Exercise: Outputting all the items of an array into the web page
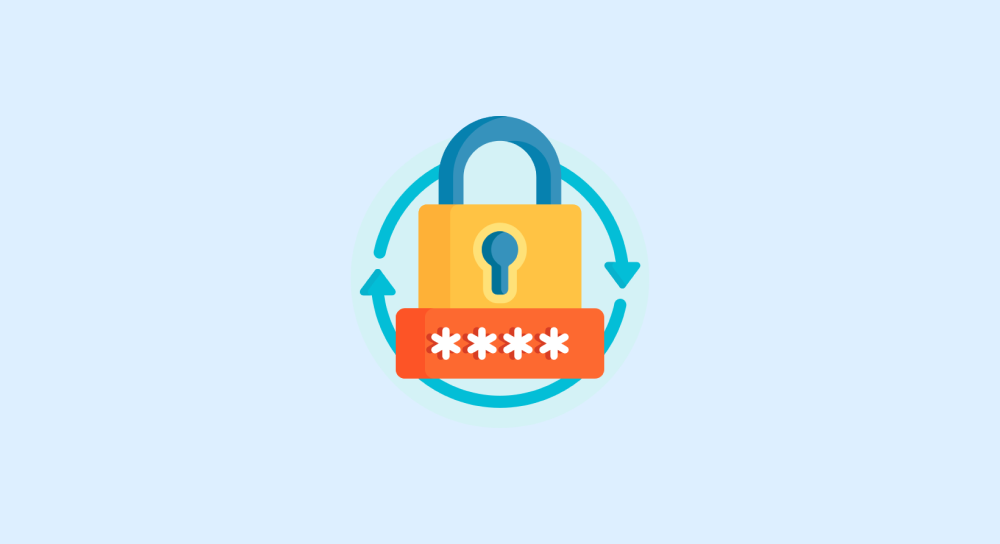
As part of this module, we have learned the following techniques so far:
- Create an array and access items inside it using the index value of each item.
- Create an HTML element and insert it into the web page
As part of this exercise, I want you to combine both the above techniques and insert a list of the most commonly used passwords into the web page as a warning:
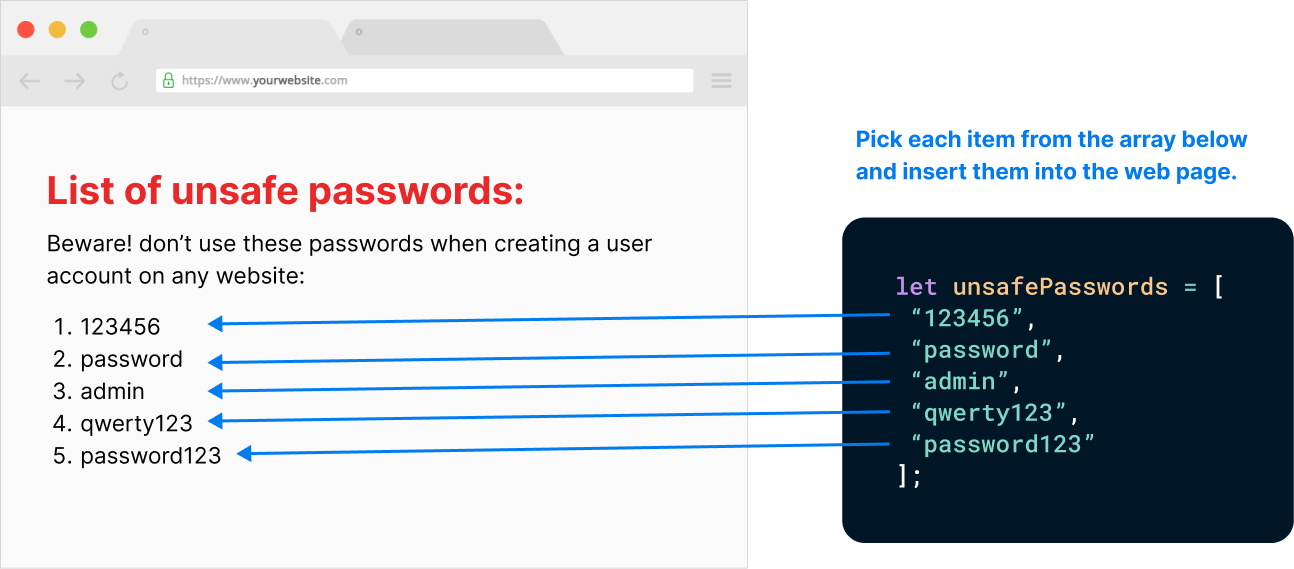
In other words, access the items of an array one by one and output them into the web page.
This is more than a common scenario.
If you read the previous lessons and understood them, this exercise must be easy for you.
Come on, download the starter files for this exercise and open them in your code editor:
<!DOCTYPE html>
<html>
<head>
<link rel="stylesheet" href="style.css">
</head>
<body>
<h1>List of unsafe passwords:</h1>
<p>Beware! don’t use these passwords when creating a user account on any website:</p>
<ol id="unsafe-passwords"></ol>
<script src="index.js"></script>
</body>
</html>
If you notice the markup inside the index.html
file, there is an empty ol
element.
Then, if you notice the code inside the index.js
file, there is an array with a list of unsafe passwords:
let unsafePasswords = [
"123456",
"password",
"admin",
"qwerty123",
"password123"
];
Your job for this exercise is simple:
- Select the
ol
element with theid="unsafe-passwords"
as the parent element - Create an
li
element. - Access the first item inside the
unsafePasswords
array and add it as a text content to theli
element you have created previously. - Append the
li
element you have created previously as a child element of the selectedol
element from step 1. - Finally, repeat the steps 2, 3, and 4 for the rest of the items of
unsafePasswords
array.
I gave you the answer sheet.
Come on, take it as a challenge and perform the exercise by yourself.
Look at the solution below only if you get stuck.
The Solution:
Outputting the first item from the array into the web page:
let unsafePasswords = [
"123456",
"password",
"admin",
"qwerty123",
"password123"
];
//Select the parent element
const olElement = document.querySelector("#unsafe-passwords");
// Outputting the first item in to the web page
const firstLiElement = document.createElement("li");
firstLiElement.textContent = unsafePasswords[0];
olElement.appendChild(firstLiElement);
First, I have selected the ol
with the id="unsafe-passwords"
as the parent element because I will push individual passwords from the array as li
elements inside it.
const olElement = document.querySelector("#unsafe-passwords");
Next, I want to output the first password from the array, which is "123456"
, into the web page.
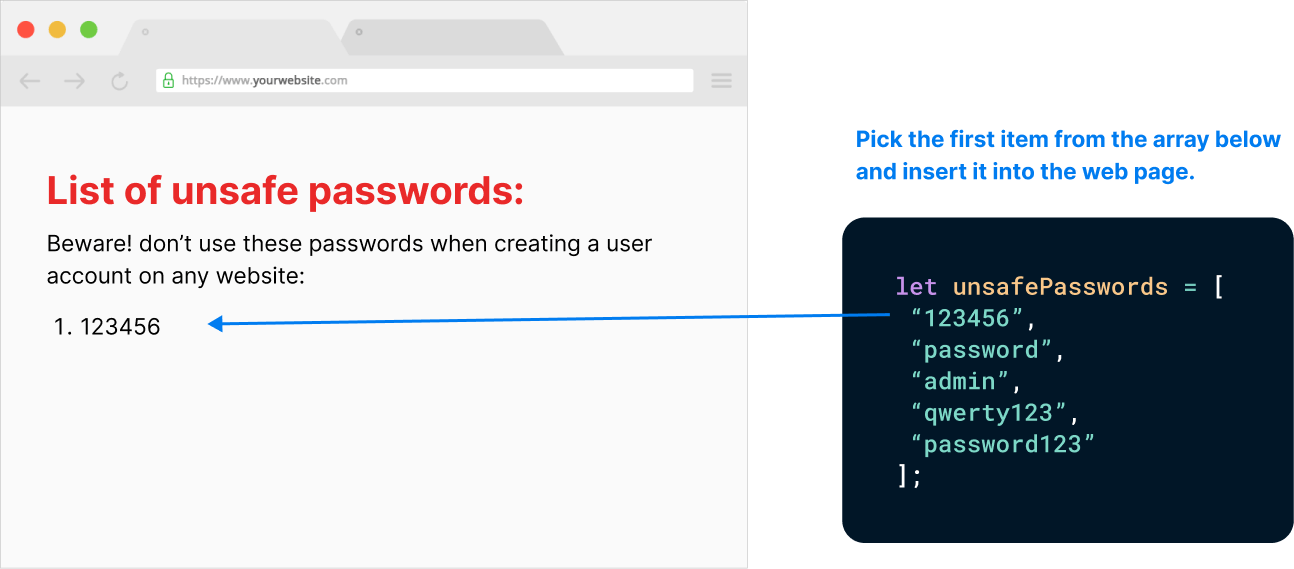
So, I have created an li
element for it:
const firstLiElement = document.createElement("li");
This will result in an empty li
element:
<li></li>
Next, for the content of this new li
element, I am assigning the value of the first item inside the unsafePasswords
array:
firstLiElement.textContent = unsafePasswords[0];
When Javascript executes the above line of code, it translates to:
firstLiElement.textContent = "123456";
And because of the above, here is how the updated li
element looks like:
<li>123456</li>
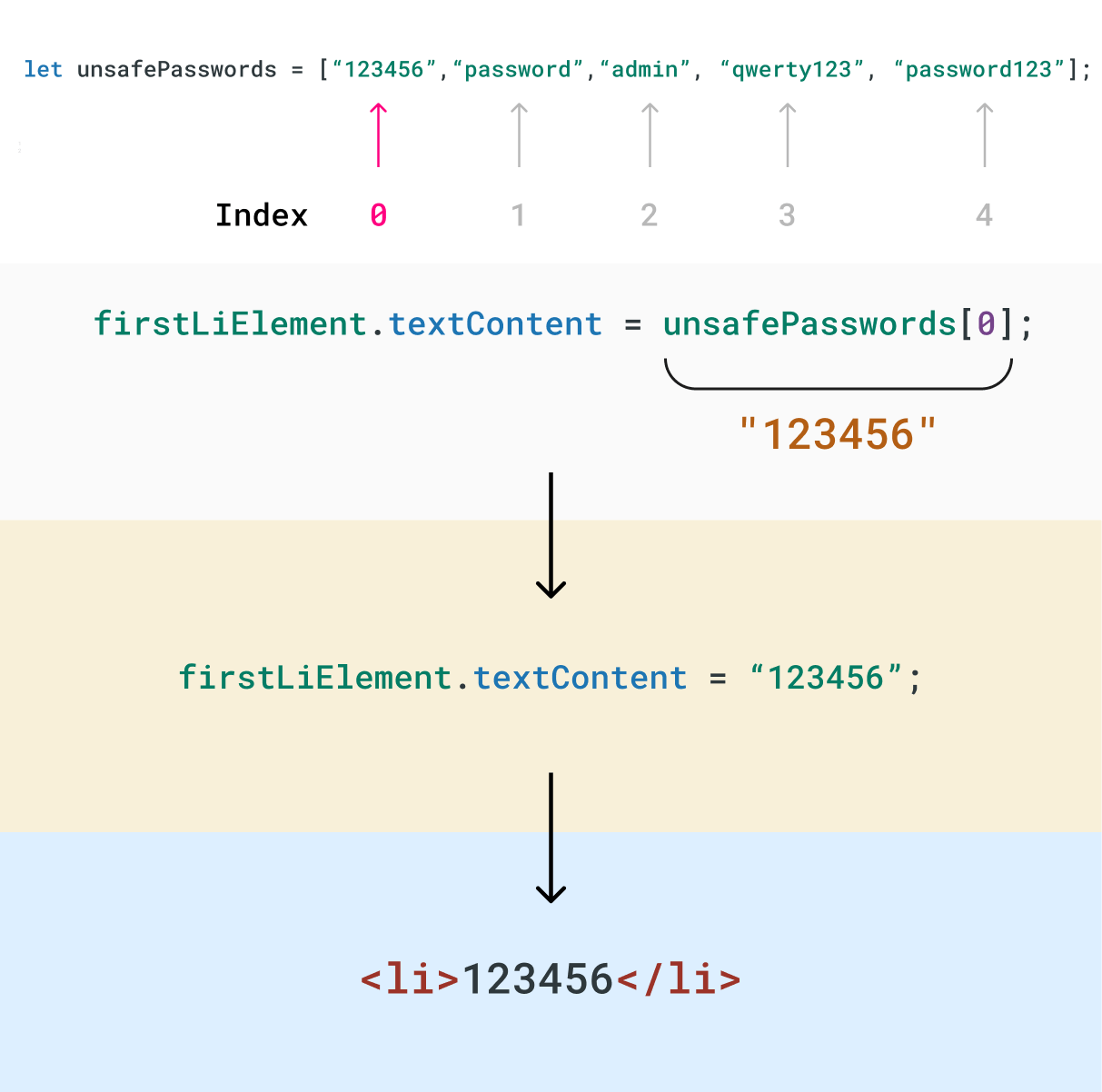
Finally, I have added this newly created li
element as a child element to the ol
element using the appendChild
method :
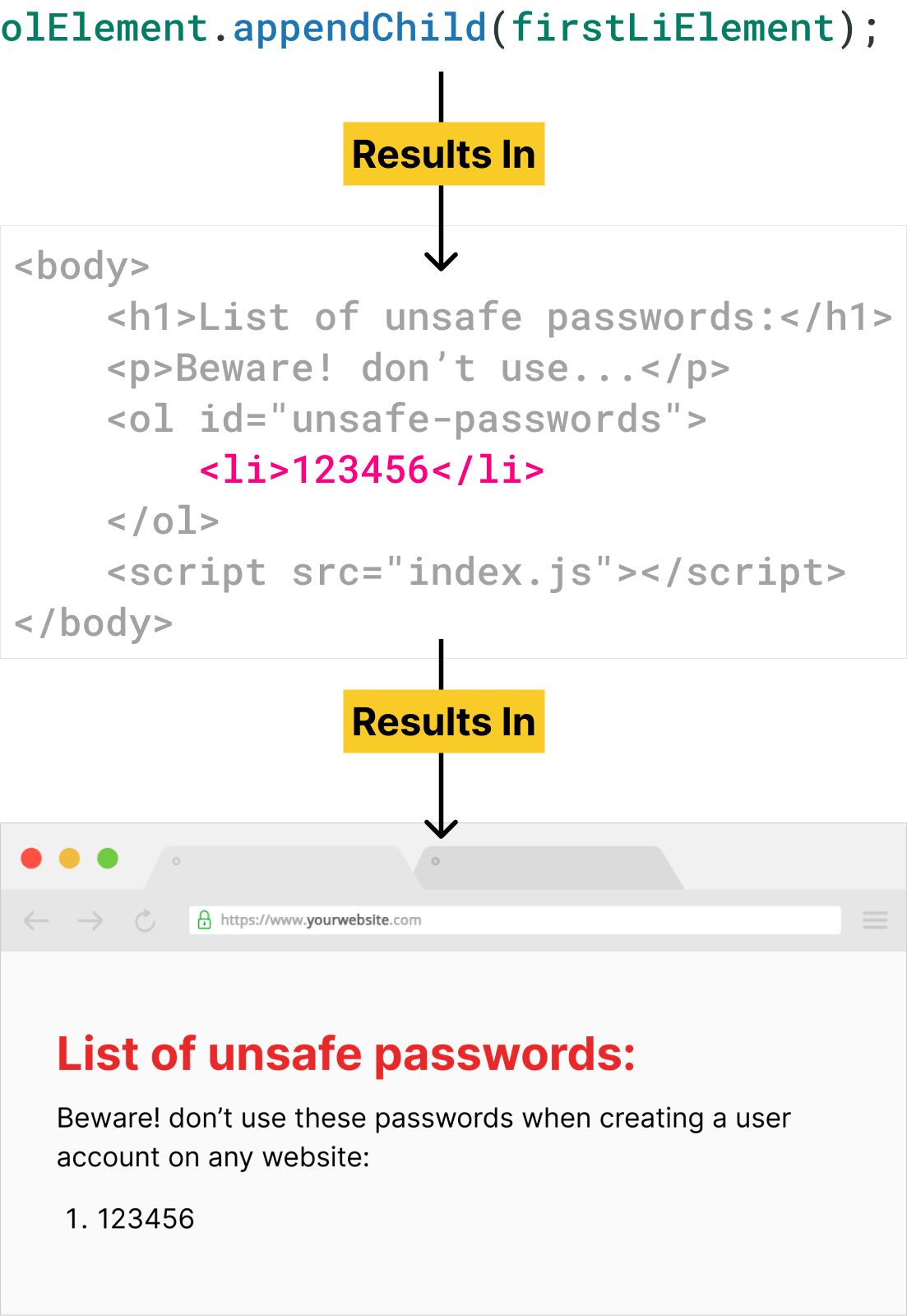
Nice, now let's do the same for the rest of the passwords inside the array.
Here is how you'll output the second password from the array:
// Outputting the second item into the web page
const secondLiElement = document.createElement("li");
secondLiElement.textContent = unsafePasswords[1];
olElement.appendChild(secondLiElement);
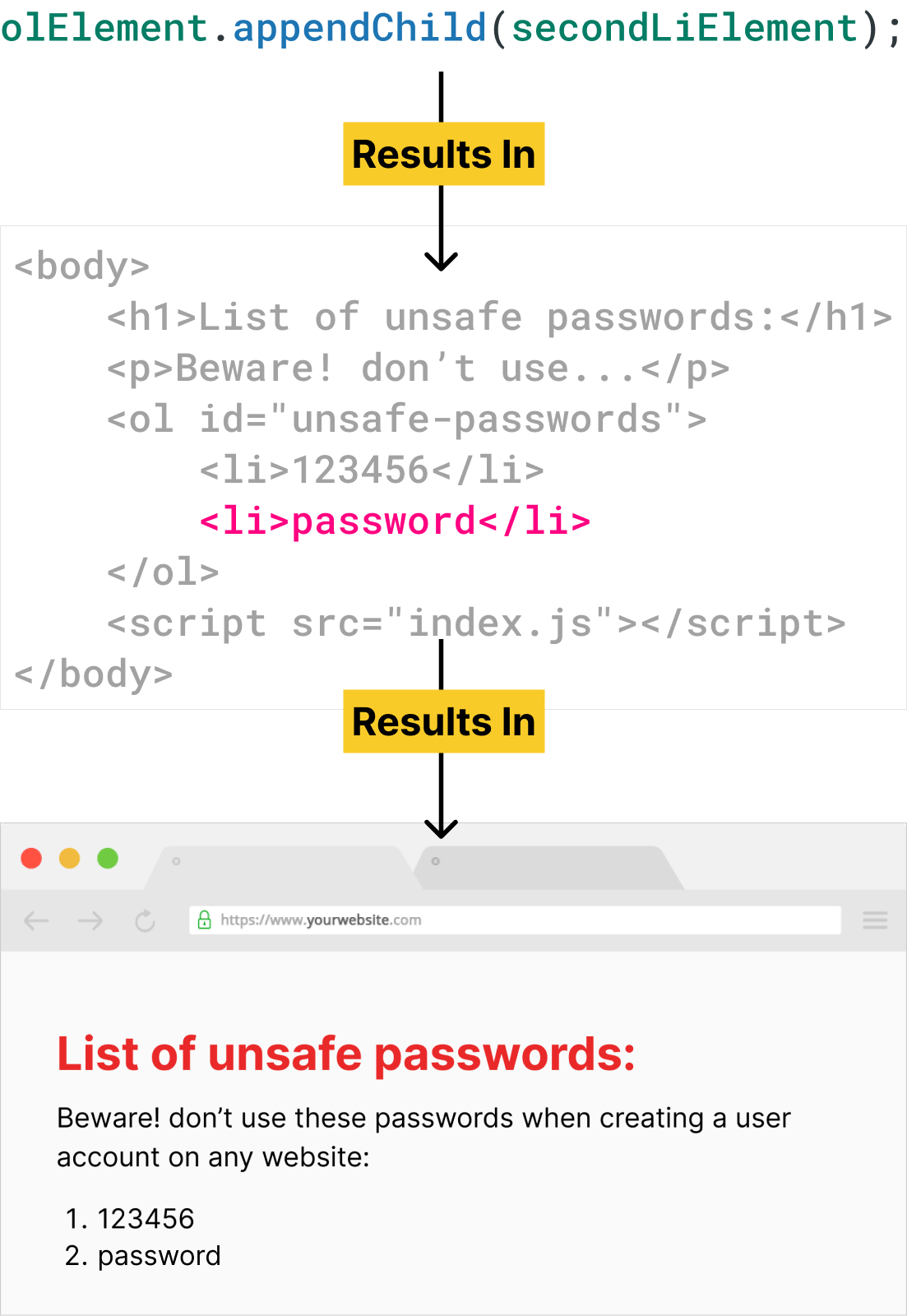
You get the idea, right?
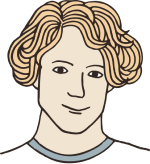
Yep!
Here is the javascript final code for this exercise where all the passwords from the array are getting outputted onto the webpage:
let unsafePasswords = [
"123456",
"password",
"admin",
"qwerty123",
"password123"
];
//Select the parent element
const olElement = document.querySelector("#unsafe-passwords");
// Outputting the first item in to the web page
const firstLiElement = document.createElement("li");
firstLiElement.textContent = unsafePasswords[0];
olElement.appendChild(firstLiElement);
// Outputting the second item in to the web page
const secondLiElement = document.createElement("li");
secondLiElement.textContent = unsafePasswords[1];
olElement.appendChild(secondLiElement);
// Outputting the third item in to the web page
const thirdLiElement = document.createElement("li");
thirdLiElement.textContent = unsafePasswords[2];
olElement.appendChild(thirdLiElement);
// Outputting the fourth item in to the web page
const fourthLiElement = document.createElement("li");
fourthLiElement.textContent = unsafePasswords[3];
olElement.appendChild(fourthLiElement);
// Outputting the fifth item in to the web page
const fifthLiElement = document.createElement("li");
fifthLiElement.textContent = unsafePasswords[4];
olElement.appendChild(fifthLiElement);
If you notice, we are selecting the parent element (ol
) only once, and we are using it for the array items.
Here is the final output of the web page for this exercise:
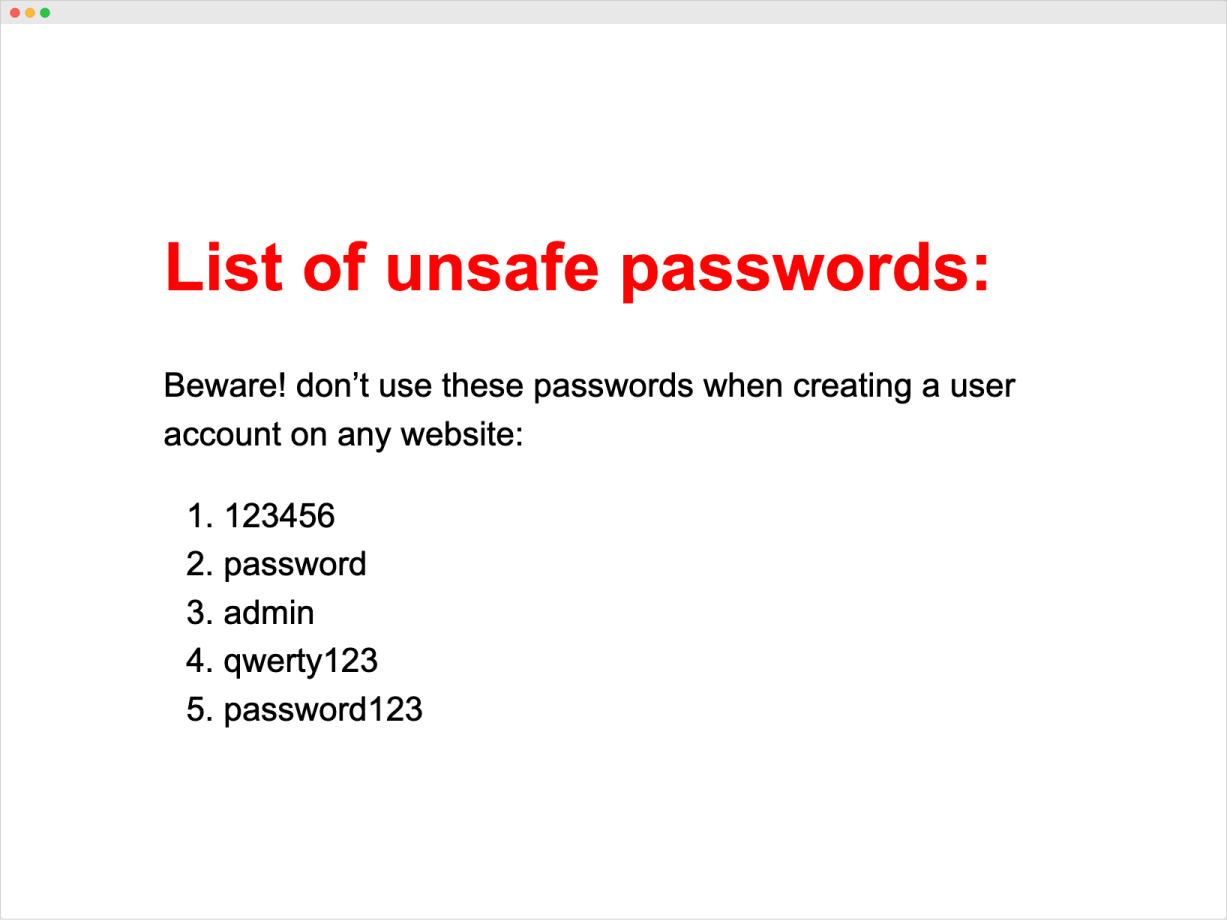
But there is a big problem with the code we have written during this exercise, and we will talk about that in the next lesson.