How to manage objects
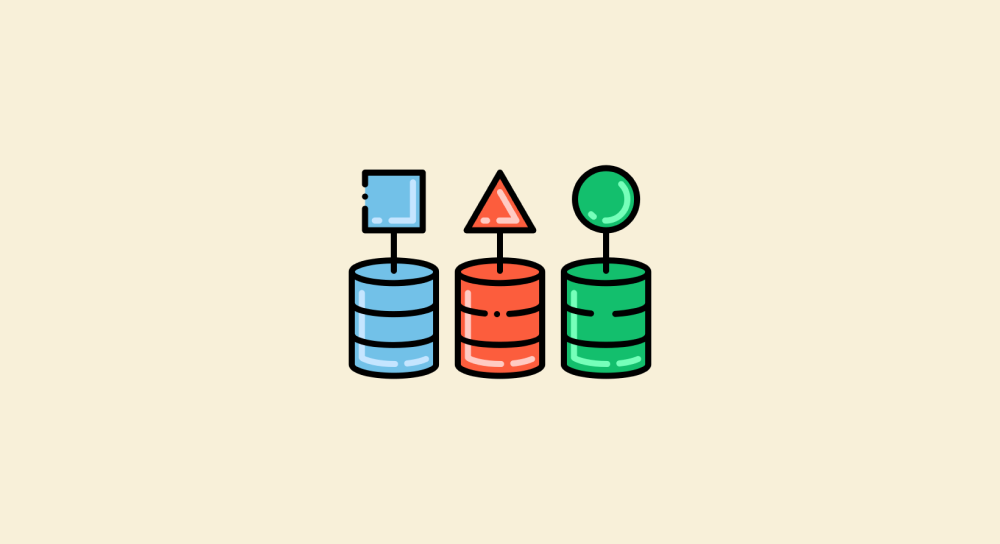
How to access the data inside an object
There are two ways for this.
The Object Dot Notation
We can access the value of a particular property by using something called Object Dot notation.
It simply means that you have to write the name of the object, followed by a dot .
, followed by the name of the property.
Object.propertyName;
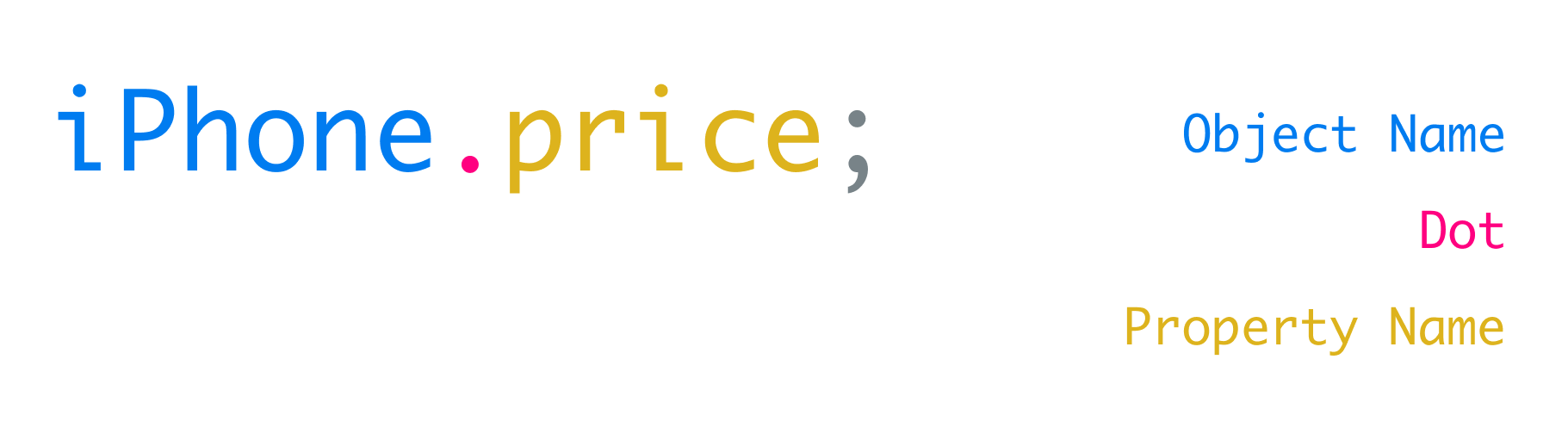
Let's just say you have the information of an iPhone as an object.
let iPhone = {
price: 1200,
brand: "Apple",
"13mm Jack": false
};
And now let's just say you want to access the value of the price
property.
Here is how you do it with the help of the Object Dot notation:
iPhone.price;
This is your way of saying: "Hey Javascript, from the iPhone object, get me the value of the price
property."
Anyway, I want you to try this out by opening up the Console:
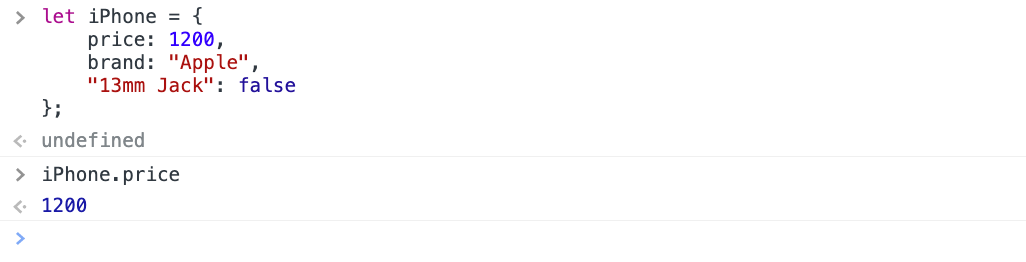
Inside the Console, you can enter multiple lines of code without executing them by hitting the shift
+ enter
on the keyboard.
This will tell the console that "Hey, I want to enter the next line of code. Don't execute the line of code written so far."
That's it. As simple as that.
Bracket Notation
We can also access the value of a particular property using the Bracket Notation.
Bracket Notation simply means the name of the property will go inside brackets:
iPhone['price'];
And when using the bracket notation, you have to surround the property name with the quotes.
The bracket notation is especially helpful if you use an invalid variable name (invalid identifier) as the name of a key.
For example, try out the following code in the Console:
let iPhone = {
"13mm Jack" : false
};
//And this is how you can access a property name with a space in it.
iPhone["13mm Jack"];
How to set the value of a new property
Sometimes, you might want to add more information to the object once it is created.
// The object is already created
let iPhone = {
price: 1200,
brand: "Apple",
"13mm Jack": false
};
So, here is how you can add a new property to an existing object and store a value in it:
iPhone.stock = "Sold out";
The above line of code will create a new property called stock
and stores the value of "Sold out"
in it.
The thing is, the dot notation will also help you add a new property to an existing object.
And you can store a value inside the newly created property by using the equal =
symbol.
You can also use bracket notation in the same way:
iPhone['stock'] = "Sold out";
How to update the value of a property
Now let's just say that the iPhone is back in stock again with 400 items.
And here is how you will update the stock
property again.
// The object is already created
let iPhone = {
price: 1200,
brand: "Apple",
"13mm Jack": false,
stock: "Sold out"
};
//Updating the stock property
iPhone.stock = 400;
We just have to access the property and assign a new value to it.
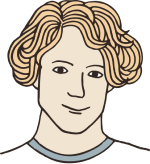
What! It is same as adding a new property, right? I am confused!
Sorry about that.
The technique is the same for both adding a new property and updating it
// The object is already created
let iPhone = {
price: 1200,
brand: "Apple",
"13mm Jack": false
};
//Updating the stock property
iPhone.stock = 400;
When Javascript first sees iPhone.stock
, it will first check whether the property named stock
is already defined inside the iPhone
object.
If it is already defined, then will update the value of the existing stock
property with the value 400
.
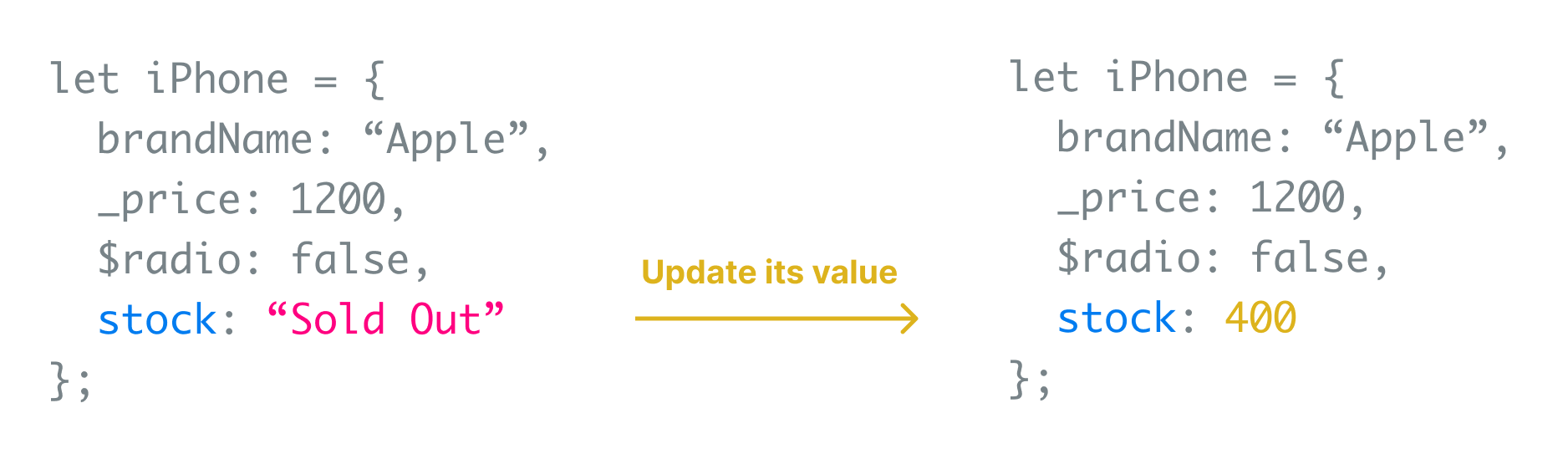
But if the property is not already defined, then it will create a new property and store the assigned value.
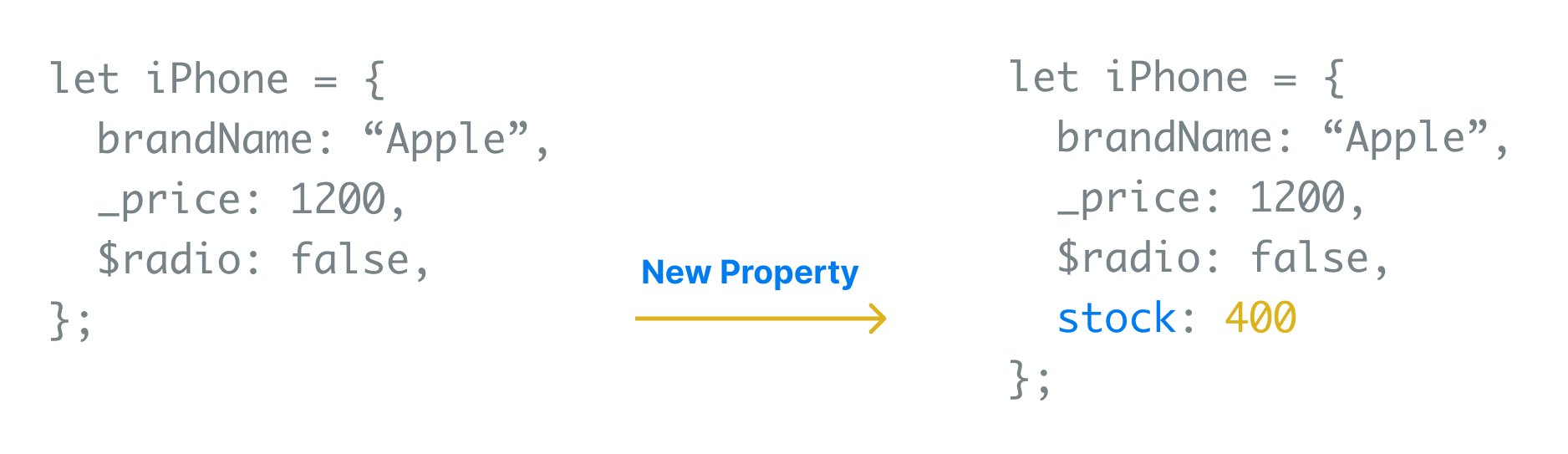
That's it.
I hope this clears up your confusion.
How to delete a property
You can delete a property by using the delete
keyword:
// The object is already created
let iPhone = {
price: 1200,
brand: "Apple",
"13mm Jack": false,
stock: 400
};
delete iPhone.stock;
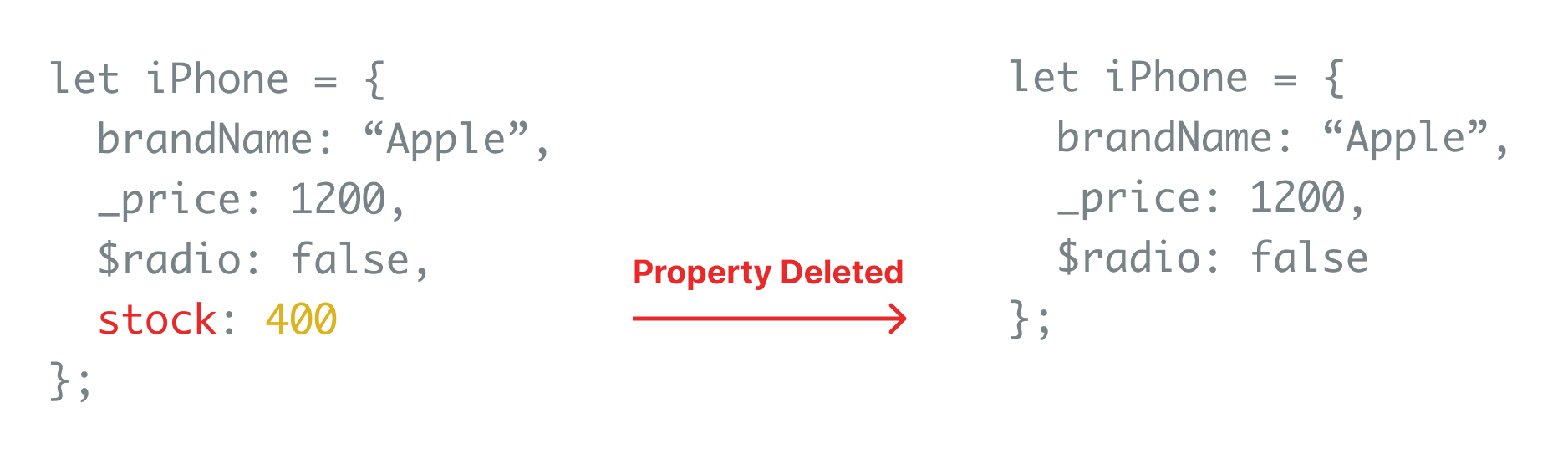
Once you delete a property, you can always add it back but the data of the previously deleted property will be lost forever.
In the next lesson, we will talk about the most important concept when it comes to objects.