Exercise: Subscribe or Unsubscribe
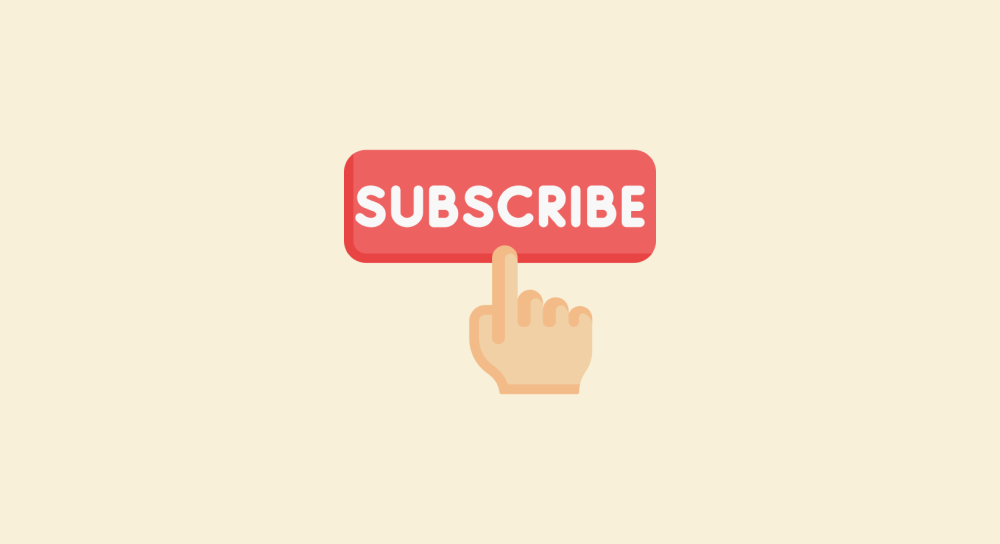
In this lesson, I want to test your understanding of the If/Else statements.
I want you to use an If/else statement to change the button text interactively.
The Exercise
Here is what you should achieve:
Here is a hint about how to achieve it.
If the button is clicked:
- First, we need to check(evaluate) if the button's text is "Subscribe" or "Unsubscribe".
- If it is currently "Subscribe", we need to change the button text to "Unsubscribe".
- Else, If it is currently "Unsubscribe", we need to change the button text to "Subscribe".
And here is the exercise starter files:
The Solution
I hope you've figured it out.
If not, I want you to read the last couple of lessons again or message me.
Anyway, here is the javascript to make the "Subscribe/Unsubscribe" functionality work:
const subscribeBtn = document.querySelector("#subscribe-btn");
subscribeBtn.addEventListener("click", function(){
if(subscribeBtn.textContent === "Subscribe"){
subscribeBtn.textContent = "Unsubscribe";
} else {
subscribeBtn.textContent = "Subscribe";
}
});
First, we are adding an event listener to the "Subscribe" button.
When the button is clicked, first we are evaluating if the button text is currently "Subscribe" or not:
subscribeBtn.textContent === "Subscribe"
Initially, when the button is clicked for the first time, the button text is "Subscribe":
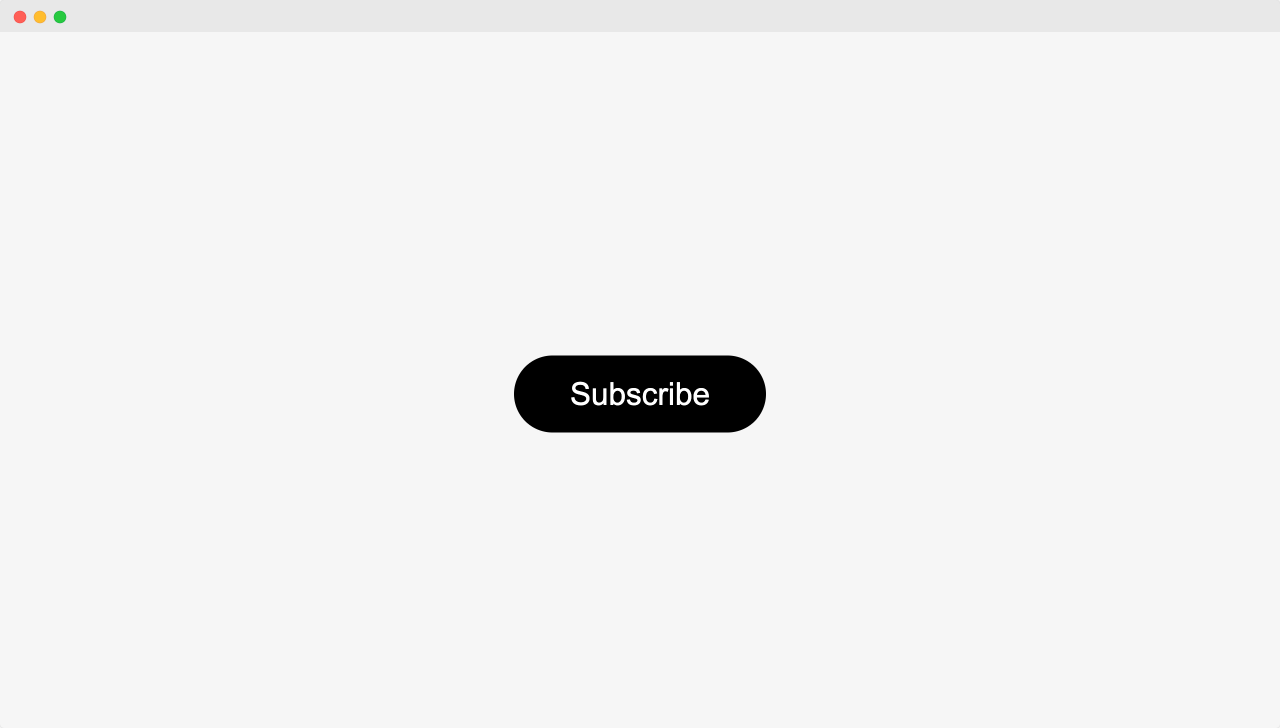
So, the expression subscribeBtn.textContent
produces a value of "Subscribe".
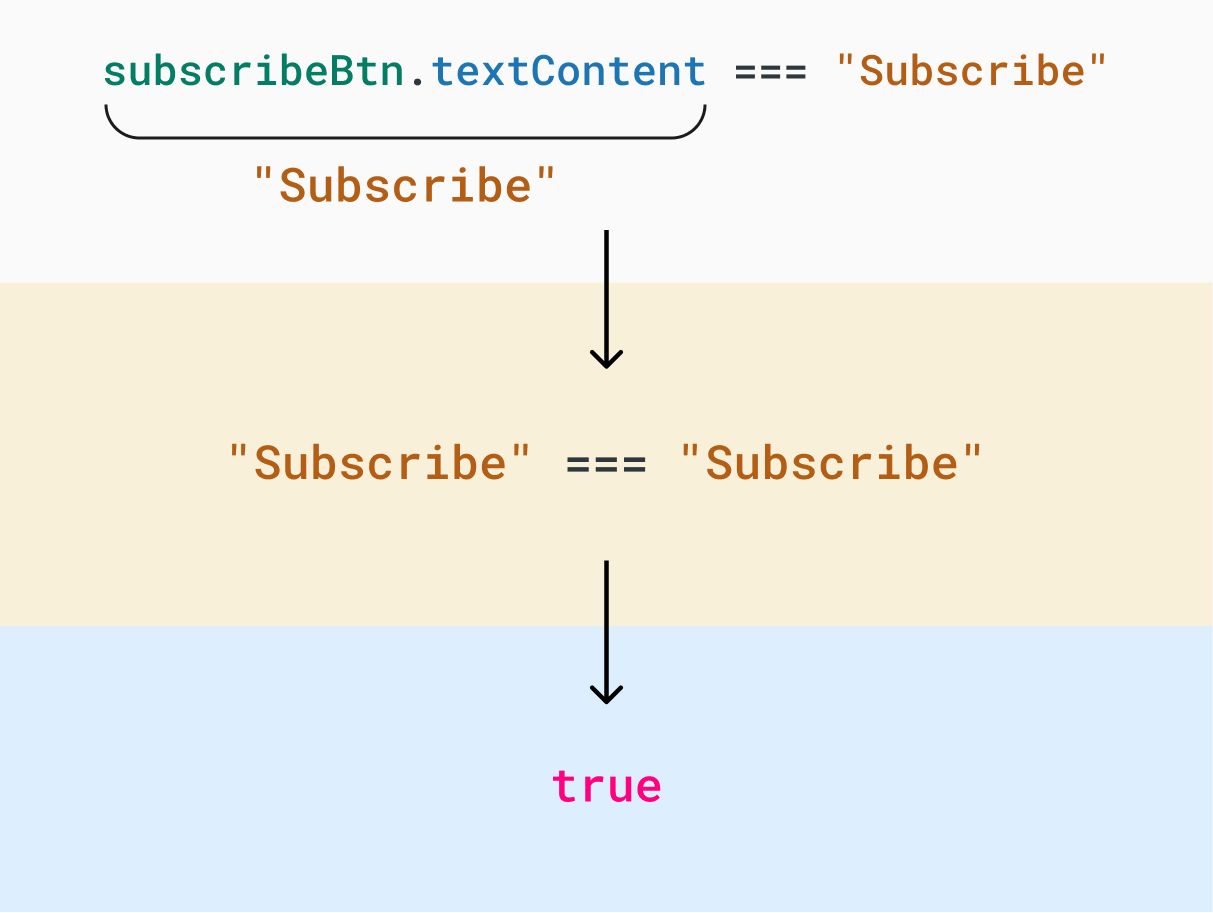
The condition is true, right?
so, we are changing the button text to "Unsubscribe" using the code inside the "If" block:
subscribeBtn.textContent = "Unsubscribe";
After this, the code inside the "Else" block is skipped and the browser comes out of the execution flow of the index.js
file.
And when the button is clicked for the second time, this time, the button text is "Unsubscribe":
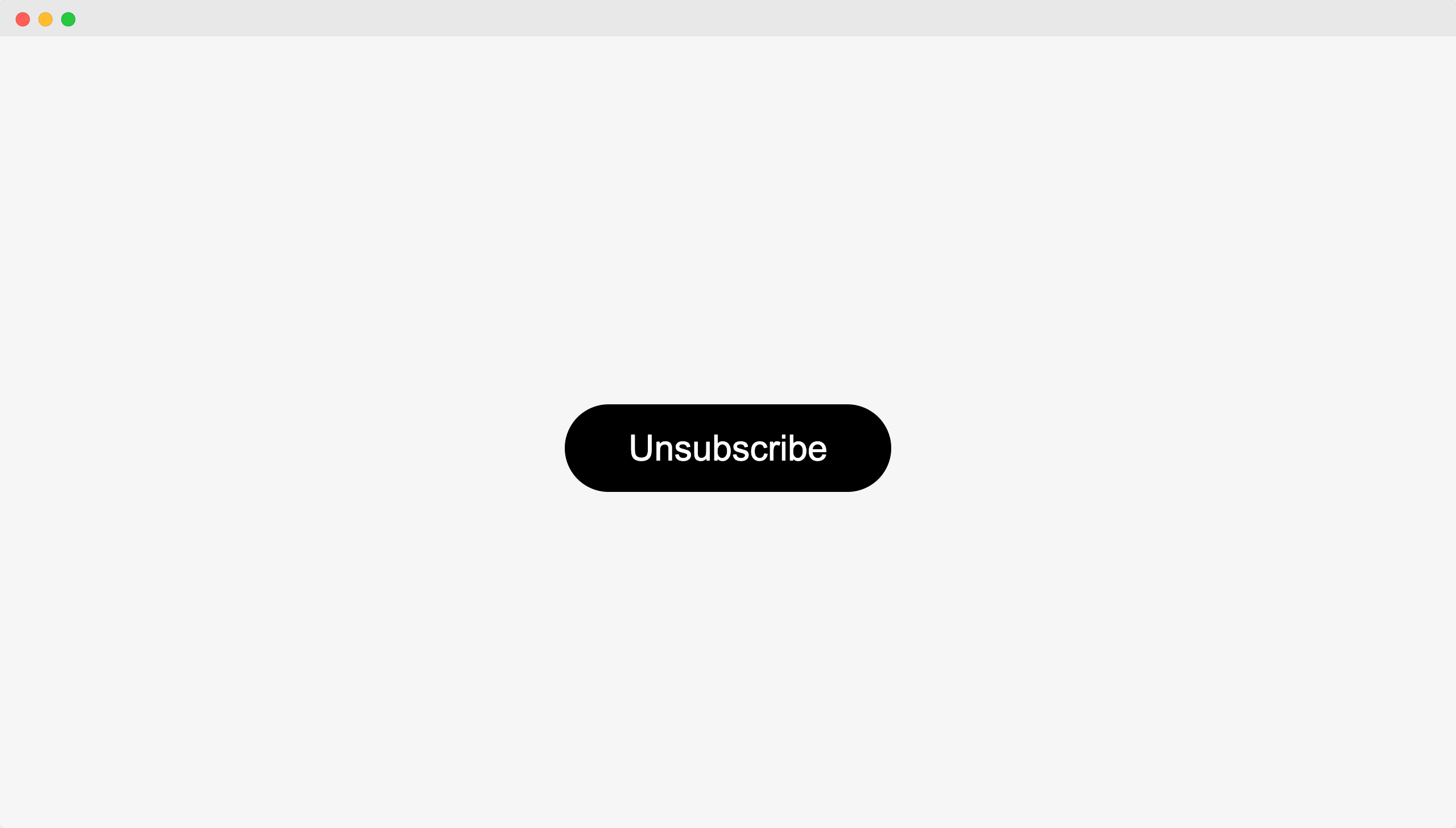
So, the expression subscribeBtn.textContent
produces a value of "Unsubscribe".
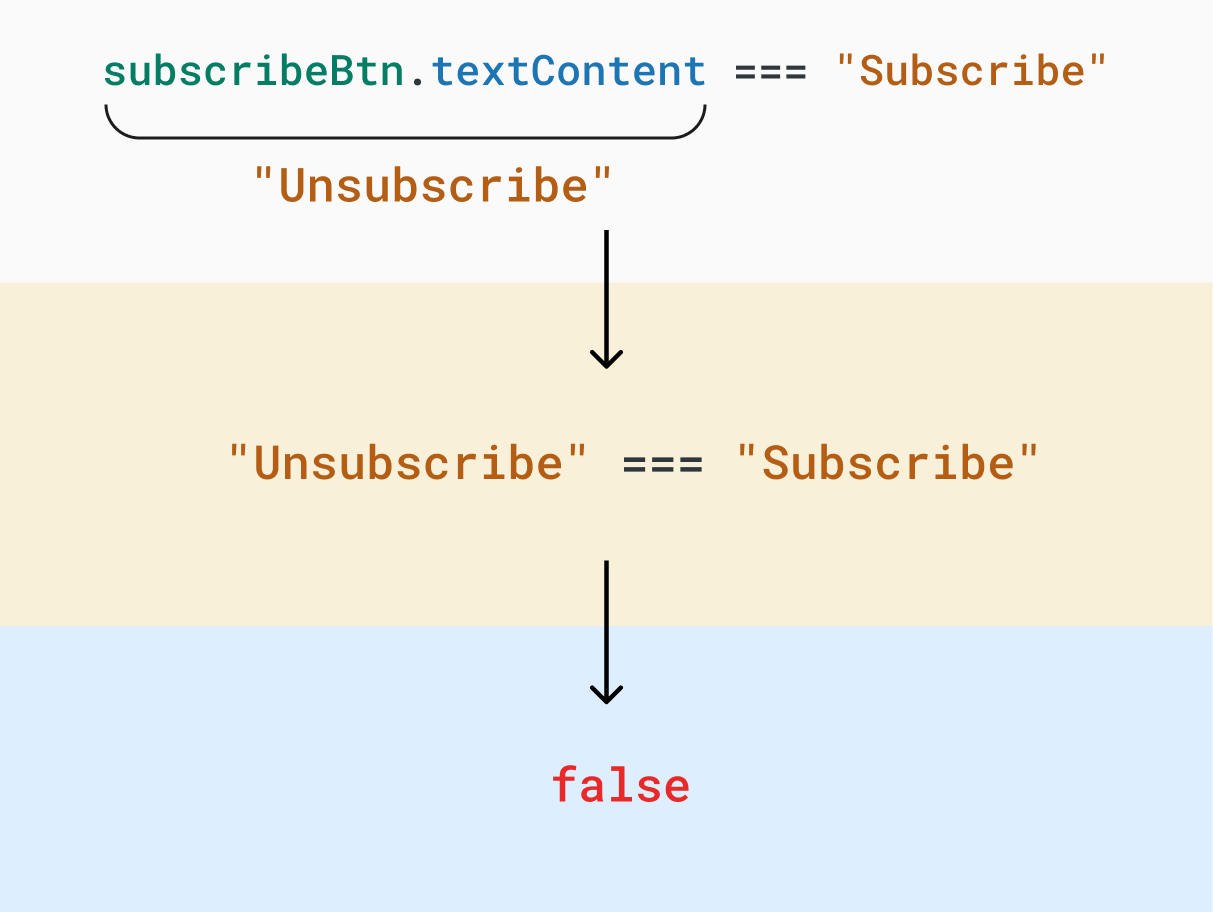
The condition is false, right?
so, we are skipping the "If" block and we are changing the button text back to "Subscribe" using the code inside the "Else" block:
subscribeBtn.textContent = "Subscribe";
After this, the browser comes out of the execution flow of the index.js
file.
That's all.
Another Task: Enhancing the solution
If you notice the actual goal video, when the button text changes to "Unsubscribe", the button background color and text color are getting changed too:
But in our case, only the button text is getting changed:
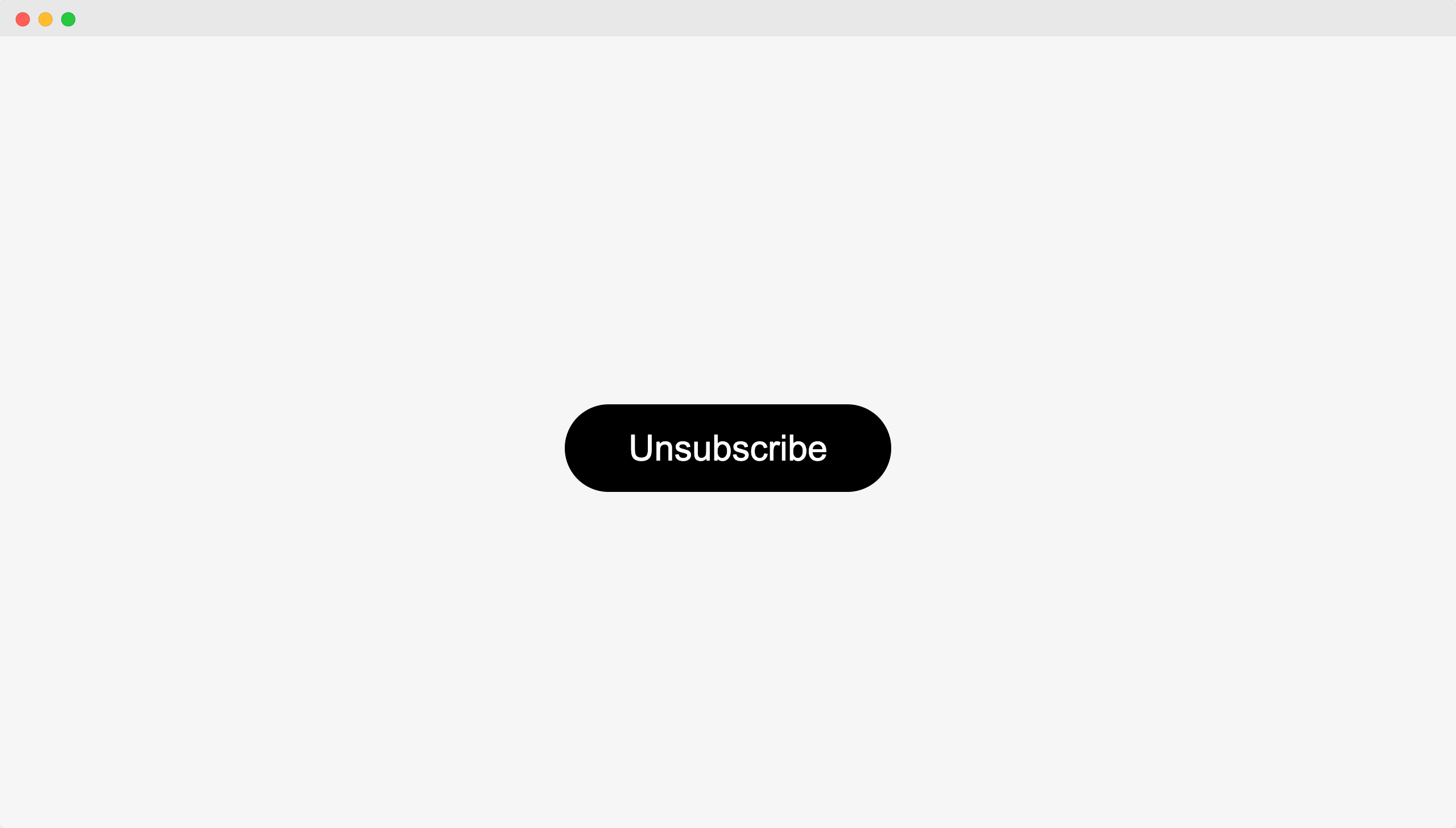
Fix it by changing the CSS styles of the button when the text is "Unsubscribe".
You already know how to do this :)
Solution
const subscribeBtn = document.querySelector("#subscribe-btn");
subscribeBtn.addEventListener("click", function(){
if(subscribeBtn.textContent === "Subscribe"){
subscribeBtn.textContent = "Unsubscribe";
subscribeBtn.style.backgroundColor = "#ccc";
subscribeBtn.style.color = "black";
} else {
subscribeBtn.textContent = "Subscribe";
subscribeBtn.style.backgroundColor = "black";
subscribeBtn.style.color = "white";
}
});
You can also simplify the above code further by giving the burden of changing the background color and text color to CSS like this:
const subscribeBtn = document.querySelector("#subscribe-btn");
subscribeBtn.addEventListener("click", function(){
if(subscribeBtn.textContent === "Subscribe"){
subscribeBtn.textContent = "Unsubscribe";
subscribeBtn.classList.add("unsubscribed");
} else {
subscribeBtn.textContent = "Subscribe";
subscribeBtn.classList.remove("unsubscribed");
}
});
We are just adding the class of "unsubscribed"
to the button element when the button text is changed to "Unsubscribe" and removing the class when the text "Subscribe".
But adding or removing the class is not enough.
Here is the supporting CSS:
button.unsubscribed{
background-color:#ccc;
color:black;
}
The output
In the next lesson, we will learn how to check for multiple conditions inside your code.