Expressions can contain other expressions
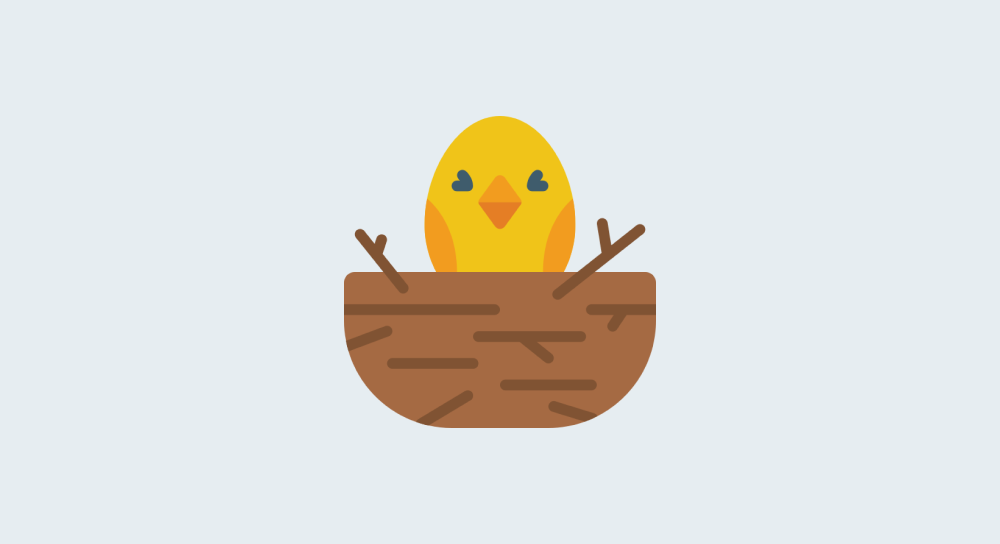
Expressions can be part of other expressions.
For example:
(2 + 3) * 5;
//Produces a value of 25
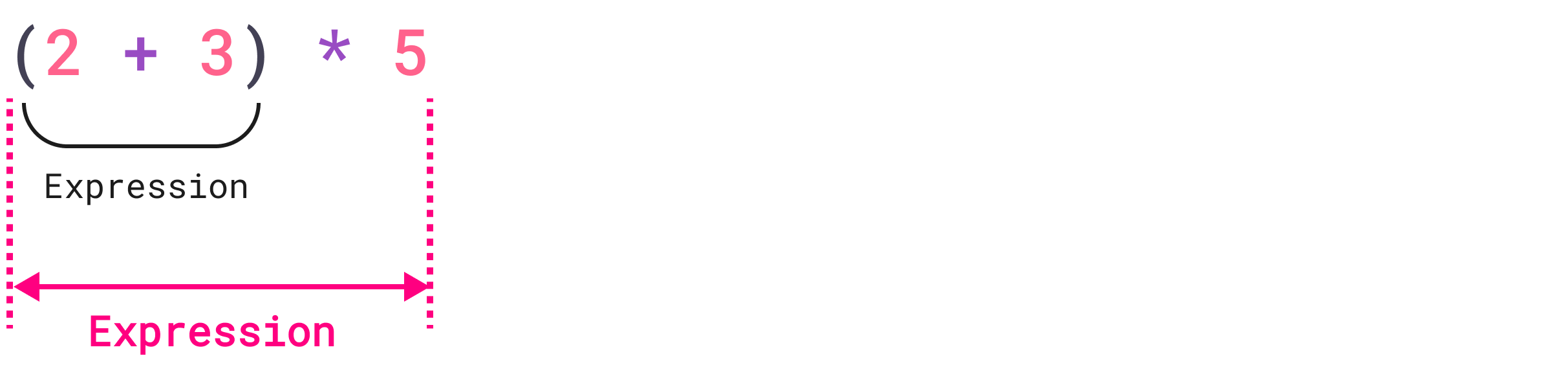
But how does the expression (2 + 3) * 5
produces the value of 25?
The answer is simple.
When Javascript tries to execute the expression (2 + 3) * 5
:
- It will first execute the nested expression
(2 + 3)
and produces a value of5
. - So internally, the expression
(2 + 3) * 5
now becomes5 * 5
. - And finally, when
5 * 5
is executed, it produces a value of25
.

And there is a reason why (2 + 3)
will get executed first in (2 + 3) * 5
. But we will talk about that in a future lesson.
Anyway...
Another quick example with a quick test of your knowledge
Let's just say we have a button with the text "Subscribe".
<button id="subscribe-btn">Subscribe</button>
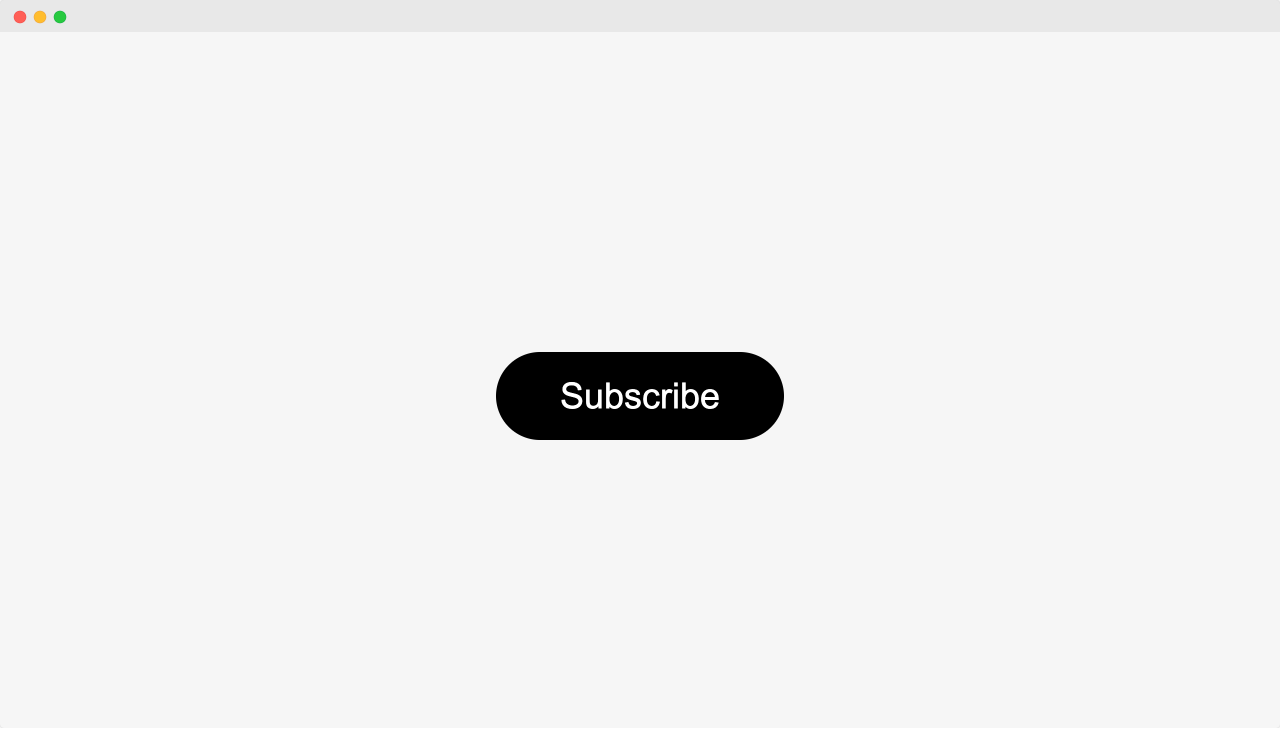
Then we wrote some javascript:
const subscribeBtn = document.querySelector("#subscribe-btn");
console.log( subscribeBtn.textContent === "Subscribe" );
In the above code, first, we are selecting the button and then we are logging the value of the following expression to the console:
subscribeBtn.textContent === "Subscribe"
The above expression is a boolean expression because we are using the ===
operator and it basically checks whether the button text is "Subscribe" or not.
Now tell me.
What is the message that gets logged to the browser console?
Is it true
or false
?
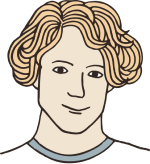
It logs
true
bro!
Nice! You got it correct.
For people who didn't understand, here is how:
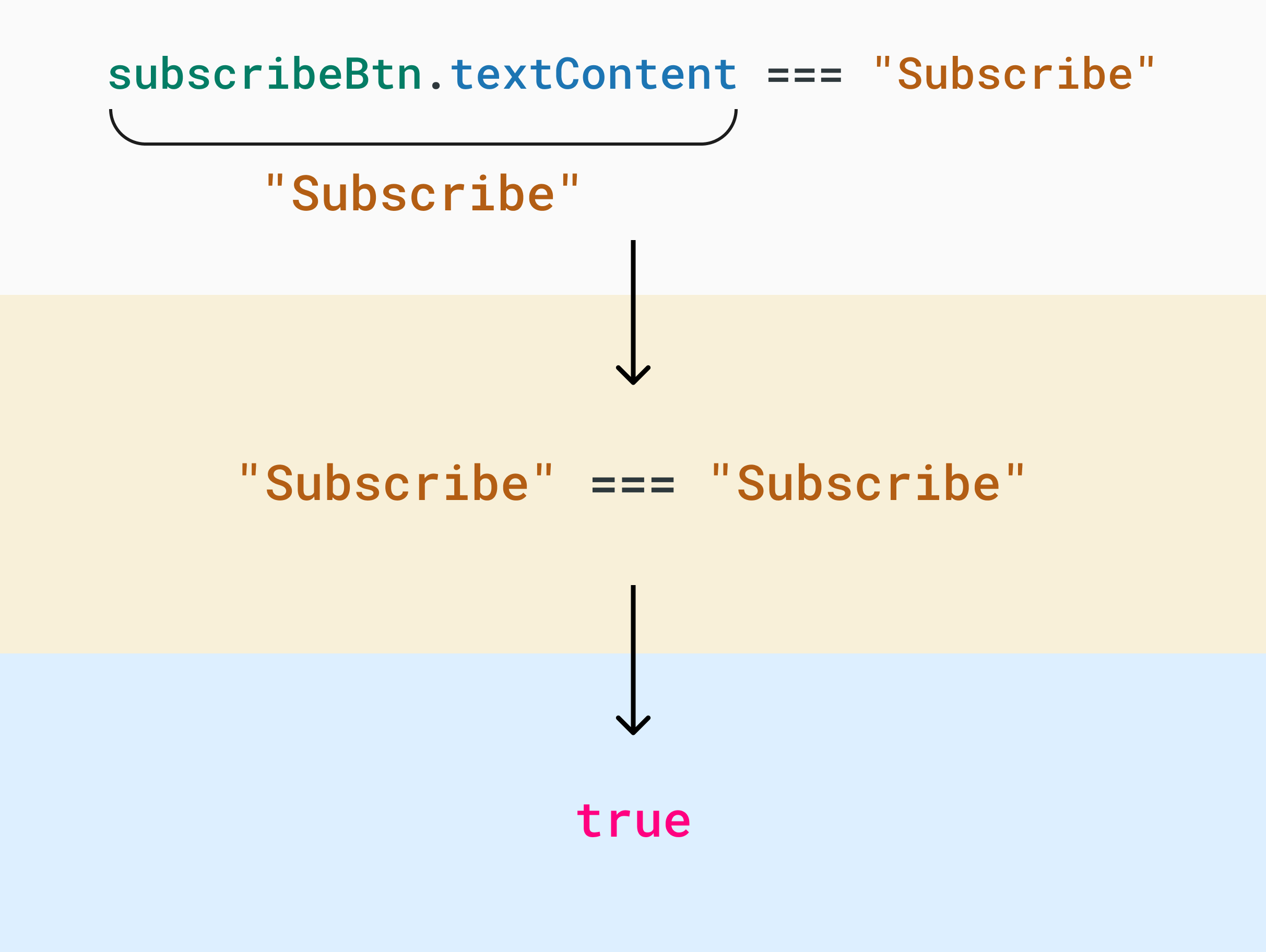
When javascript tries to execute the expression:
subscribeBtn.textContent === "Subscribe"
In notices the nested expression subscribeBtn.textContent
and evaluates it first.
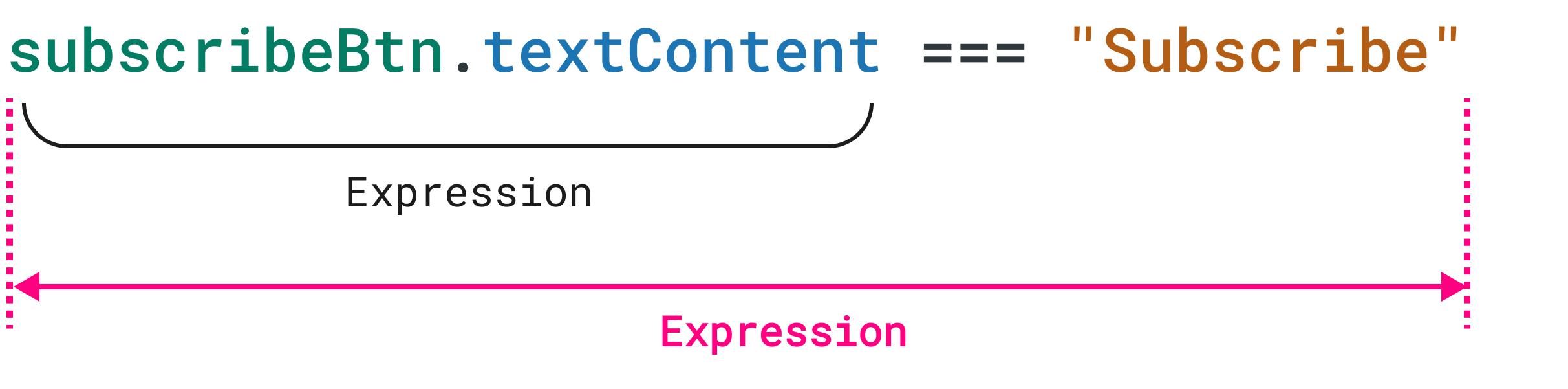
The textContent
property contains the button's text as the value.
In our case, the button text is "Subscribe".
So, when javascript evaluates the expression subscribeBtn.textContent
, it produces a value of "Subscribe"
.
So, internally, the expression now becomes:
"Subscribe" === "Subscribe"
Is the text "Subscribe" equal to "Subscribe"?
In other words, Is the text "Subscribe" same as "Subscribe"?
True, right?
So, the boolean value true
is produced.
That's all you need to know about expressions and nested expressions for now.
Having said that, expressions are never used alone.
They are not that useful when they are executed as is.
They must be part of something called a statement.
And we will learn about statements in the next lesson.