Getting to know variables a bit more
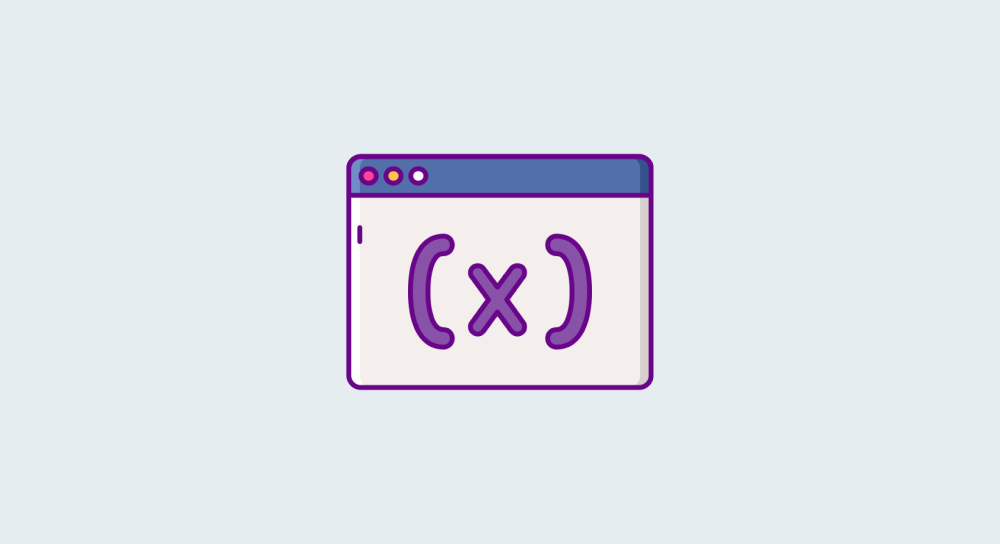
Before we move on to our next simple exercise, I want to provide a few more important details about variables.
Shortcut for creating variables
In the last lesson, we learned that there is a two-step process for using a variable:
//Step 1: Declare a variable
let age;
//Step 2: Use the name of the variable to assign a value to it
age = 34;
But there is a shortcut to this.
We can also declare a variable and assign a value to it in the same line of code.
let age = 34;
At the end of the day, it all boils down to personal preference and coding style.
How to access the value we stored inside a variable
Simple, we have to use the name of the variable to access its value.
In the world of programming, accessing the value of a variable is also called referencing a value.
Come on, let's see this in action.

Open up the Console and type the following code in it:
( Do not copy/paste but type it )
let graduation = "Masters in Computer Science";
We have now stored a value inside the graduation
variable and here is how you can access it:
graduation;
However, the above way of accessing the data is not practical because we are not doing anything with it.
We will see proper scenarios for accessing the variables soon.
Declaring and using multiple variables
A javascript program can have multiple variables.
You can declare as many variables as you want.
let name = "Naresh Devineni";
let age = 34;
let graduation = "B.Tech";
Pay attention to the data type you are storing inside the variables
We can store a lot of data types in types but currently let's take a look at a couple of them rather quickly:
let name = "Naresh Devineni"; // Text data
let age = 34; // Numeric data
let graduation = "B.Tech"; // Text data
Quotes or no quotes
When we are storing text data that includes letters, we surround it with quotes like this:
let message = "Howdy! Good Morning!";
let rollNumber = "06951A1222";
In programming, the terminology for the text data is called String.
You have to surround the string data with quotes even if there is a single letter inside the data. If not Javascript will throw an error.
But if you storing a numeric data, then you shouldn't use quotes:
let age = 34;
let transactionReferenceNumber = 893489048293482903;
We will learn more about the data types in multiple future lessons.
Updating variables
In order to update the variable, we just have to reassign a new value to it.
//Creating variables and assigning values to them.
let name = "Naresh Devineni";
let age = 34;
let graduation = "B.Tech";
//The person finished Ph.D graduation after B.Tech. So we have to update the information related to the person's graduation.
// Updating the variable
graduation = "Ph.D in Computer Science";
let
keyword when updating the variable. You should use the let
keyword only when creating the variable and we can create a variable only once. After the variable is created, you just have to use the name of the variable to update it or delete it.
var and const
You can also use the keywords var
and const
to declare variables instead of let
.
var is replaced by let
var
is the old way of declaring variables and you can still use it:
var age = 34;
But when the let
keyword got introduced in ES6, it became the recommended approach for declaring variables instead of the var
keyword.
There are a lot of benefits to using let
instead of var
and we will learn them when you start learning advanced Javascript.
The only reason why I am introducing var
now is that if you dig up some older scripts, you will find a lot of var
usage and I don't want you to get confused.
const has a special place
The const
keyword is used for declaring variables whose value doesn't change.
For example, once we take an account in a particular bank, our bank account number doesn't change unless we delete our account.
So, we can use the const
keyword for declaring a variable that stores the bank account number:
const bankAccountNumber = 50600089278656;
There are many other benefits to const
but we are not ready to learn them yet.
Anyway, don't worry if you don't understand this yet, it will be more clear once you start working on the projects in future lessons.
In the next lesson, we will have a very simple exercise.