Nested objects
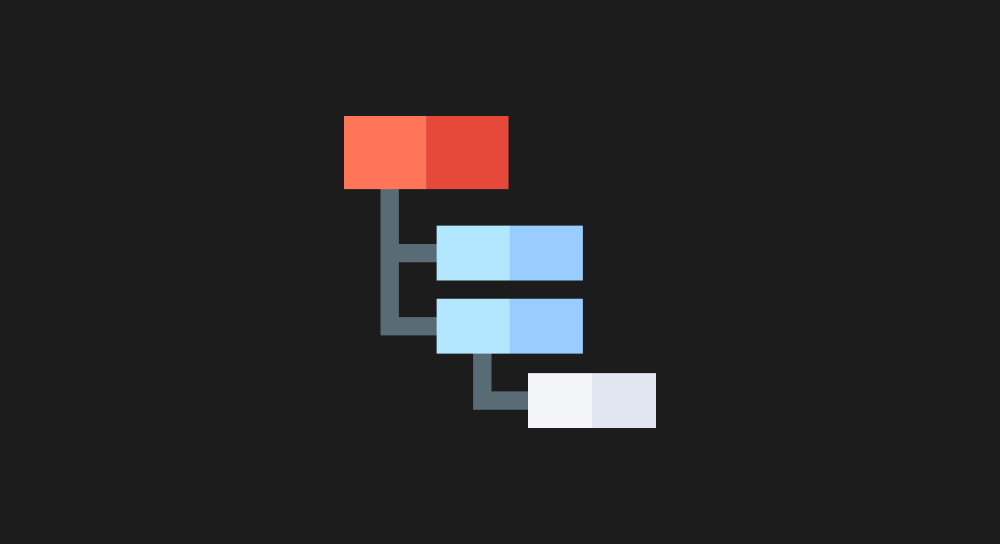
Most of the time, objects will have child objects in Javascript.
Simply put, objects inside of another object.
Here is an example of the bankAccount
object.
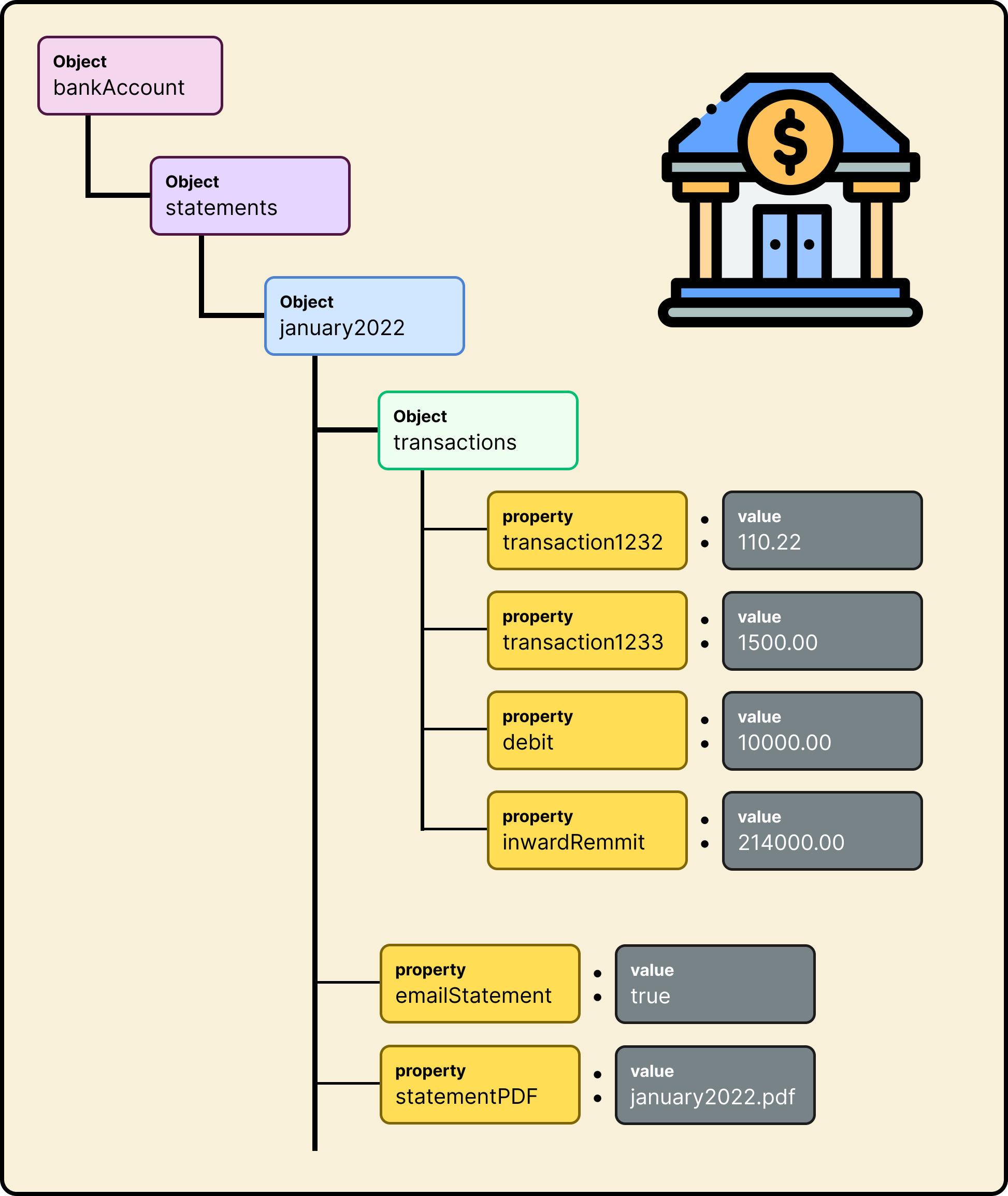
I want you to take a moment and understand the bankAccount
object illustrated above.
It has three levels of child objects.
If we translate the above illustration to an actual Javascript object, it looks like this:
let bankAccount = {
// Level 0
statements: {
// Level 1
january2022: {
// Level 2
transactions:{
// Level 3
transaction1232: 110.22,
transaction1233: 1500.00,
debit: 10000.00,
inwardRemmit:214000.00
},
// Rest of the level 2 properties
emailStatement: true,
statementPDF: "january2022.pdf"
}
}
};
Now, let's see how to access those nested objects and their properties.
Come on, open up the Browser Console and type the above code inside the Console.
Spartanssss. Try it out with meeeeee.
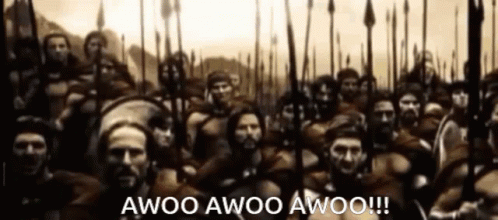
How to access nested objects
You can access child objects just like any other property of the parent object.
For example, let's see how to access the statements
object that is a direct child of bankAccount
object.
bankAccount.statements;
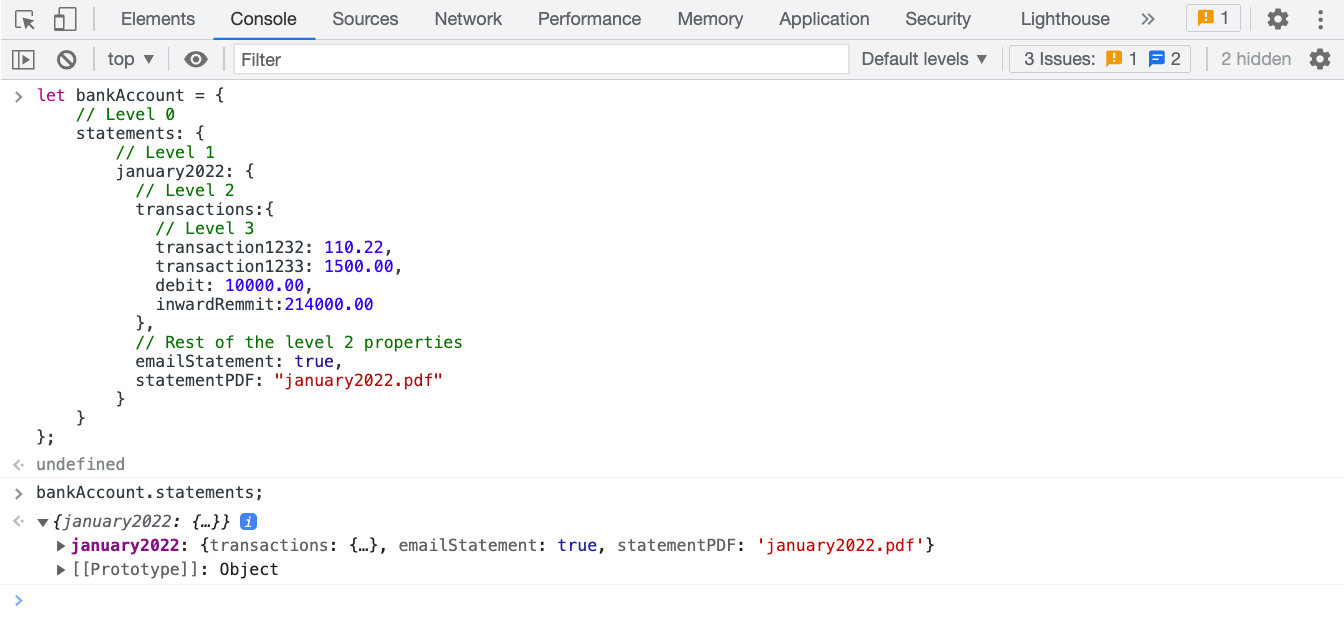
Now let's go to another level deep.
Let's see how to access the january2022
object which is a child of statements
object and grand-child of bankAccount
object.
bankAccount.statements.january2022;
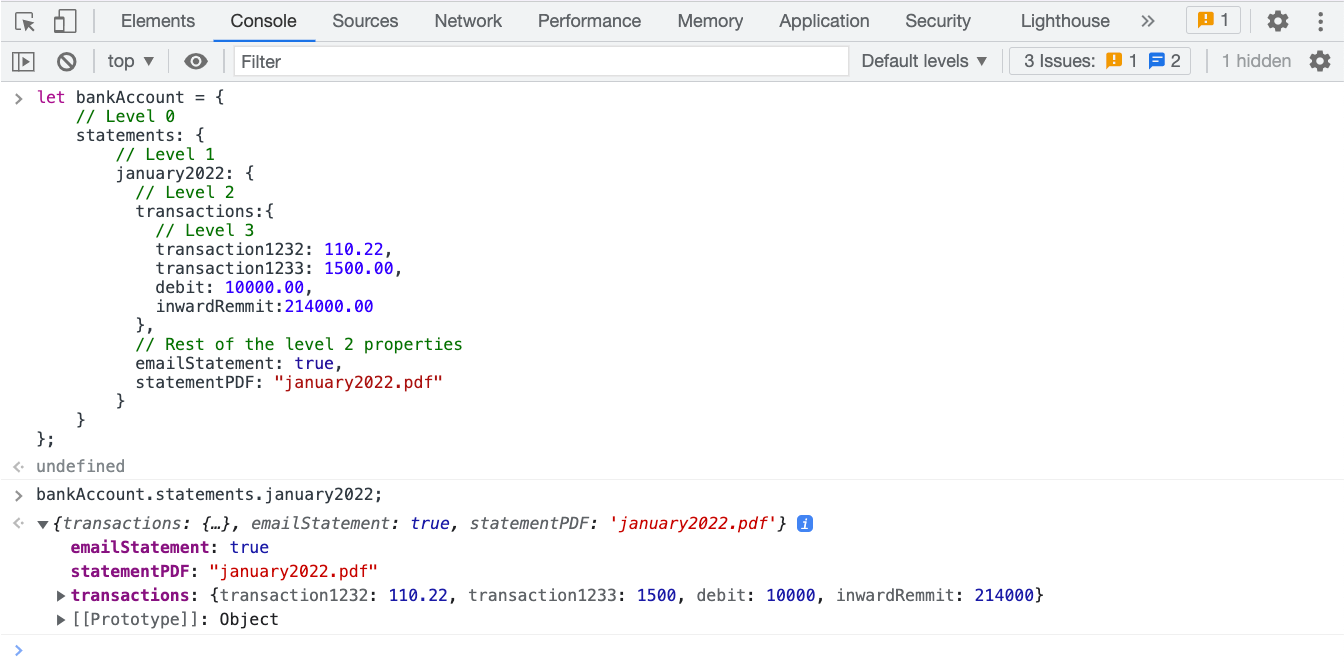
As you can see, in order to access the january2022
object, we have to start from the root object (level 0) and then go down the chain.
- We are first accessing the
bankAccount
object (root object). - Then, we are accessing the
statements
object (child object). - Finally, we are accessing the
january2022
object (grand-child object).
This is mandatory.
One last example, I want to access the debit
property of the transaction
object and here is how I do it:
bankAccount.statements.january2022.transactions.debit;
You get the idea, right?
It is important that you understand this because you will be doing this a lot in the upcoming lessons.
Anyway, in the next lesson, we will learn another important concept related to objects.