Introducing the script tag
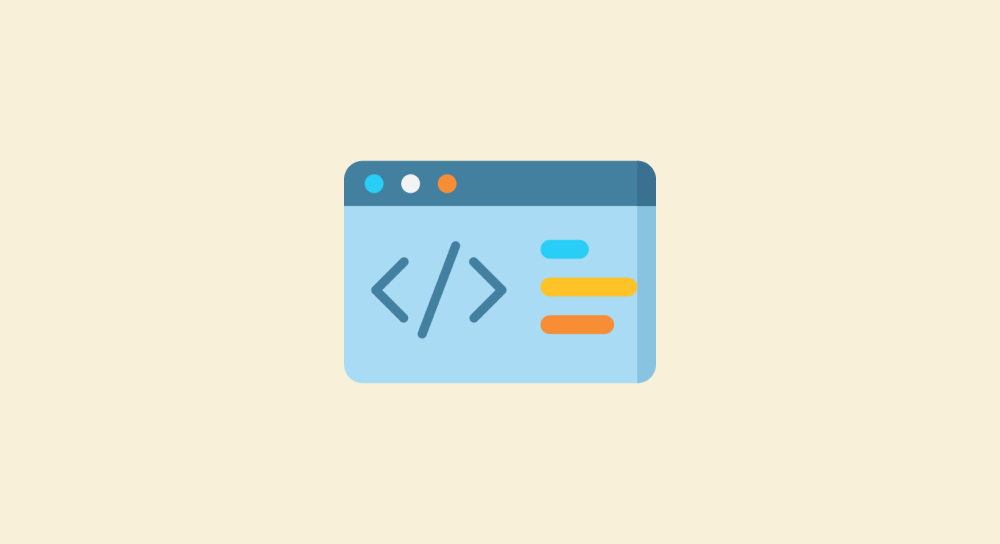
Imagine we fell sick.
And we have to take medicine to get normal again.
How do we take the medicine?
We have to some how get the medicine inside our body, right?
That is the only way it can fix our bodies.
Similarly, if we want to add Javascript-based interactivity to our web pages, we have to put Javascript inside our web pages.
Web pages are built with HTML.
So, we have to use an HTML tag to add Javascript to the web page.
And that tag is the <script>
tag.
Out of all HTML tags, <script>
tag is the only HTML tag that can deal with the Javascript code.
And you can use it in two ways:
- Put the Javascript code inside the
<script>
tag directly. - Put the Javascript code inside a separate file and then link that file to the HTML document using the
<script>
tag.
In this lesson, we will talk about the first method.
You can directly add Javascript code inside the <script>
tag.
For example:
<!DOCTYPE html>
<html lang="en">
<head>
...
</head>
<body>
<p>Some HTML Content</p>
<script>
//Javascript code goes here
alert("Javascript is successfully linked!");
</script>
</body>
</html>
If you notice the above snippet, I have placed the <script>
tag at the end of the HTML document (just before the closing </body>
tag).
You can also put the <script>
tag inside the <head>
tag:
<!DOCTYPE html>
<html lang="en">
<head>
...
<script>
//Javascript code goes here
alert("Javascript is successfully linked!");
</script>
</head>
<body>
<p>Some HTML Content</p>
</body>
</html>
However, it is recommended to put the <script>
tags at the end of the HTML document. More on this later.
Anyway, you can add any amount of Javascript code inside the <script>
tag
<script>
window.dataLayer = window.dataLayer || [];
function gtag(){dataLayer.push(arguments);}
gtag('js', new Date());
gtag('config', 'UA-139834303-1');
</script>
Example of a Google Analytics Javascript Code
If you see the above code, it is only a few lines of code, and it is perfect for going inside the script
tag directly.
But usually, you'll end up writing a lot of custom Javascript code with 100s of lines, if not 1000s of lines.
If that is the case, putting the Javascript code inside the script
tag is no longer recommended.
It is not a good idea to mix Javascript code with HTML code for a lot of good reasons.
For the curious mind: Here is why, with a more elaborate explanation
Readability Perspective:
It hurts the readability of the code around it.
When you write custom Javascript code, you'll often write 100s of lines of code and 1000s of lines of HTML content accompanying it.
The readability of the HTML document is important for writing good HTML or modifying the existing HTML.
So, it is also advised to keep the HTML as lean as possible without any clutter.
Basically, Mixing Javascript with HTML is against this principle and will hurt the readability of the entire HTML document.
In other words, The document becomes messy with HTML and Javascript code.
So, don't do it. Period.
Maintenance Perspective:
It increases the maintenance of the code and could result in errors.
Usually, you'll end up using the same custom Javascript code inside other pages of your website.
For example, imagine writing the Javascript code for implementing an off-canvas menu for the website.
You'll use the same off-canvas menu for other site pages like About Us, Contact Us, etc.
Including the Javascript code for the homepage is not enough if you want the off-canvas menu to work on the other website pages, right?
You'll need to include the same Javascript code inside other pages as well.
In this scenario, if you put your Javascript code inside the <script>
tag instead of a separate Javascript file, then you'll keep copying the same piece of code again and again inside multiple HTML files.
Not only that, sooner or later, you will end up modifying the code, and when you do that, you'll end up editing the code inside other HTML files as well.
Don't you think it is a lot of maintenance? It is.
The solution to this problem is simple.
Put your Javascript code inside a separate file and include that file inside all the necessary HTML files of the website.
And this is why, From a maintenance and readability perspective, it is recommended to write Javascript in a separate file.
And we will learn about it in the next lesson.