Data type: Object
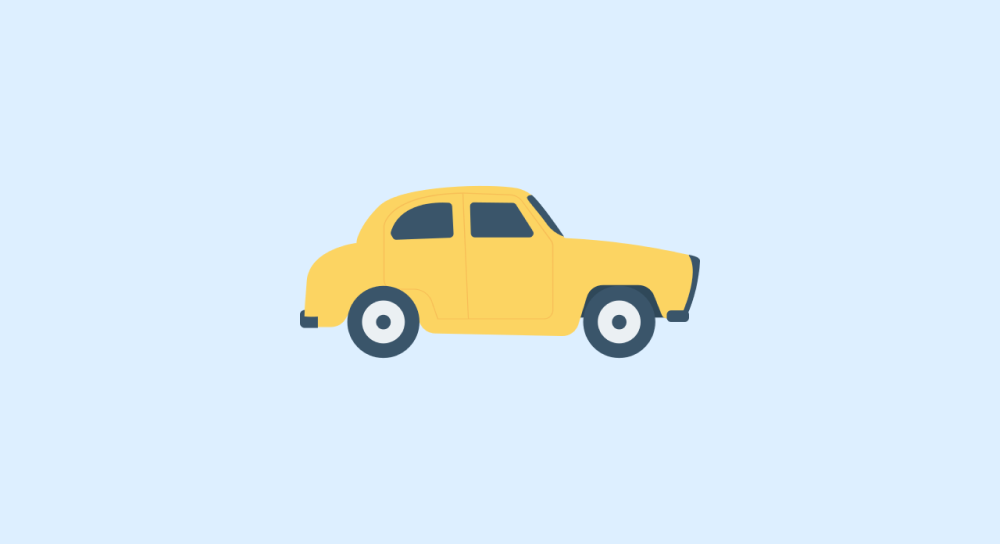
If you understand objects, then you understand Javascript.
If there are no objects, then there is no Javascript.
Remember that when reading the rest of the lesson :)
So far, we learned how to store simple values inside a variable.
For example:
const name = "Naresh Devineni"; // String
let age = 34; // Number
let isMarried = false; // Boolean
const hasRemarks = null; // Null
But what if you want to store a more detailed piece of information inside a variable?
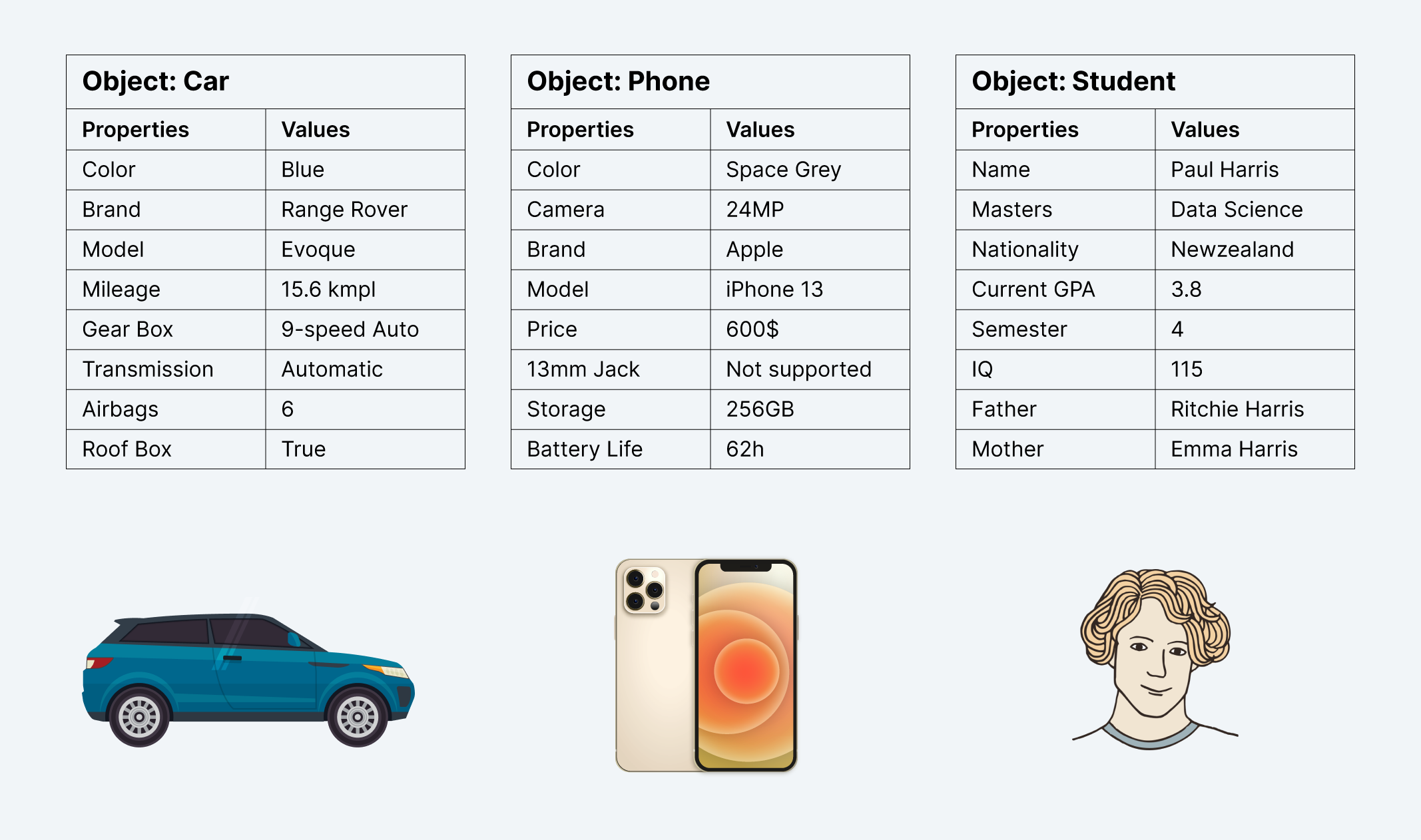
For example, what if you want to store all the information about a particular car inside a single variable?
If you realize, a car has a lot of properties (specifications).
It is ideal to group all the specifications of a car together as one tight unit of information.
So, how do you store all the specifications of a particular car inside a variable?
Just like what we did with the hobbies
variable, one option is to store car specifications in the form of one big string:
let carSpecifications = "Blue, Range Rover Evoke, 15.6 kmpl, 9-speed Auto, Automatic, 6, True";
And this might look right, too.
But if you notice, the specifications are not easy to grasp for a person with a basic understanding of cars.
For example, what does the value 6
represent?
Is it about the number of gears? Or is it about the number of airbags?
Similarly, what does the value true
represent?
It is not clear, right?
So, it would be helpful if we could store information in a more detailed way with easy-to-understand labels.
Labels will kill the assumptions.
And this is where the Object
data type comes in.
So, what is an object?
Objects help us store data about a single entity in a detailed way.
When I say single entity, I mean:
- HTML Element
- Mobile phone
- Car
- Person
- Laptop
- Shopping cart
You get the idea, right?
An object can have properties.
If we take any entity in this world, it will have a lot of properties or specifications.
For example, let's take a car as an entity.
A car can have properties like:
- Color
- Fuel type
- Airbags
- Mileage
And 20 other properties.
Similarly, objects in Javascript can have properties as well.
In other words, when we create an object in Javascript, we have to add properties to it, and we can get as many details as possible.
In Javascript, there is an easy mechanism for storing and managing the properties of an object.
Come on, let's see this in action.
How to create an object and store the data of properties in it
You can start creating an object by using curly braces {}
:
let iPhone = {};
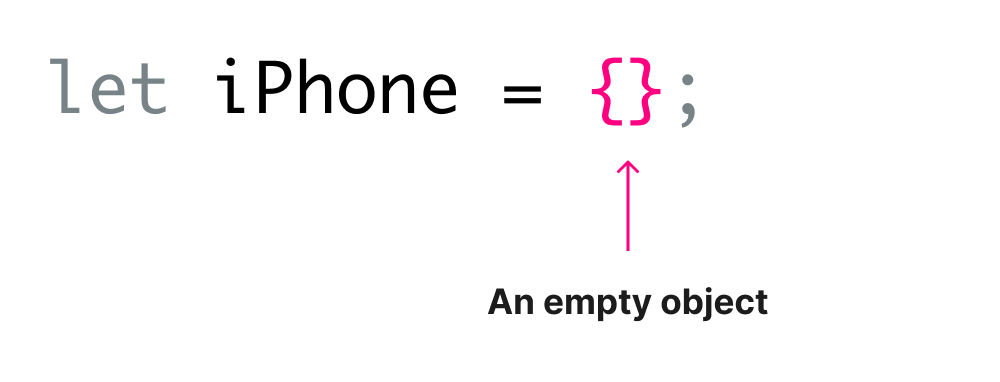
The opening {
and closing }
curly braces will create an object.
And, the properties must go inside the curly braces {}
as key-value pairs.
let someObject = {
keyOne: "Value one",
keyTwo: "Value two",
KeyThree: "Value three"
};
A key
is nothing but the name of a property
and you can link a value to it.
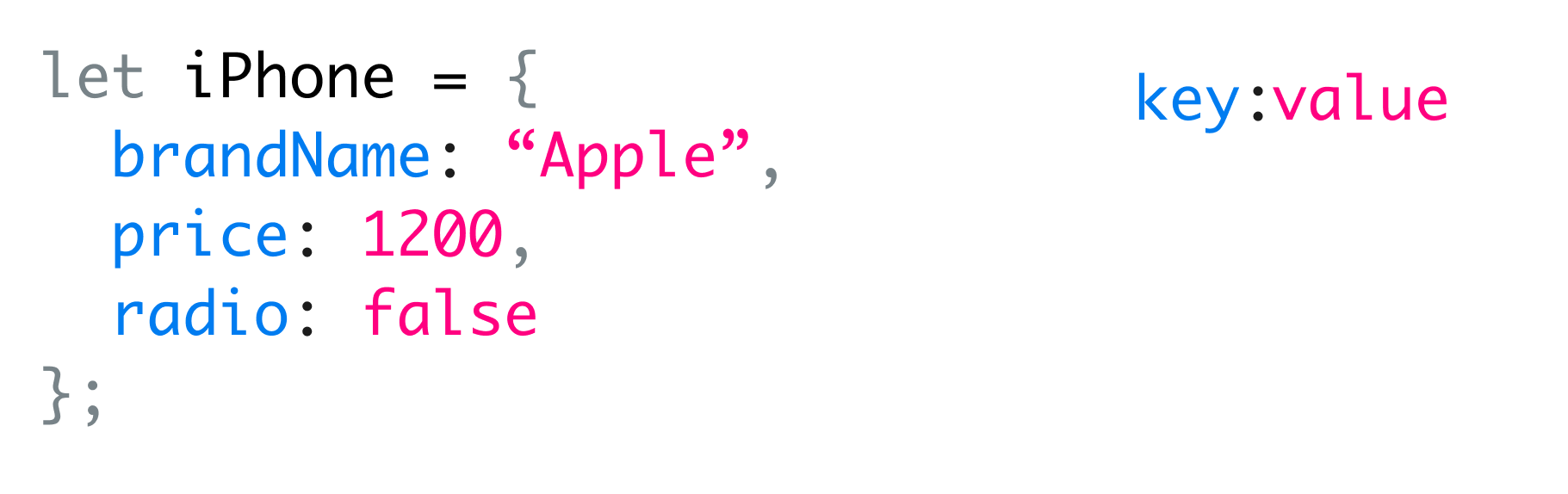
A value
must be assigned to a key
using the colon :
symbol.
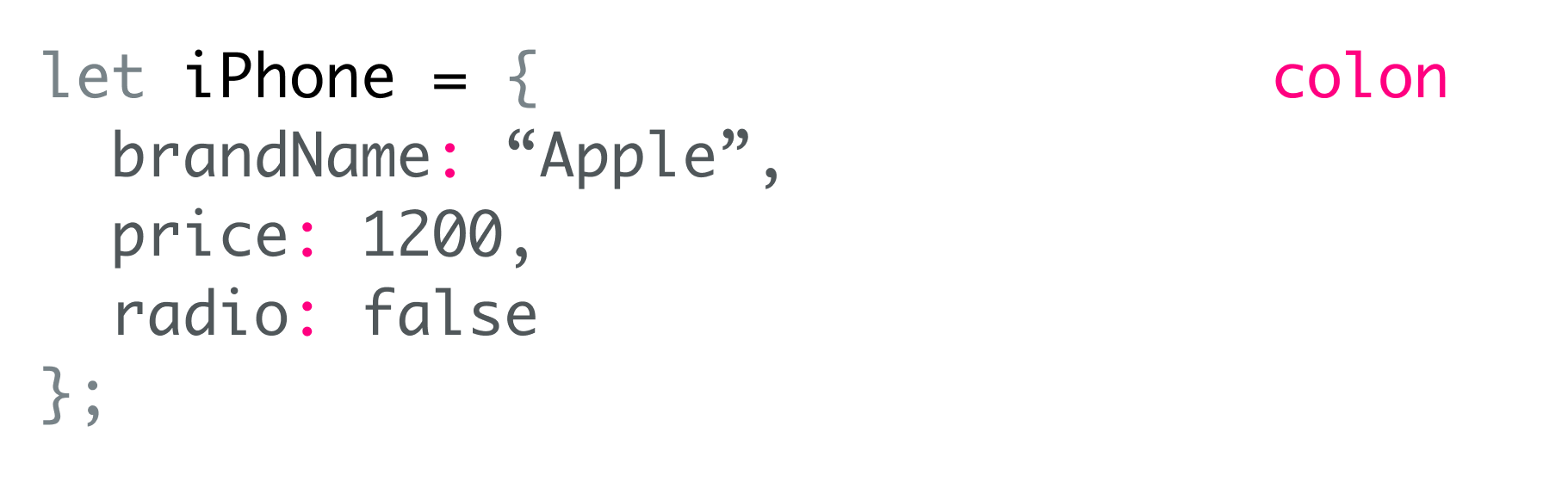
A comma must separate each key-value pair.
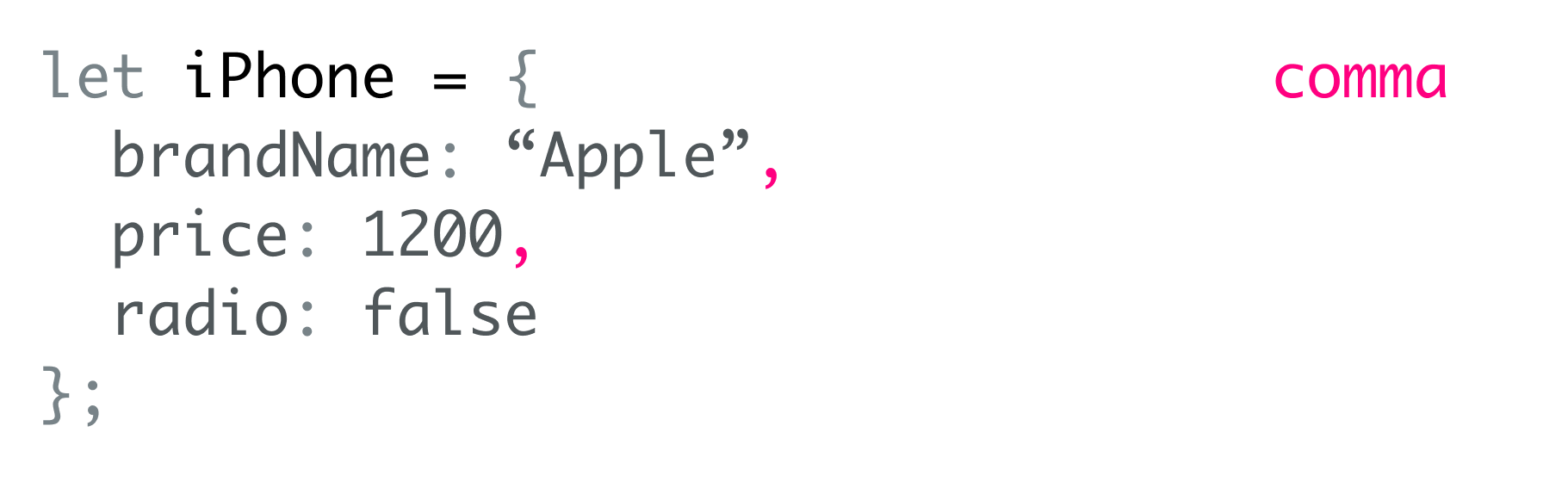
You can omit the comma
for the last key-value pair inside an object. If you want to keep it, then it's completely fine, too.
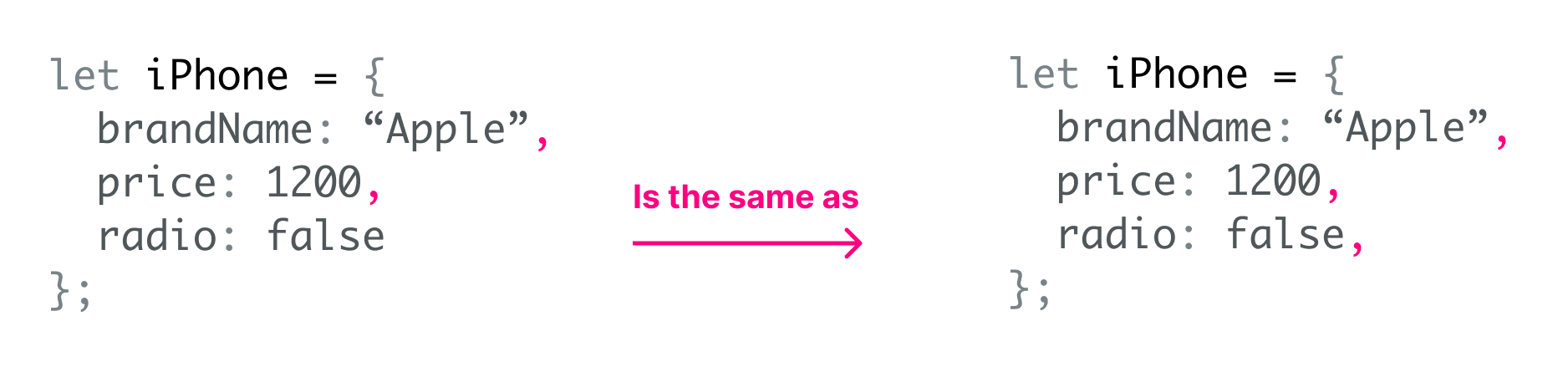
Here is a more realistic example:
let iPhone = {
brandName: "Apple",
modelName: "iPhone 13",
color: "Space Grey",
resolution: "750 x 1334 pixels",
chipset: "Apple A15 Bionic",
cardSlot: false,
radio: false,
price: 600,
//... remaining specifications
};
An iPhone is represented as an object.
If you notice, you can assign any data type for properties inside the object.
Rules of naming the keys
A key
inside an object is similar to a variable name but differs technically.
Having said that, they both share the same responsibility of helping you reference a value.
So, the same naming rules of variables also apply to an object's key as well.
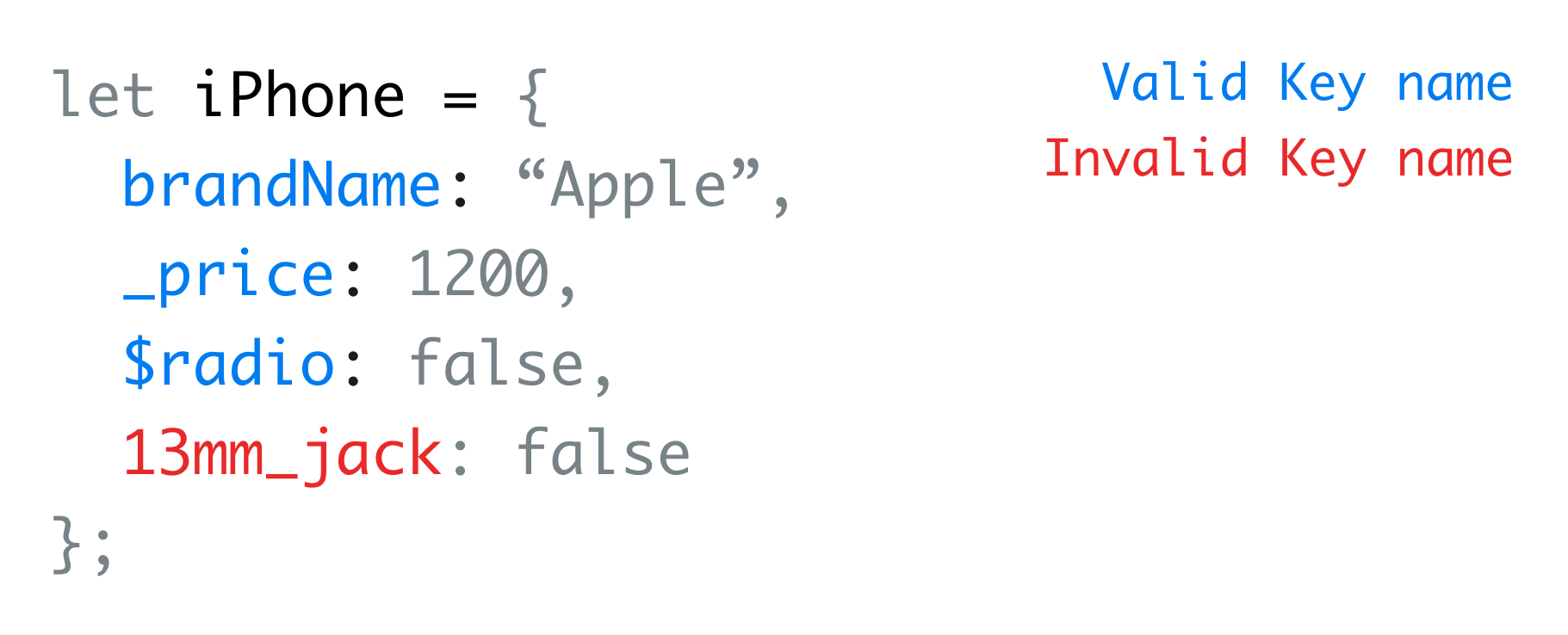
But there is one important freedom.
You can use an invalid variable name for a property name.
For example, 13mm Jack
is an invalid identifier because it has a space in it.
But you can make it a valid property name by surrounding it with quotes. Otherwise, Javascript will throw a syntax error.
let iPhone = {
"13mm Jack": false
};
Using this kind of invalid identifier as a key
is especially useful when you output both the key name and its value as information on a web page.
This is better shown than explained, but the time is not right yet :D
Data types for the value of a property
You can assign a value of any data type to an object's property.
String, Number, Boolean, null, undefined, Object, symbol, bigInt, Array.
All of them.
let iPhone = {
brandName: "Apple", // String
cardSlot: false, // Boolean
price: 1200, // Number
headPhoneJack: null, // Null value
misc: { // Object
colors: ["black","red", "space grey" ], // Array (more on this later)
batterLife: "62 Hours", // String
models: ["A2783", "A2595", "A2785"] // Array
}
output_properties_as_table: function() {} //Function (more on this later)
};
An iPhone is represented as an object
As you can see, you can also store an object inside another object.
Isn't that fantastic?
But I can't stress this enough: The data must belong to a single entity
There is no one to police you from creating an object that contains information about multiple entities.
For example, you can keep the data of multiple iPhones in the same object:
let iPhones = {
iPhone14: {
modalName: "Apple",
cardSlot: false,
price: 1200,
headPhoneJack: null
},
iPhone15: {
modalName: "Apple",
cardSlot: false,
price: 1500,
headPhoneJack: null
}
};
Multiple iPhones represented in a single object
But this is the wrong usage of an object.
For the sake of easy programming, an object must represent a single entity.
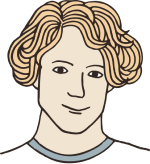
Got it. But then, what if I want to store multiple entities in a single variable?
This is where the "Array" data type comes in.
Arrays allow us to store a collection of entities inside a single variable
let iPhones = [
{
modalName: "Apple",
cardSlot: false,
price: 1200,
headPhoneJack: null
},
iPhone15: {
modalName: "Apple",
cardSlot: false,
price: 1500,
headPhoneJack: null
}
];
Apart from objects, you can store simple values of any data type inside an array:
let hobbies = ["Playing Cricket", "Watching TV", "Reading Books"];
But we will discuss Arrays in a future lesson.
They are not needed for our progress as of now.
For now, we will perform a small exercise to cement our understanding of objects.