The document object: Selecting HTML elements
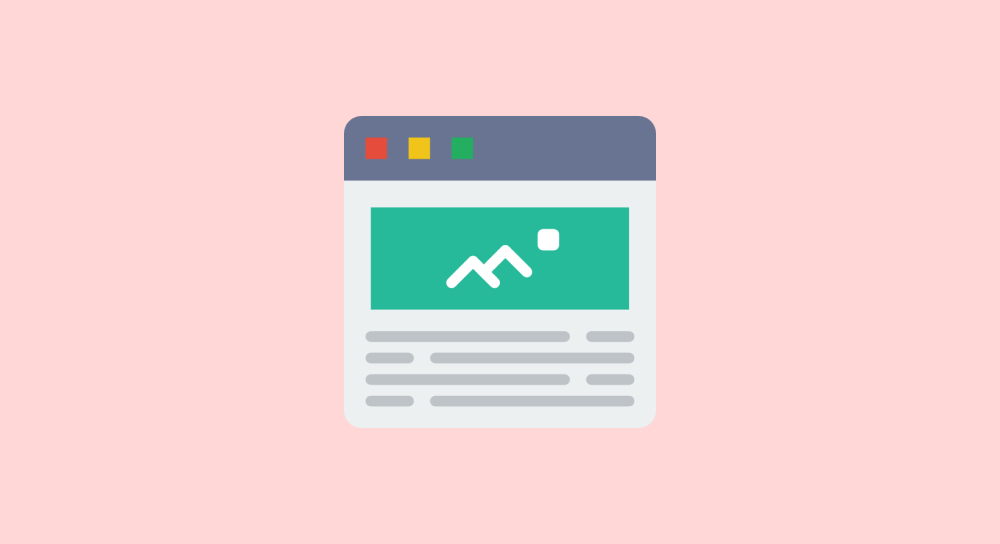
The document
object helps us perform actions on the HTML Document (web page).
So, if you are trying to code any component in Javascript, you can not do it without using the document
object.
For example, when you're trying to code anything in Javascript:
- You need to select an HTML element
- And then manipulating its attributes or content to achieve the desired interactivity.
And this is where document
object comes in.
It comes loaded with methods that help you select elements inside the HTML document.
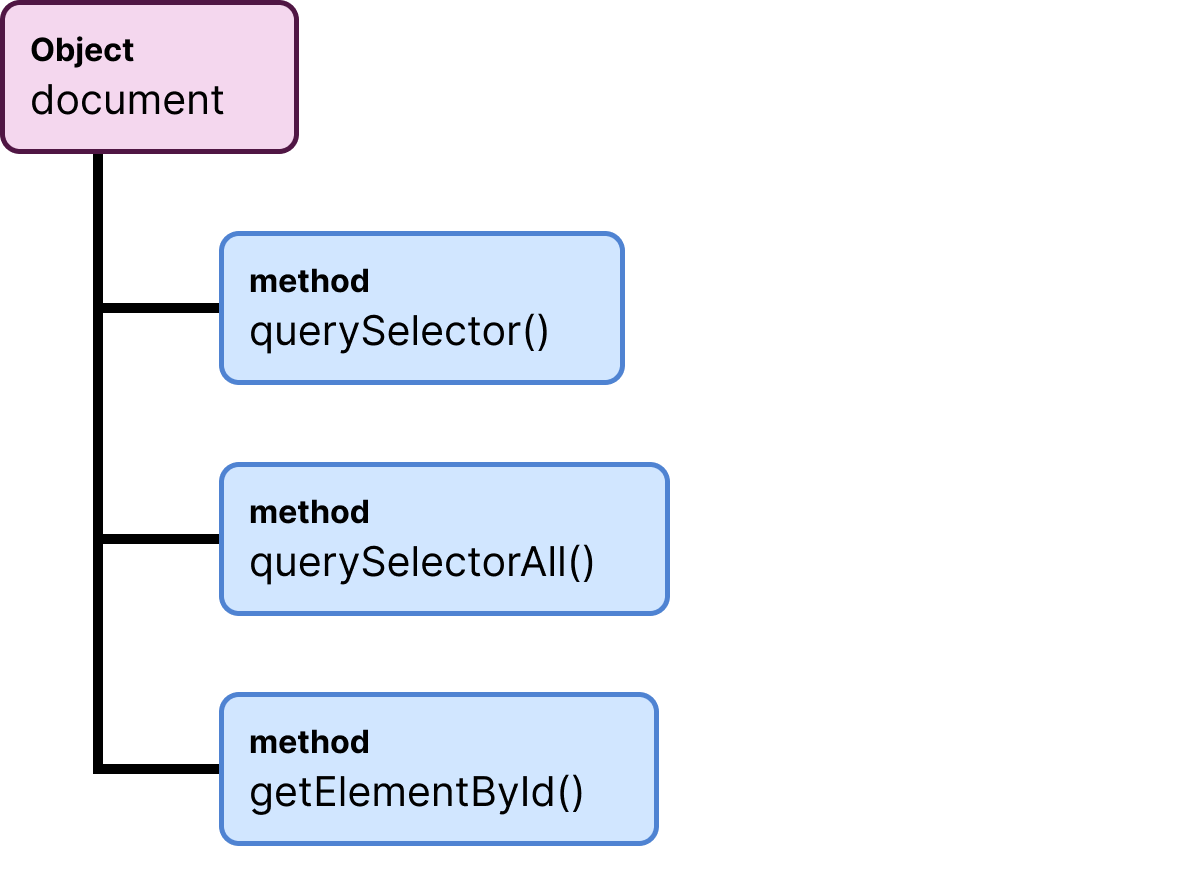
Not just selecting elements, the document
object also helps you check whether the HTML document is fully loaded.
It is ideal for you to perform some JavaScript-based operations only once the HTML document is fully loaded.
So, it is very important that you get good with the document
object.
Anyway, in this lesson, to understand the role of the document
object, let's see how to use it to select HTML elements on a web page.
Selecting HTML elements using the document object
There are a lot of methods for selecting HTML elements, and we have to choose the right method based on the task at hand.
Most of the time, the method is chosen based on how many HTML elements we are selecting at the same time.
If you want to select only one HTML element, we can use:
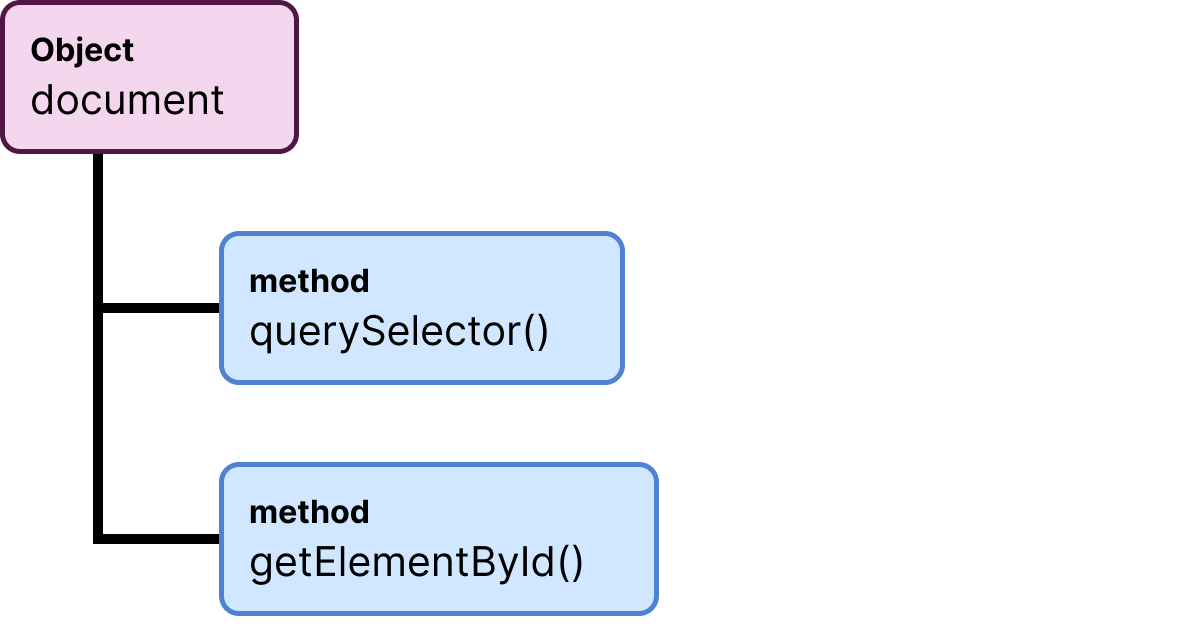
1) Using document.querySelector()
Remember, the querySelector()
method helps select only one element.
And this method accepts one input and it must be a valid CSS selector surrounded by quotes.
// Syntax
document.querySelector("css-selector");
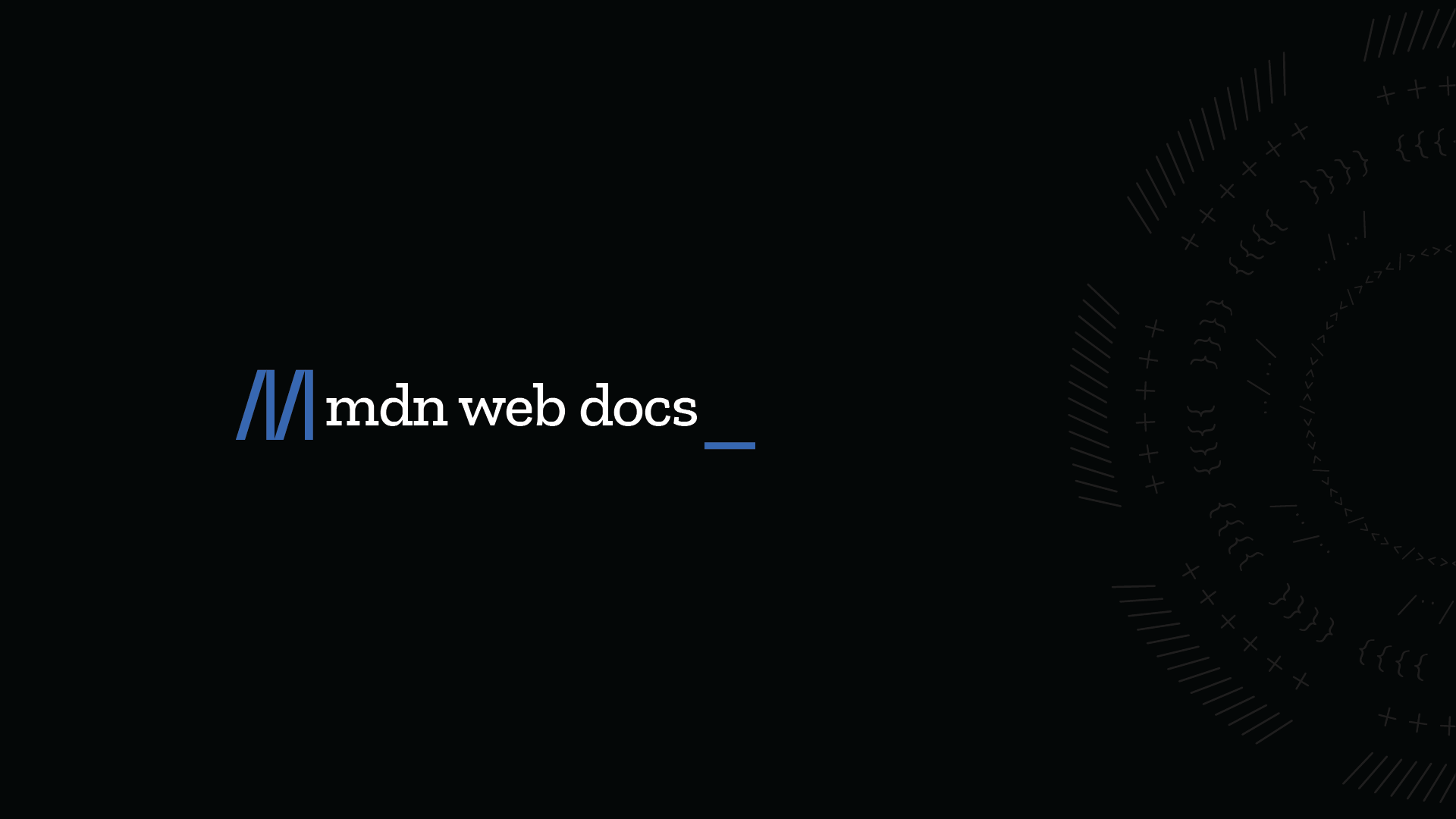
//Usage
document.querySelector("section"); // Tag Selector
document.querySelector("#main-content"); // ID Selector
document.querySelector(".sidebar"); // Class Selector
document.querySelector("[aria='hidden']"); // Attribute Selector
The querySelector
method returns only the first element it finds with the CSS selector provided.
For example, let's just say there are multiple HTML elements with the class name sidebar
:
<div class="sidebar sidebar-1">...</div>
<div class="sidebar sidebar-2">...</div>
<div class="sidebar sidebar-3">...</div>
In this case, the method returns the first element it finds with the sidebar
class and that is:
document.querySelector(".sidebar");
// Returns: <div class="sidebar sidebar-1">...</div>
2) Using document.getElementById()
As the name suggests, the getElementById
method helps you select a single HTML element based on a particular HTML element's id
attribute.
This method accepts one input, and it must be a valid HTML element ID name surrounded by quotes.
// Syntax
document.getElementById("id-of-an-html-element");
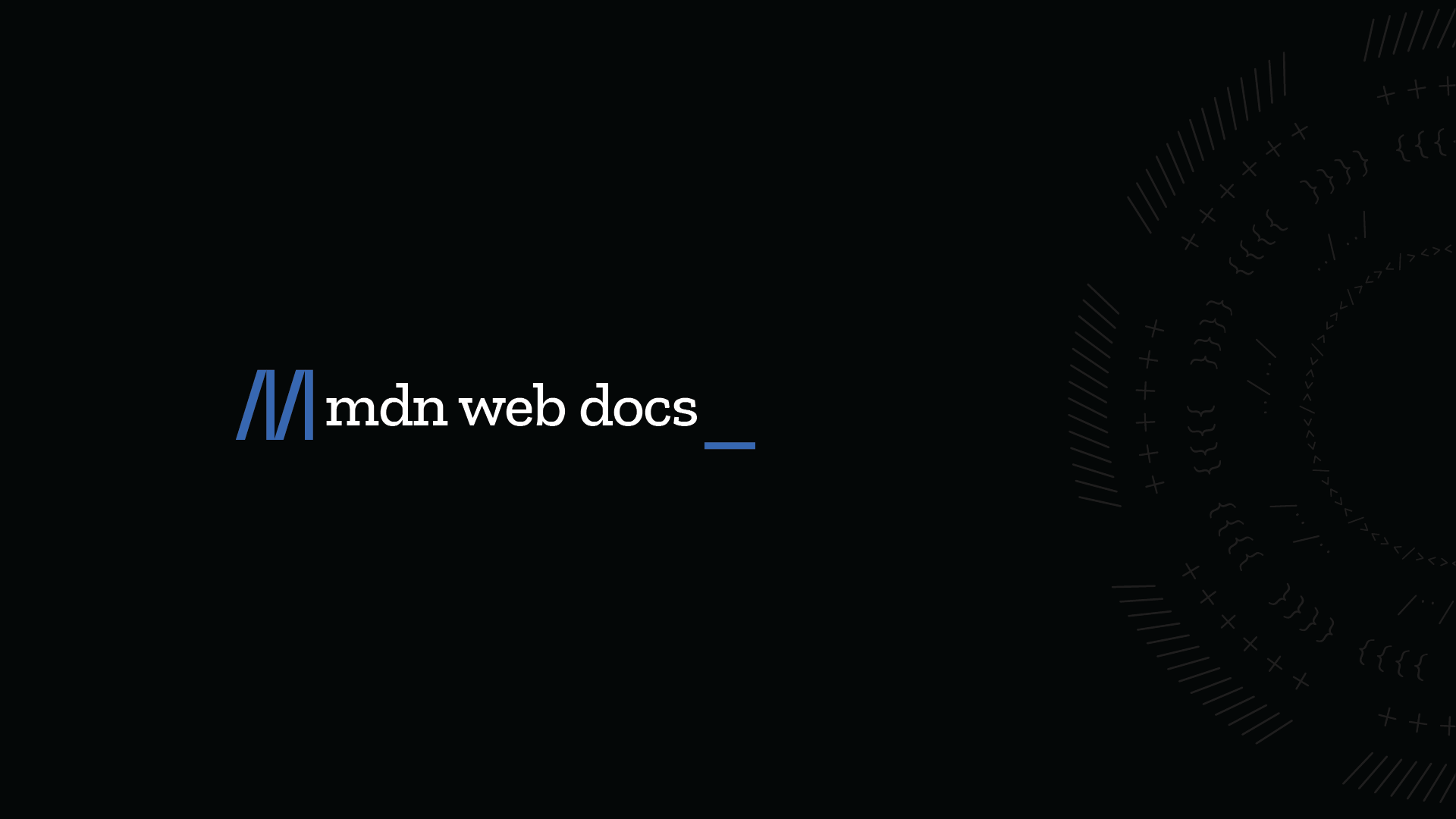
//Usage
document.getElementById("main-content");
Remember, don't include the #
while providing the id name.
This is wrong:
//Doesn't work
document.getElementById("#main-content");
Anyway, If you want to select multiple HTML elements at the same time, then we have to use:
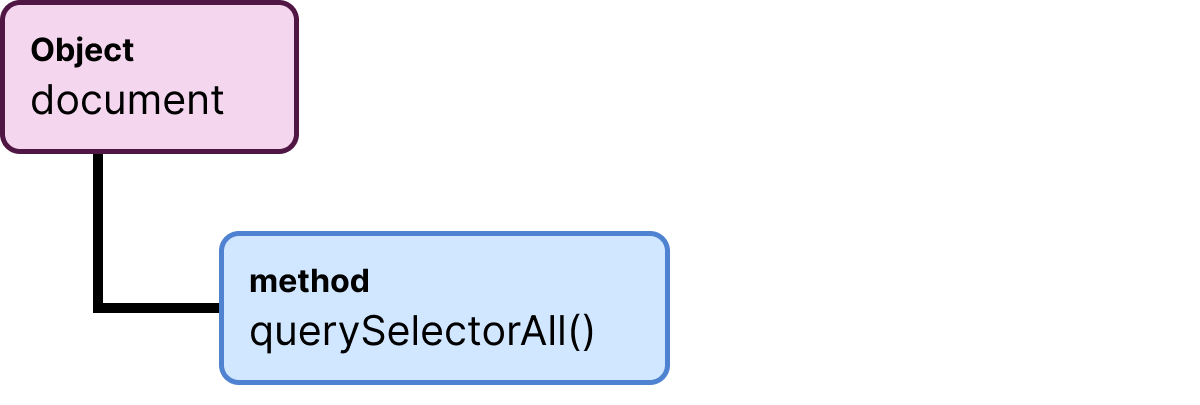
document.querySelectorAll();
As the name suggests, this method helps to select multiple HTML elements at the same time.
This method accepts one input, and it must be a valid CSS selector surrounded by quotes.
//Syntax
document.querySelectorAll("css-selector");
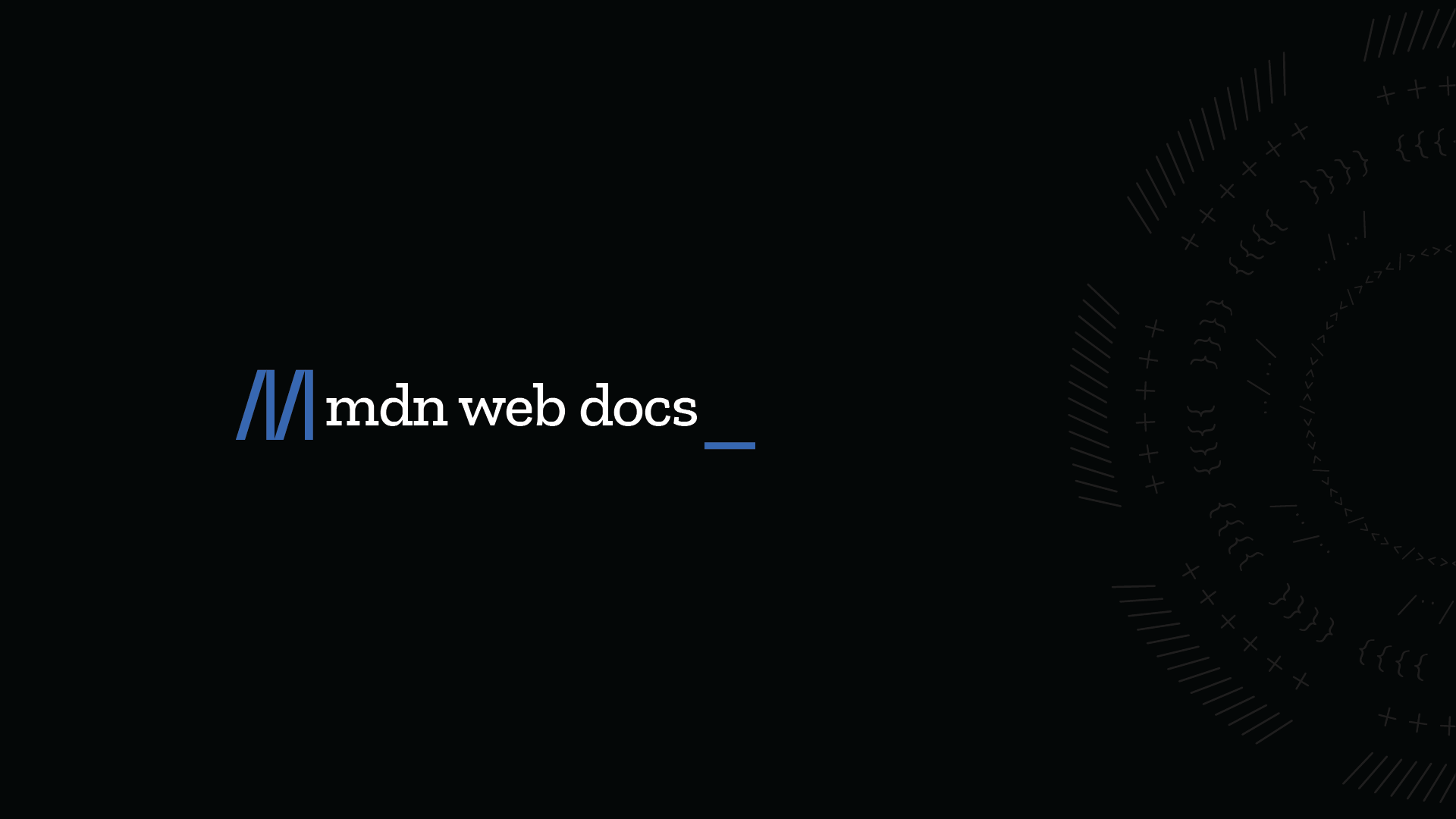
For example, let's just say there are multiple HTML elements with the class name sidebar
:
<div class="sidebar sidebar-1">...</div>
<div class="sidebar sidebar-2">...</div>
<div class="sidebar sidebar-3">...</div>
In this case, the querySelectorAll()
method returns all three sidebar elements it finds with the sidebar
class.
document.querySelectorAll(".sidebar");
//Returns:
/*
<div class="sidebar sidebar-1">...</div>
<div class="sidebar sidebar-2">...</div>
<div class="sidebar sidebar-3">...</div>
*/
And that is pretty much what you need to know about the document
and its selector methods for now.
In the next lesson, we will see what happens when we select an HTML element.