Exercise: Personal variables
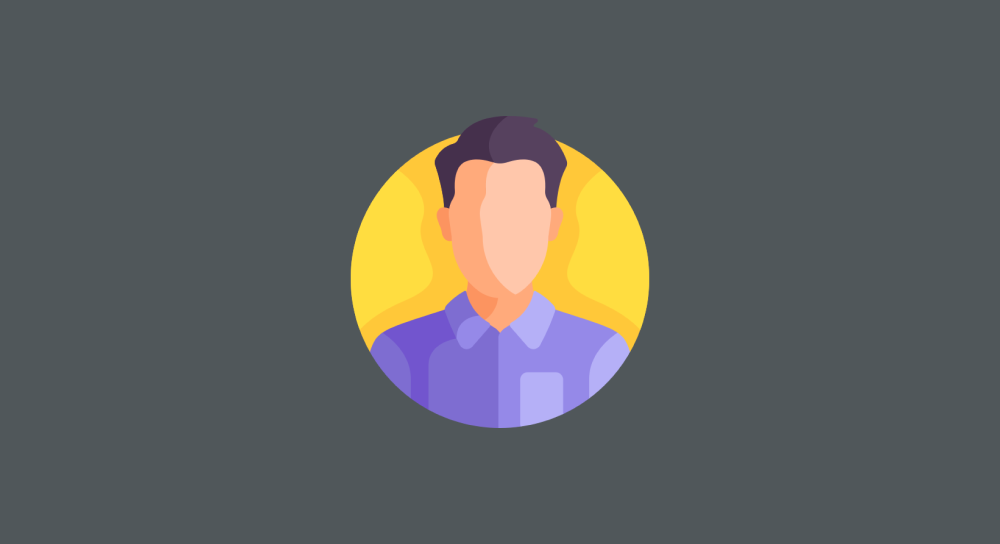
You are now equipped with enough knowledge to create variables and store data in them.
So let's have a small exercise where you create variables for storing your personal information.
You can use a Javascript playground like CodePen, Plunkr, or RunJS App for this exercise. If you are doing that, use fake data.
Exercise:
- Create a variable called
firstName
and store your first name in it. - Create a variable called
lastName
and store your last name in it. - Create a variable called
age
and store your age in it. - Create a variable called
father
and store your father's name in it. - Create a variable called
mother
and store your mother's name in it. - Create a variable called
interestedInSports
and store a boolean value in it. - Create a variable called
married
and store a boolean value in it. - Create a variable called
height
and store a value in centimeters. - Create a variable called
weight
and store a value in kilos. - Create a variable called
hobbies
and store your hobbies as a string in it. - Create a variable for
address
for storing your address. - Update the
age
by increasing it by one year. - Update the
married
by switching your current marital status. - Update the
weight
by reducing it.
Solution
It's okay if your solution looks different from mine. Remember, it's okay to make mistakes.
💡
Read the comments inside the following code to understand the solution better.
//First name and last name are always a string
let firstName = "Naresh";
let lastName = "Devineni";
//It is recommended to store age as a number because you might end up incrementing it automatically every year
let age = 34;
// Names are always strings
let father = "D. Narsaiah";
let mother = "D. Laxmi";
//If "yes", "no" are the only possible answers, use Booleans
let interestedInSports = true;
let married = false;
/*
Now comes the complicated part with measurement related data. Different countries use different measurements for weight.
For example, US people use pounds for weight while India, Newzealand Kilos for weight.
I have instructed you to use kilos for weight and centimeters for height.
So, are you going to store them as strings?
or Will you store them as numbers by assuming that everybody will provide their weight in kilos and height in cm?
The thing is there is no right answer for this. It changes from project to project based on the requirment at hand.
So, for now, I am going with strings. But, later we will go with a proper solution.
*/
let height = 176cm; // This will throw a Javascript error because 176cm is not a valid number.
let height = "176cm"; // This is the correct way!
let weight = "72kgs";
/* Usually, we use arrays or objects for storing a collection of values inside a variable, but for the time being, let's go with a string */
let hobbies = "Playing Cricket, Performing SEO, Reading books";
// Addresses are full of text. So, they must be strings
let address = "HIG 314, Flat. no: 400, Ameerpet, Hyderabad, India, 500072";
/*******************************************
****** Now let's update some variable ******
********************************************/
// As you can see, you can get fancier with comments :P
// Anyway, I grew older by one year :(
age = 35;
/* When you are updating variables, you can change the data type if you want to. For example, I changed the data type from Boolean to a String in the below example */
married = "It's complicated";
// I am now under weight :D
weight = "70kg";
//End of the exercise 🥳. As you can see, you can also have emojis inside your code 🙈.
In the next lesson, we will talk about arrays.