Functions return data to us
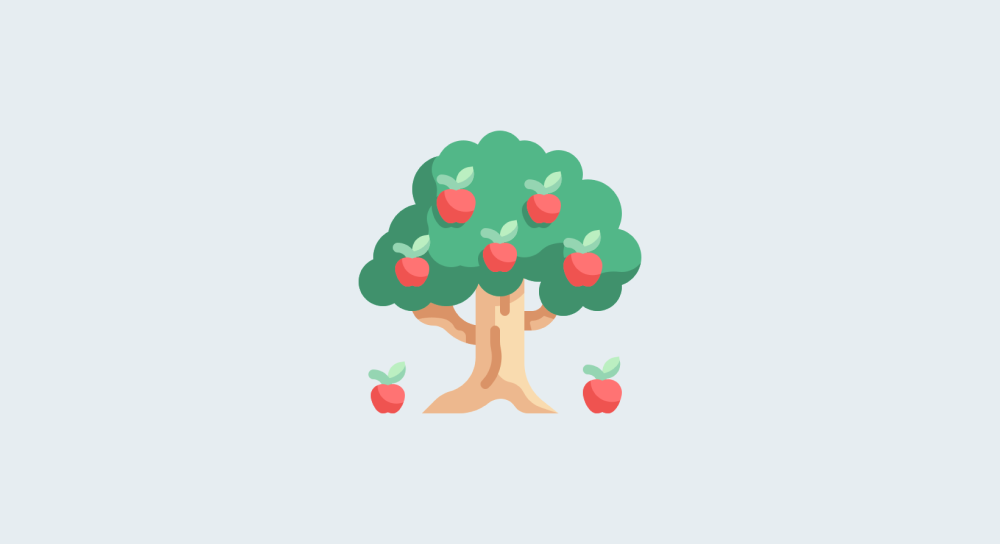
In the last lesson, we programmed the browser to collect the information from a visitor using the prompt
functionality:
// Collect the Country
prompt("Heya, Welcome! Which country do you live in?", "Canada");
// Collect the Name
prompt("Great! What is your name?");
// Collect the Age
prompt("Got it! One last question. What is your age?");
And imagine that the visitor has provided their details:
If you notice, the browser has collected all the details.
But how do we access those details?
We are collecting them for a purpose, right?
We want to do something with them, if not now then later.
This is where the return value from a function comes in.
A Function can return a value
The whole purpose of a coffee machine is to return us a cup of coffee.
Similarly, the most important characteristic of a function
is that it is capable of returning a value to us.
In other words, a function is capable of returning a value when it is executed.
This is better shown than explained.
Come on, open up the console in the browser and type the following code in it:
prompt("What is your age?");
And hit enter.
Did you see that? What did just happen?
The prompt
function is executed (triggered) with a message as input.
And, as soon as we executed the prompt
function, it opened up a dialog in the browser to collect details.
And, As soon as we have entered 34
for the age and hit okay, the prompt
function took that value and returned it to us.
This is called a return value from the function.
To be more clear, let's quickly compare the prompt
function to a coffee machine. And let's observe them with three easy steps:
Step 1: Input (parameters)
The coffee Machine takes coffee beans, water, and milk as input.
Similarly, the prompt
function takes a message as input a.k.a parameter.
prompt("What is your age?");
Step 2: Do something with the input taken
We push the button so that the coffee machine starts working. It crushes the beans, stirs the milk, and will mix them with the hot water.
And most importantly, by doing all that, it makes coffee out of that process.
Similarly, we execute the prompt
function.
And when we execute it, it starts working by opening up a dialog box in the browser in order to present a question to the user and take an answer from the user.
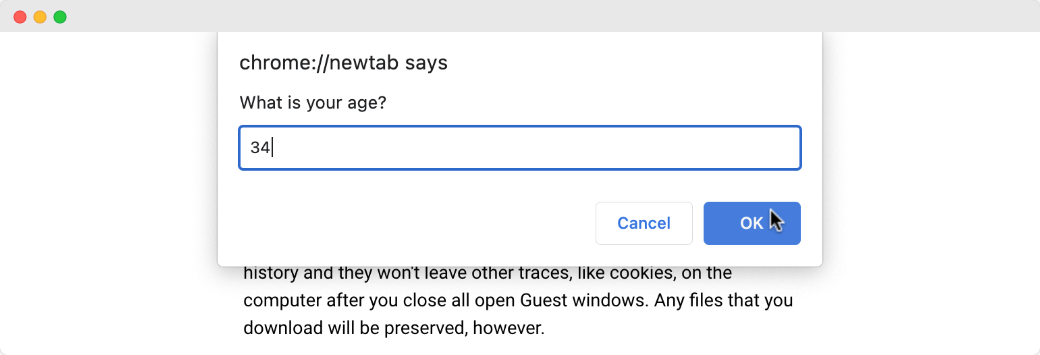
Step 3: Return the output
Finally, once the coffee machines makes coffee, it dispenses the coffee from the machine.
Similarly, once the prompt
finishes the task of collecting the details, it returns the answer (value) to us.
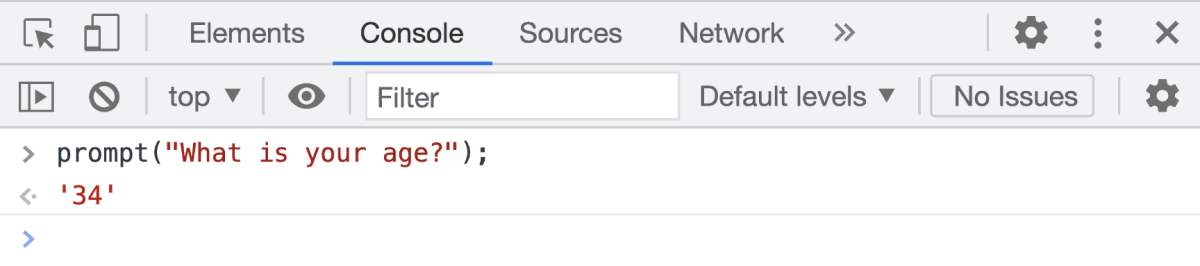
That's it. Nothing complicated.
A function doesn't have to return a value
The thing is, some functions do not return a value and it's okay.
It is like performing a task but that task doesn't produce any output.
Not all tasks we perform need to return a value.
It is not mandatory for a function to return a value.
For example, the alert
function doesn't return a value because it is not collecting any information from the user.
It is just used to send across a message to the user.
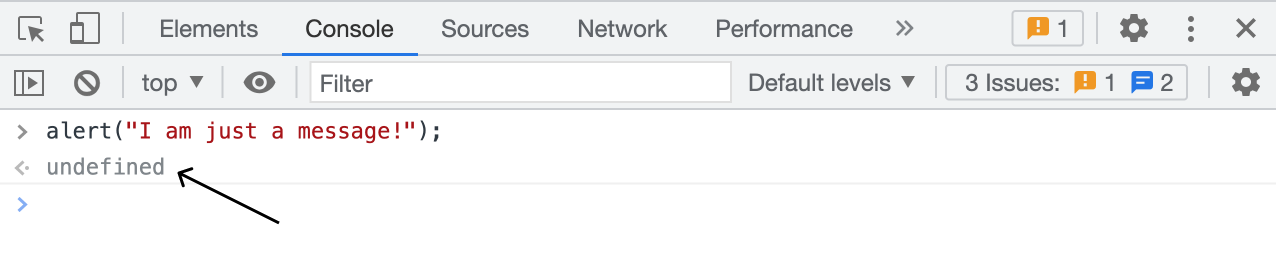
And this is why we see the value of undefined
when we try out alert
function in the Console.
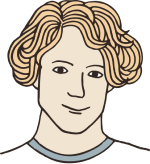
This is all good. But how do we know if a particular function returns a value of not?
Nice thinking!
MDN documentation to the rescue again
MDN documentation for Javascript will tell us whether a particular function returns a value or not.
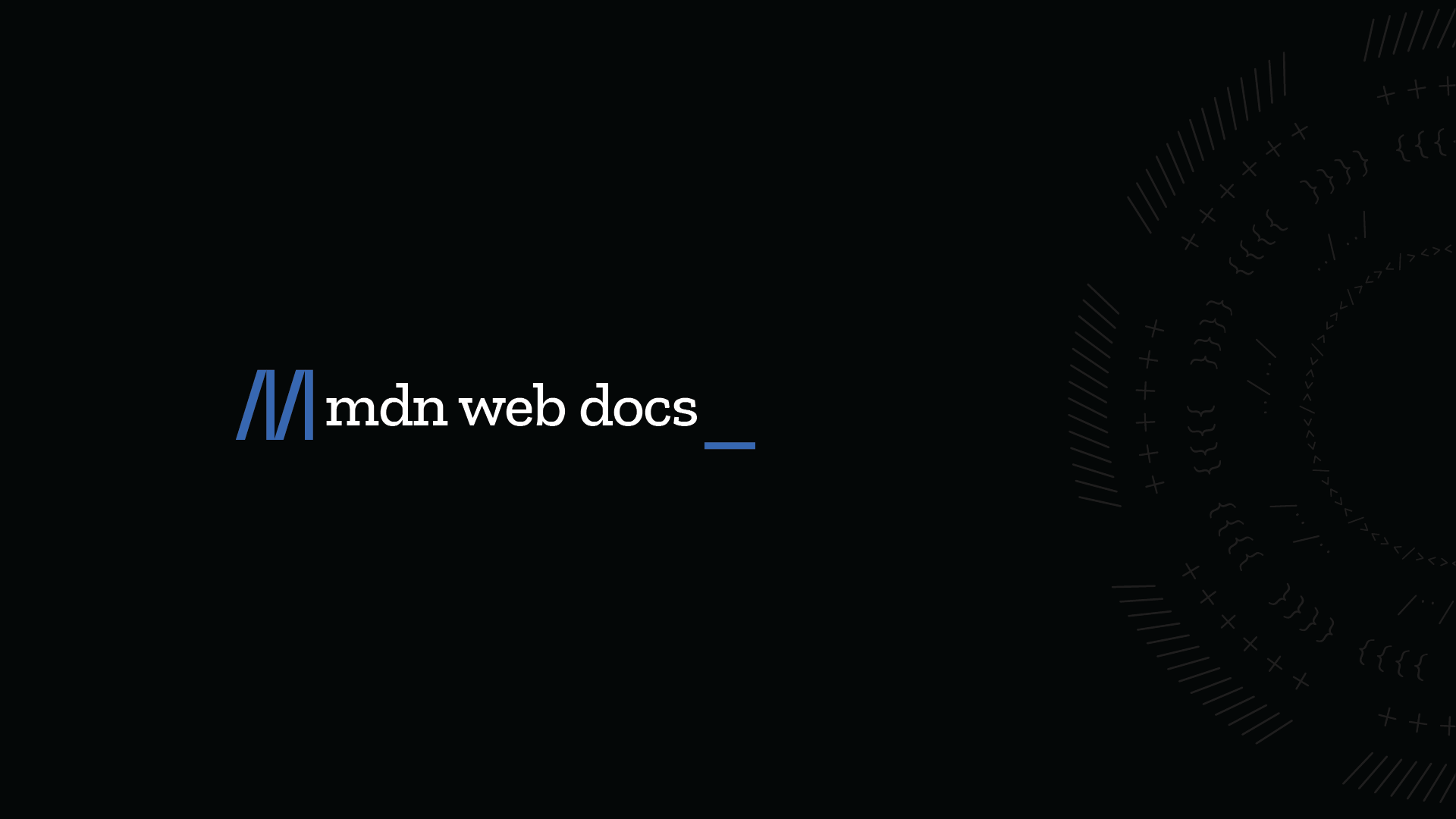
For example, the documentation for the prompt
function says it indeed returns a value and that value is the text entered by the user.
Similarly, the documentation for the alert
says that it doesn't return a value:
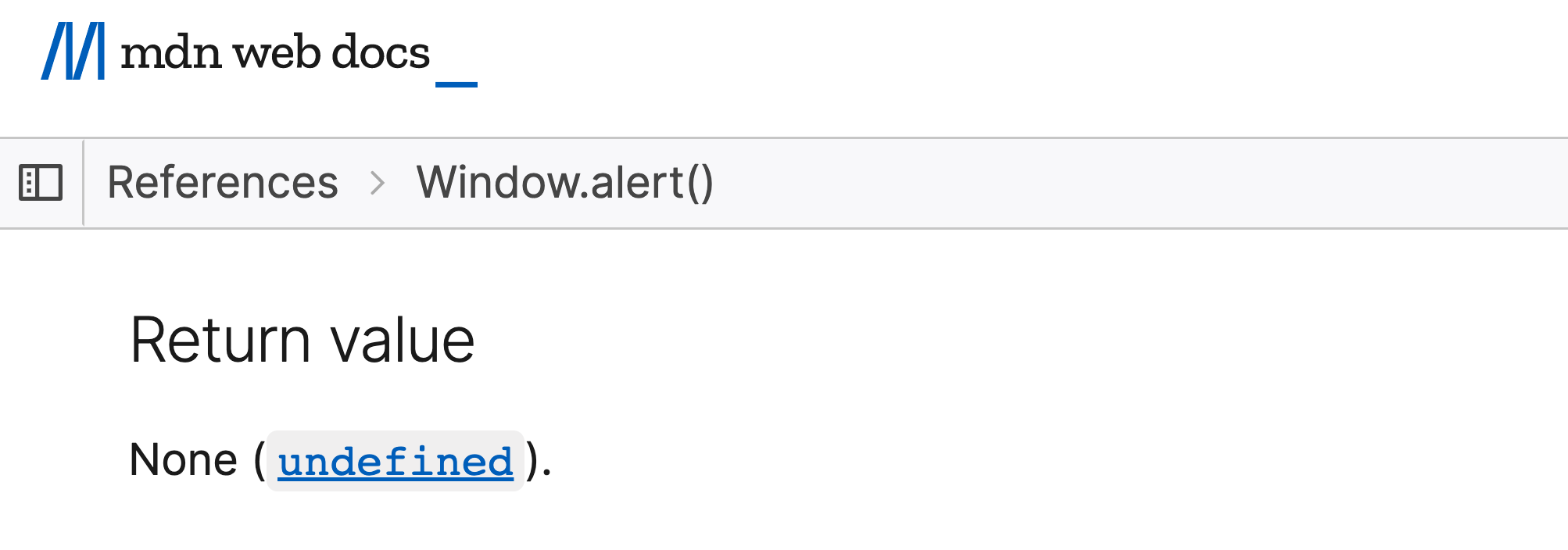
Nice, Kada?
How do we save the data returned?
We now know how to collect and access the data.
But what are we doing with it?
Nothing!
In the real world, if we are even collecting a phone number, we will save it on our phone so that we can call them later, right?
Our phone has a mechanism to save contact numbers.
Computer programs must be written in a similar way too.
In the case of this project, we have collected a Name, age, and Country.
We have to save them somehow for later use.
And this is where Javascript Variables come in and we will start learning them in the next lesson.