Event Listeners and event handlers in Javascript
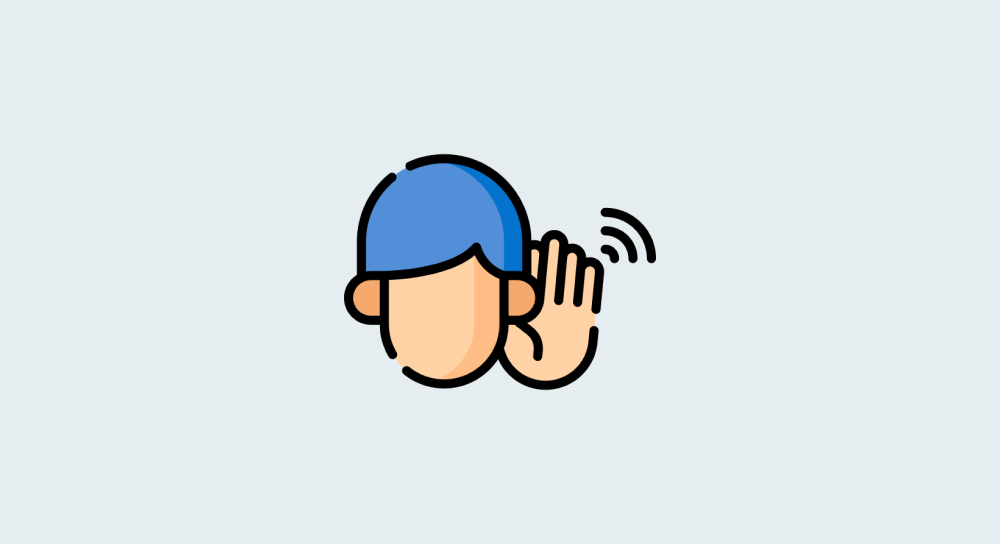
In programming, event listening simply means waiting for something to happen so that we can do something.
For example, we want to listen to the "click" events on the "Unlock your surprise" button so that we can reveal the modal with a discount coupon.
And a good thing is, we can add event listeners to any HTML element using Javascript.
We can do that in two easy steps:
- Select the HTML Element
- Then, add an event listener to it by using the
addEventListener()
method.
Another good thing is, the addEventListener
method also lets you handle the event by letting you do something.
This is better shown than explained.
How to attach an event listener to the "Unlock your surprise" button
Open up the "Discount Modal" project in the code editor.
Then, open up the index.js
file.
function showModal(){
//Step 1: Select the Discount Modal element
let discountModal = document.querySelector("#surprise-discount-modal");
//Step 2: Change the display property of the element to "block"
discountModal.style.display = "block";
}
The index.js
file already contains the function declaration for showModal
function.
Next, after the function declaration of showModal
, type the following code:
//Step 1: Select the "Unlock your surprise" button
let showModalButton = document.querySelector(".show-modal-btn");
//Step 2: Attach the event listerner to it
showModalButton.addEventListener("click", showModal);
Here is what's happening.
First, we are selecting the "Unlock your surprise" button:
let showModalButton = document.querySelector(".show-modal-btn");
Then, we are attaching a "click" event listener to it using the addEventListener
method:
showModalButton.addEventListener("click", showModal);
This line of code says:
"Hey! Call the showModal
function every time the "Unlock your surprise" button is clicked."
If you notice, the addEventListener
method is accepting two parameters:
- Event type - For the event type, we are providing the value of
"click"
. - Event handler - For the event handler, we are providing the name of the function called
showModal
.
The thing is, every time an event is generated, the event handler (showModal
function) will get called.
So, every time a "Click" event is generated on the button, the showModal
function will get called.
And every time the showModal
function is called, it performs the task of revealing the modal.
Come on, let's test this out.
If all goes well, the modal should be revealed when the button is clicked.
Save the index.js
file and then open up the index.html
in the browser.
Finally, click on the button.
It is working indeed.
The modal is getting revealed.
Anyway, if you notice, our code just got complicated.
We can understand what's happening in a better way if we take to analyze the execution flow again.
This is the final code for implementing the modal functionality in javascript:
function showModal(){
//Step 1: Select the Discount Modal element
let discountModal = document.querySelector("#surprise-discount-modal");
//Step 2: Change the display property of the element to "block"
discountModal.style.display = "block";
}
//Step 1: Select the "Unlock your surprise" button
let showModalButton = document.querySelector(".show-modal-btn");
//Step 2: Attach the event listerner to it
showModalButton.addEventListener("click", showModal);
And here is the execution flow of the "Discount modal" project:
1) The user visits the web page and the browser starts parsing the index.html
file.
2) It then finds the style.css
and loads it.
3) By the time it loads the index.js
file, the web page looks like this:
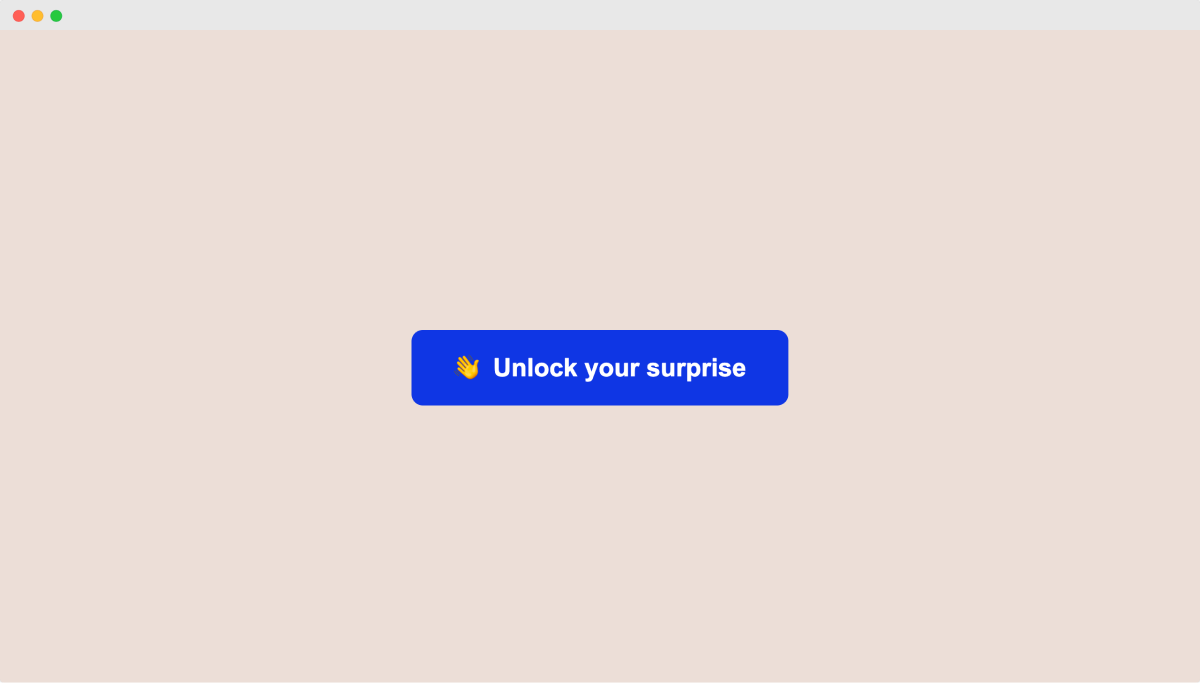
4) Finally, the browser finds the index.js
file and starts executing it line by line.
First, it sees the showModal
function declaration but it does nothing by skipping to the next line of code.
But at the same time, the browser remembers that there is a function declaration called showModal
and it might find the execution call for it.
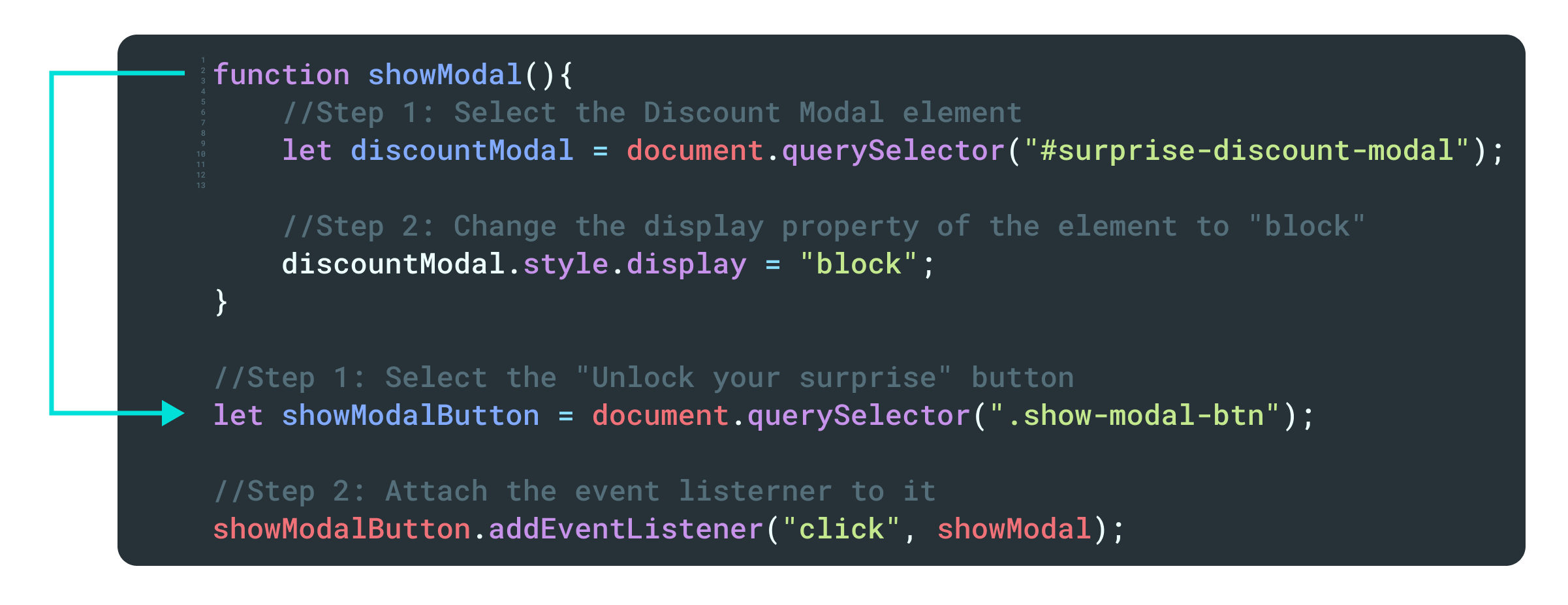
Next, it gets the "Unlock your surprise" button and saves it inside the showModalButton
variable.
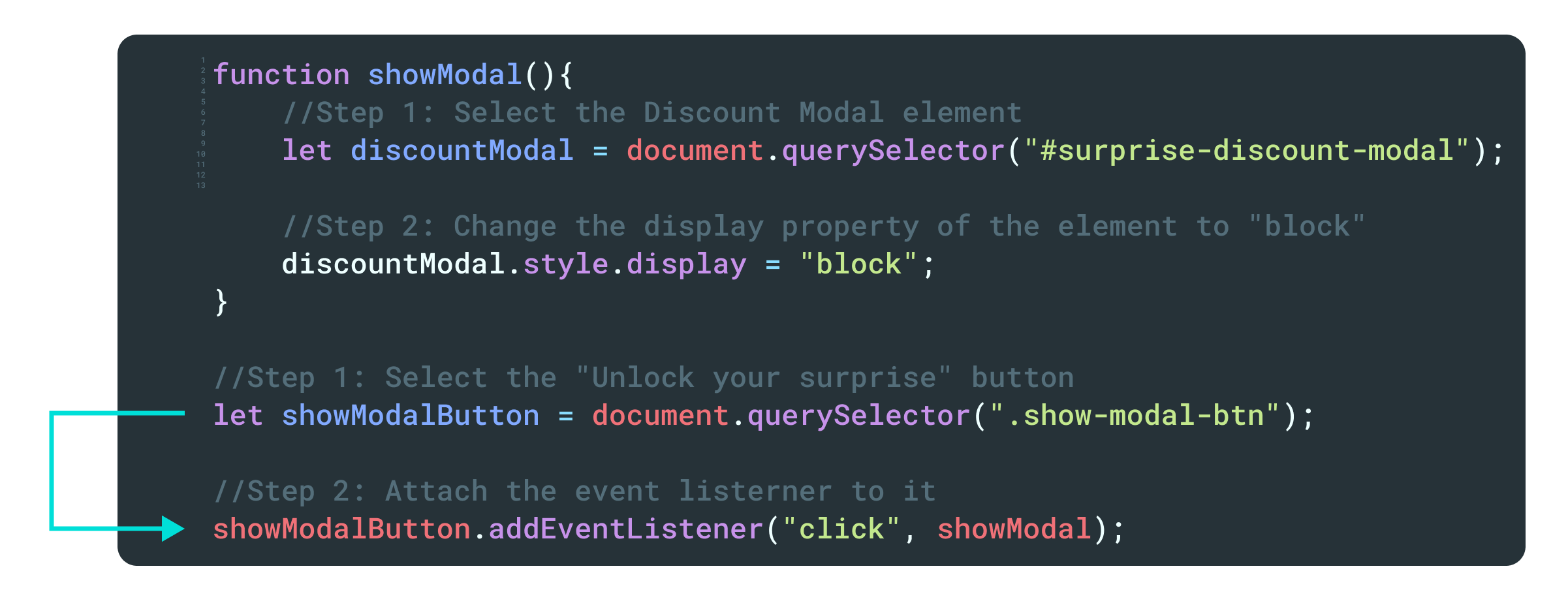
Finally, it attaches the "click" event listener to the "Unlock your surprise" button and thinks that:
"Oh! I must call the showModal
function every time a 'click'
event is generated on the 'Unlock your surprise'
button element."
And then it exits out of the execution flow of index.js
completely and gets busy with other work.
showModal
function will get executed only when the button is clicked.So, the browser has nothing to do currently inside the
index.js
and exits out of the file and finished the rendering of the page.5) Now let's just say that the user has clicked on the button.

6) As soon as the user clicks on the button, a "click"
event is generated on the button.
And this reminds the browser that a "click" event is attached to the button and it starts executing the showModal
function because the showModal
function is provided as the event handler.
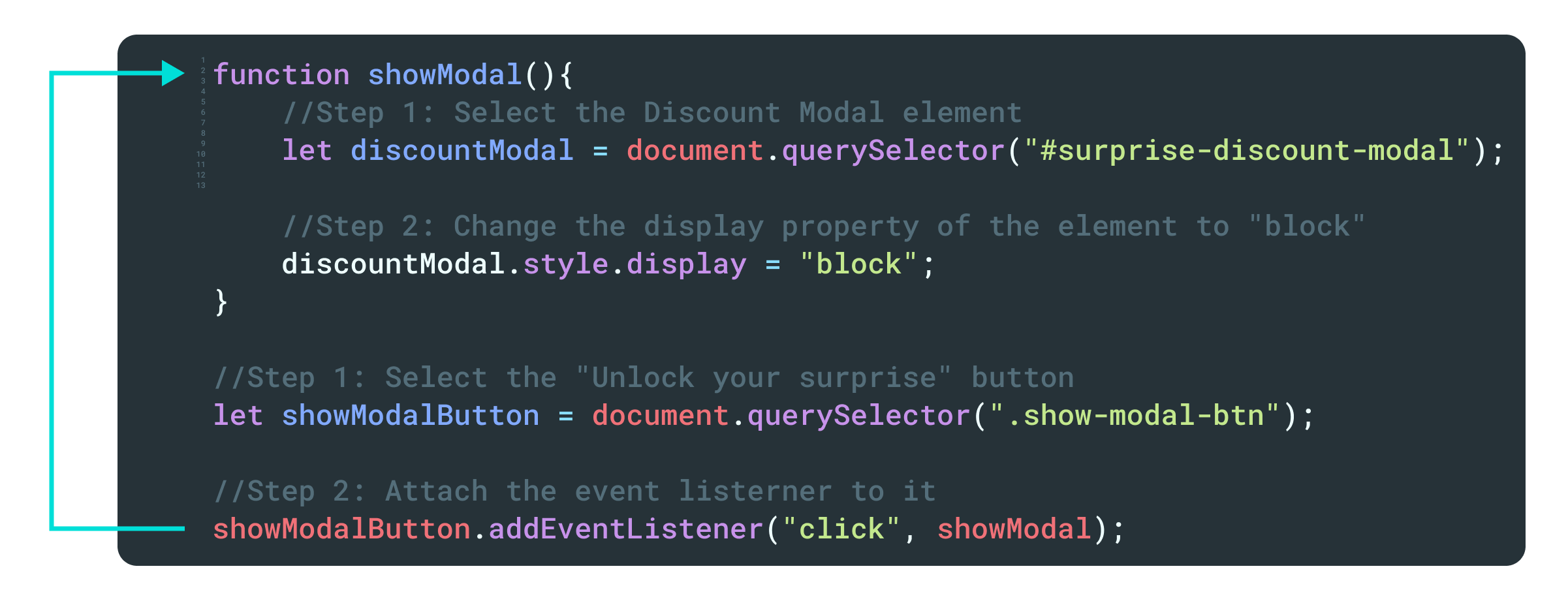
Next, inside the showModal
function, it selects the modal element and saves it inside the discountModal
variable.
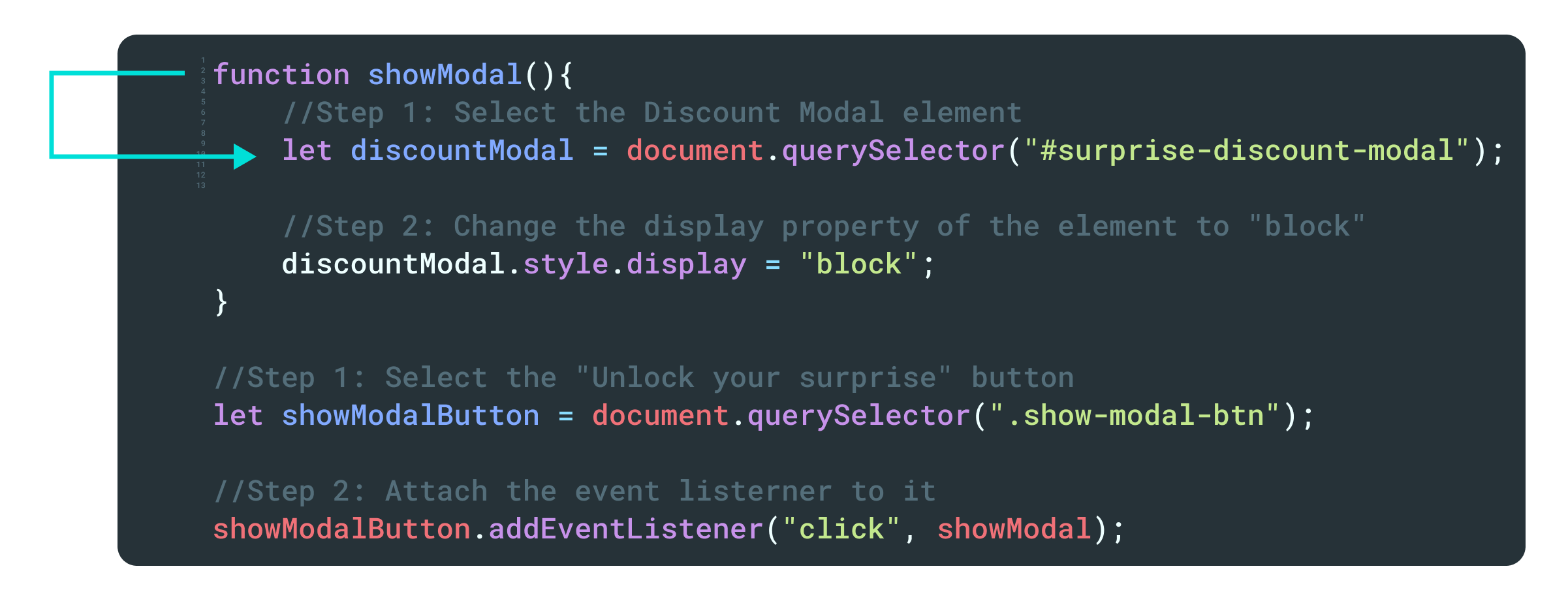
Finally, the browser reveals the modal by display
property of the modal is changed to "block"
to reveal the modal.
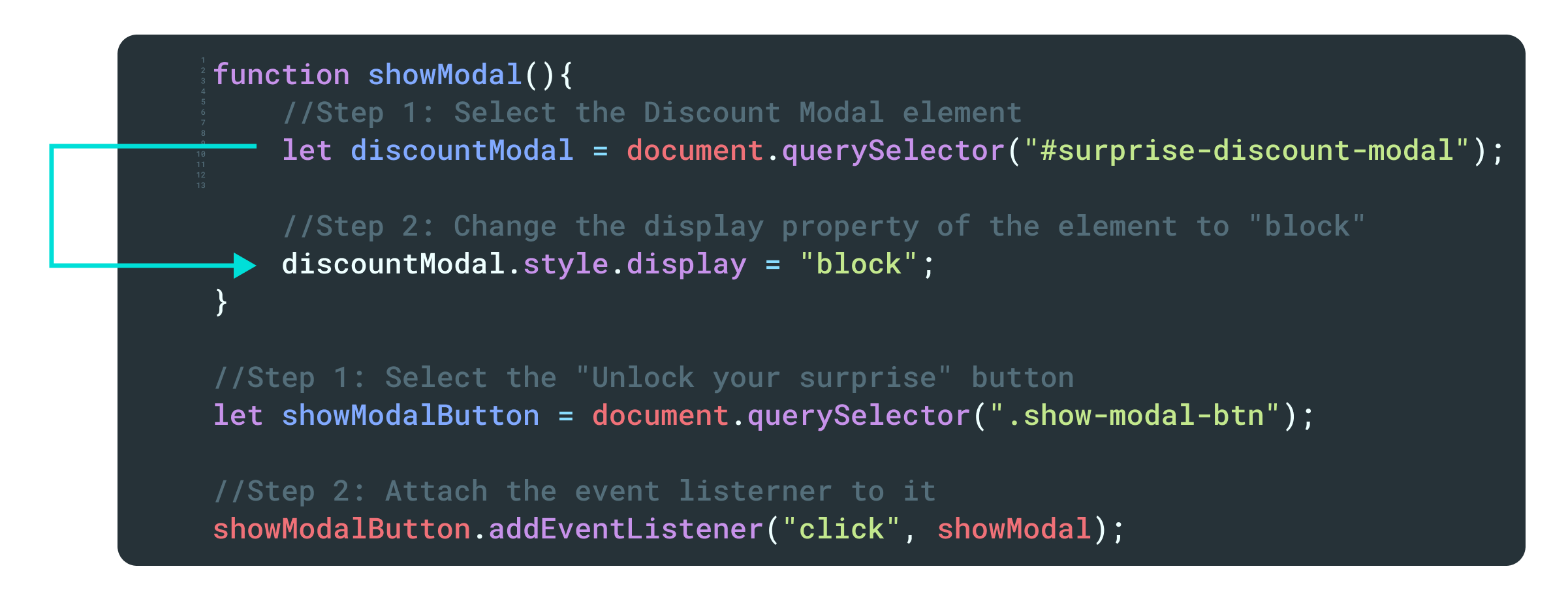
Here is the result of executing that last line of code inside the showModal
function.
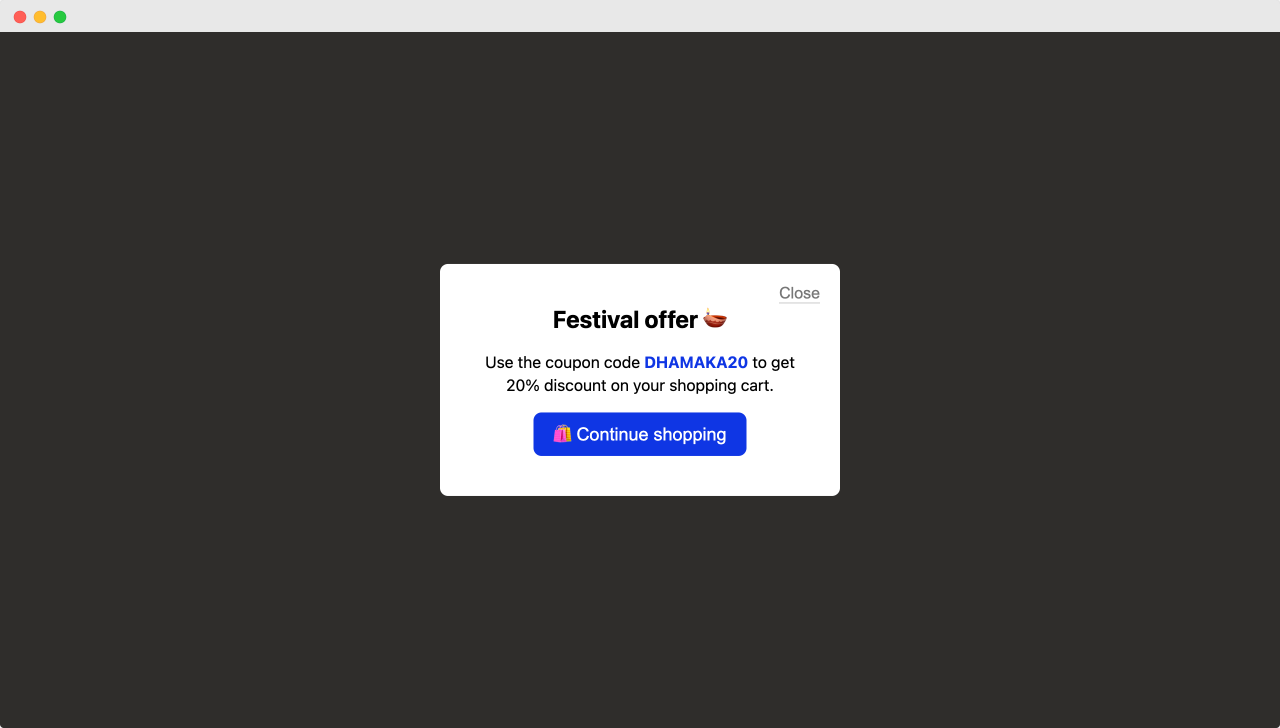
Finally, the browser exits the showModal
function and gets back to other work.
showModal
function will get called to reveal the modal. This is exactly how the events, event listeners, and event handlers work inside Javascript.
But we are not done yet.
If you notice, we are currently revealing the modal, but there is no way to close it.
So, in the next lesson, I have a small test for your knowledge.