Exercise: Collect and save details
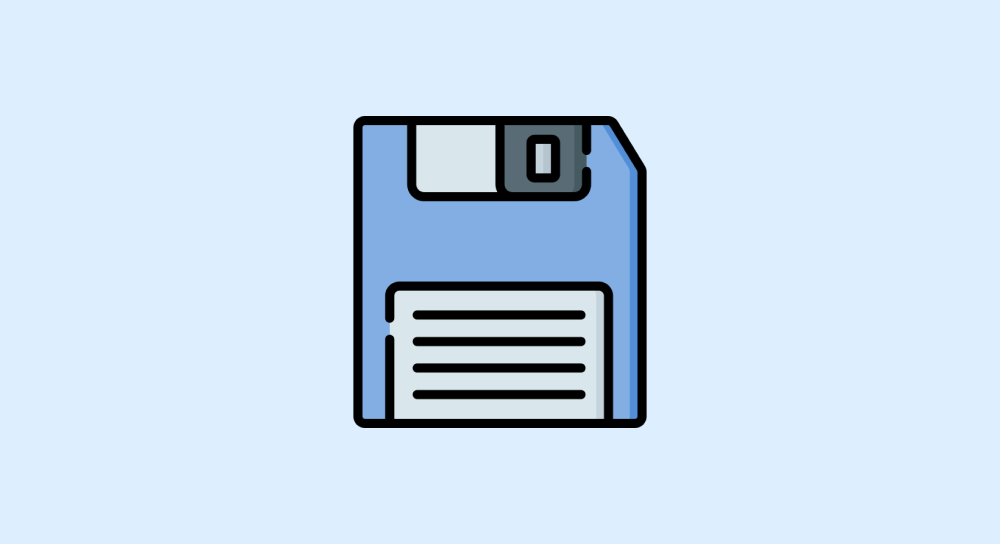
The Exercise
In our previous project "Collect Details", we have written Javascript code for collecting the details using the prompt
function:
// Collect the Country
prompt("Heya, Welcome! Which country do you live in?", "Canada");
// Collect the Name
prompt("Great! What is your name?");
// Collect the Age
prompt("Got it! One last question. What is your age?");
But the above code doesn't have the brains to save the details provided by the user.
So, you job is to give those brains to the above code using variables.
And here is your exercise:
- Declare a variable called
country
- Declare a variable called
name
- Declare a variable called
age
Then assign the respective prompt
function calls to those variables.
Solution
//Declare the variables
let country;
let name;
let age;
// Collect the Country and save it
country = prompt("Heya, Welcome! Which country do you live in?", "Canada");
// Collect the Name and save it
name = prompt("Great! What is your name?");
// Collect the Age and save it
age = prompt("Got it! One last question. What is your age?");
An alternative solution using the shortcut method for variable creation and assignment:
// Collect the Country and save it
let country = prompt("Heya, Welcome! Which country do you live in?", "Canada");
// Collect the Name and save it
let name = prompt("Great! What is your name?");
// Collect the Age and save it
let age = prompt("Got it! One last question. What is your age?");
I hope you got the exercise right.
If not, feel free to reach out to me :)