Exercise: Convert personal variables to an object
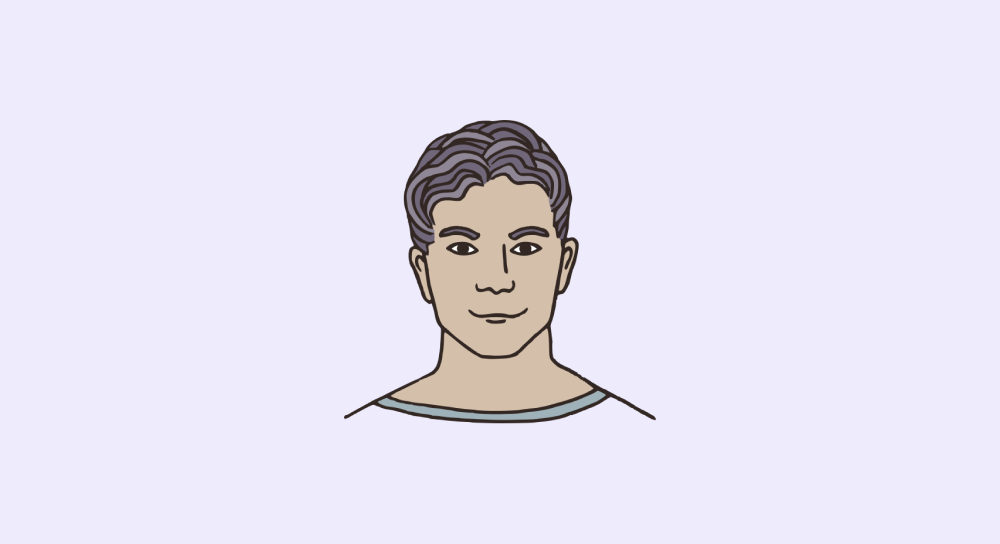
The Problem
If you remember, we previously worked on an exercise called "Personal Variables".
And here are my personal variables, yours will be different:
let firstName = "Naresh";
let lastName = "Devineni";
let age = 34;
let father = "D. Narsaiah";
let mother = "D. Laxmi";
let interestedInSports = true;
let married = false;
let height = "176cm";
let weight = "72kgs";
let hobbies = "Playing Cricket, Performing SEO, Reading books";
let address = "HIG 314, Flat. no: 400, Ameerpet, Hyderabad, India, 500072";
Now, technically, all this information belongs to me, right?
And, according to the previous lesson, in the world of programming, I am an entity with properties too.
So, it makes sense to group all my data together inside an object, right?
Basically, when you store the information related to an entity in separate variables, it conveys that the data stored in those variables are not related to each other.
But using an object to store that data as properties and values will convey that the data belongs to one single entity, me.
And this is what we want.
The Exercise
Convert the above individual variables into a single object with properties and values.
To achieve this, create an object called person
.
The Solution
let person = {
firstName: "Naresh",
lastName: "Devineni",
age: 34,
father: "D. Narsaiah",
mother: "D. Laxmi",
interestedInSports: true,
married: false,
height: "176cm",
weight: "72kgs",
let hobbies = "Playing Cricket, Performing SEO, Reading books",
address: "HIG 314, Flat. no: 400, Ameerpet, Hyderabad, India, 500072"
};
Easy enough, right?
In the next lesson, we will learn about how to access and manage objects.