Exercise: Show the password
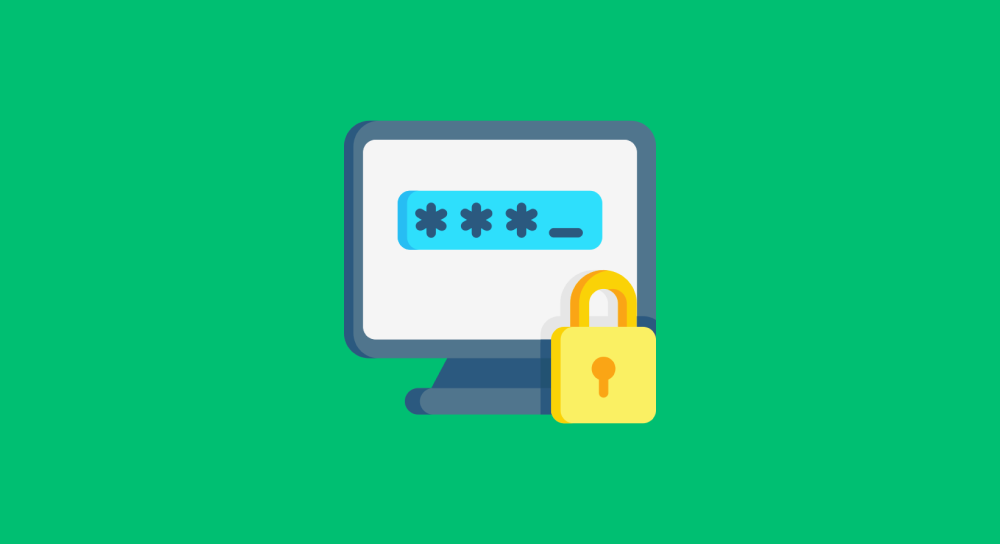
The Exercise
You are now equipped with enough knowledge to change HTML in a basic way.
So, let's have a small exercise.
We have a login form
<!DOCTYPE html>
<html lang="en">
<head>
<title>Show/Hide Password</title>
<link rel="stylesheet" href="style.css" />
</head>
<body>
<form class="login-form">
<div class="fields-container">
<h1>Hi, Welcome back!</h1>
<label for="email">Email</label>
<input class="email-field" id="email" type="email" placeholder="Email">
<label for="password">Password</label>
<input class="password-field" id="password" type="password" placeholder="Password">
<input type="Submit" value ="Log In" />
</div>
</form>
<script src="index.js"></script>
</body>
</html>
And inside this login form, there is a password input field:
<input class="password-field" id="password" type="password" placeholder="Password">
And your task is to change the password input type
to from "password"
to "text"
using Javascript.
<input type="password" />
Use the exercise files below:
Solution
//Step 1: Get the password field
let passwordField = document.querySelector("#password");
//Step 2: Set its "type" property to text
passwordField.type = "text";
The above code will help us change the "type" attribute of the Password field to "password" so that the user can see the password entered.
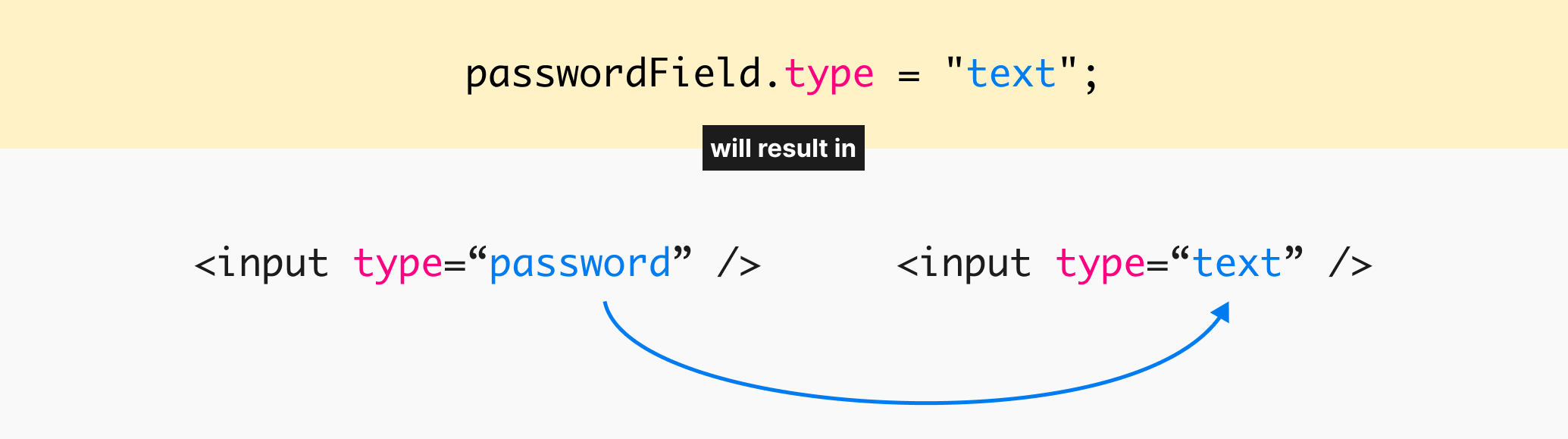
Easy, right?
Anyway, so far, we have only seen how to change HTML markup using by assigning a value to a property.
But sometimes, we have to use methods for that.
And we will see that in the next lesson.