Naming rules for Javascript Variables
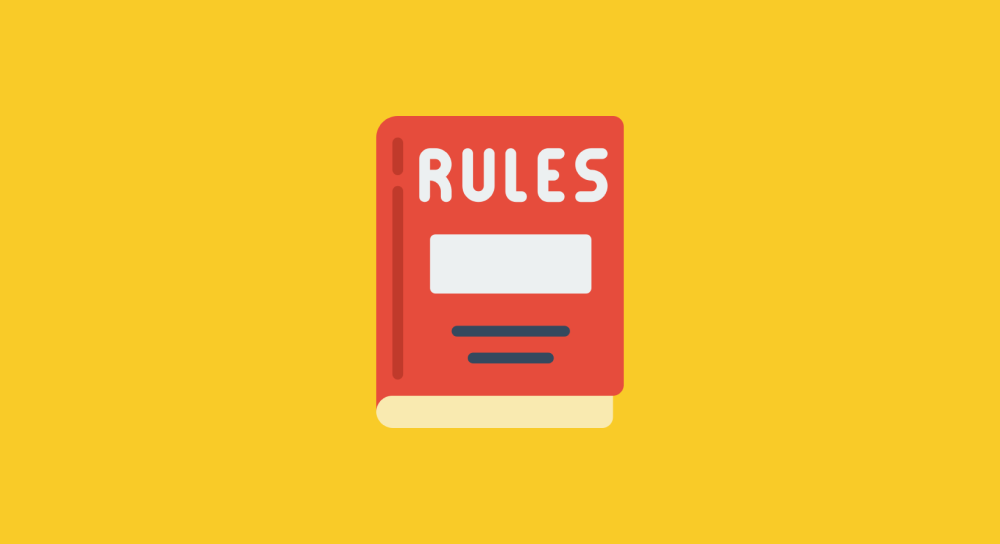
You can not create variables in Javascript without naming them.
And you must follow some rules while naming a variable.
Rule 1: Variable names should only contain letters, digits, _, and $ symbols.
Here are some examples of valid variable names:
let bankAccountNumber;
let bank_balance;
let $factor_of_life;
let _isActive;
If you notice the variable names in the above snippet, they have multiple words as part of their variable name.
And, whenever we have a variable name with multiple words, it is common to use camel case, pascal case, etc.
For example, When it comes to the bankAccountNumber
variable name, we are using the camel case.
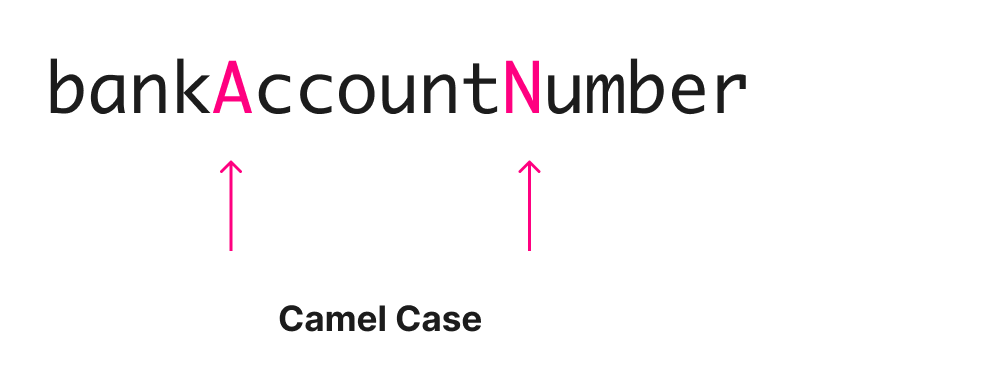
In the camel case pattern, the first letter of the first word is small and the remaining words will have their initial letters in a capital case.
When it comes to the bank_balance
variable name, we are using the snake case.
When using the snake case pattern, the words in the variable name are separated with an underscore(_
).
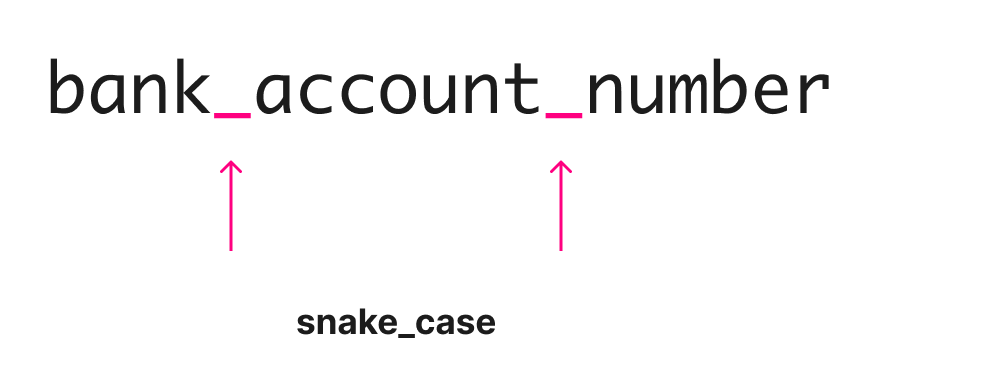
You can also name variables using a single character
For example:
let a = 10;
let b = 20;
let $ = "Some string";
let _ = "Some other string";
These are valid variable names but it is a bad practice because it hurts the code readability.
Basically, when you name variables, use descriptive names such as bank_balance
or age
.
This way, if you stumble up a code you have written six months ago, you can still understand what kind of information we are dealing with.
Case Matters
Variables named bankAccount
, bankaccount
, and BANKACCOUNT
are considered as three different variables.
So, just be careful.
Stick with English words while naming variables
The following variable naming is valid too:
//Variable Name in Chinese
let 爱 = "Love";
//Variable Name in Telugu (My mother tongue :D)
let బ్రిటానికా = "Brittanica";
But it is better to stick with English variable names because your code might end up read by people from other countries and English is the most used language in the Software Industry.
Having said that, let's see more some special don'ts.
Rule 2: Variable names can not start with a digit
For example:
let 12digitalspassword;
This will throw a Javascript Syntax error.

Rule 3: Variable names can not have special symbols like -, &, *,%
And, there is a good reason behind it.
In Javascript code, the hyphen (-
) symbol is considered as a minus operator.
Similarly:
*
is used for multiplication/
is used for division%
is used for getting the remainder of a division.
And they can be used only for mathematical operations like this:
// Performing substraction
let remaningBankBalance = 100000 - 20000;
// Calculating rate of of Interest
let rateOfInterest = ( 100 * 20 ) / 10000;
So, it is obvious for you to not use these special symbols for variable names.
And, we can not have spaces in variable names either.
//Invalid Javascript, throws an error
let remaining - bank - balance = 10000 - 2000;
Did you notice the problem?
Finally, let's talk about keywords.
Rule 4: You can not use reserved keywords to name variables
Javascript programming language reserves some English words for its own usage.
For example, The let
keyword is reserved by Javascript to help you declare variables.
So, you can not use it as a variable name:
//Javacript will throw error
let let = "go of it!"
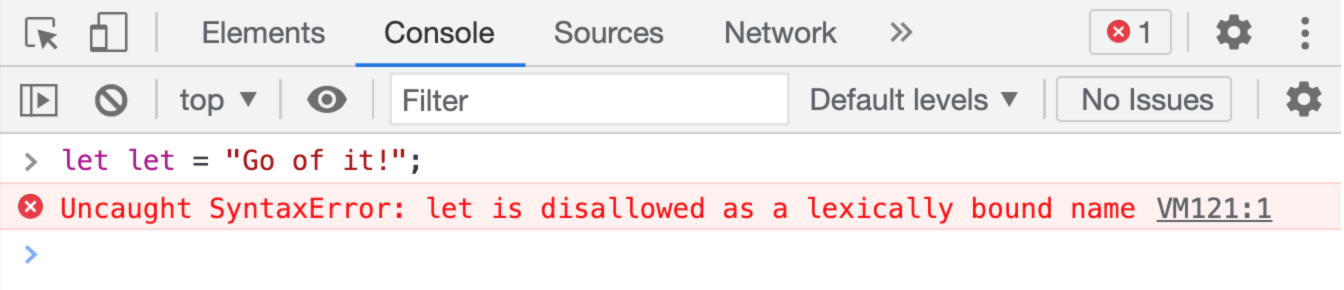
But if you try to use it anyway, Javascript will throw error saying it is disallowed.
Similarly, there are other keywords like for
, else
, return
, function
, etc. that you can not use because they have a specific purpose in Javascript.
Here is the full list of reserved words that you can not use for variable names:
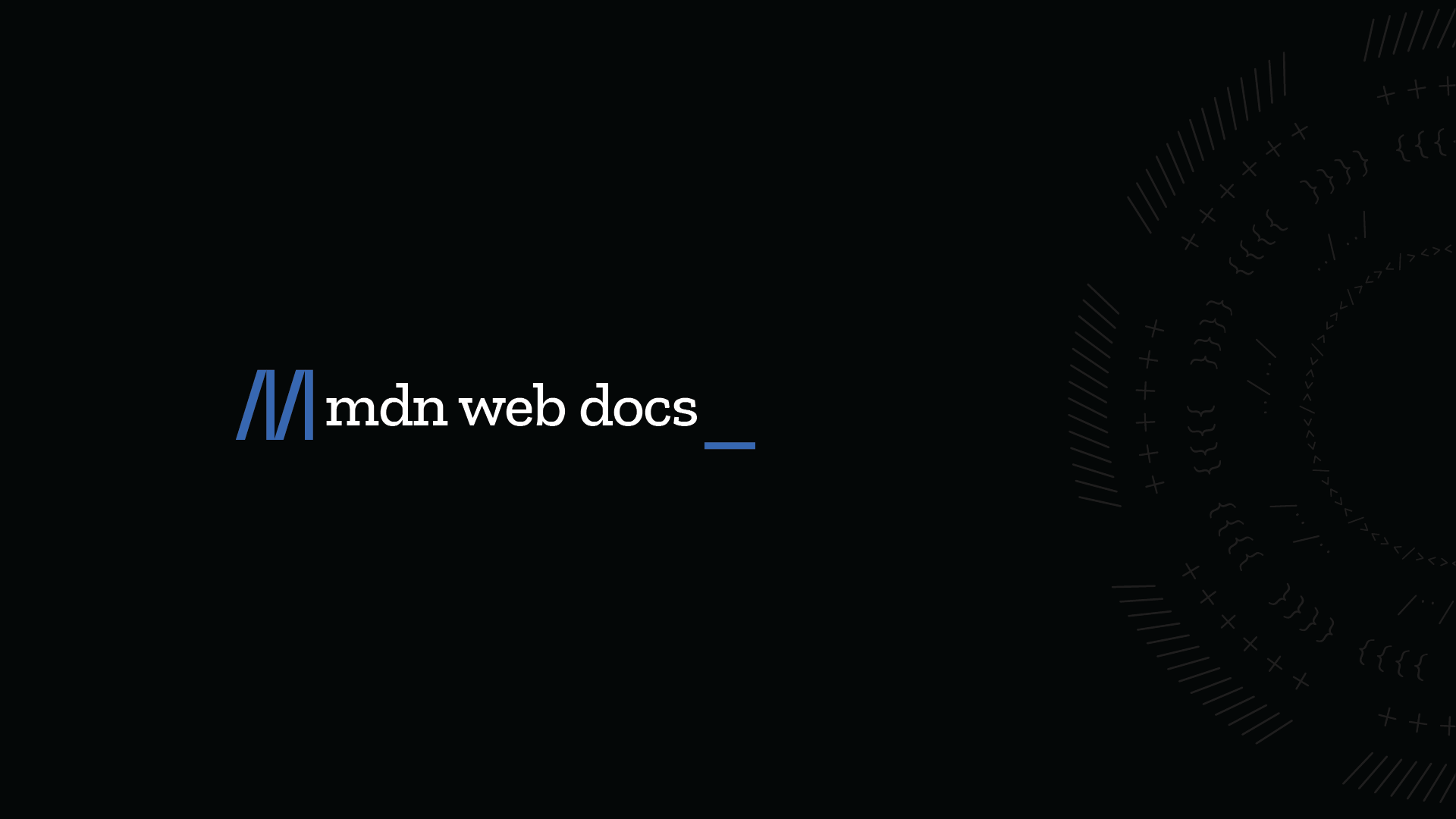
Having said all this, you don't have to remember every one of the reserved keywords.
You'll remember them automatically as you spend more and more time with Javascript.
The Conclusion
That's all you need to know when it comes to naming variables.
In the next lesson, we will learn about the readability of your Javascript code.