The true use of the console.log
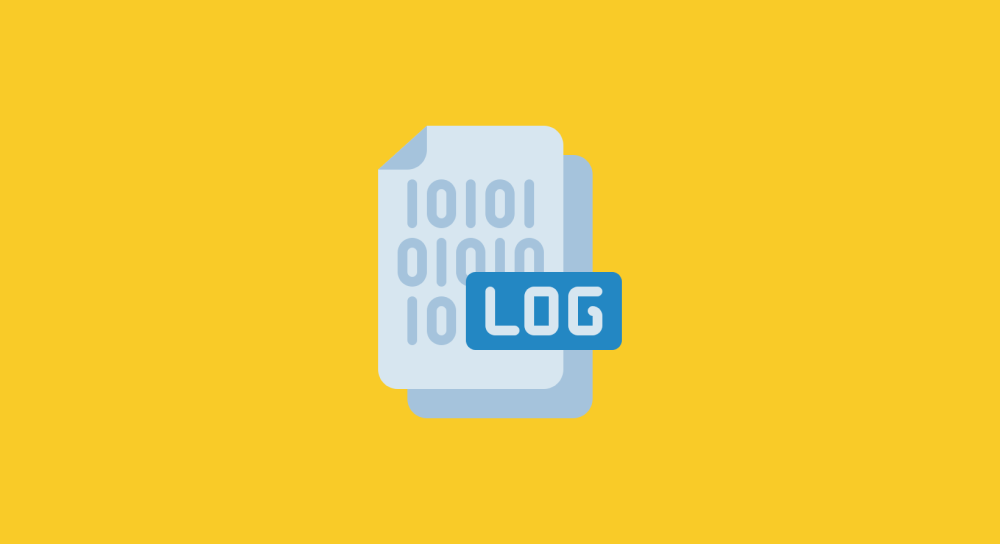
In the last lesson, we learned that the console.log
method is capable of logging a text message to the Browser Console.
The console.log method can also log variables
console.log(variableName);
To log the value of a variable, all you have to do is provide the variable's name as an input to the console.log
method.
let brandName = "Mini Lessons";
console.log(brandName);
In fact, being able to log variables helps us a lot while working on real-world tasks.
Come on, let's try it out.
This time, instead of trying from the Browser Console, let's create a project on our computer.
Download the starter files:
If you open up the main.js
file in the code editor, you'll find a variable called cart
.
There is a syntax error in the main.js
file. Fix it.
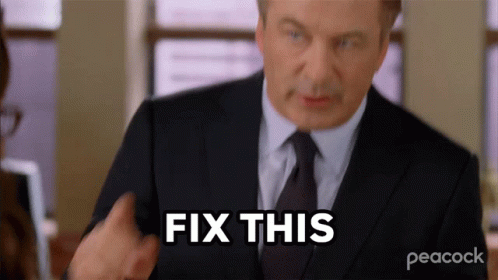
Did you fix it?
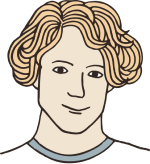
Yep!
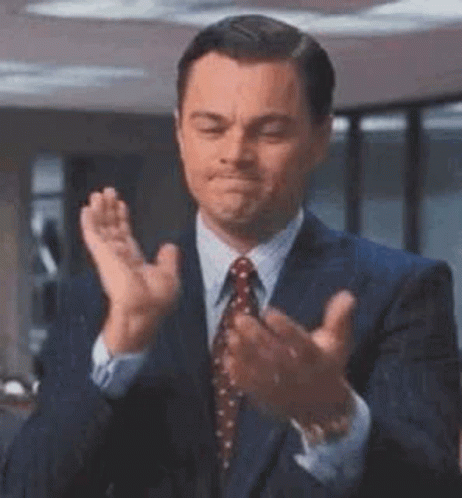
If you can't fix it, here is a hint:
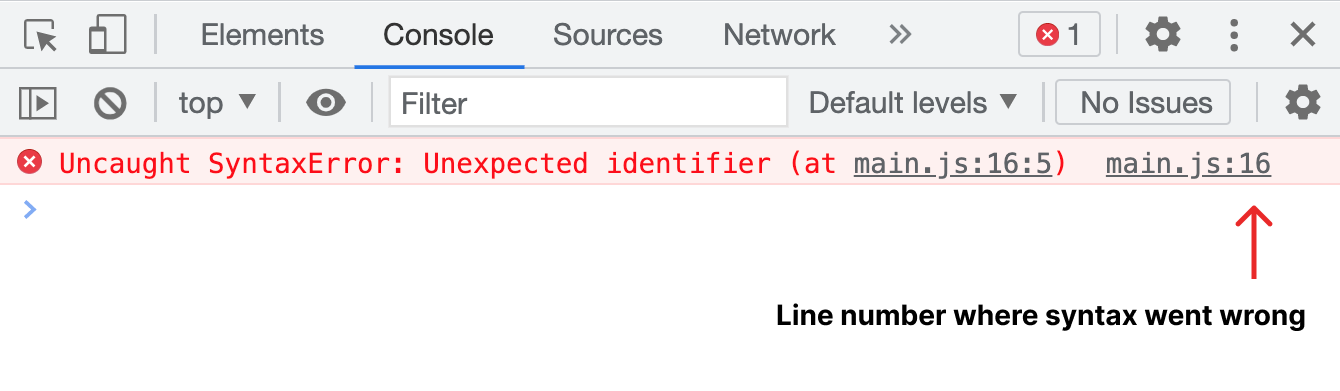
Anyway, if you notice, the variable cart
stores an object with some of a customer's shopping details.
Next, type the following line of code at the end of the main.js
file.
console.log(cart);
This line will log the data stored inside the cart
object to the Browser Console.
Here is the final main.js
file:
let cart = {
products: [
{
name: "iPhone 13",
brand: "Apple",
price: 400
},
{
name: "iPhone Charger",
brand: "Apple",
price: 79
}
],
itemsCount: 2,
shipping: "Free",
shippingTaxes: null,
couponCodeUsed: "20PERCENT",
subTotal: 479,
totalAmount: 479,
message: "Add one more product to be eligible for 5% additional discount."
};
console.log(cart);
Finally, open up the index.html in the browser and then the Browser Console.
If all goes well, you should see the cart
object logged inside the Browser Console.
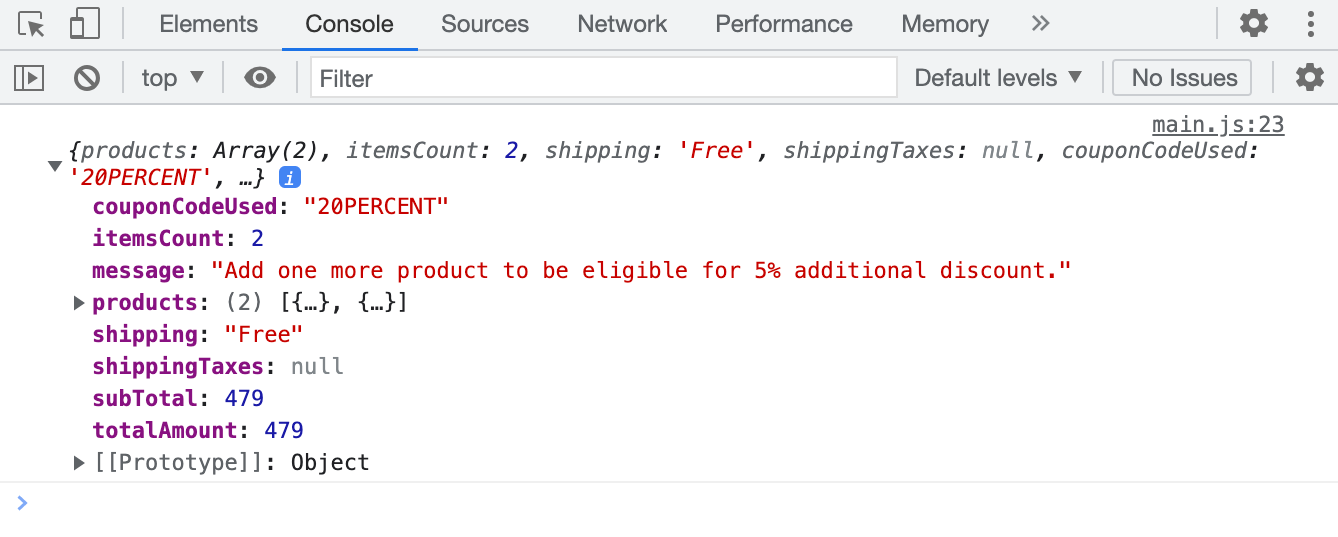
This is all great! But what if I want to log the products
property of the cart
object?
We can easily do that, too.
console.log(cart.products);
The above line will tell the Console that:
"Yo! Console! log the data of the products
property of the cart
object."
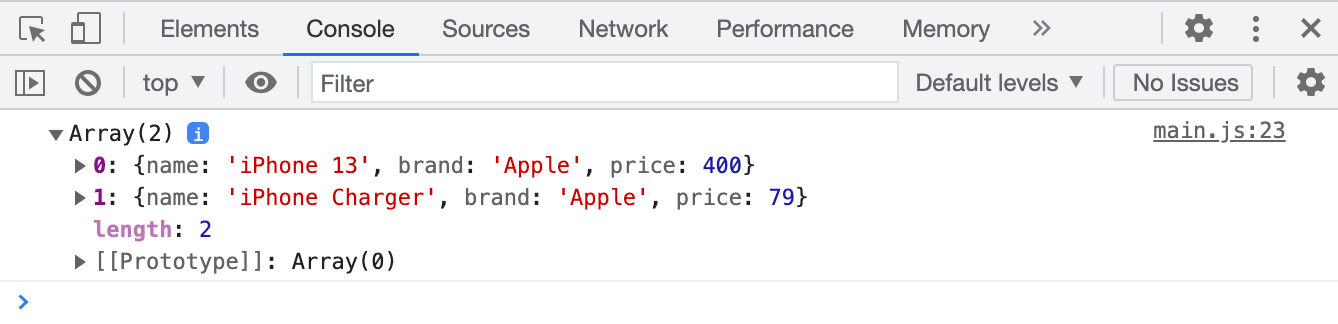
Anyway, it is boring to see the data of product
data in the default format of the Console.
So, I want to log the products
property in the format of a table.
And we can easily do that with the table
method of the console
object.
console.table(cart.products);
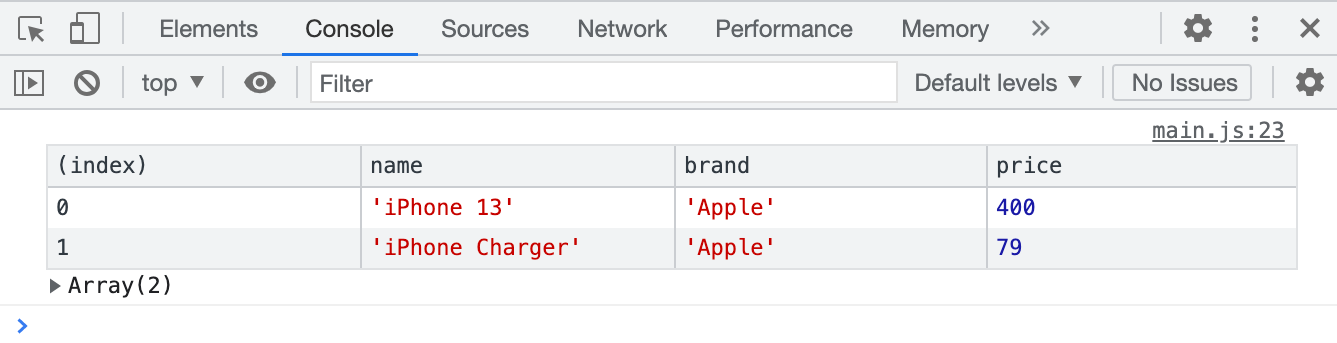
Wow! Did you see that? The data is much easier to scan this way.
I love the Console :D
Anyway, the table
method is the same as the log
method, but it is just a bit easier on our eyes :P
Having said that, there are a lot more methods that are fancier than the log
method.
You can see them by accessing the console
object itself.
console.log(console);
Yep, you can provide just about any object to the console.log
method, including the console
itself.
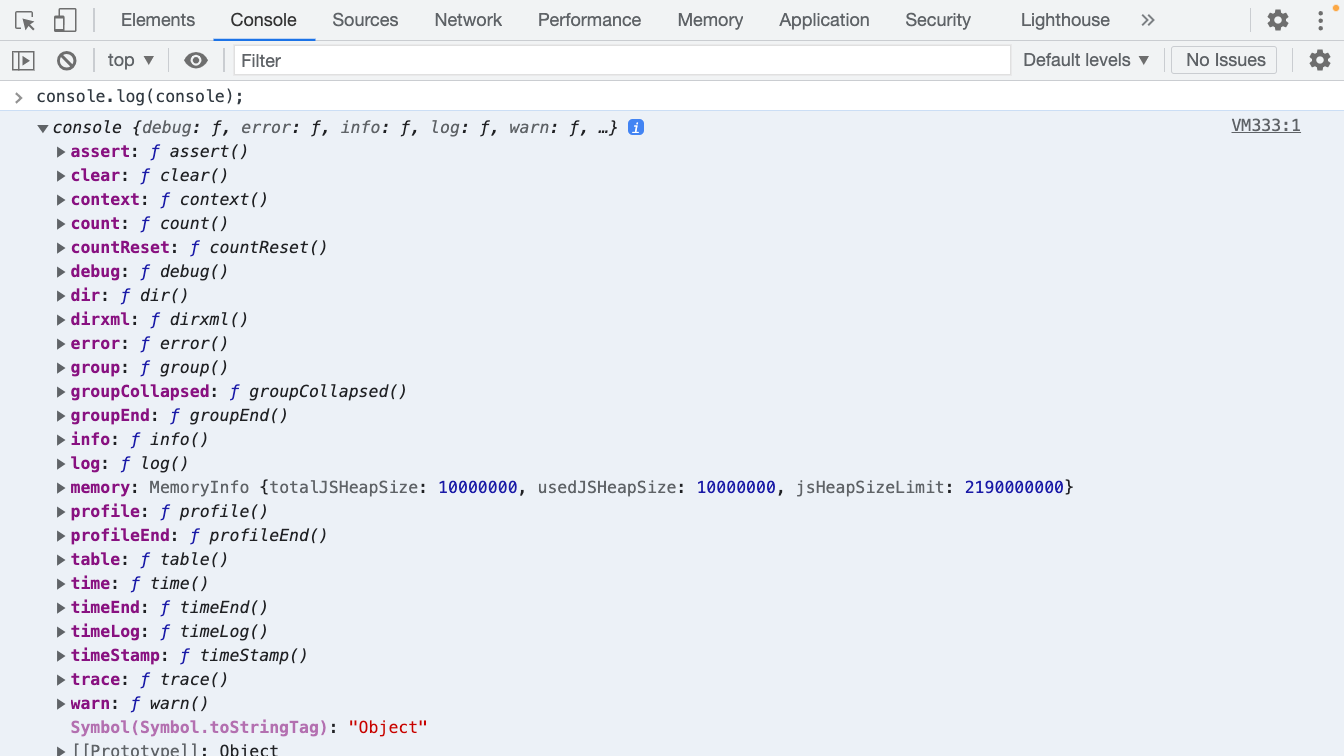
Isn't it powerful?
Anyway, I hope this lesson will help you understand the methods a bit more.
Now that we know how to use object methods properly, in the next lesson, we will quickly learn about the window
object.