The need for defining custom functions in Javascript
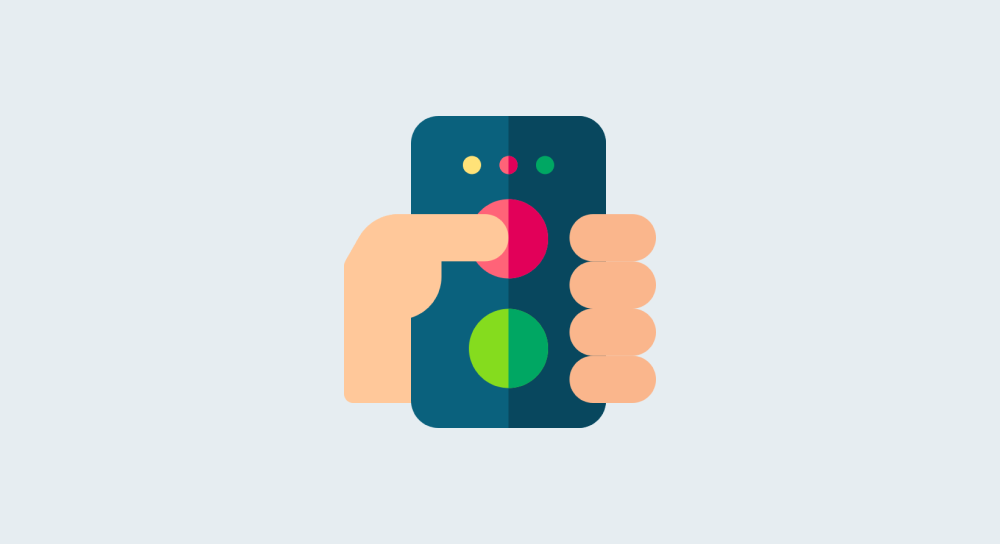
Before you start reading this lesson, I want you to read the Function 101 lesson as a refresher.
Anyway, from the beginning of this course, we performed a lot of small tasks.
And while performing these small tasks using javascript, we learned:
- How to change an image by changing its attributes
- How to show the password entered
- How to reveal a modal by changing its CSS style
- How to change CSS classes
The thing is, based on the javascript component we are building, we might have to perform the above tasks over and over again.
They are not one-time tasks.
For example, let's talk about the project at hand.
We are currently building a modal component in Javascript, right?
And you already know how to reveal a modal.
You performed this task during the last lesson.
//The code for revealing the modal
//Step 1: Select the Discount Modal element
let discountModal = document.querySelector("#surprise-discount-modal");
//Step 2: Change the display property of the element to "block"
discountModal.style.display = "block";
But there are two problems with the above code because they are just individual lines of code:
- The browser will execute the above code as soon as the page is loaded and the modal is revealed.
- If we want to reveal the modal again for the second time, there is no way we can execute the individual lines of code again.
This is better explained by understanding the execution flow of the above code from a high-level perspective.
Simplified overview of how the execution flow of Javascript code works when a web page is loaded
It all starts with index.html
getting loaded in the browser.
Once the index.html
file is loaded, and the browser will start parsing and rendering the file line by line.
<!DOCTYPE html>
<html lang="en">
<head>
...
<link rel="stylesheet" href="style.css" />
</head>
<body>
<div class="button-container">
...
</div>
<div id="surprise-discount-modal">
...
</div>
<script src="index.js"></script>
</body>
</html>
Because we have placed the link to the index.js
file at the end of the document, the browser will first render the HTML with the styles from the style.css
file.
And this will result in the following output:
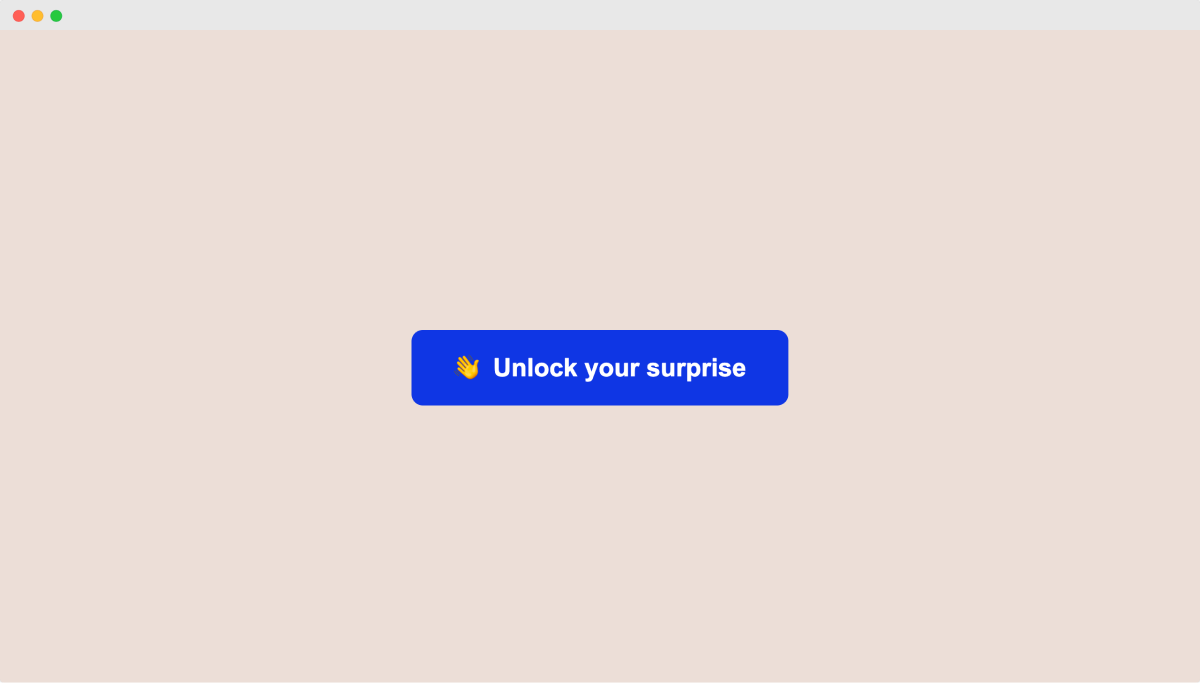
After this, when the browser reaches the end of the HTML document, it will find the link of index.js
file and it will load the file and starts parsing it.
As soon as the parsing of index.js
file begins, the browser will try to execute the code line by line immediately because they are individual lines of code.
This is also called Synchronous Javascript execution flow.
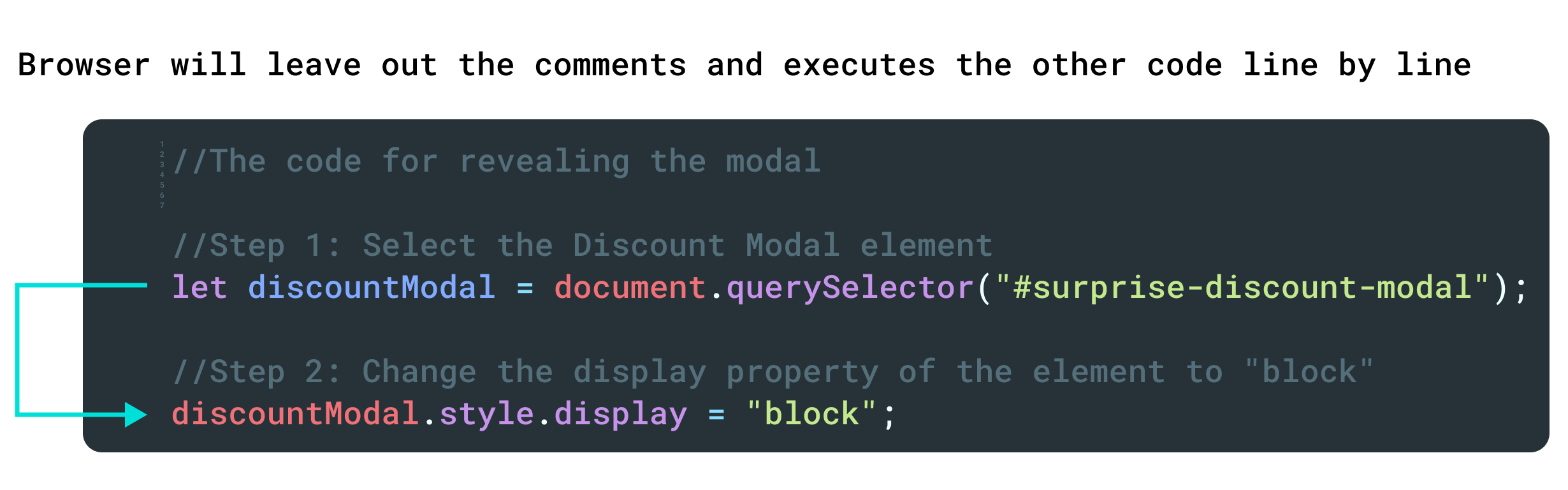
And this will result in this output:
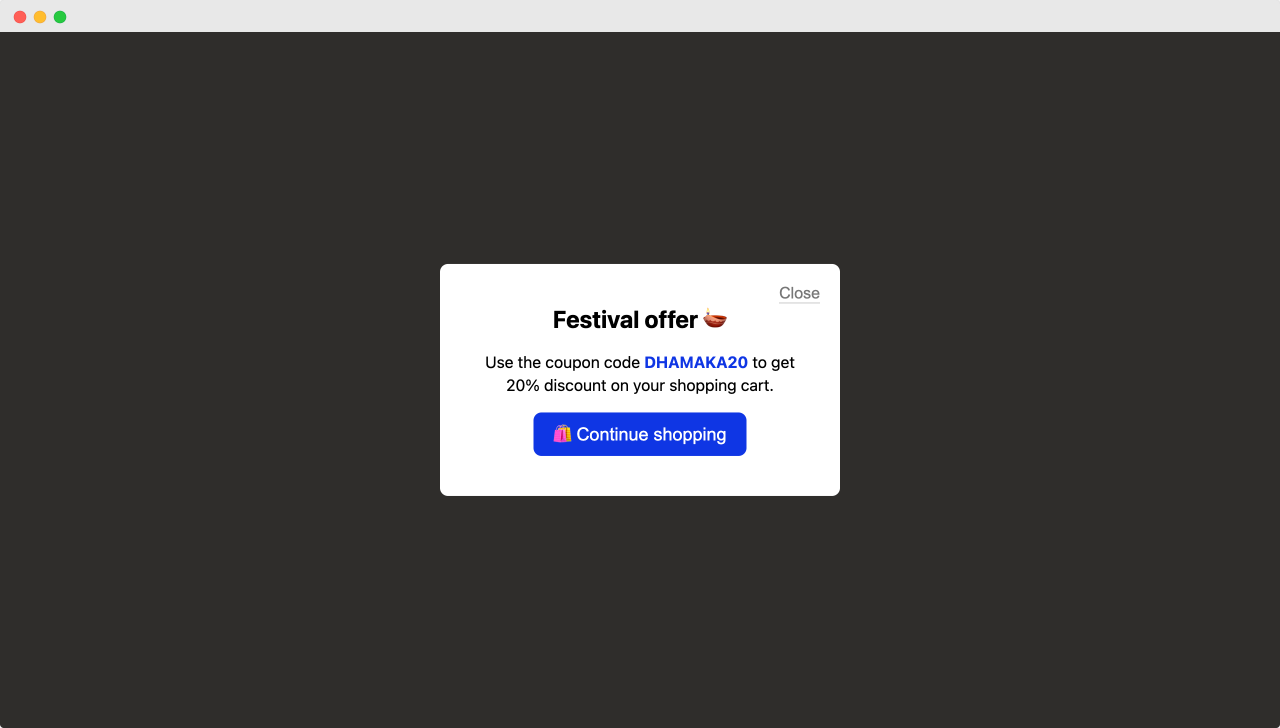
And there are three problems with the execution flow of index.js
file.
- Once the browser executes the individual lines of javascript code, it doesn't execute them again unless the browser is reloaded.
- Because of this, there is no way to reveal the modal multiple times.
- And there is no way to reveal the modal when the button is clicked.
But, Usually, on websites, we have to reveal the modal multiple times.
And we usually reveal a modal only when a button is clicked.
For example:
In the above scenario, we have to reveal the modal every time the button is clicked.
We don't exactly know how many times the user will click on the button.
Long theory short, we need a way to execute the code revealing the modal every time the button is clicked.
And this is where functions come in Javascript.
The need for defining a custom function in Javascript
A function
is nothing but a self-contained block of code that performs a specific task.
Also, If you remember:
- A
function
will help us perform a task over and over again. - And it gives us the control to perform the task only if we want to.
And most important, the superpower of a function
is, the browser doesn't execute a function
as soon as it sees it.
We have to explicitly instruct the browser to execute the function by calling its name.
This will give us all the control we need.
So, if we want to execute the code for revealing the modal:
- Multiple numbers of times
- And only when we want to ( For example, only when the button is clicked)
Then we have to transform the code into a function.
And we will learn how to do that in the next lesson.