How to create a custom function in javascript
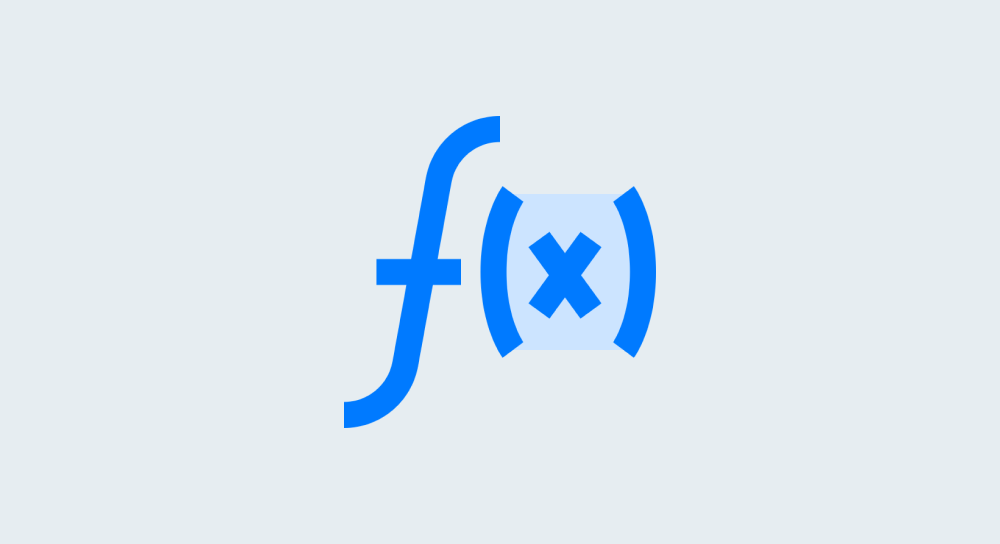
Come on, open up the Project: Discount Modal
folder from the last lesson in the code editor.
Currently, the index.js
file contains individual lines of code that reveal the modal:
//Step 1: Select the Discount Modal element
let discountModal = document.querySelector("#surprise-discount-modal");
//Step 2: Change the display property of the element to "block"
discountModal.style.display = "block";
Now transform the above code into a custom function with the name showModal
:
function showModal(){
//Step 1: Select the Discount Modal element
let discountModal = document.querySelector("#surprise-discount-modal");
//Step 2: Change the display property of the element to "block"
discountModal.style.display = "block";
}
In the above code, we defined a function called showModal
.
Defining a function simply means creating a new custom function.
We can also call this a function declaration or function definition.
The syntax for defining a function in Javascript
In order to define a new function, we need to use the keyword function
.
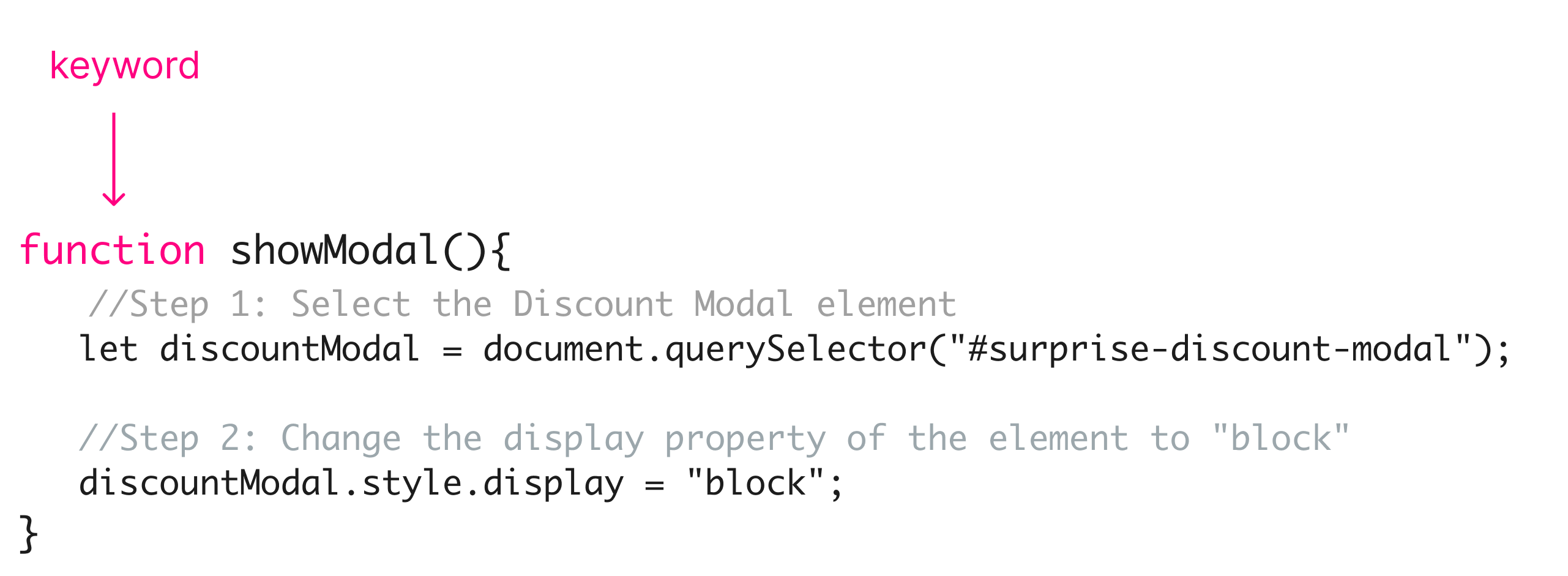
When Javascript sees the keyword function
, it understands that it is a function definition.
Then we need to provide a name for the function so that we can use it later to call it:
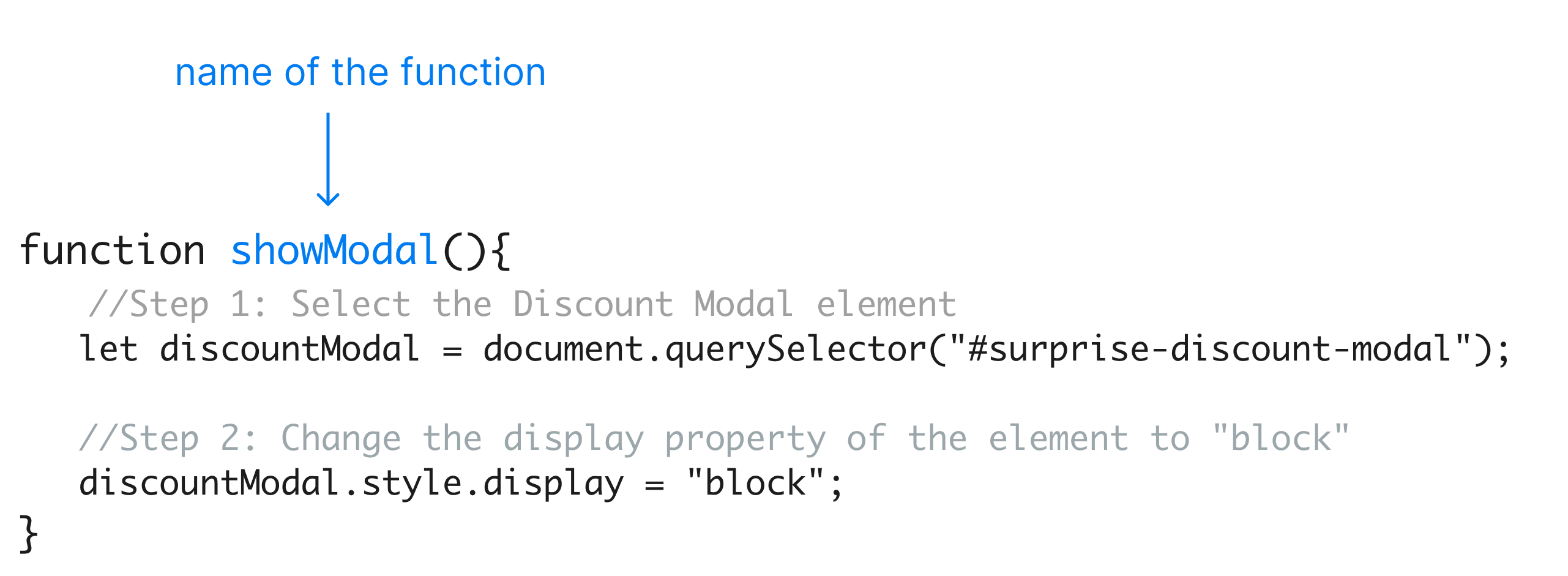
,
But it is totally optional. More on this in an upcoming lesson.
Either way, we must use ()
parenthesis while defining the function.
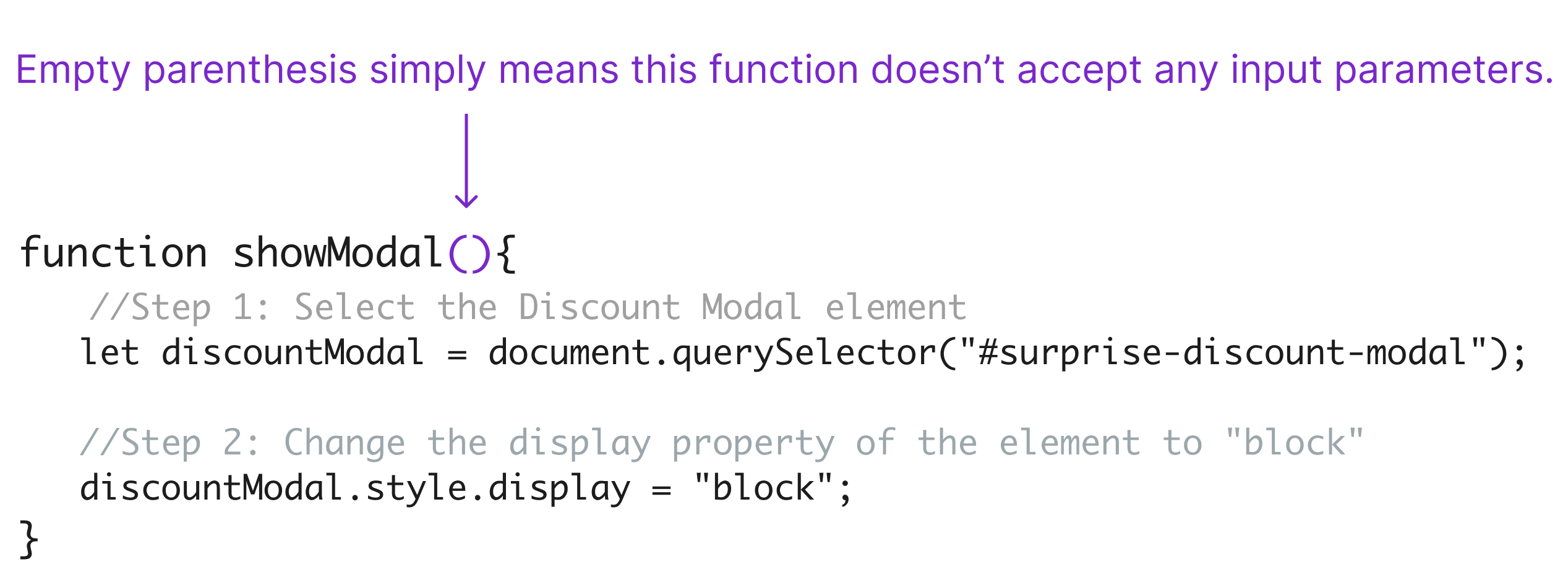
We are using empty parenthesis in the case of showModal
function because it doesn't accept any input parameters.
Finally, the code for implementing the task goes inside the curly braces {}
:
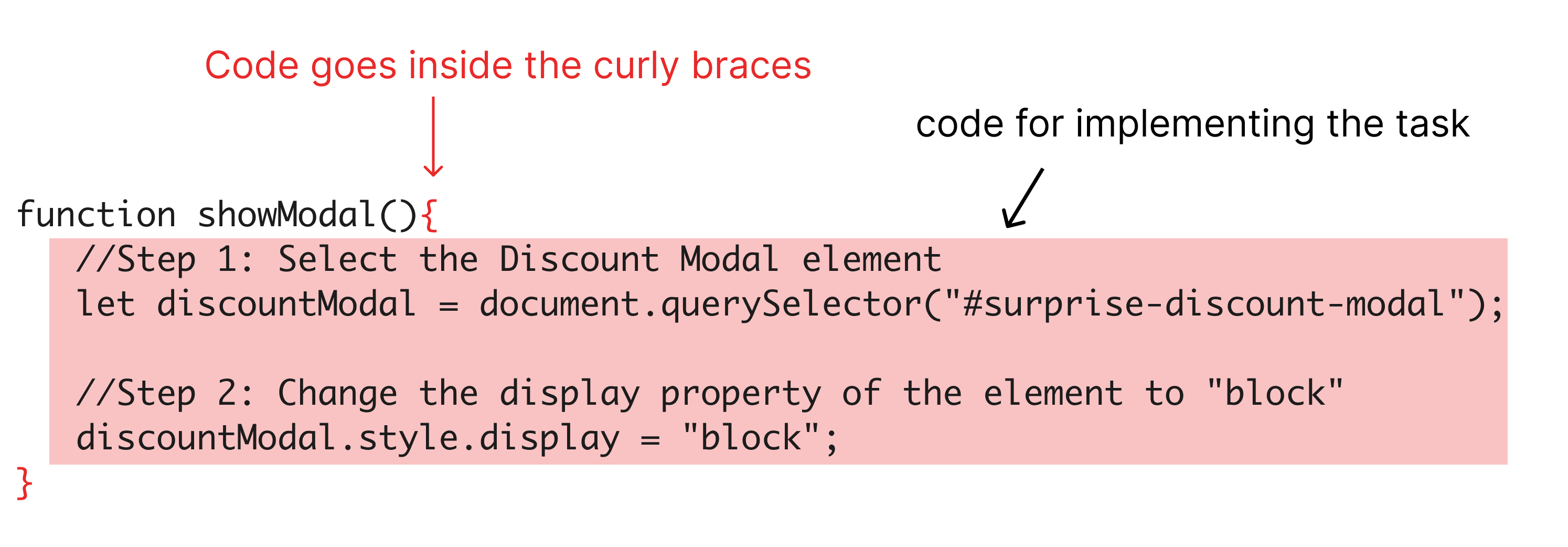
All this is great.
And now I have a small question for you.
If we now preview the index.html
file in the browser, do you think the modal would be visible?
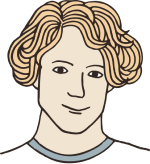
No because the browser will not execute the code inside the
showModal
function unless we instruct it.
Correct!
You are such a smarty.
Indeed, we can not see the modal:
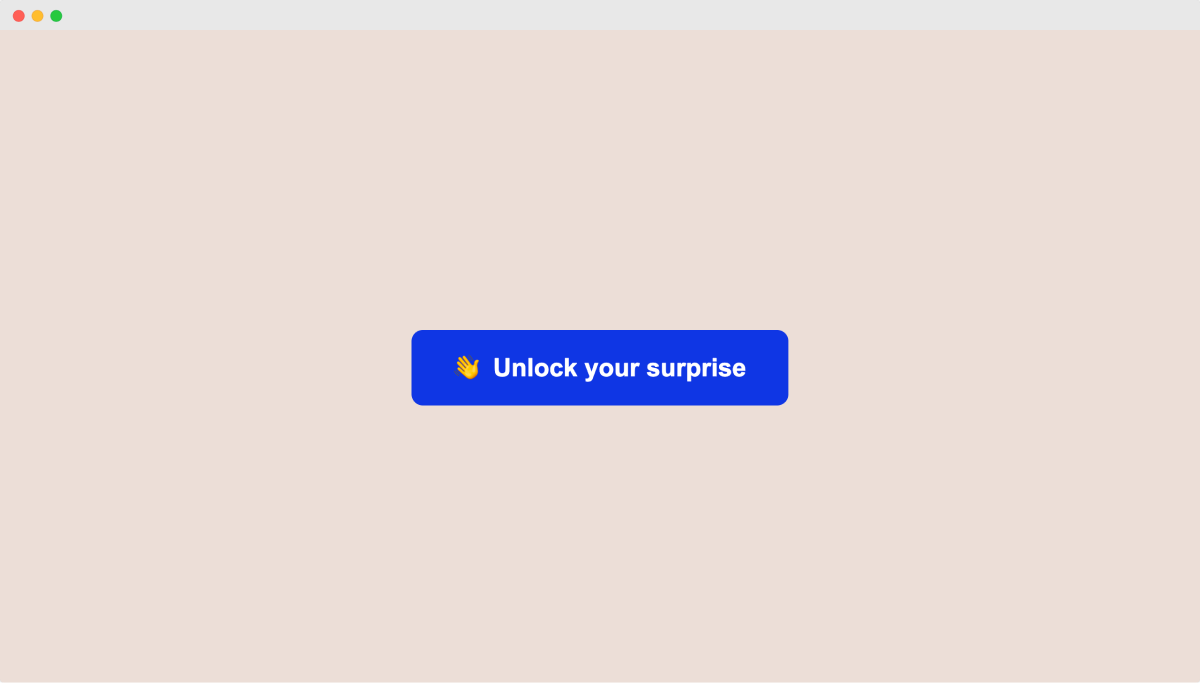
Basically, when the browser loads the index.js
, it sees the showModal
function definition but it does nothing it can not execute it without explicit instruction.
And now the only way to reveal the modal is, we have to explicitly call the showModal
function like this:
showModal();
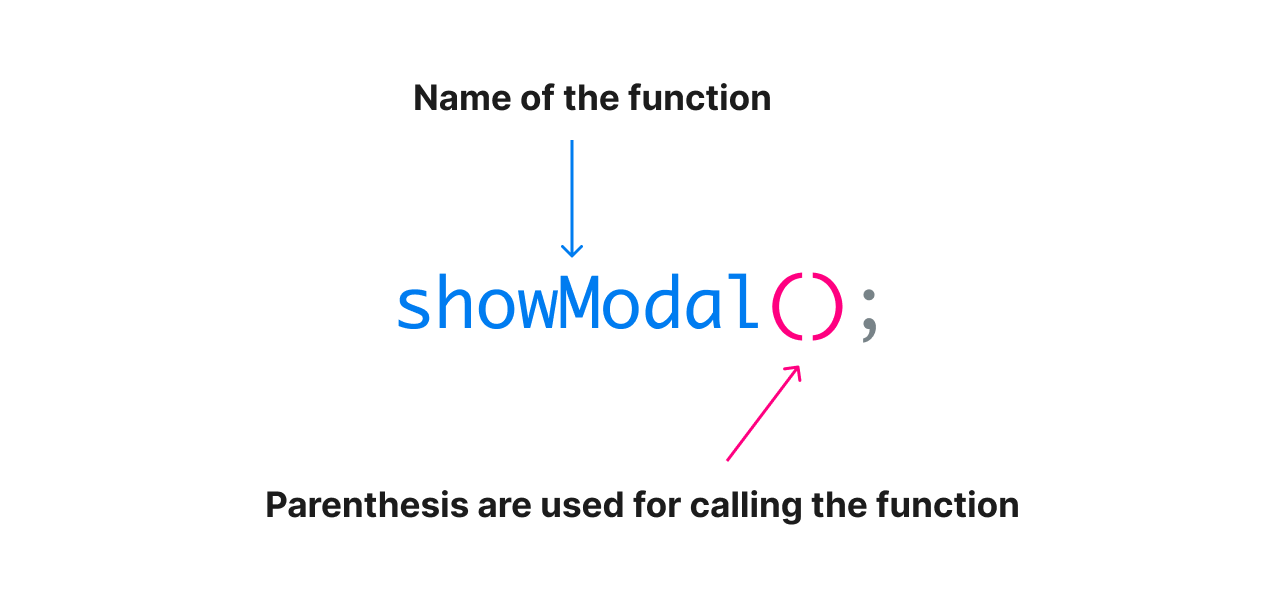
This is called a function call.
When we call the function, the code inside the function will get executed and this results in revealing the modal.
()
is the signal for the browser to call a function
and execute the code inside it line by line.Here is the updated execution flow of the index.js file
1) When the browser loads the index.js
, it sees the showModal
function definition but it does nothing by skipping to the next line of code.
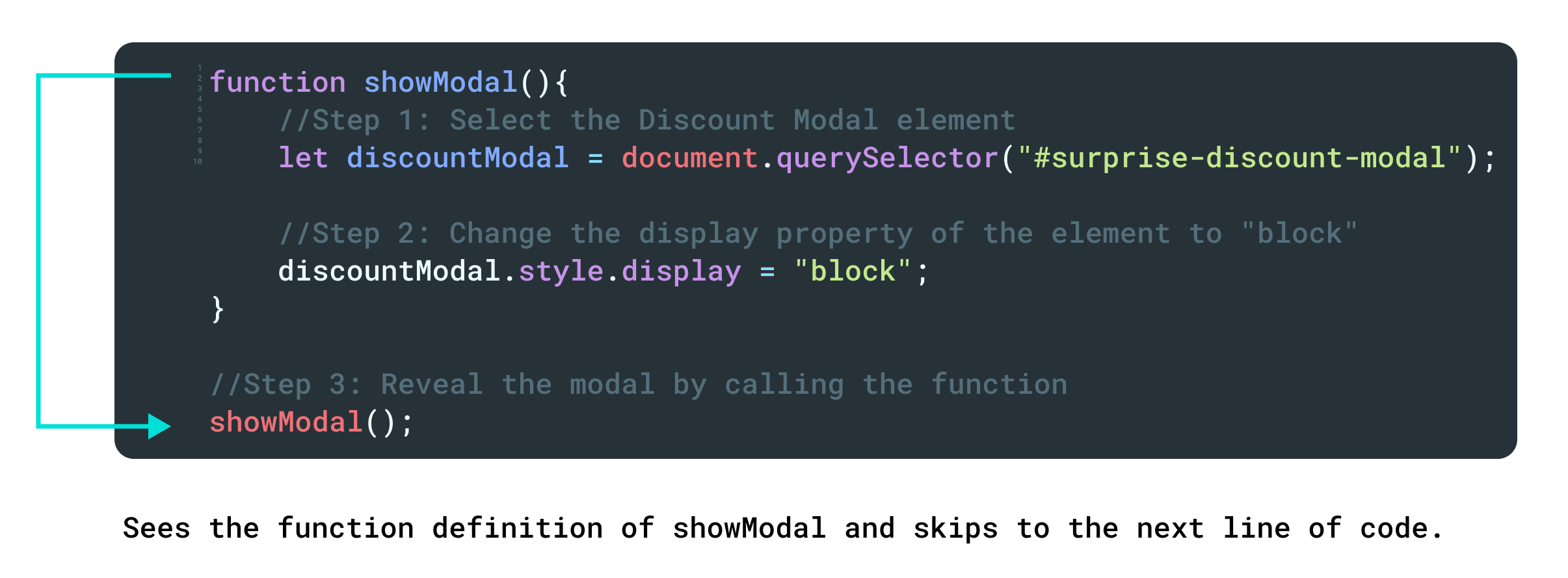
But at the same time, the browser remembers that there is a function definition called showModal
and it might find the call for it.
Then it when comes across the showModal()
function call, and it thinks that:
"Oh! I remember this function definition called showModal
, now I will execute the code inside it line by line!"
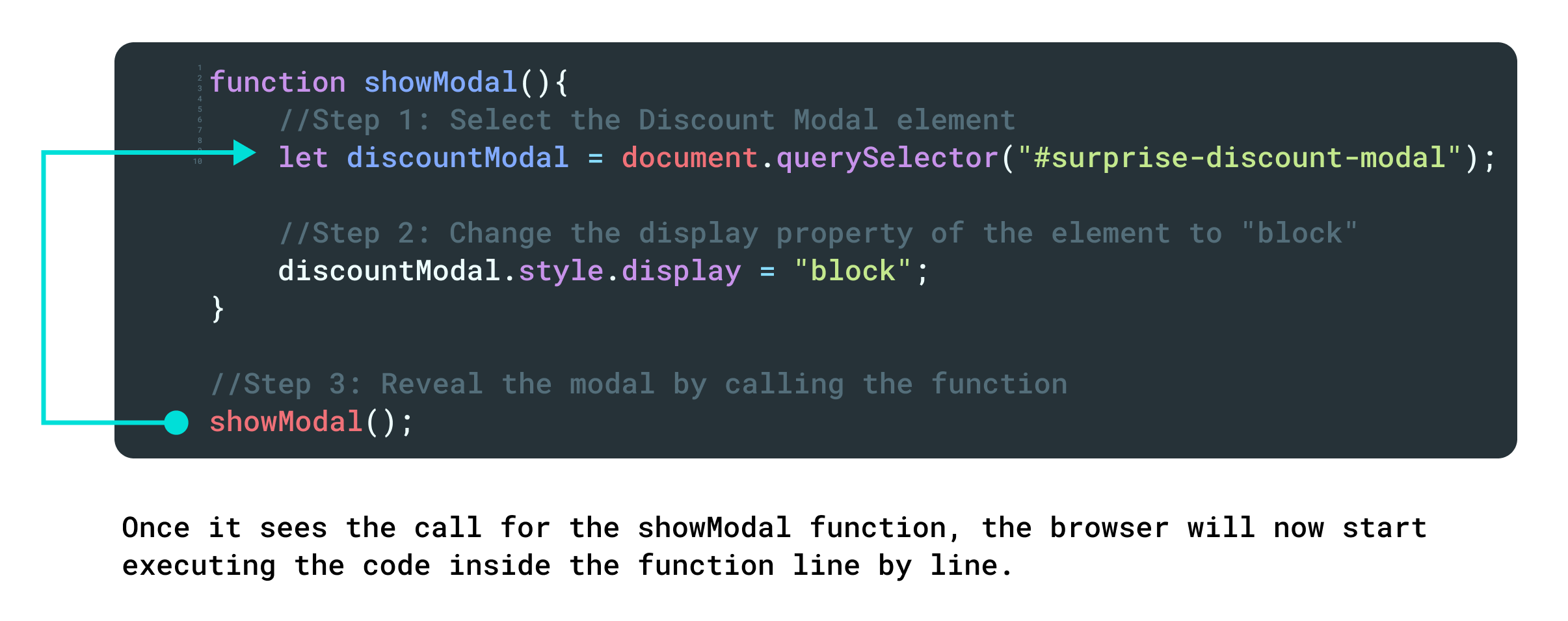
And finally, when the browser executes the last line of code inside the showModal function, the modal is revealed to the user.
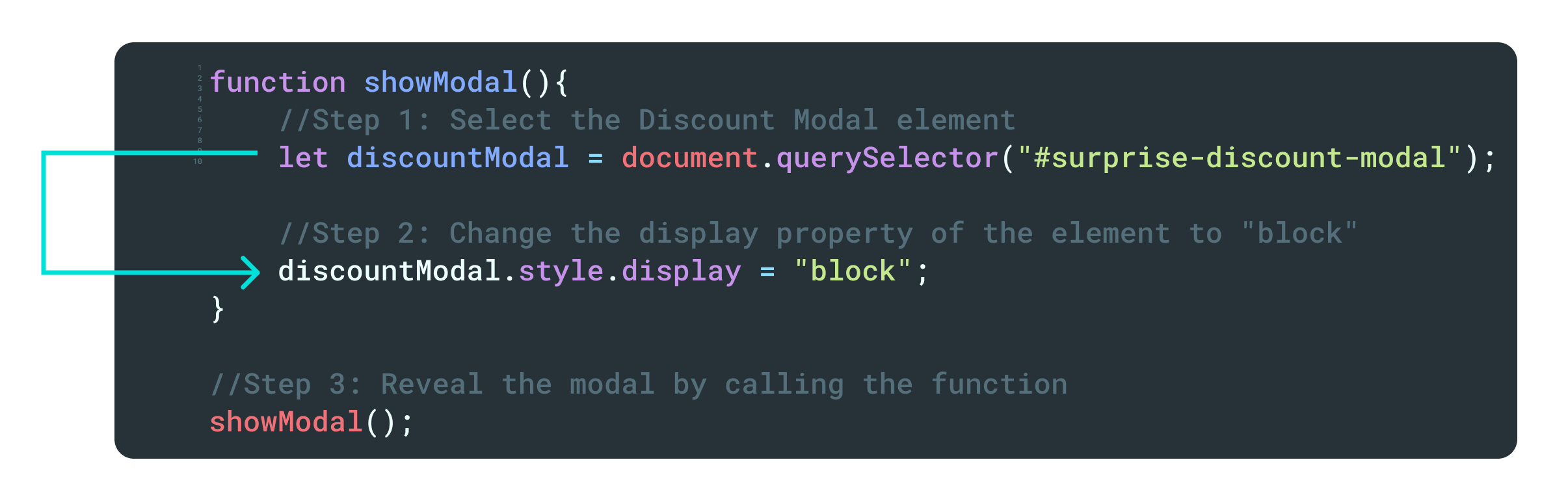
Anyway, put this showModal()
function call at the end of the index.js
file.
Here is the updated code for the index.js
file:
function showModal(){
//Step 1: Select the Discount Modal element
let discountModal = document.querySelector("#surprise-discount-modal");
//Step 2: Change the display property of the element to "block"
discountModal.style.display = "block";
}
//Step 3: Reveal the modal by calling the function
showModal();
If we now preview the index.html
file again, this time the modal is indeed getting revealed:
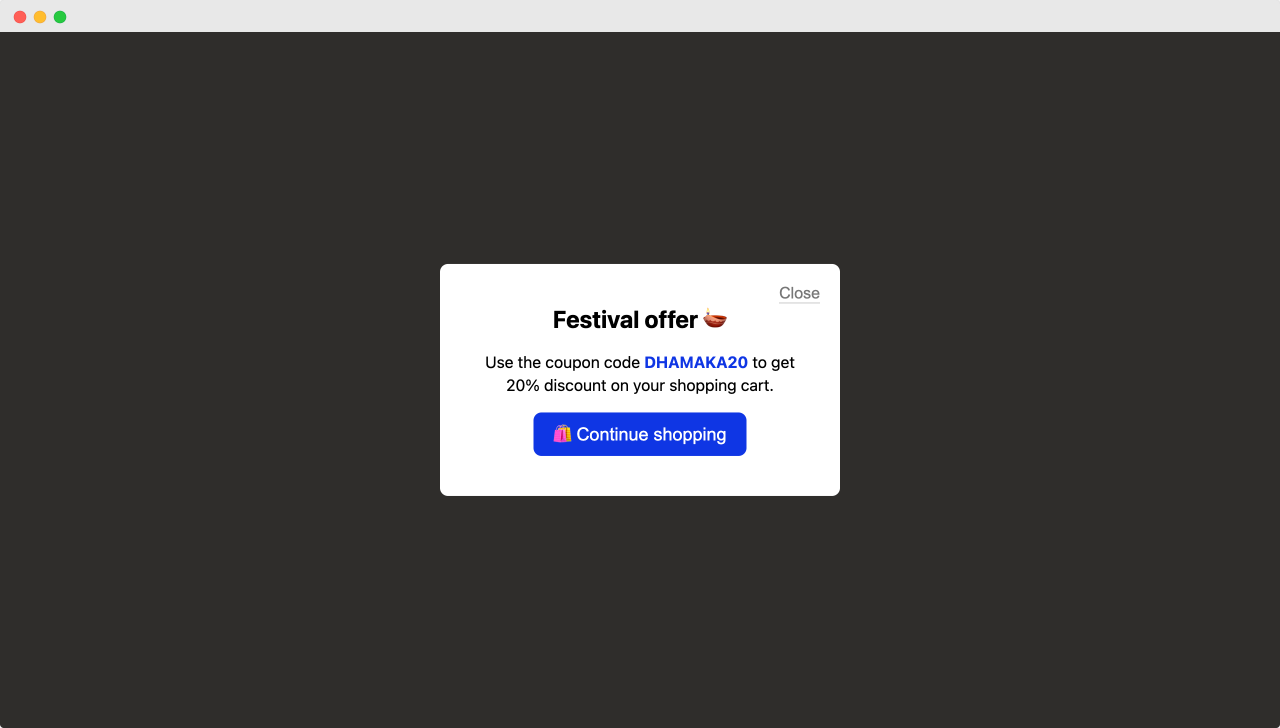
You can call a function any number of times but it is not practical
Also, remember, you can call a function
any number of times.
So, although it is not practical, the following code is totally valid:
showModal();
showModal();
showModal();
showModal();
showModal();
And it is not practical because, usually, we display a modal only when the button is clicked.
In other words, we need to call the showModal
function only when the button is clicked.
But how do we do that?
This is where events and event listeners come in Javascript, and we will learn about them in the next lesson.
But for now, because directly calling the showModal
function is not practical, remove its function call showModal()
from the index.js
file.
This will make the modal hidden again.
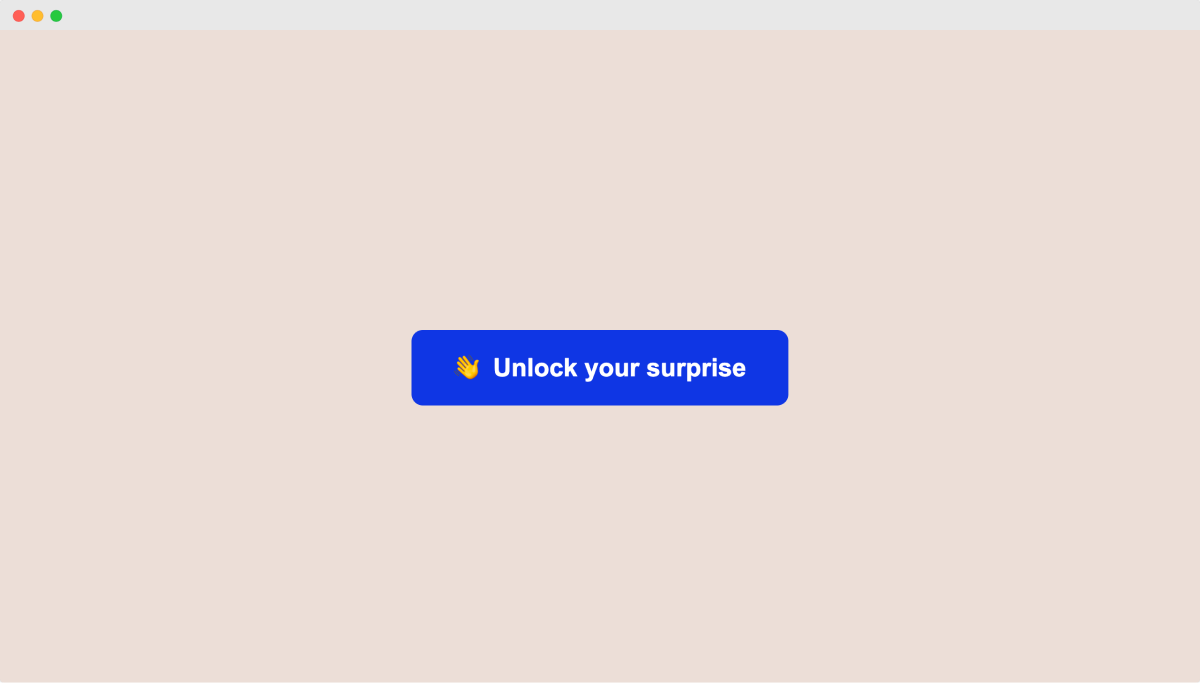
And here is the final index.js
file for this lesson:
function showModal(){
//Step 1: Select the Discount Modal element
let discountModal = document.querySelector("#surprise-discount-modal");
//Step 2: Change the display property of the element to "block"
discountModal.style.display = "block";
}