Form events in Javascript
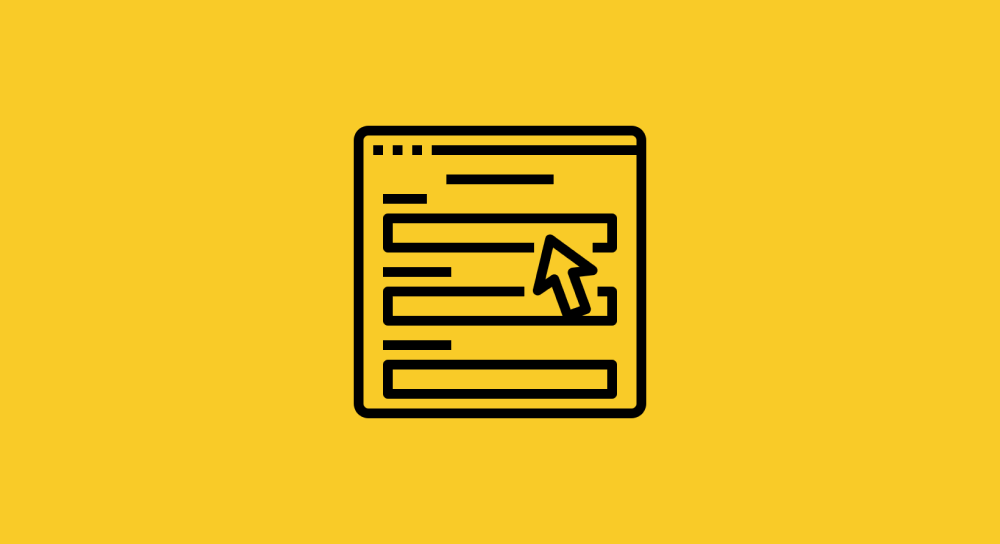
The first step in enhancing an HTML form with Javascript is listening to the form-related events.
Javascript gives us an opportunity to listen and react to many kinds of form events.
Download the project files and work along with me to understand how each form event works.
The index.html
file contains a form with some common fields related to user registration.
Throughout this lesson, we will attach various event listeners to the form and its fields to understand how form events work.
1) A submit
event is generated whenever a form is submitted
const accountForm = document.querySelector(".account-form");
accountForm.addEventListener("submit", function(event) {
//Handle the form submission here
});
Submitting an HTML form usually refreshes the page, wiping out entered data:
For example, in the above video, submitting the form by clicking on the "Login" button refreshed the page and cleared the form details we entered.
This is fine if you're processing the form data using a server-side language like PHP because they can extract the filled-out details during the page refresh.
But if you want to process the form submission with Javascript, you need to prevent this default behavior (form submission's refresh) by using event.preventDefault()
on the submit
event:
const accountForm = document.querySelector(".account-form");
accountForm.addEventListener("submit", function(event) {
// Prevents the default form submission behavior
event.preventDefault();
// So that you can validate and process the form data here
});
This allows us to work with the form data without losing it because of a page refresh.
For example, here is how the same form submission behaves after calling the event.preventDefault()
method on the form submission event:
Did you see that?
There is no longer any page refresh after the form submission, and the form details I entered are still intact.
This is the exact behavior we want if we want to process the form data using Javascript.
So, please remember to utilize the event
object as part of the callback function that handles the form submission event.
Next, let's check out the events related to individual input fields inside the form.
2) The change
event is triggered by the input field when a user makes changes to it and then moves away from it
The change
event is useful for capturing final user input and can be used in conjunction with validation techniques to ensure data accuracy before the form submission.
For example, in the above video, the change
event is triggered on the "Full Name" field when I entered the name and then moved away from it by hitting the tab
key.
It gave us access to the final value I typed, that is, "Naresh Devineni".
const fullNameField = document.querySelector("#full-name");
fullNameField.addEventListener("change", function(event) {
console.log(event.target.value);
});
This also means that the change event must be attached to an individual field inside the form but not to the main form element.
event.target
always represents the HTML element on which the event is fired. The
event.target.value
represents the value of that target element. More on this in the next lesson.The change event
applies to most form elements except file uploads.
Please remember that text-based input fields (text, password, email, number, textarea) fire the change
event when the user loses focus after making changes.
However, there is a small exception to this "lose focus to trigger change
event" rule.
The select boxes, checkboxes, and radio buttons trigger the change
event immediately after being clicked or selected. You don't have to lose focus to trigger the event:
Here is the Javascript code to recreate the above interaction:
const countryField = document.querySelector("#country");
countryField.addEventListener("change", function(event){
console.log("Country selected:", event.target.value );
});
const showPasswordField = document.querySelector("#show-password");
showPasswordField.addEventListener("change", function(event){
console.log("Is checkbox checked?", showPasswordField.checked);
});
You'll understand this code in the next lesson.
3) An input
event is triggered with every keystroke or change within the input element, providing immediate feedback to users.
If you notice, the input
event is firing every time we put a character inside the "Email" field:
const emailField = document.querySelector("#email");
emailField.addEventListener("input", function(event) {
console.log(event.target.value);
});
This event allows us to capture data as the user types, enabling features like real-time character count or input suggestions.
For example, the above video demonstrates how to provide real-time suggestions about the strength of the password as the user types it.
4) The keyup
and keydown
events
These events are triggered when the user presses (keydown
) or releases (keyup
) a key on the keyboard.
They offer similar functionality to the input
event for capturing user input.
But just like the input
event, we can use both keyup
and keydown
them to get the data as the user types it in.
const emailField = document.querySelector("#email");
emailField.addEventListener("keyup", function(event) {
console.log(event.target.value);
});
There are a few important differences between keyup
, keydown
and input
events, but those differences are better understood while working on real-world projects.
You will understand the difference when we will work on the password validation project in an upcoming module.
5) The focus
event is triggered when an input field receives focus (e.g., clicking on it or tabbing to it).
document.querySelector("#email").addEventListener("focus", function() {
// Code to execute when the input field receives focus
});
6) Finally, the blur
event is triggered when an input field loses focus (e.g., clicking elsewhere or pressing a tab).
document.querySelector("#email").addEventListener("blur", function() {
// Code to execute when the input field loses focus
});
That's all you need to know about form events for now.
In the next lesson, we will learn how to get the values of the form fields when they are changed or when the form is submitted.