How to create an array
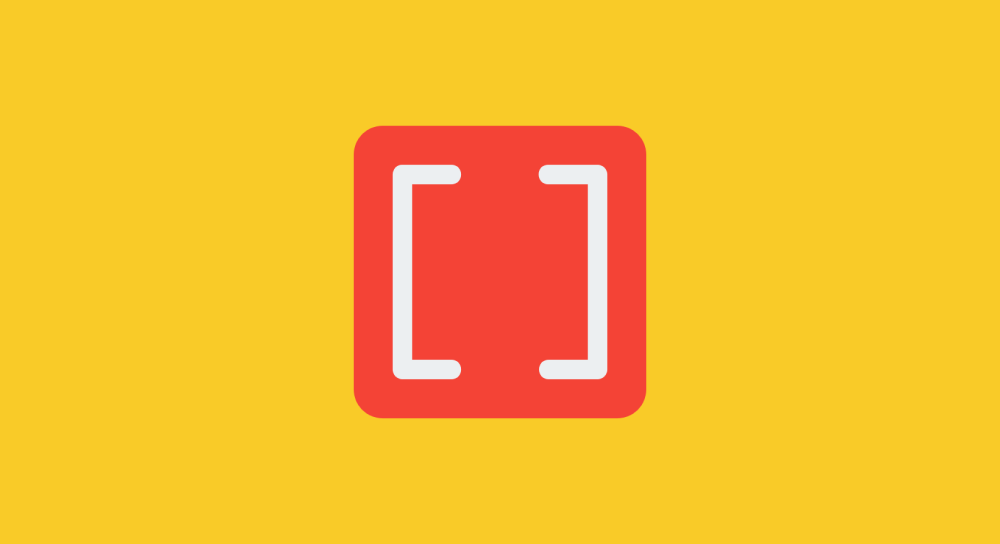
Just to recap:
- An array allows us to store and manage a list of data.
- An array is ordered. That means each item inside an array has an index that you can use to retrieve, delete, and change the order of the item.
To achieve the above tasks, we must first learn how to create an array.
So, How to create an array and store values inside it?
You can start creating an array by using squared brackets []
:
let iPhones = [];
The opening [
and closing ]
squared brackets will create an array.
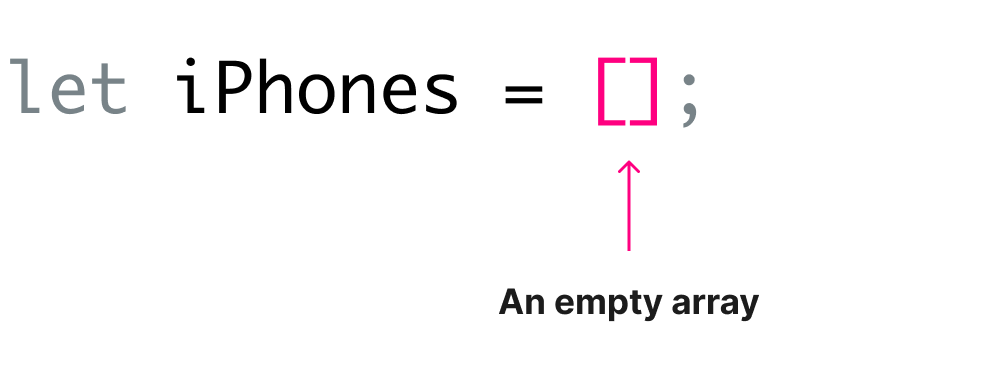
The data must go inside the squared brackets []
as individual items.
let iPhones = ["iPhone 13", "iPhone 12", "iPhone 11"];
And a comma must separate each item
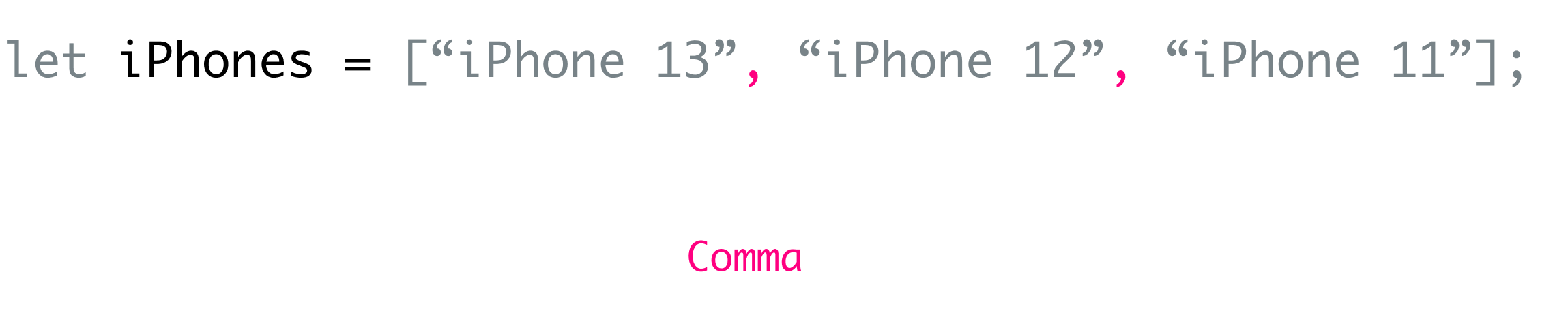
If you omit commas between each item, Javascript will throw an error.
You can store values of any data type inside an array
Yes, we can store items of any kind of data type (including arrays and objects).
To be more specific: String, Number, Boolean, null, undefined, object, array, symbol, bigInt.
All of them.
let randomArray = [
"Iron Man", // string
77, // number
true, // boolean
{ name: "Naresh", age:34 }, //object
["iPhone 13", "iPhone 12", "iPhone 11"] //array
];
Also, technically, the values that go inside an array don't need to be related at all.
This is why we are calling them individual items.
You will always put data that are related somehow, and you'll know these while working on real-world projects.
Now that you understand how to create an array, the next steps would be learning accessing items inside an array.
And we will learn about that in the next lesson.