Project: Collect details
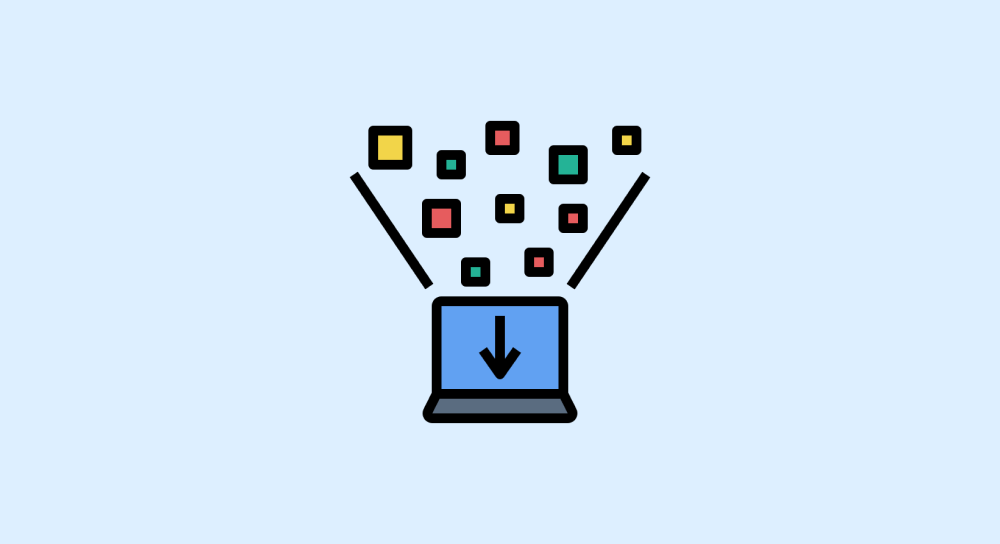
Project idea
In this lesson, we will build a very small project.
We will use the most basic way to collect information from a visitor and we will be collecting their name, age, and country.
Project output
What you'll learn
By the end of this project, you'll cement your understanding about:
- How to use any in-built Javascript function.
- What syntax means and how to use it to avoid errors.
- A bit more understanding of the function parameters.
Download and set up starter files
To get started, download the starter files below:
Next, extract the zip file downloaded and open up the project folder inside your code editor.
If all goes well, you should see the following project structure:
└── 02-know-them/
├── index.html
└── assets/
├── css/
│ └── main.css
├── js/
│ └── main.js
└── images/
└── globe.png
This is one of the most widely used project structures you'll see on the internet.
Now, open up the index.html
in the browser and you should see the following output:
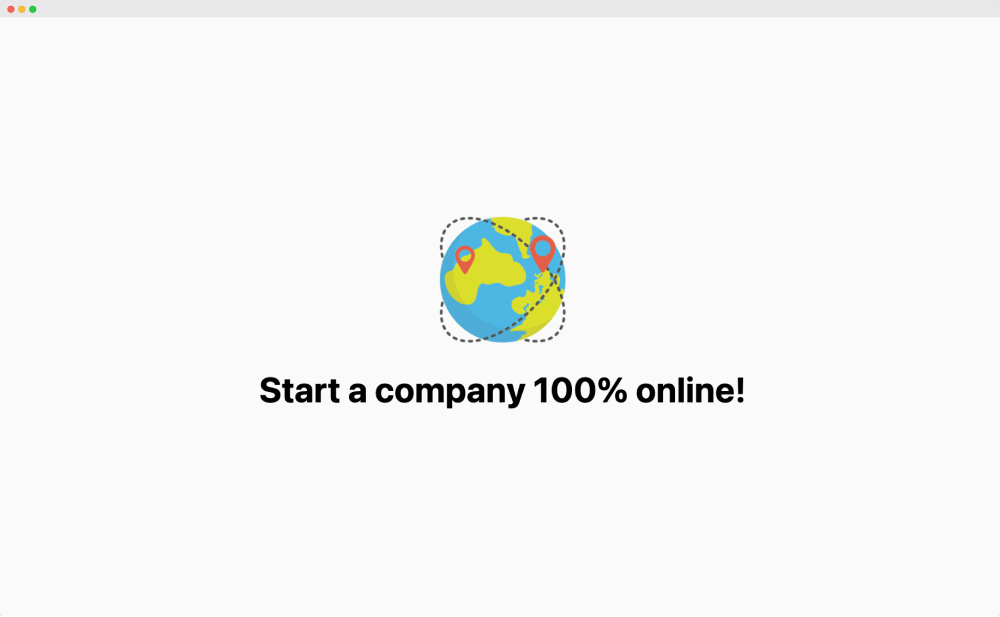
Are you seeing it?
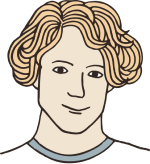
Yep!
Great!
Next, because this is a fictional website for helping people register their company online, It is important that we collect the following details from the users:
- Country
- Name
- Age
And, we will use the prompt
function to get the job done.
And we are going with the prompt
function because we are not yet familiar with the other Javascript features. So, we will improve this project in a future lesson.
Javascript prompt()
In Javascript, the prompt
function allows you to collect a single piece of information from the website visitor.
It is generally useful for asking a question and getting an answer to it.
For example:

If you notice the above image, the prompting dialog box is similar to the alert box.
But this time, instead of just a message, we are seeing a message that acts as the question and a text input field that allows the user to provide an answer.

This is all good.
But I have some questions for you! Consider this as a small quiz.
How do we use the prompt function? How is that question displayed inside the popup?
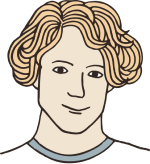
I don't know. So, I have to read the documentation of the prompt function.
Nice thinking!
So, basically, we have to read the syntax of the prompt
function here:
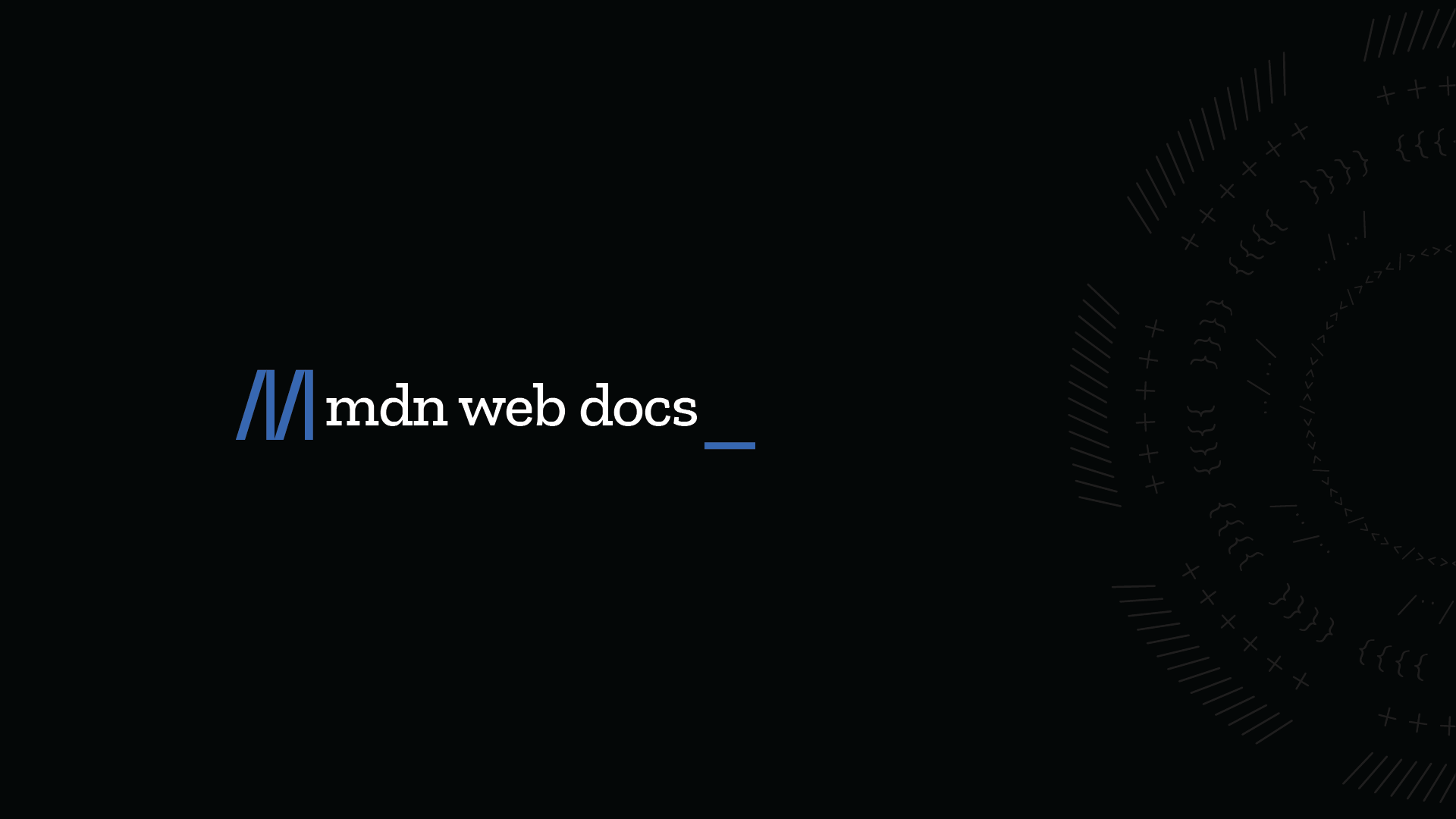
Syntax for the prompt function
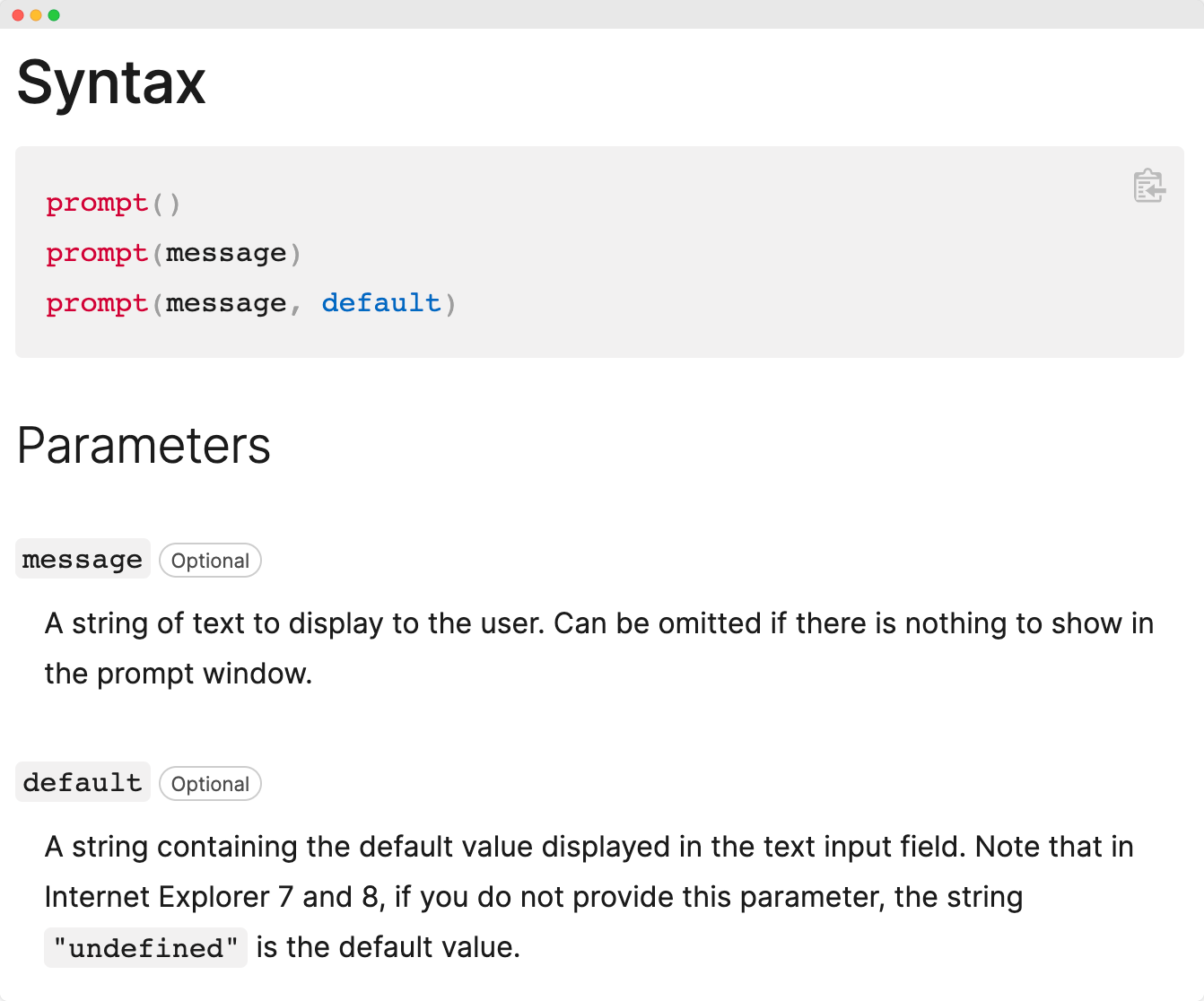
Now tell me one more thing. Based on the above syntax, how many parameters can the prompt
function accept?
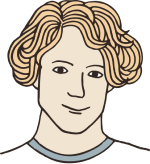
Two!
Correct!
It says that the both parameters are optional. What does that mean?
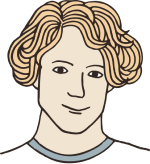
It simply means that we don't have to provide parameters in order for the prompt function to work. But the prompt dialog box is useless if we are not asking any question and getting answer to it.
It looks like you've got a good grip of functions and parameters now.
Anyway, now let's take a quick look at the parameters of the prompt function.
Parameters of prompt()
message
- This parameter will help us ask a question to the visitor. For example "Which country do you live in?"default_value
- This parameter will help us provide a default value for the user's answer. For example "India".
So, do we have all the information for using the prompt function?
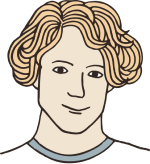
Yep!
Great!
It's time to collect the details.
First, let's go back to the code editor where the starter files are opened.
Next, open up the main.js
file inside the code editor.
Just to recap, we have to collect the following information from the user:
- Country
- Name
- Age
So, first, we will try to get the user's country name.
And for that, we can use the following syntax of the prompt
function:
prompt(message, default);
But we can't put the above syntax inside the main.js
file directly.
We have to convert that syntax to use actual data like this:
// Collect the Country
prompt("Heya, Welcome! Which country do you live in?", "Canada");
Anyway, write the above line of code inside the main.js
file and refresh the index.html
in the browser.
This is what you should see:
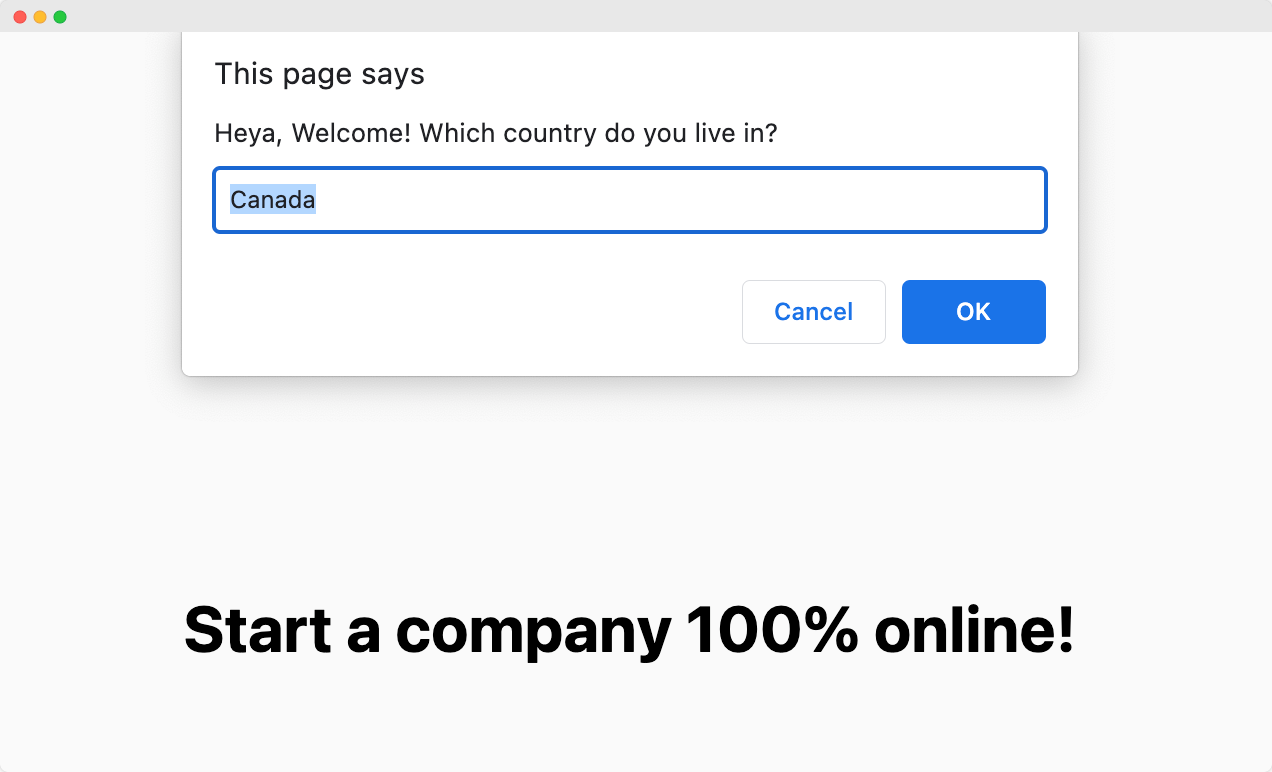
As you can see, both the message
and default
inputs are being put to good use by the browser.
According to the fictional Google Analytics data about my website, most of my customers are from Canada.
So, I provided a default value so that I can save the time of the user if he/she is from Canada.
Users from the rest of the world will have to remove the default value from the input field and should type in their own country manually.
Next, we have to collect the Name and the age.
But how do we do that?
The prompt
function can only collect a single piece of data at a time.
How can we collect more?
A Function can be used as many times as you want
The coffee machine is not made for one-time use, right?
You'll use it every time you want to drink a coffee.
In other words, you can use it any number of times.
Similarly, a function can be used any number of times in the life cycle of a computer program.
In fact, performing a repetitive task, again and again, is the only reason why functions exist in programming.
In other words, a function is usually created to be used a lot of times.
This means that we can use the prompt
function any number of times.
In our case, we want to collect the Name and the age as well, right?
So, here is what we can do with ease:
// Collect the Name
prompt("Great! What is your name?");
// Collect the Age
prompt("Got it! One last question. What is your age?");
Now, don't copy and paste the above code directly inside your main.js
file.
Type the above code manually by understanding each line of code.
Are you done typing? And Did you save the main.js
file?
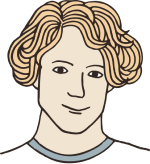
Yes! But, why didn't you provide the default parameter for collecting the name and age?
The default value makes more sense when asking the visitor about their country, zodiac sign, etc.
Simply put, it makes sense only if we can guess the information provided by the visitor.
With names, that's not the case, isn't it? :D
If you now open up the index.html
in the browser, you'll see the Browser asking you two more questions one by one.
Nice, isn't it?
Finished Files:
But where is the information going?
We have programmed the browser to collect the details from the visitor by prompting them with questions.
And imagine that a visitor answered all those questions.
Where are those answers provided by the visitor?
And how can we access them?
We will figure that out in the next lesson.