Project: Discount Modal
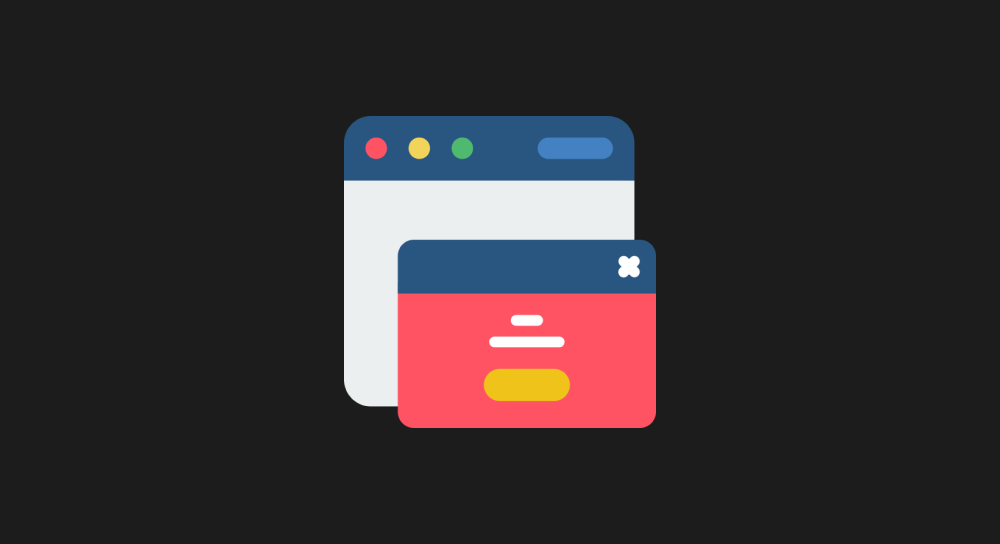
Starting from this lesson, we will learn how to create a modal component in Javascript from scratch.
This project will span several lessons and along the way, you'll learn essential concepts of Javascript such as:
- How javascript depends on HTML and CSS to implement a component.
- How to change CSS styles using Javascript.
- How to create custom function definitions.
- How Event listening and Event handling works.
- How to execute a custom
function
when a button is clicked. - Understand synchronous and asynchronous code in Javascript.
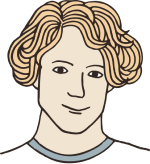
Okay! Bro, what's all this :(
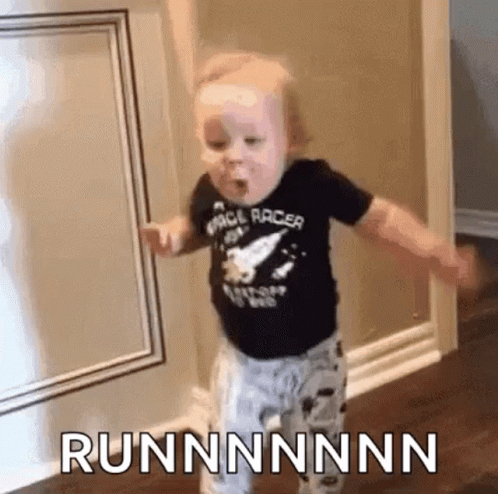
Haha, we have a lot of ground to cover.
But don't get scared. It's easy.
You just have to take some time and read the lessons with patience and following typing, the code along with me will help you a lot.
If you get stuck, just get in touch with me.
Come on, let's get started by answering a simple question.
So, What is a modal?
A modal is nothing but a pop-up that displays content on top of the main page.
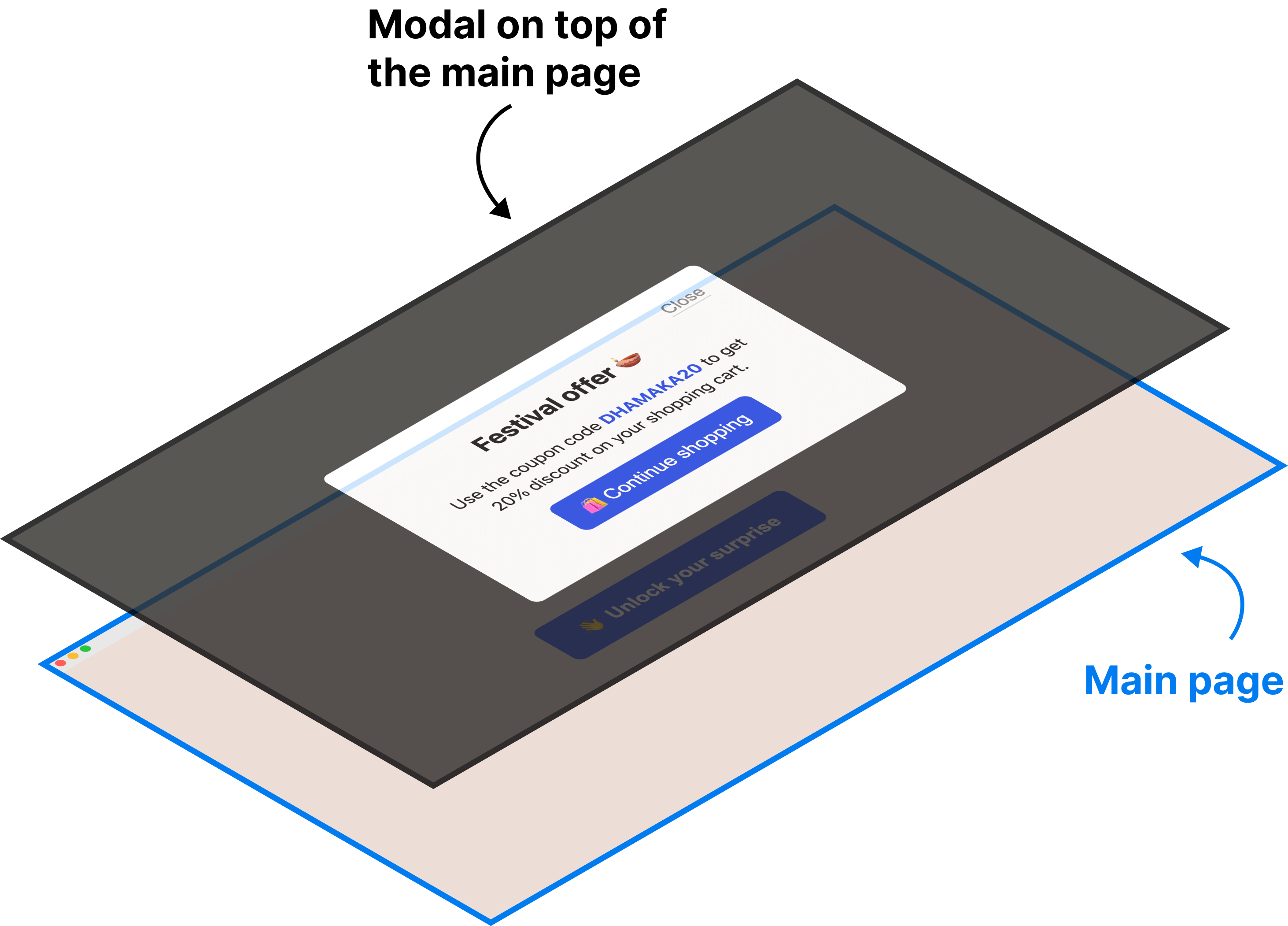
And a modal is usually triggered with a button click from the user.
For example, in the above video, we are popping up some discount information on top of the main page only after the button is clicked.
And this lesson is all about preparing the stage using HTML and CSS.
Creating a component in Javascript is not just about writing the javascript code.
You should also understand how Javascript is collaborating with HTML and CSS for achieving the desired result.
The starter files
Download the project starter files and open the project inside your code editor.
The project folder contains three files:
.
└── modal-starter-files/
├── index.html
├── style.css
└── index.js
First, let's create a button
Open up the index.html
.
It contains very basic HTML that links index.js
and style.css
files to the HTML document.
Next, inside the index.html
file, let's create a button that is used for triggering the modal.
<!-- Making use of container to center the "modal trigger" button on the screen -->
<div class="button-container">
<!-- Modal Trigger -->
<button class="show-modal-btn"><span>👋</span> Unlock your surprise </button>
</div>
Type the above code inside the <body>
tag and just above the <script>
tag.
Next, open up the style.css
file and type the following CSS to style the button:
/* Modal Trigger Button and its container styles */
.button-container{
min-height: 100vh;
display:flex;
align-items: center;
justify-content: center;
}
button{
background-color:#0E36E4;
color:white;
border:0;
border-radius: 8px;
font-weight: 600;
cursor:pointer;
font-size:18px;
padding:15px 30px;
}
button span{
padding-right:5px;
}
As you can see, the CSS code is nothing fancy.
We are just trying to center the button on the web page.
Anyway, If you now preview the index.html
in the browser, you should see the following output:
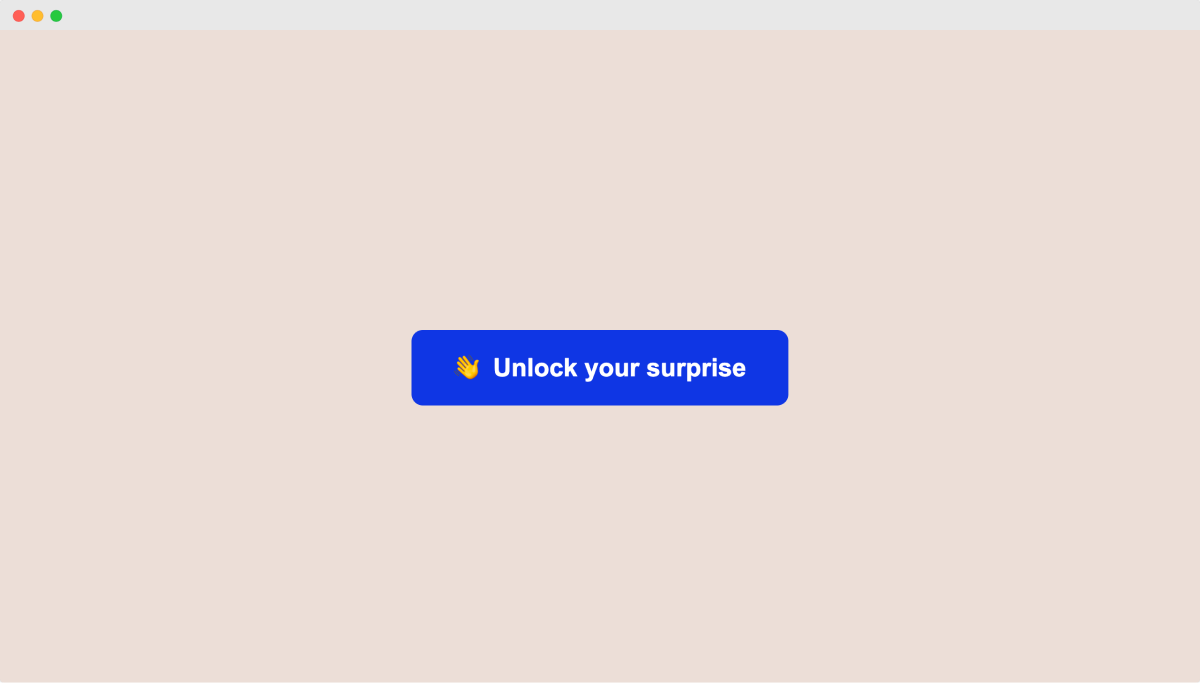
Next, let's create the modal
Open up the index.html
file, type the following HTML at the end of the body
tag and just above the <script>
tag.
<!-- Modal content with overlay-->
<div id="surprise-discount-modal">
<div class="modal-content">
<button class="hide-modal-btn" title="Close the modal">Close</button>
<div>
<h2>Festival offer 🪔</h2>
<p>Use the coupon code <strong>DHAMAKA20</strong> to get 20% discount on your shopping cart.</p>
<button class="continue-shopping-btn"><span>🛍️</span>Continue shopping</button>
</div>
</div>
</div>
Again, nothing fancy going on here.
The modal is just a div
tag with some discount information.
Finally, let's style the modal
Currently, the modal is getting displayed at the bottom of the page and you can only see it if you scroll down.
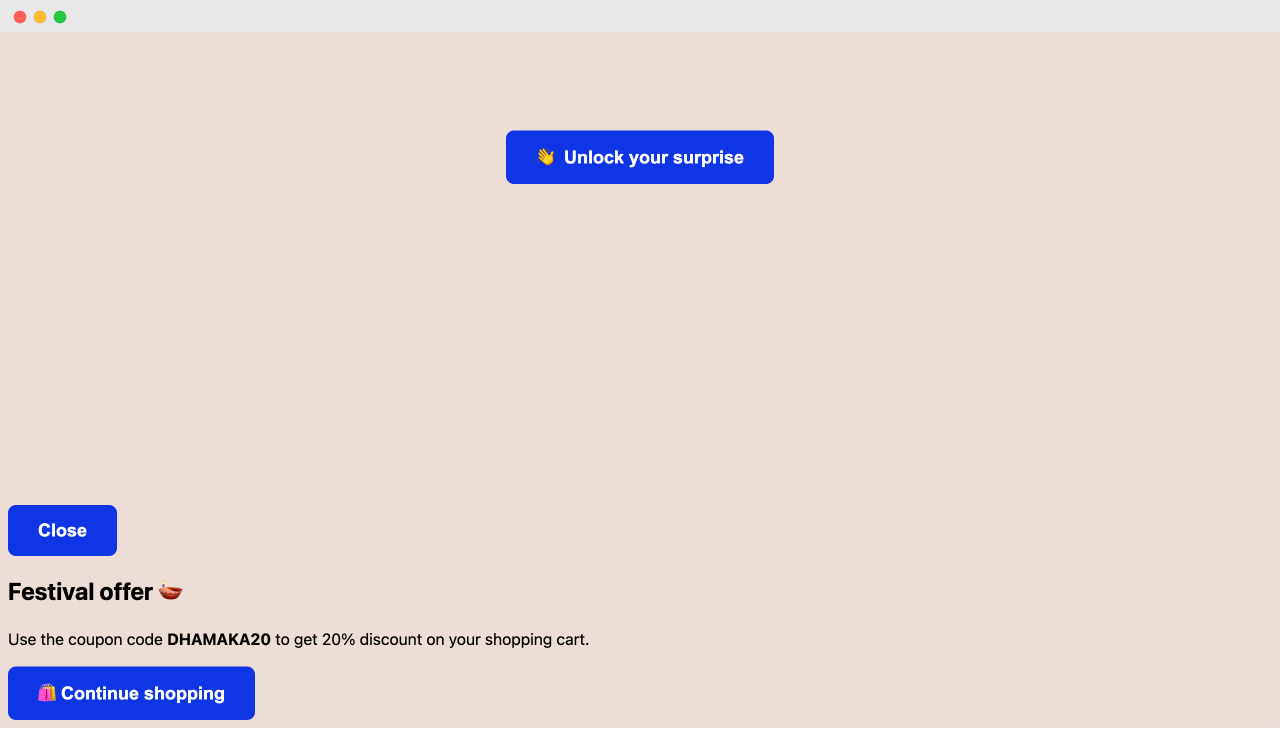
And, if you remember, the modal must be displayed on top of the main page.
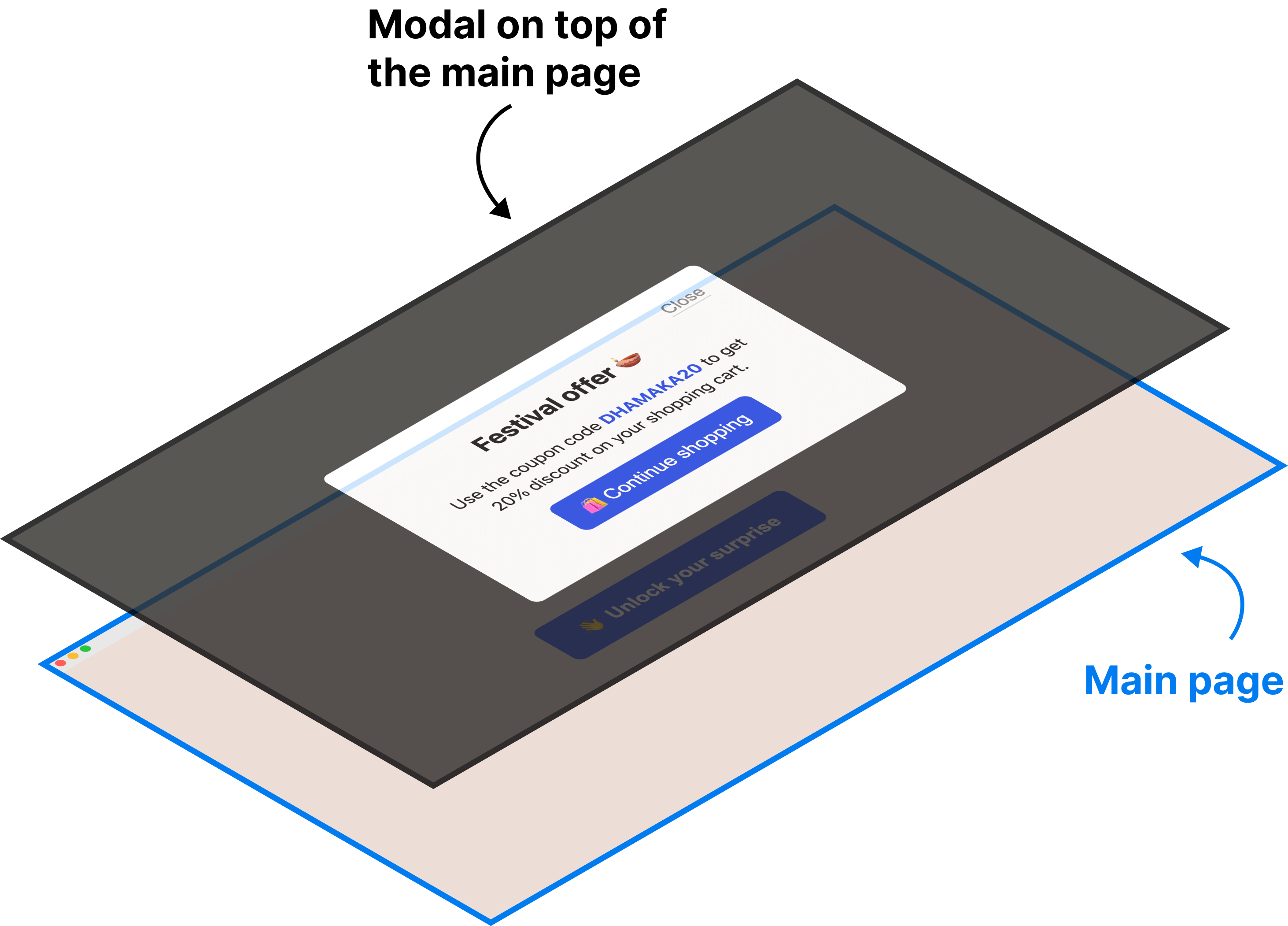
So, using CSS, the modal element must be taken out of the regular document flow and we should make its position:fixed
so that it sits on top of the main page.
#surprise-discount-modal{
position:fixed;
background-color:rgba(0,0,0,0.8);
top:0;
left:0;
bottom:0;
right:0;
}
Type the above code at the end of the style.css
file.
And if you now refresh the browser, the Modal is indeed getting displayed on top of the main page.
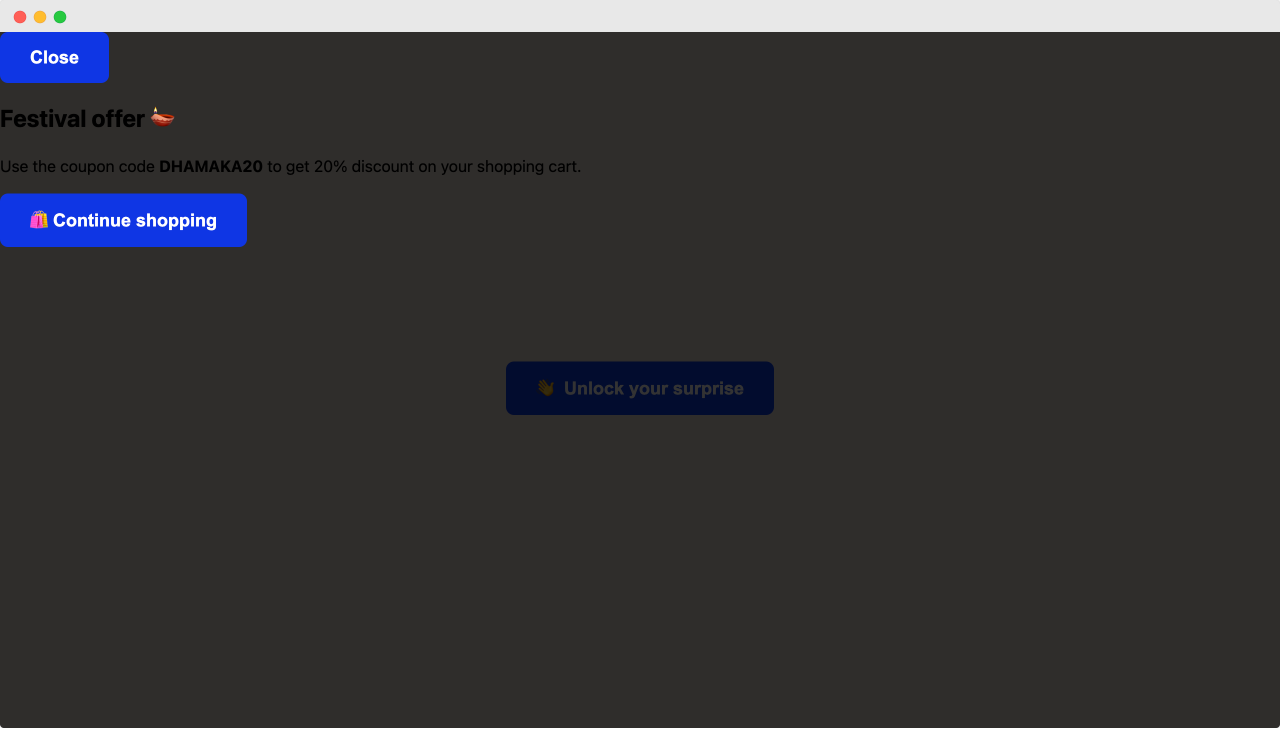
But it is not greatly designed.
Come on, let's style it.
Type the following code at the end of the style.css
file:
.modal-content{
background-color:white;
padding:40px;
border-radius:8px;
text-align: center;
position:absolute;
width: 90%;
max-width:400px;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
box-sizing: border-box;
}
.modal-content h2{
margin-top:0;
margin-bottom:0;
}
.modal-content strong{
color: #0E36E4;
}
.modal-content p{
margin-bottom:0;
margin-top:15px;
}
.hide-modal-btn{
font-size:16px;
color:#777;
background-color:transparent;
border-bottom:1px solid #ccc;
border-radius: 0;
font-weight:normal;
padding:0;
position:absolute;
right:20px;
top:20px;
}
.continue-shopping-btn{
margin-top:16px;
padding:10px 20px;
font-weight: normal;
}
If you now refresh the browser, you should see a well-designed modal:
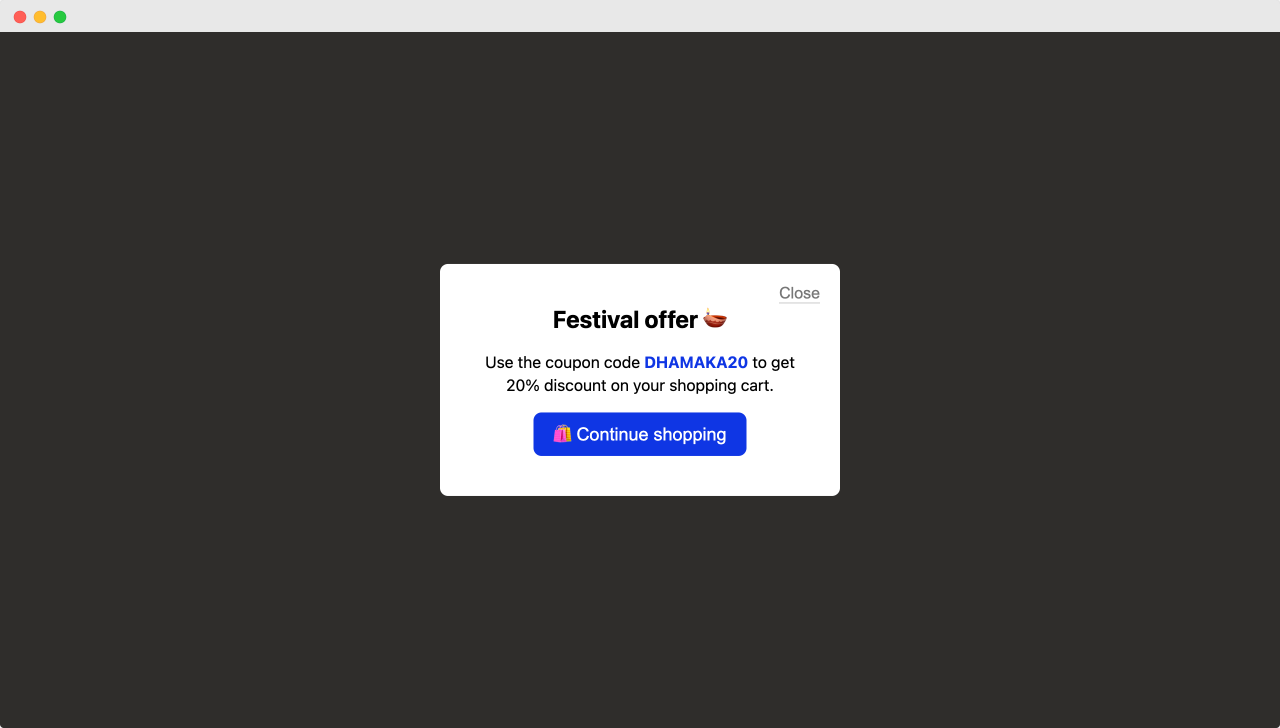
Alright, this is looking great.
But this modal should be visible only when the "Unlock your surprise" button is clicked.
So, let's temporarily hide it by making it display:none
:
#surprise-discount-modal{
position:fixed;
background-color:rgba(0,0,0,0.8);
top:0;
left:0;
bottom:0;
right:0;
display:none;
}
If you now refresh the browser, you should just see the button but not the modal:
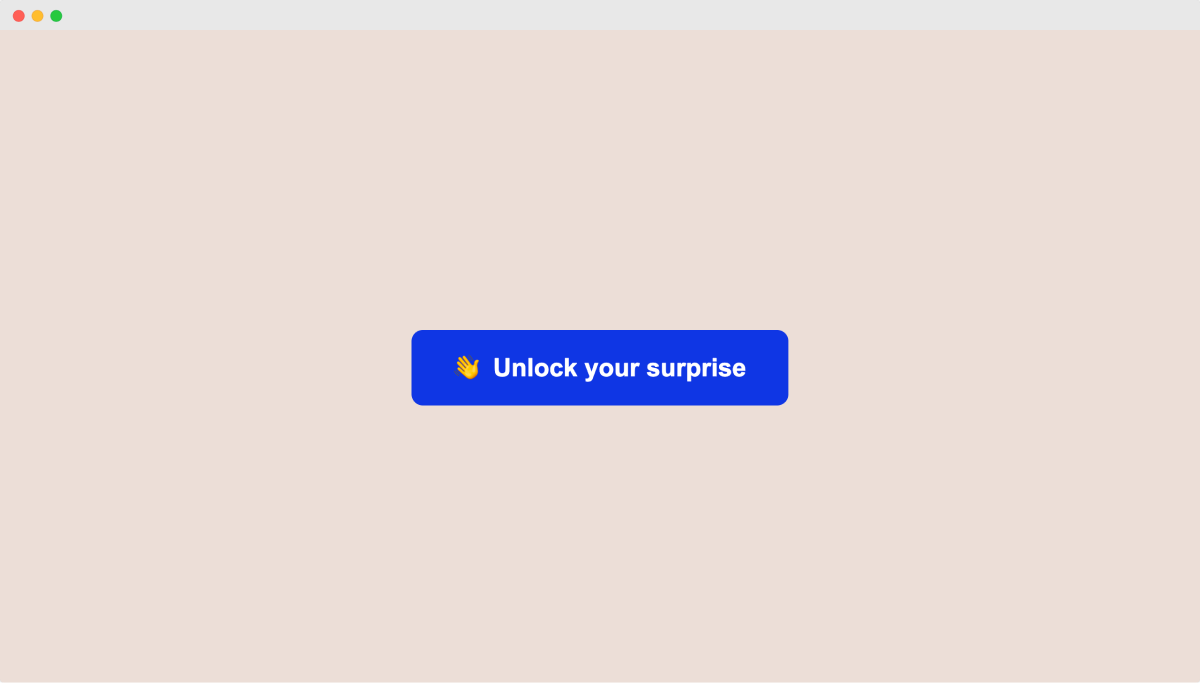
Here are the finished files for this lesson:
All this HTML and CSS code will make our job really easy to open up the Modal when the "Unlock your surprise" button is clicked.
The trick is, whenever someone clicks on the button, we just have to change the style of the Modal to display:block
using Javascript.
And for achieving that, we need to change the CSS styles using Javascript.
We will figure that out in the next lesson.