Task: Disable Right Click using event.preventDefault()
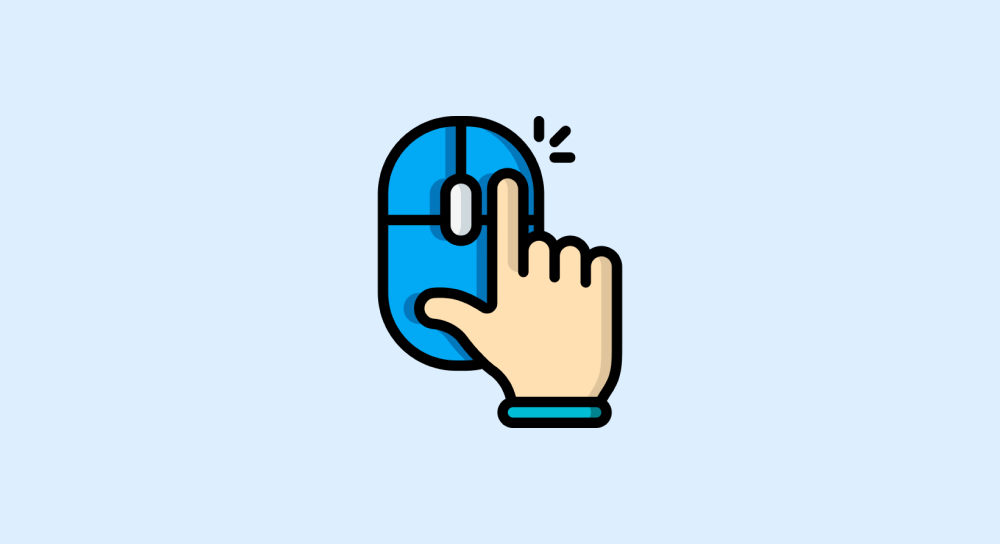
Client: Hey Naresh, I want to disable right-click on my web page.
Me: Oh, that is bad for the user experience because we should never go against the default behavior that a user expects. It just annoys the website visitors. If they disable Javascript, they can still access the content on the page. So, it is not worth the effort.
Client: Blah, Blah...I know you would spit out something like this. I don't care about morals here. I need the right-click disabled. Can you do it or not?
Me: Haha 😅, it is my responsibility to tell you what's wrong so that you can make an informed decision. I will implement it for you, but can I know the reason so that I can think of an alternative solution?
Client: I don't have your research budget for this. So, please disable the right-click for me. I am presenting a video to my client, but I don't want him to save it by right-clicking and using the "Save video as" option from the context menu.
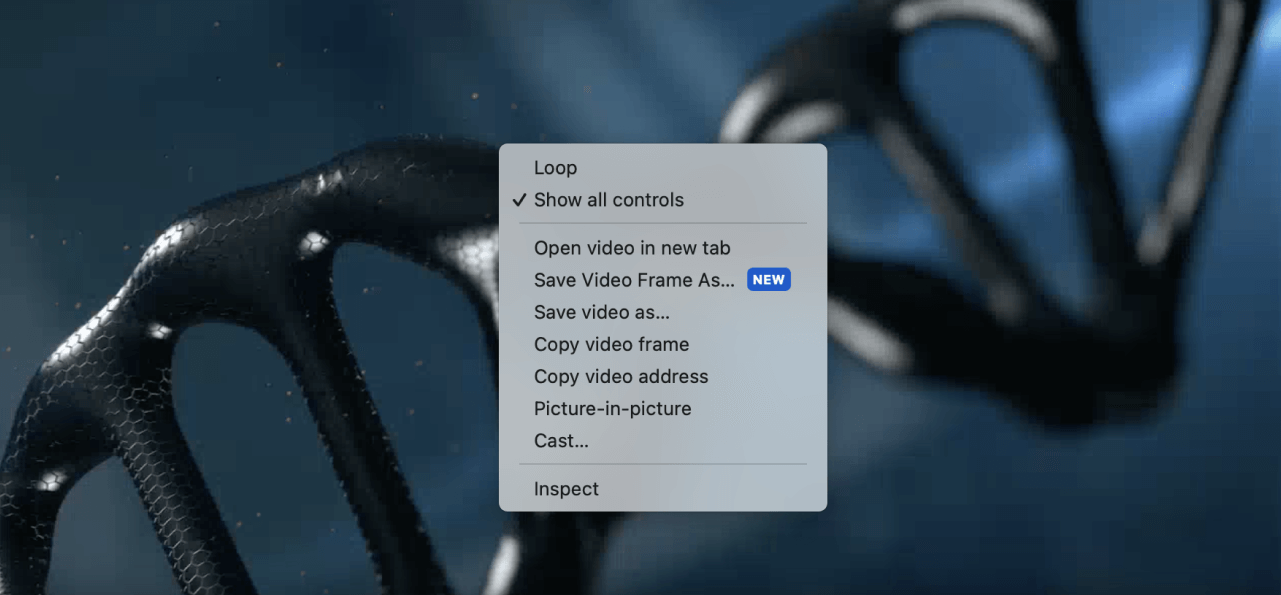
Client: My client is not tech-savvy enough to disable Javascript or use other workarounds. So, although it is not a 100% foolproof method to protect my design, but it is just for a couple of minutes. I will delete the page later.
Me: Got it, I will do that. It will cost you $30. Is that okay?
Client: Yes, go ahead and send me the invoice.
I have a conversation similar to this at least once every couple of months because I work with many online educators and freelancers in other professions.
Although it is not a good practice to disable the right-click and annoy the user, our clients will have their own strong reasons.
They often don't care about accessibility. It is unimportant in their view, and sometimes they are not wrong.
Sometimes, we just have to deliver what the client wants instead of losing him forever in the name of best practices.
Disabling the right-click is controversial because not everyone shares the same views about why and how to protect content on the Internet.
So, In this lesson, I will show you how to disable right-click on any web page.
Just use this technique with caution and only after educating the client of the accessibility issues that the users will face.
Download the starter files and open them up inside the code editor:
The index.html
file has a simple purpose:
<div id="main-content">
<h1>DNA Animation: Version 11</h1>
<video src="dna-animation.mp4" controls autoplay></video>
</div>
It just has a headline and a video.
Video Credits: Pressmaster (Thank you)
Anyway, if you preview the index.html
in the browser, you can currently right-click on the video to access the context menu and save the video to your computer:
But our client doesn't want to because he fears that his client could use the video without paying the remaining freelance fee.
So, let's disable the right-click.
Also, the whole point of disabling the right-click is not to let the user use the context menu like this:
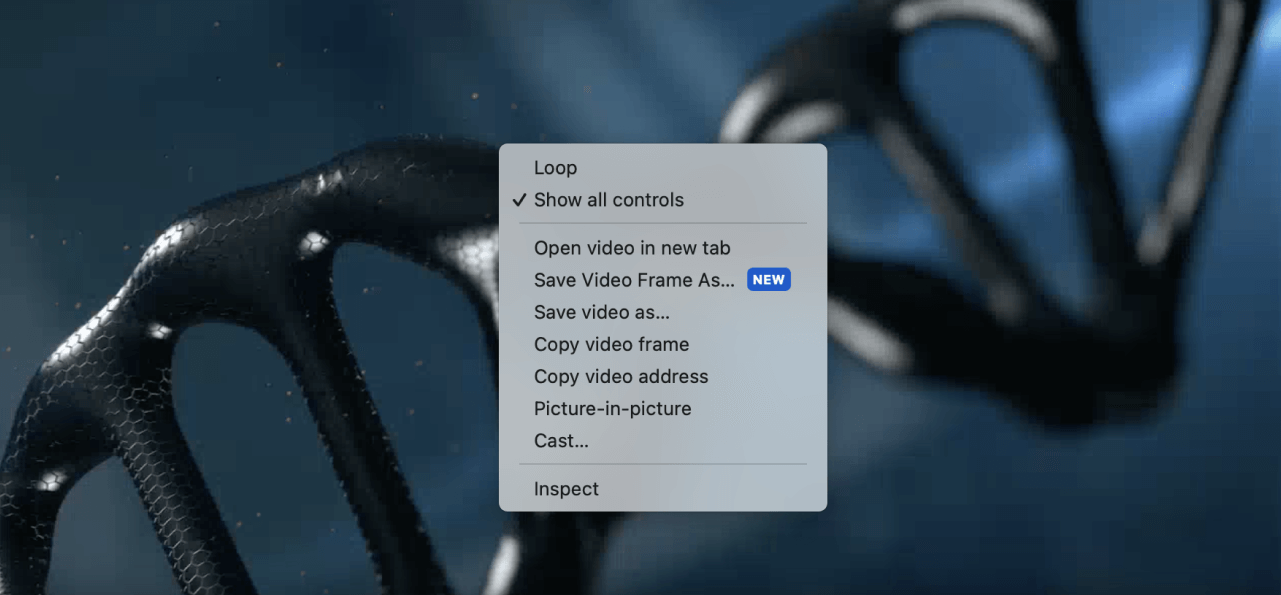
So, disabling right-click simply means stopping the above context menu from appearing.
We can disable right-click in two easy steps:
- Listen to the "contextmenu" event on the target element
- Prevent the default behaviour of the "contextmenu" event, that is, prevent the context menu from appearing using the
event.preventDefault()
method.
Step 1: Listen to the "contextmenu" event on the target element
You already know that a "click" event will help us listen to mouse clicks and finger taps on the HTML elements or the entire HTML document.
Similarly, the "contextmenu" event will help us listen to a right-click on any HTML element or the entire HTML document.
The "contextmenu" event triggers whenever the user right-clicks on the web page because the context menu only appears when we right-click.
Come on, put the following code inside the index.js
file to see how the "contextmenu" event works:
//Step 1A: Select the video element
const video = document.querySelector("video");
//Step 1B: Attach contextmenu event listerner to it
video.addEventListener("contextmenu", function(event){
console.log(event.target);
});
We don't want to disable the right-click on the entire web page.
We just want to disable the right-click on the video
element so that it can't be downloaded, but the rest of the page remains accessible.
So, in the above snippet, we first selected the video
element as the target element and then attached a "contextmenu" event listener to it.
This will now detect a right-click on the video
element.
To test if everything is working properly, we are console-logging the event
object's target
property:
console.log(event.target);
The event.target
property will give us the element on which the right-click was made.
So, when we right-click on the video element, event.target
will give us the <video>
element.
Come on, open up the browser and test it out:
As you can see, the browser triggers the "contextmenu" event only when we right-click on the video element. The event is not getting triggered when we right-click anywhere else on the page.
Only <video>
element getting printed to the console is proof of this.
This also means that the event is getting generated as expected.
But, although the "contextmenu" event is getting is generated, the context menu still appears.
This is because we are not preventing the default action of the "contextmenu" event, that is, opening up the context menu.
How do we prevent it?
Simple.
Step 2: Preventing the default behaviour of the "contextmenu" event, using the event.preventDefault()
method.
The event.preventDefault()
method in JavaScript is used to prevent the default behavior of an event.
This is necessary when you want to override or customize the default action associated with a particular event.
For example, The default behavior of a click
event generated on a hyperlink is to take you to the target website:
<a href="https://google.com">Visit Google's website</a>
Simply put, clicking on the above hyperlink should take you to Google's website.
anchorElement.addEventListener("click", function(event){
//This will prevent the link from opening up the website
event.preventDefault();
});
But if you can call the event.preventDefault()
method when the above hyperlink's click
event is generated, the link will stop working.
That is, it will not take you to Google's website:
Similarly, the default behavior of a contextmenu
event generated on a video element is to open up the context menu with an option to save the video.
But if you can call the event.preventDefault()
method when the contextmenu
event is generated on the video, the context menu with "Save video as" option will not appear:
//Select the video element
const video = document.querySelector("video");
//Attach contextmenu event listerner to it
video.addEventListener("contextmenu", function(event){
console.log(event.target);
//This will disable right-click on the video element
event.preventDefault();
});
In the above code, as soon as the "contextmenu"event is generated on the video element, we are calling the event.Preventdefault()
method inside the event handler.
Because of this, if you now try to right-click on the video, the context menu doesn't appear, no matter what you do.
There are workarounds, but not everybody knows them, and the client thinks that his client is not capable of using those workarounds.
So, our task is now accomplished, all thanks to the event.preventDefault()
method.
There are many other use cases for the event.preventDefault()
method, such as...
Override default keyboard shortcuts associated with certain key combinations.
document.addEventListener('keydown', function(event) {
if (event.key === 'Enter' && event.ctrlKey) {
event.preventDefault();
// Custom handling for Ctrl+Enter key combination
}
});
Prevent the default form submission behavior to perform client-side validation before submitting the form to the server.
document.getElementById('myForm').addEventListener('submit', function(event) {
event.preventDefault();
// Custom form validation logic
});
That's all you need to know about the event.preventDefault()
method for now.
Starting from the next lesson, we will understand how to work with forms.