Keep the loop performance in mind
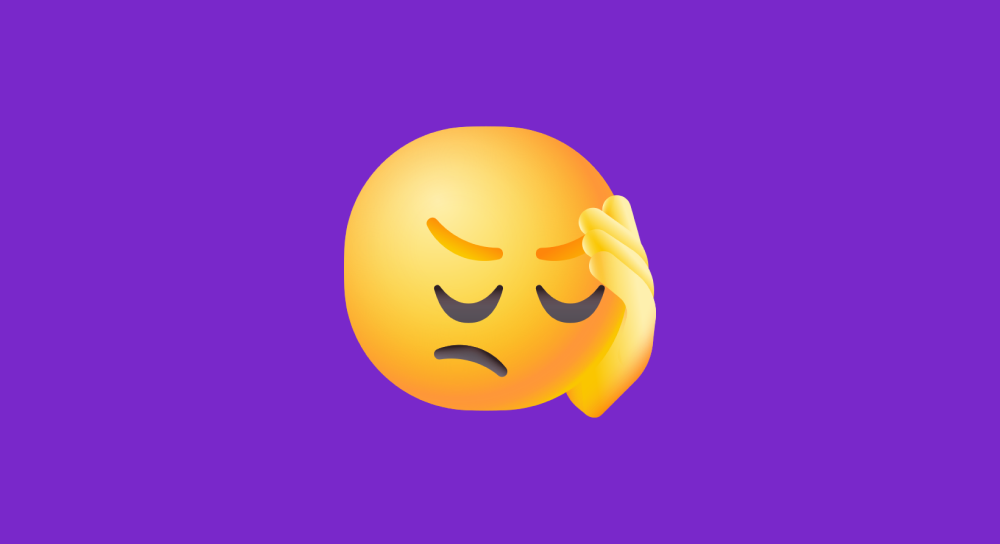
document.querySelector("#unsafe-passwords");
Whenever you select an HTML element using Javascript, a query is performed to find that element and then select it.
And this querying takes a certain amount of time.
For example, if you are selecting an element once, it could take 10 milliseconds (probably faster than that).
So, if you're selecting the same element 20 times, it could take around 200 milliseconds (probably faster than that):
//1st time
document.querySelector("#unsafe-passwords");
//second time
document.querySelector("#unsafe-passwords");
//third time
document.querySelector("#unsafe-passwords");
//fourth time
document.querySelector("#unsafe-passwords");
//...
//...
//twentieth time
document.querySelector("#unsafe-passwords");
You get the idea, right?
Long story short, the large number of HTML selection queries you make via Javascript could slow down the execution time of your entire Javascript file and, therefore, could slow down the entire web page for the site visitor.
So, in this lesson, let's explore the impact of code structure on performance in a basic way.
More specifically, let's see the importance of minimizing HTML selection and manipulation within loops.
We'll compare two similar code snippets and analyze their efficiency to understand why one approach is better than the other.
Scenario
During the last challenge, we have outputted a list of array items into the web page:
let unsafePasswords = [
"123456",
"123456789",
"12345",
"qwerty",
"password",
"12345678",
"111111",
"123123",
"1234567890",
"1234567",
"qwerty123",
"000000",
"1q2w3e",
"aa12345678",
"abc123",
"password1",
"1234",
"qwertyuiop",
"123321",
"password123"
];
const ulElement = document.querySelector("#unsafe-passwords");
for(let i = 0; i < unsafePasswords.length; i++){
const liElement = document.createElement("li");
liElement.textContent = unsafePasswords[i];
ulElement.appendChild(liElement);
}
As part of the process, we selected a <ul>
element using Javascript so that we can create and place <li>
elements inside it.
But if you notice, we selected the <ul>
element only once outside the loop. This means that the selection operation is performed only once.
And then, inside the loop, we reused the same selection every time we inserted an li
element onto the webpage.
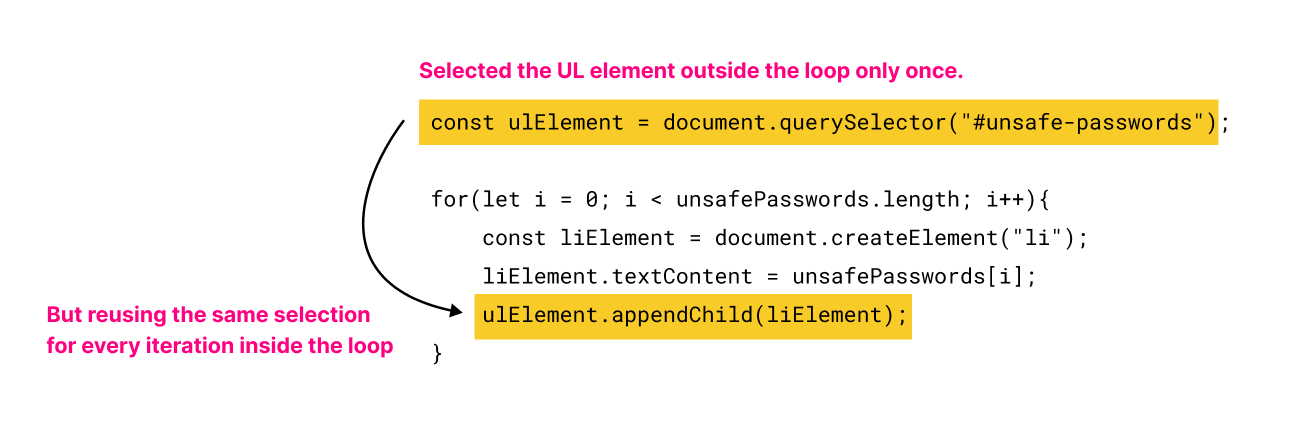
There is nothing wrong with this approach.
Selecting HTML elements outside the loop is the recommended approach.
But sometimes, on the internet or your teammate's Javascript, you'll find something like this:
let unsafePasswords = [
"123456",
"123456789",
"12345",
"qwerty",
"password",
"12345678",
"111111",
"123123",
"1234567890",
"1234567",
"qwerty123",
"000000",
"1q2w3e",
"aa12345678",
"abc123",
"password1",
"1234",
"qwertyuiop",
"123321",
"password123"
];
for(let i = 0; i < unsafePasswords.length; i++){
const ulElement = document.querySelector("#unsafe-passwords");
const liElement = document.createElement("li");
liElement.textContent = unsafePasswords[i];
ulElement.appendChild(liElement);
}
If you notice, this time, the UL element (ulElement
) is selected inside the loop for each iteration.
This results in the selection operation being executed repeatedly, once for every item in the unsafePasswords
array.
This approach is less efficient and can lead to unnecessary overhead.
Long story short, you have to try to minimize HTML queries within loops to avoid performance issues.
In our case, we only dealt with an array of 20 items. So, selecting the UL element (ulElement
) "inside the loop for every iteration" or "selecting it outside the loop only once" doesn't make much difference.
But this definitely becomes a problem when dealing with 2000 items, and it is not an uncommon scenario.
When you're dealing with such a large set of data, inefficient HTML queries can noticeably impact performance.
Users may experience longer loading times, unresponsive interactions, or even browser crashes.
This can lead to frustration, lower engagement, and potentially hurt your website's overall performance.
So, as a best practice, make sure to perform HTML queries outside of the loop, irrespective of whether you're dealing with a smaller set or a larger set of data.
This also applies to other HTML operations such as:
- Creating HTML elements
- Changing the content of HTML elements
Just be mindful of not making extra HTML queries when they are not needed.
Anyway, now that we have a good idea about Arrays and Loops, let's shift our focus to a different kind of list called NodeLists.
We will learn about NodeLists starting from the next lesson.