Event listeners will keep listening until we stop them
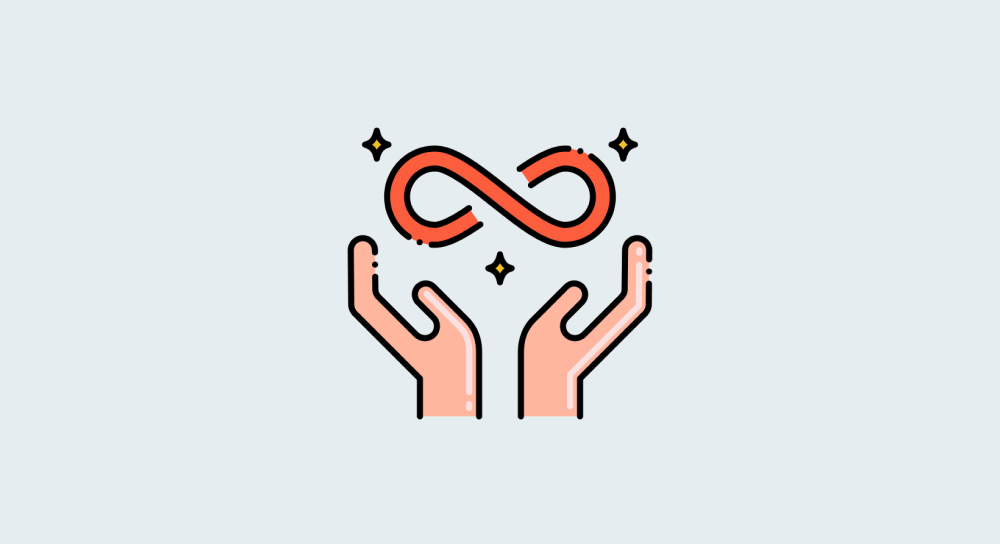
If not already, I want you to realize a very important characteristic of event listeners in Javascript.
And that is, once we attach an event listener to a particular HTML element, we can keep listening to events generated on that particular element. Repeatedly.
removeEventListener
method.Anyway, during the last couple of lessons, we attached a "click"
event listener to the "Unlock your surprise" button.
This means a "click" event is generated is every time the button is clicked.
This also means that the event handler will also get called every time the button is clicked.
And this is why we were able to open the modal repeatedly.
And this is what the user expects too.
The user expects the modal to show up every time the "Unlock your surprise" button is clicked.
The user expects the modal to hide every time the "close" button is clicked.
For most of you, this could be obvious but I just want to be on the same page with others who didn't understand.
Come on, let's do a small exercise to understand the theory I have explained so far.
Open up the index.js
from the last lesson.
Next, add a console.log
message inside the showModal
function:
/* Functionality for revealing the modal */
function showModal(){
console.log("Show modal button is clicked!");
//Step 1: Select the Discount Modal element
let discountModal = document.querySelector("#surprise-discount-modal");
//Step 2: Change the display property of the element to "block"
discountModal.style.display = "block";
}
Basically, the above code will log a message to the browser console every time the "Unlock your surprise" button is clicked.
Similarly, add a console.log
message inside the hideModal
function:
/* Functionality for hiding the modal */
function hideModal(){
console.log("Close modal button is clicked!");
//Step 1: Select the Discount Modal element
let discountModal = document.querySelector("#surprise-discount-modal");
//Step 2: Change the display property of the element to "none"
discountModal.style.display = "none";
}
The above code will log a message to the browser console every time the "close" button is clicked.
Come on, let's test this out:
Did you see that?
A "click" event is getting generated every time we click the "Unlock your surprise" button.
This is making the associated event handler gets called too.
So, we are seeing the modal and a console message saying that "Show modal button is clicked!".
The same thing is happening with the "close" button as well.
Now that I have clarified what's happening with event listeners and their handlers, in the next lesson, we will quickly create a "Content toggle" component.