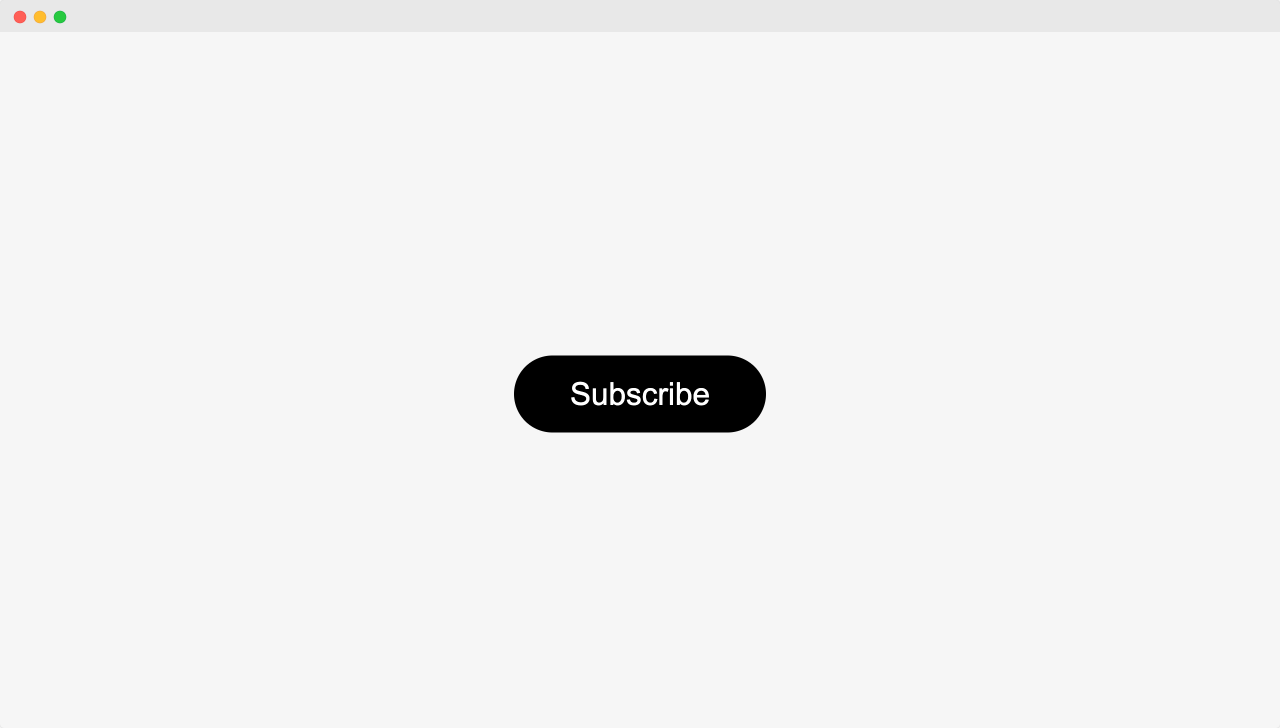
Imagine there is a button on a web page called "Subscribe" with the following HTML:
<button class="btn">Subscribe</button>
Next, to perform any action on the button, let's say, changing its text, you have to select the button and change its text:
const button = document.querySelector(".btn");
button.textContent = "Unsubscribe";
If you notice the above snippet:
- First, you select the button and save it inside a variable called
button
. An HTML object representing the button is now insidebutton
variable. - Then, using the HTML object located inside the
button
variable, you are accessing thetextContent
of the button and changing its text.
This is good.
But you can also achieve the same task by using "Dot Notation Chaining" a.k.a "Object chaining":
document.querySelector(".btn").textContent = "Unsubscribe";
It looks more concise and easy to read, doesn't it?
The difference here is:
- We are not saving the button selection to a variable. It saves us some time and memory space.
- And we are directly accessing the textContent method on the
document.querySelector()
method.
Here is how it works
document.querySelector(".btn").textContent = "Unsubscribe";
If you're seeing something like this for the first time, you might think that querySelector
method contains a property called textContent
.
But that is not the case.
When the Javascript engine comes across the above line of code, it first sees the document
object and access the querySelector
method on it using the "Dot Notation":
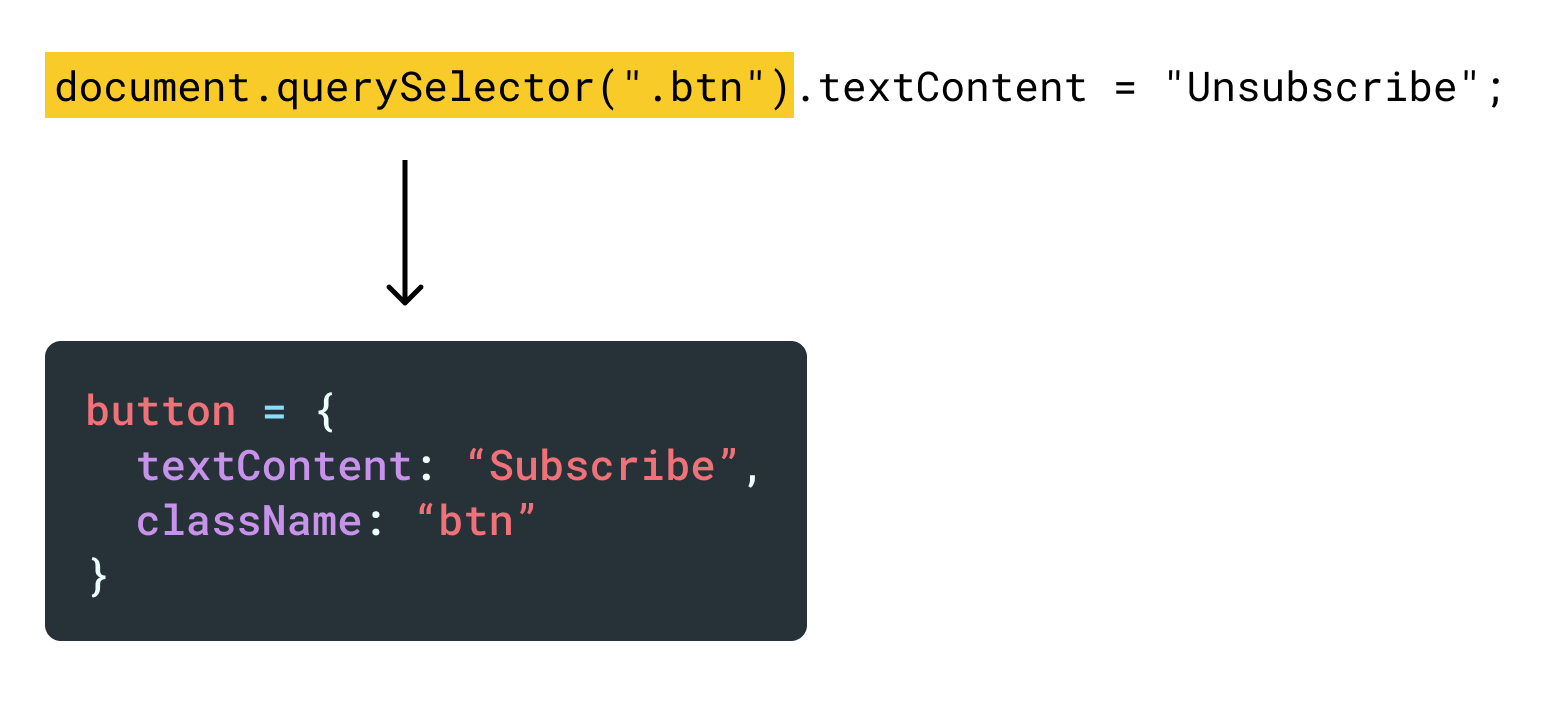
As a whole, document.querySelector(".btn")
returns a Javascript object that represents the HTML button selected:
button = {
textContent: "Subscribe",
className: "btn"
}
And it is on this returned button object we are calling the textContent
property:
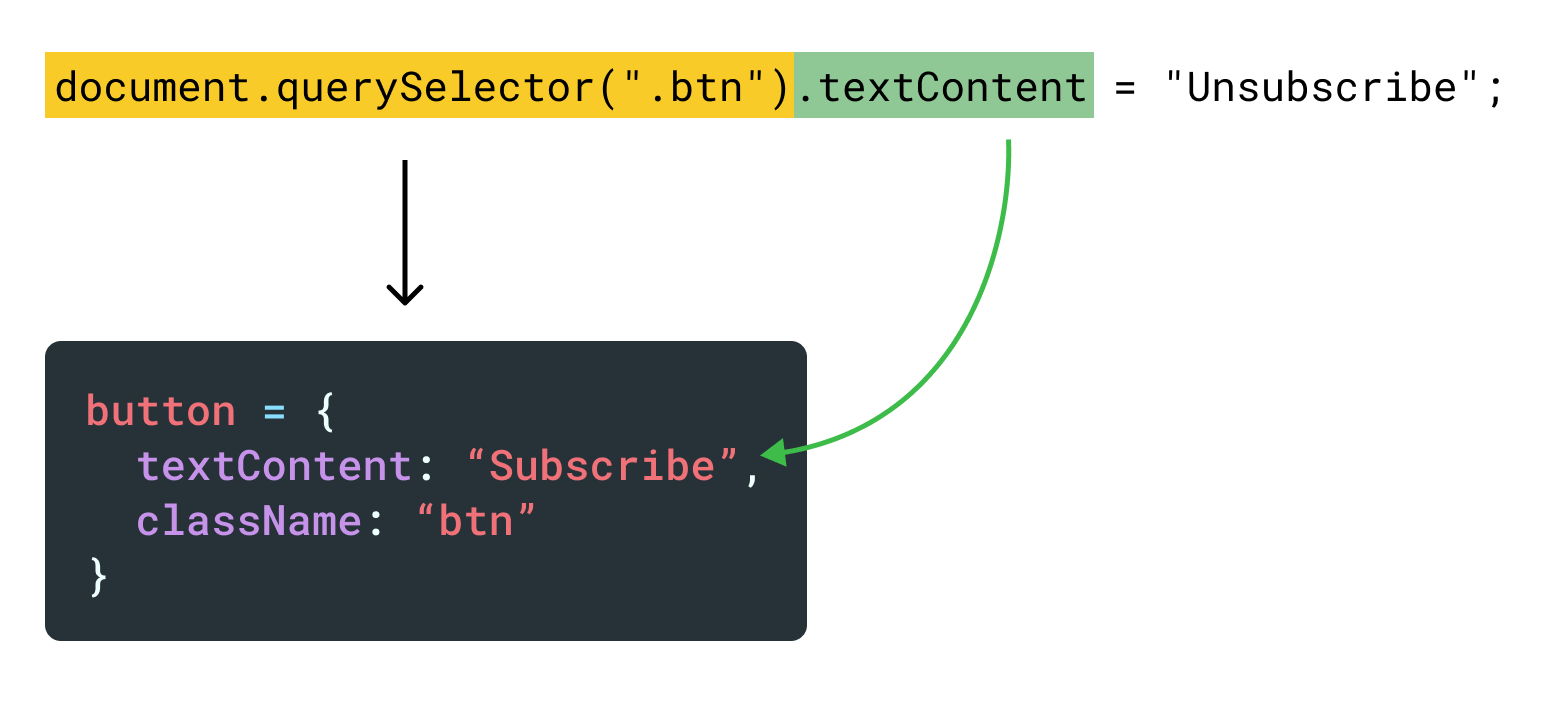
Simply put, after creating the button object, the Javascript engine immediately sees .textcontent
and realizes that:
"Oh, I am trying to access the textContent
property on the button object that was returned as a result of running document.querySelector(".btn")
"
And finally, it assigns the value of "Unsubscribe" to the button object's textContent
property:
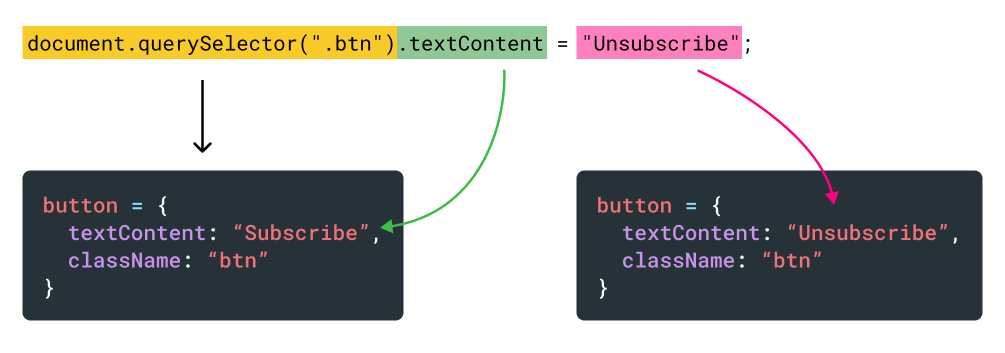
That's all.
Also, we call this approach "Dot Notation Chaining" because we are sequentially accessing properties and methods of multiple objects by connecting them with a dot:
document.querySelector(".btn").textContent = "Unsubscribe";
For example, in the above code:
- We are first accessing the
document
object. Then, using a dot, we are accessing its property calledquerySelector()
. This would result in another object that represents the HTML button element. - Then, on this ne button object, we are chaining another dot to access its
textContent
property.
Hence, the term "Dot Notation Chaining".
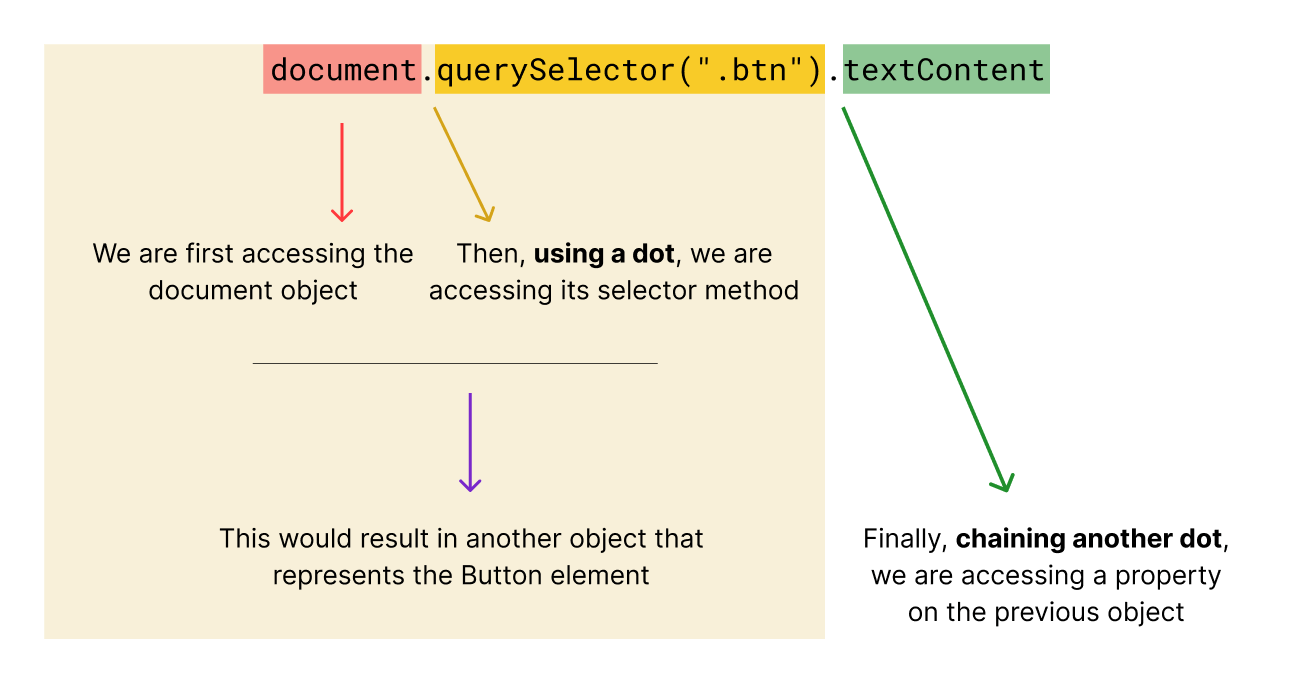
We did the same thing during the previous lesson, too
faqHeader.parentElement.classList.toggle("is-open");
In the above code:
1) faqHeader
is an object that represents the headline element of a specific FAQ:
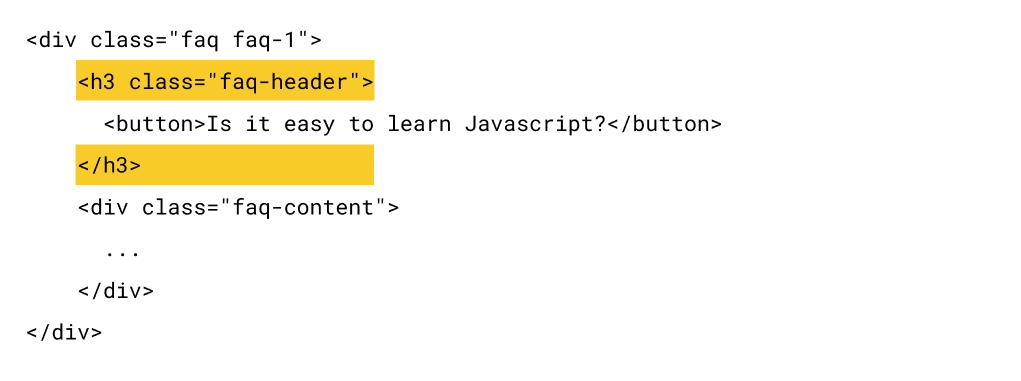
2) Because faqHeader
is an object representing the headline element, we are accessing its direct parent element using the dot notation and parentElement
property:
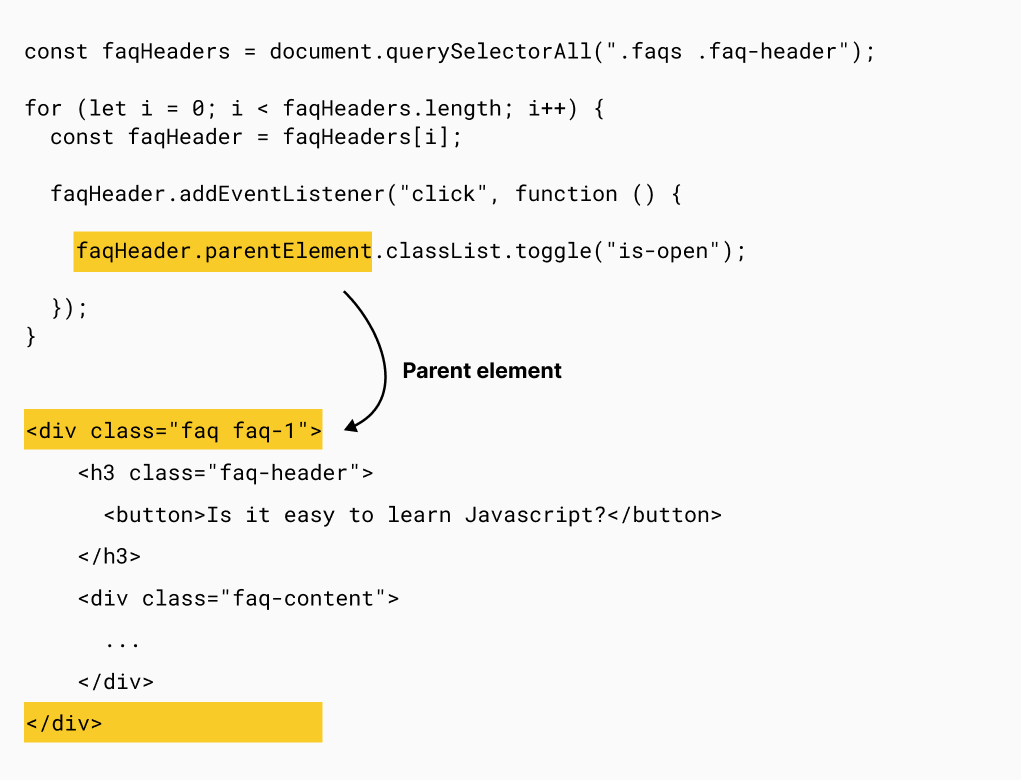
3) The result of running faqHeader.parentElement
is another object that represents the parent HTML element.
And you already know that:
- You can access the nested
classList
object on any HTML object - And then you can access the
toggle()
method on theclassList
object.
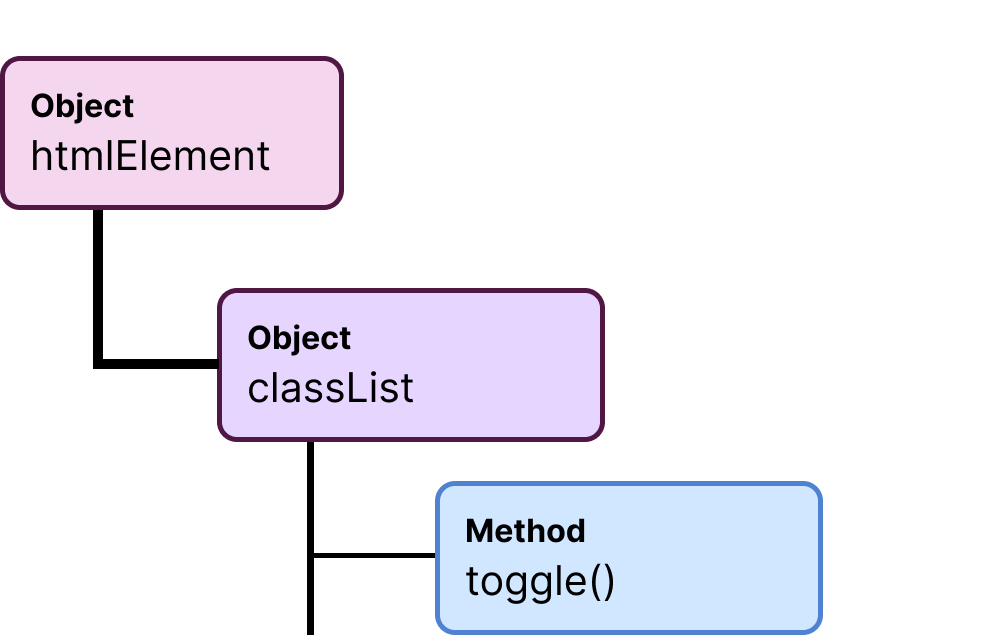
So, you're using the "Dot Notation Chaining" and accessing nested objects, methods, and properties to achieve your task:
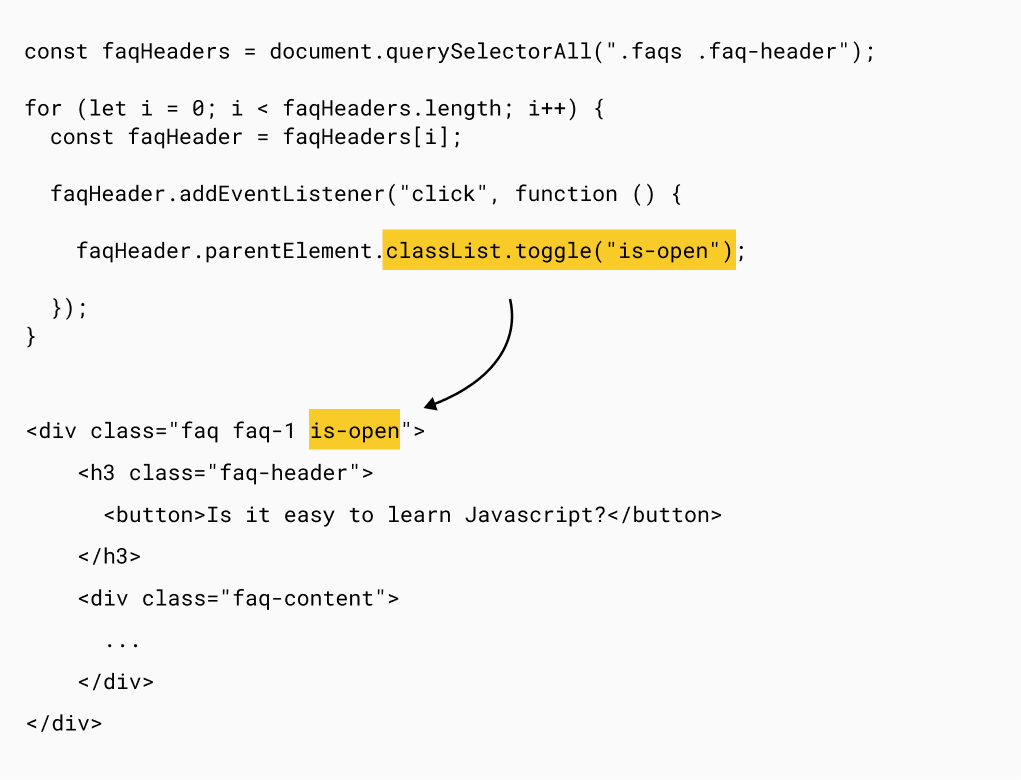
Always remember that the "Dot Notation Chaining" technique depends on the result of the previous computation.
There is a simple thinking technique you can use when trying to chain multiple objects together.
faqHeader.parentElement.classList.toggle("is-open");
If you are dealing with a something like the above code:
- First, think about what does
faqHeader
represent. Is it an object? If yes, is it an HTML object? - If it indeed represents an HTML object, then use the dot notation and access its property or method. In our case,
faqHeader
represents an HTML element. So, we are accessing theparentElement
property on it to add a class to it. - Next, think about the result of calling another necessary property or method on it. For example, what is the result of running
faqHeader.parentElement
? Is it an object again? If yes, is it an HTML object? - If it indeed represents an HTML object, then use the dot notation again and access the necessary property or method. In our case,
faqHeader.parentElement
represent an HTML element, too. And because we want to add a class to it, we access theclassList
object on it,faqHeader.parentElement.classList
.
We should continue this object process until we achieve what we want to achieve.
That's how the "Dot Notation Chaining" works.
In fact, you have been using the "Dot Notation Chaining" technique already, but you just didn't know the terminology of it.
Having said that...
The chaining could break and result in an error
document.querySelector("h4").parentElement.classList.toggle("is-open");
For example, imagine that there is no <h4>
level headline element on the webpage.
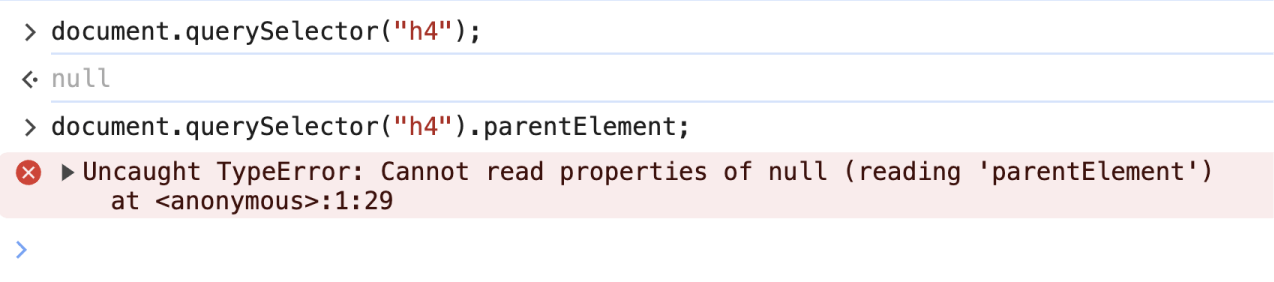
If that is the case, running document.querySelector("h4")
would return null
.
And if you're calling parentElement
property on a null value, it would return a TypeError saying: Cannot read properties of null.
This could break other Javascript code on the page.
For example, an e-commerce checkout functionality could break because of Javascript errors like the above.
So, be wary of this problem and always be careful while chaining objects.
Sometimes, using Dot Notation Chaining could make code unreadable
It is a good idea not to chain too many objects together.
Chaining up to three or four levels is fine, but after that, the code could become confusing.
Anyway, in the next lesson, we will learn some important things about the plus operator +
.