Exercise: Integrating event object inside our callbacks
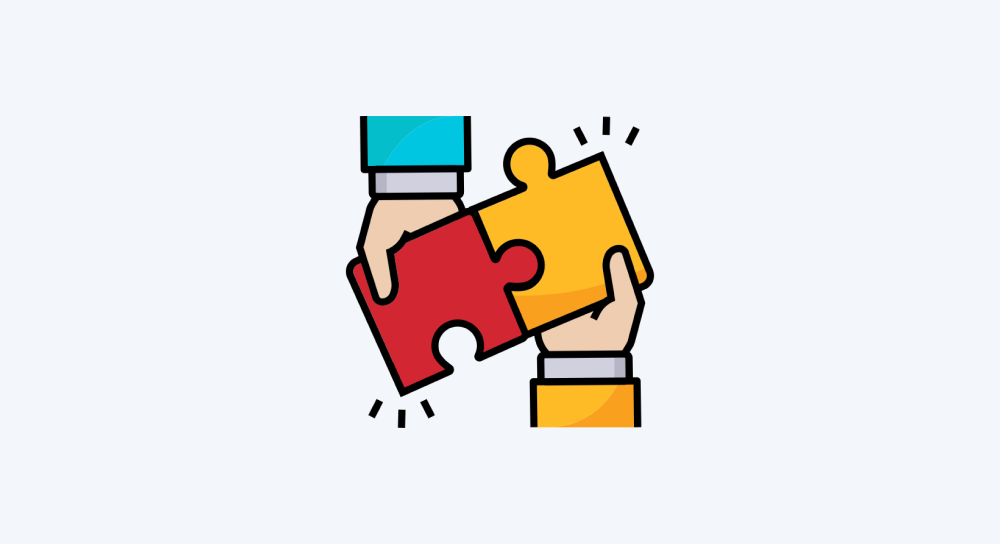
Consider it as an exercise to help you understand the concept of automatic passing of inputs to our callback functions.
If you understand this concept, you have understood the whole concept of passing inputs, be they manual or automatic.
Task: Console.log the event object whenever the user clicks on the menu icon
If you haven't worked on the project before, download the project files from above and open up the index.js
file.
//Select the Menu button
const menuToggleButton = document.querySelector(".menu-toggle");
//Add event listener to it
menuToggleButton.addEventListener("click", function(){
document.body.classList.toggle("off-canvas-is-open");
});
Next, if you notice our callback function, it doesn't utilize the event parameter as part of its definition.
Your challenge is to make it utilized and console.log the event object every time the user clicks on the menu icon.
...
...
Did you embed the event parameter?
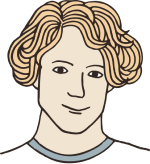
Yep!
Cool! Here is how I did it:
//Select the Menu button
const menuToggleButton = document.querySelector(".menu-toggle");
//Add event listener to it
menuToggleButton.addEventListener("click", function(event){
console.log(event);
document.body.classList.toggle("off-canvas-is-open");
});
Now, every time I click on the menu icon, the event details are logged inside the browser console as an object:
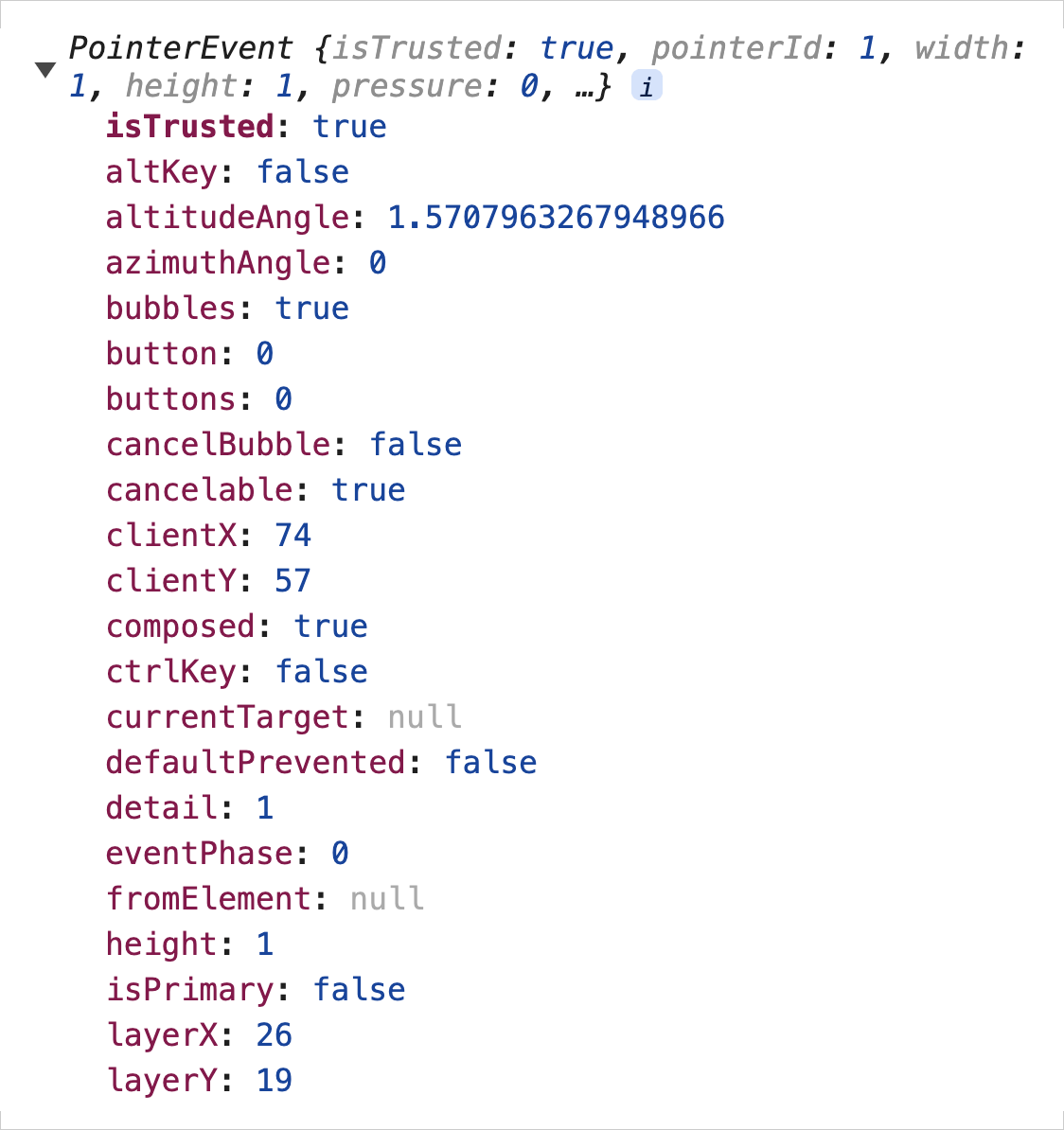
Go through the details of the event object and try to understand what each property could mean so that you can use them whenever their need comes.
Anyway, now do the same thing for the remaining projects.
Task: Console.log the event object whenever the user clicks on the "Show Modal" button and the "Close Modal" button
Download the project files and perform the task:
Here is the solution:
/* Functionality for revealing the modal */
function showModal(event){
console.log(event);
let discountModal = document.querySelector("#surprise-discount-modal");
discountModal.style.display = "block";
}
let showModalButton = document.querySelector(".show-modal-btn");
showModalButton.addEventListener("click", showModal);
/* Functionality for hiding the modal */
function hideModal(event){
console.log(event);
let discountModal = document.querySelector("#surprise-discount-modal");
discountModal.style.display = "none";
}
let hideModalButton = document.querySelector(".hide-modal-btn");
hideModalButton.addEventListener("click", hideModal);
Now, every time we open or close the modal, we are capturing the event details successfully:
That's all for this exercise.
We will put the event object to good use inside the "Disable Right Click" project that is coming up next.