Some examples of the "Else If" statement in use
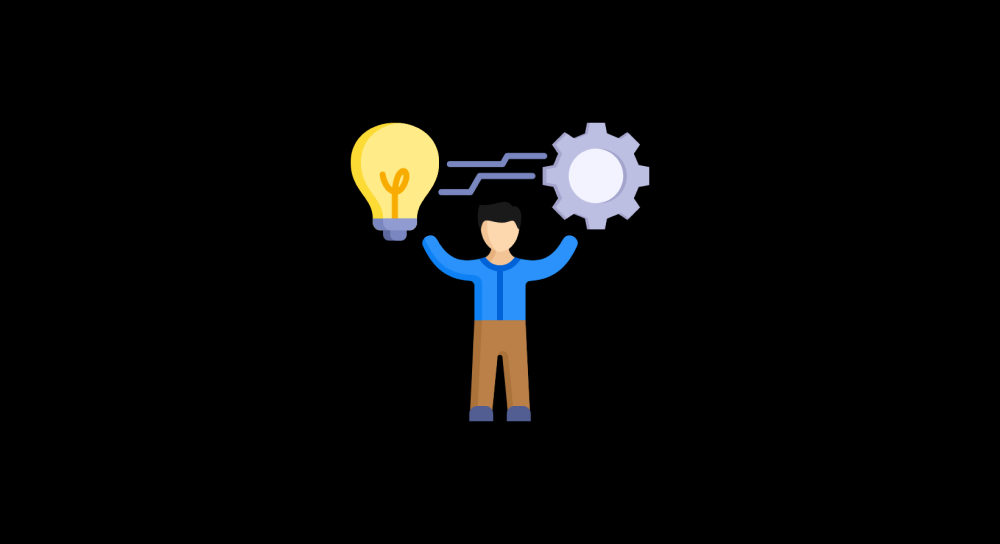
1) Show the library's loan period based on the material type
In a library, various materials such as books, magazines, DVDs, and special collections might have different loan periods due to their varying levels of demand, value, or usage.
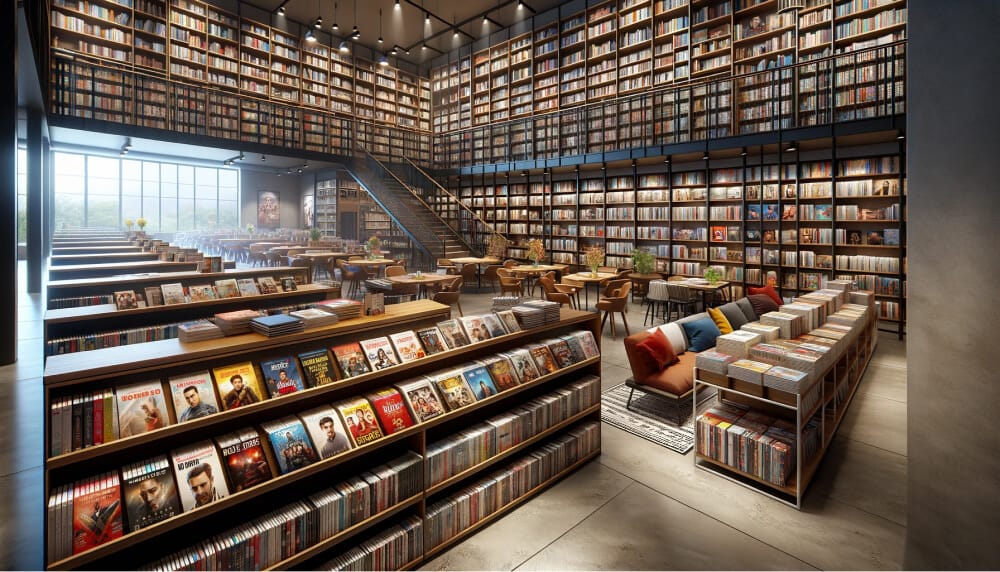
The task at hand: We have to display the loan period for the library material based on the type of material.
Here are various loan periods based on the material:
- Book - 3 weeks loan period
- Magazine - 1 week loan period
- DVD - 2 days loan period
Solution: The solution involves determining the material type and displaying the correct message.
advise
if (materialType === 'book') {
return '3 weeks loan period';
} else if (materialType === 'magazine') {
return '1 week loan period';
} else if (materialType === 'DVD') {
return '2 days loan period';
} else {
return 'Special material, consult the librarian';
}
In the above code, we are getting the data for the materialType
variable from the user and then
- First Condition (Books):
if (materialType === 'book')
: This checks if the material type is a book.- If the condition is true (the material is a book), we are returning
'3 weeks loan period'
.
- Second Condition (Magazines):
else if (materialType === 'magazine')
: This is checked if the first condition fails (the material is not a book). It checks if the material is a magazine.- If this condition is true, it returns
'1 week loan period'
. Magazines have a shorter loan period due to their more frequent publication cycle.
- Third Condition (DVDs):
else if (materialType === 'DVD')
: This condition is evaluated if both the previous conditions (book and magazine) are false.- If the material is a DVD, it returns
'2 days loan period'
. DVDs typically have a much shorter loan period, possibly due to high demand and limited copies.
- Default Case:
else
: This block executes if none of the above conditions are met.- This means the material type is neither a book, a magazine, nor a DVD. It returns
'Special material, consult the librarian'
, suggesting that some materials may have unique loan periods and should be individually checked with the librarian.
Straight forward enough?
One more example...
2) Check for the password strength and provide recommendations
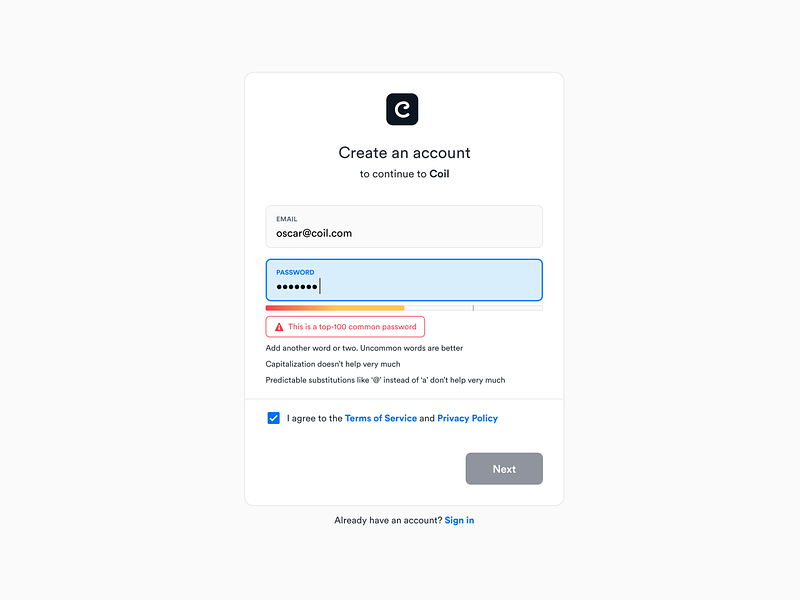
We usually see this functionality in websites or applications during user registration or password change processes.
It is important to force the users to have a strong password for their accounts. Otherwise, they will end up using hackable passwords like 12345
🥞
The task at hand: We must enforce a strong password.
Here are the characteristics of a strong password:
- It must be at least eight characters long
- It must contain special characters, such as
*_
- It must contain letters and numbers
Solution: When we get the password from the user, we need to check for the above best practices and then advise accordingly.
We can easily solve this problem by using multiple else if
conditions:
function checkPasswordStrength() {
let password = document.getElementById('password').value;
let strengthMessage = document.getElementById('strengthMessage');
if (password.length < 6) {
strengthMessage.textContent = 'Strength: Too weak (too short)';
strengthMessage.style.color = 'red';
} else if (password.length < 8) {
strengthMessage.textContent = 'Strength: Weak';
strengthMessage.style.color = 'orange';
} else if (/\d/.test(password) && /[a-zA-Z]/.test(password)) {
strengthMessage.textContent = 'Strength: Moderate (includes letters and numbers)';
strengthMessage.style.color = 'blue';
} else if (/\d/.test(password) && /[a-zA-Z]/.test(password) && /\W/.test(password)) {
strengthMessage.textContent = 'Strength: Strong (includes letters, numbers, and special characters)';
strengthMessage.style.color = 'green';
} else {
strengthMessage.textContent = 'Strength: Moderate';
strengthMessage.style.color = 'blue';
}
}
Don't worry if you don't understand the above code 😄
It uses "Regular Expressions" to determine if the password contains a best practice.
Anyway, here is what's happening in the above code:
- Getting the Password: The function first retrieves the value of the password input field using
document.getElementById('password').value
. - Weakness Due to Short Length:
if (password.length < 6)
: The first condition checks if the password length is less than six characters.- If true, it sets the
strengthMessage
text to indicate that the password is 'Too weak' due to being too short, and changes the text color to red.
- Basic Strength (Length-based):
else if (password.length < 8)
: This checks if the password length is less than eight but more than or equal to 6.- If true, the password is considered 'Weak', and the message and color are updated accordingly.
- Moderate Strength (Letters and Numbers):
else if (/\d/.test(password) && /[a-zA-Z]/.test(password))
: This checks if the password contains both digits (\d
) and letters ([a-zA-Z]
).- If true, the password is considered 'Moderate' in strength and includes both letters and numbers, with the message and color set to reflect this.
- Strong Password (Letters, Numbers, and Special Characters):
else if (/\d/.test(password) && /[a-zA-Z]/.test(password) && /\W/.test(password))
: This condition checks for a combination of letters, numbers, and special characters (\W
).- If the password meets these criteria, it is labeled 'Strong', and the message is displayed with a green color.
- Default Case (Moderate):
else
: This block is executed if none of the above conditions are met.- The password is considered 'Moderate' by default, perhaps because it meets the length requirement but doesn't have a mix of character types.
The conclusion
In a future module, after learning regular expressions and strings in a good way, we will work on the Password strength checker project in a practical.
But before that, let's understand the type of lists in programming and how to work with them in a deeper way.
Once we are done with that, we will come back to advanced IF / Else If statements.
Please bear with me.