Creating a custom function that works with parameters
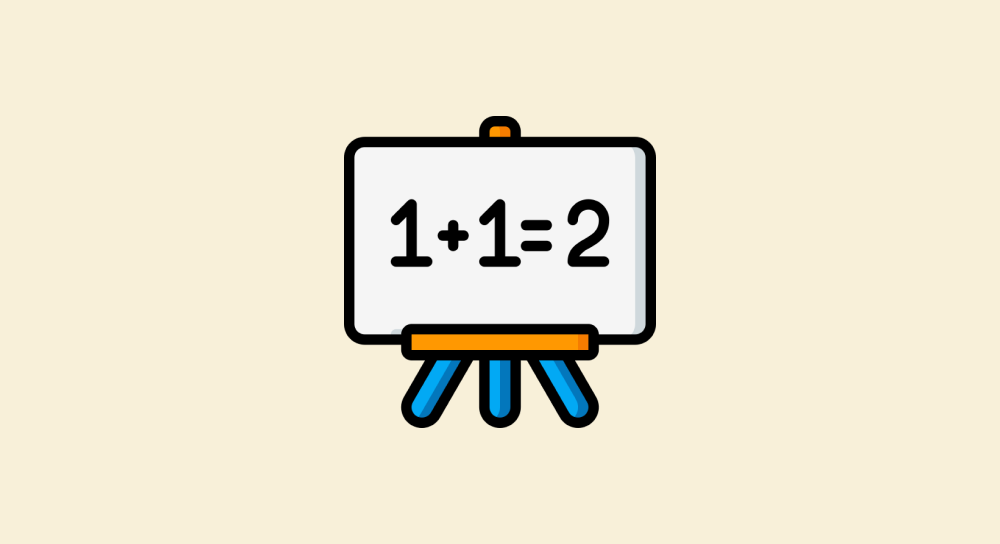
You already know how to create a custom function.
For example, during the accordion project, we created the toggleAccordionContent
function:
function toggleAccordionContent(){
const accordion = document.querySelector(".accordion");
accordion.classList.toggle("is_open");
}
Similarly, we created showModal
and hideModal
functions while working on the modal project:
function showModal(){
let discountModal = document.querySelector("#surprise-discount-modal");
discountModal.style.display = "block";
}
If you notice the above function definitions, they do not accept any input parameters.
That is because they don't need any input supply to do their job.
They are like fire extinguishers that contain everything inside them already to stop the fire.
However, sometimes, you will need to create a custom function that must work with single or multiple input parameters.
But before we learn how to create a function with input parameters, let's understand their purpose.
The need for input parameters inside custom functions
Here is a custom function that adds two numbers:
function addTwoNumbers(){
//Perform the calculation
const result = 100 + 200;
//Print the calculation's result
console.log(result);
}
addTwoNumbers(); //Outputs 300
When we call the addTwoNumbers
function, it outputs 300
to the console.
It is doing a job successfully.
But if you notice, we hard-coded the values 100 & 200 inside the function definition.
So, no matter how many times we call the function, it always outputs the sum of 100 & 200 and not any other numbers:
function addTwoNumbers(){
//Perform the calculation
const result = 100 + 200;
//Print the calculation's result
console.log(result);
}
addTwoNumbers(); //Outputs 300
addTwoNumbers(); //Outputs 300
addTwoNumbers(); //Outputs 300
So, the result of calling the addTwoNumbers
function is always 300.
If that is the case, why should we even create a function and call it?
We could just perform the calculation directly without declaring a function for it, right?
//Perform the calculation
const result = 100 + 200;
//Print the calculation's result
console.log(result);
This would still output the same result.
We usually create a function to perform a dynamic task over and over again.
In other words, we want our function to work with different values inside it.
So, a function definition should never contain hard-coded calculations:
//This is wrong
function addTwoNumbers(){
//100 & 200 are the hard-coded values
const result = 100 + 200;
console.log(result);
}
Instead, we want the addTwoNumbers
function to be able to perform the sum of any two numbers we provide.
Not just fixed calculations, such as 100 and 200.
For example, sometimes, we want the addTwoNumbers
to be able to perform the sum of 10, 10
and output the result in the console in a dynamic way.
And sometimes,we want the addTwoNumbers
to perform the sum of 38, 72
.
You get the idea, right?
How do we achieve that?
This is where input parameters come in.
When creating a custom function, we can make it accept input parameters in the following syntax:
function xyz(parameter1, parameter2,...,parameterN){
//Do something with the parameter1, parameter2, etc.
}
The input parameters must be provided in between the brackets that come after the function's name.
The input parameters will make the function accept different values when we call the function and the result will be always dynamic.
Now, making the custom function accept input parameters is only 50% of the job.
To finish our job, we should utilize those input parameters inside the function's body to let it perform an operation in a dynamic way.
function addTwoNumbers(firstNumber, secondNumber){
const result = firstNumber + secondNumber;
console.log(result);
}
For example, in the above code, we have changed the function definition of addTwoNumbers
to accept two input parameters: firstNumber
and secondNumber
.
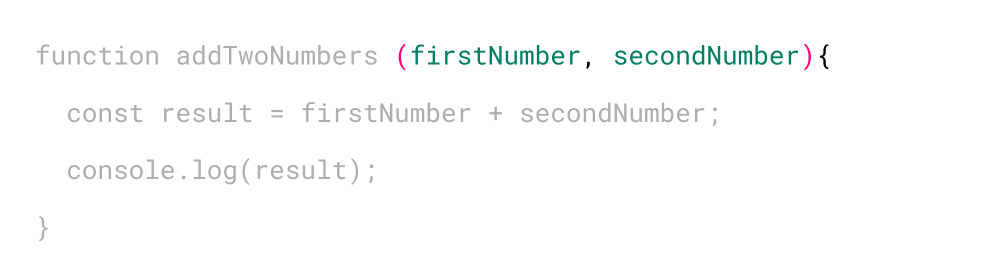
And we are utilizing those input parameters inside the function body to perform an operation (the SUM operation in the case of addTwoNumbers
function):
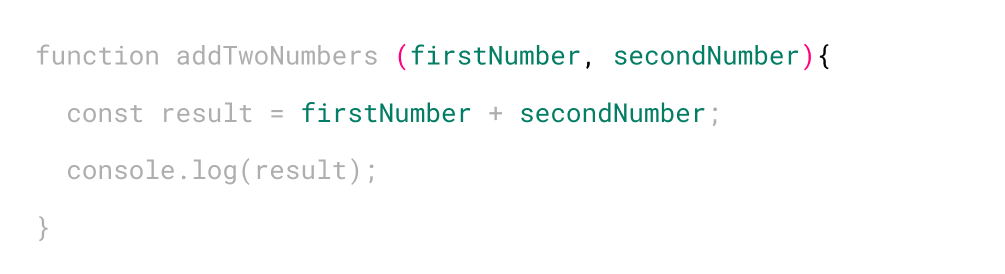
If you notice, instead of hard-coded values, we are using placeholder variables to accept input parameters and performing an operation on those placeholder variables.
Every time the custom function is executed, these placeholder variables represent real values that we will supply.
These placeholder variables make the custom function dynamic.
Because of this, we can call the addTwoNumbers
function and make it perform the sum of any two numbers we provide.
This is better practiced than explained.
Come on, go ahead and put the below code inside the browser console and see the result for yourself:
function addTwoNumbers(firstNumber, secondNumber){
const result = firstNumber + secondNumber;
console.log(result);
}
addTwoNumbers(5, 10); //Outputs 15
addTwoNumbers(29, 6); //Outputs 35
addTwoNumbers(100, 200); //Outputs 300
Here is how the above code works:
1) When the Javascript engine sees the above code, it first looks at the addTwoNumbers
function definition.
But the Javascript engine doesn't execute it because it is just a function definition and not a function call.
So, it skips to the next line of code:
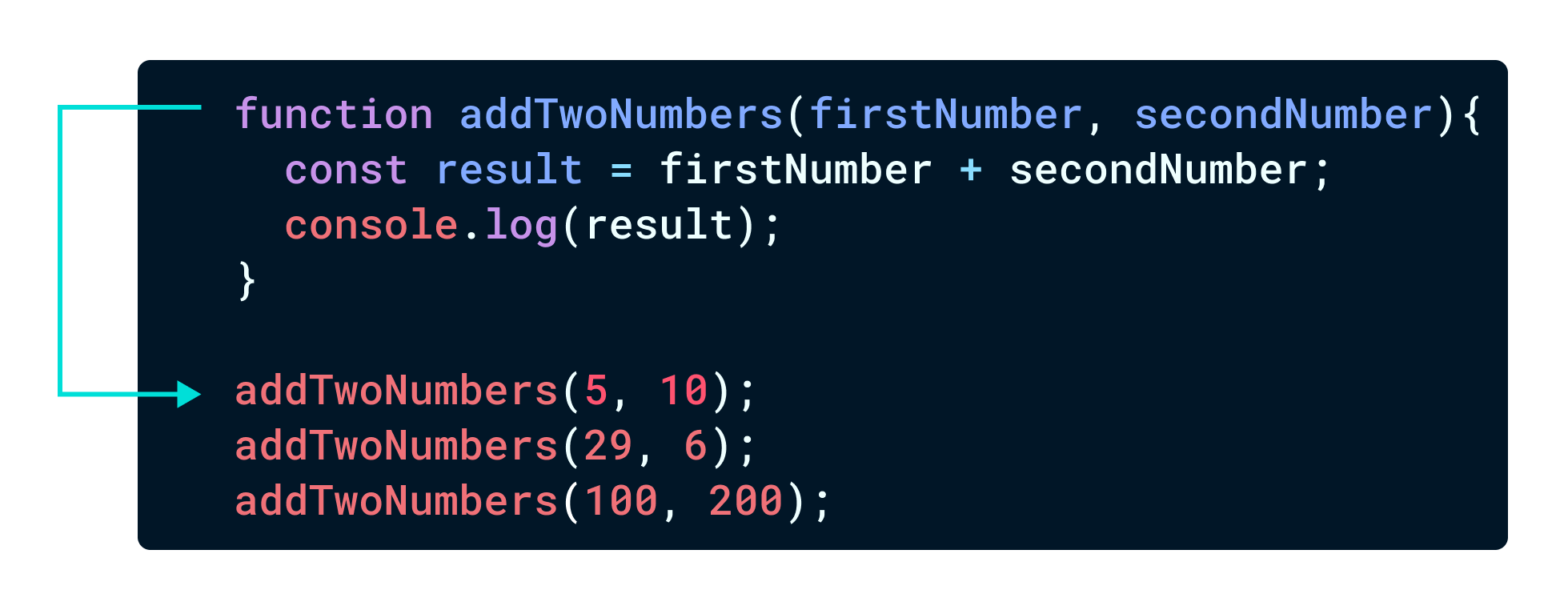
2) Next, it sees the first call to the addTwoNumbers
function and prepares itself to execute the code inside the addTwoNumbers
function definition.
3) During the preparation, it realizes that we are calling the addTwoNumbers
function by passing it two arguments: 5, 10
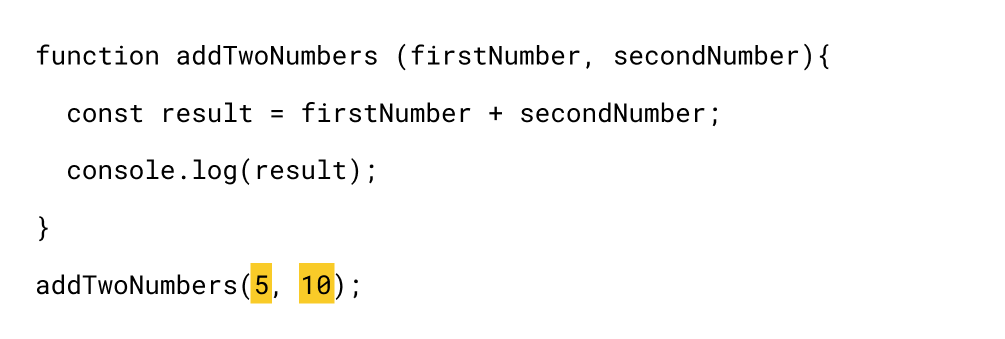
4) So, before executing the code inside the function body, these arguments will be mapped to the input parameter variables from the function definition:
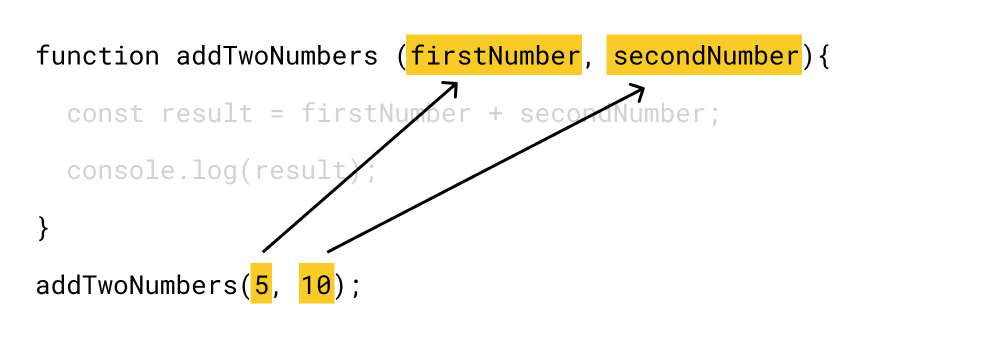
5) The arguments will be mapped to input parameters in sequential order, no matter what the variable names are:
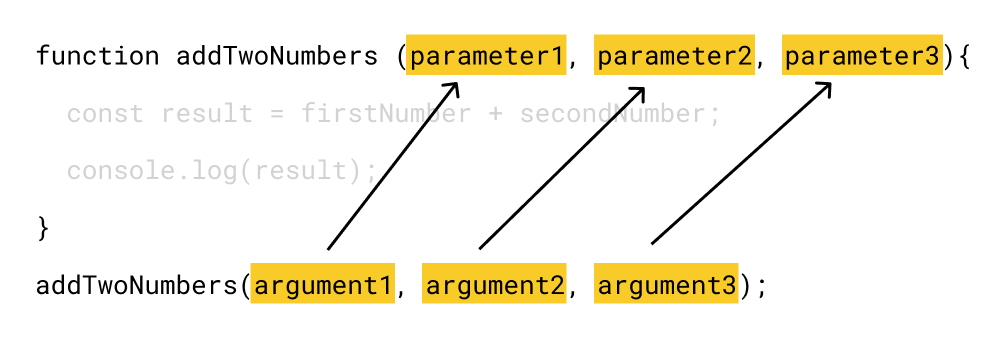
So, in our case:
A) The first argument 5
is mapped to the firstNumber
placeholder variable
B) The second argument 10
is mapped to the secondNumber
placeholder variable
Anyway, after the mapping is done, the firstNumber
variable contains the value 5
and the secondNumber
variable contains 10
:
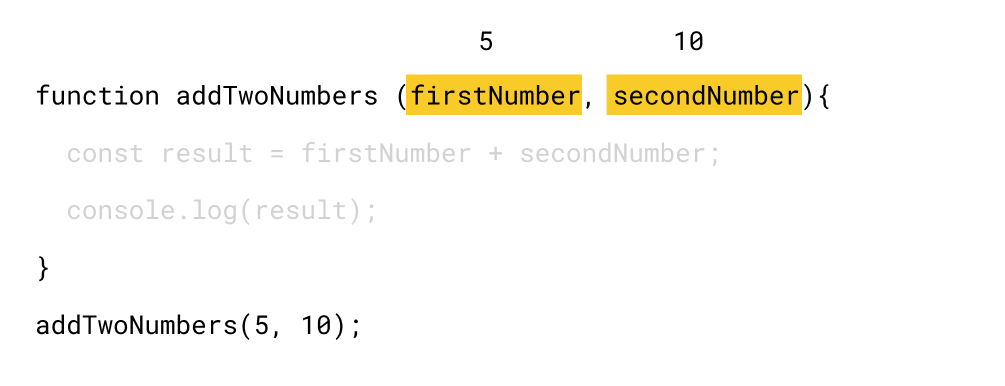
6) Next, the code inside the function body gets executed line by line:
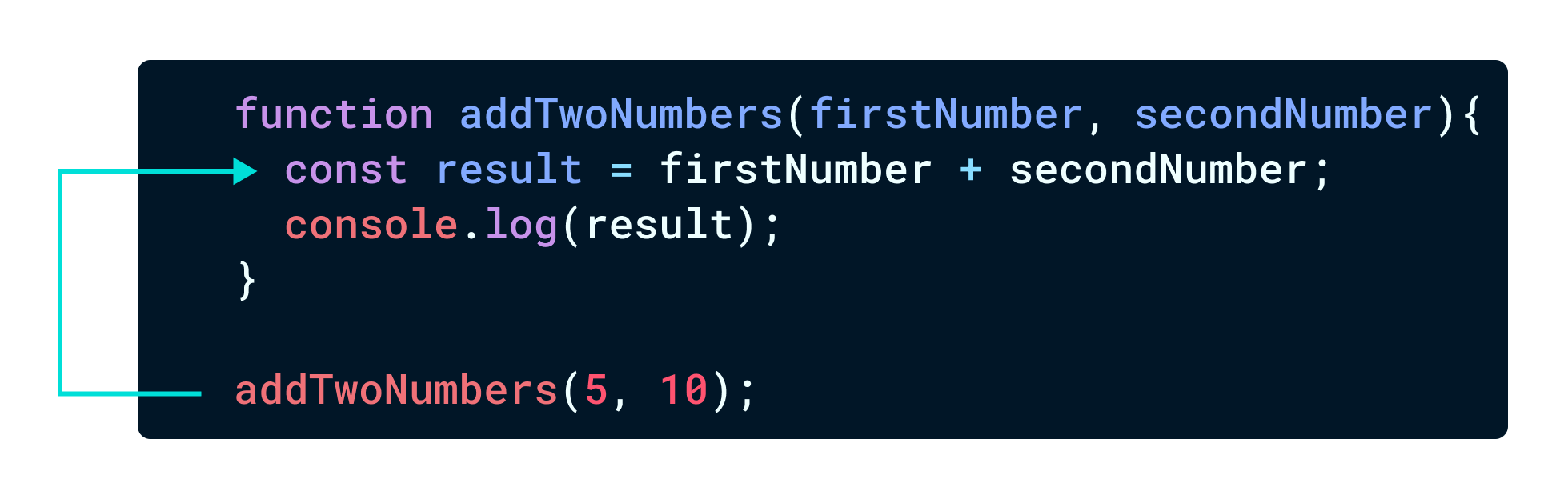
7) The first line inside the function body is adding the two numbers by utilizing the input parameter variable names:
const result = firstNumber + secondNumber;
Nothing fancy is happening here.
We are adding the value stored inside the firstNumber
(5) with the secondNumber
(10) and storing the calculation result 15
inside the result
variable.
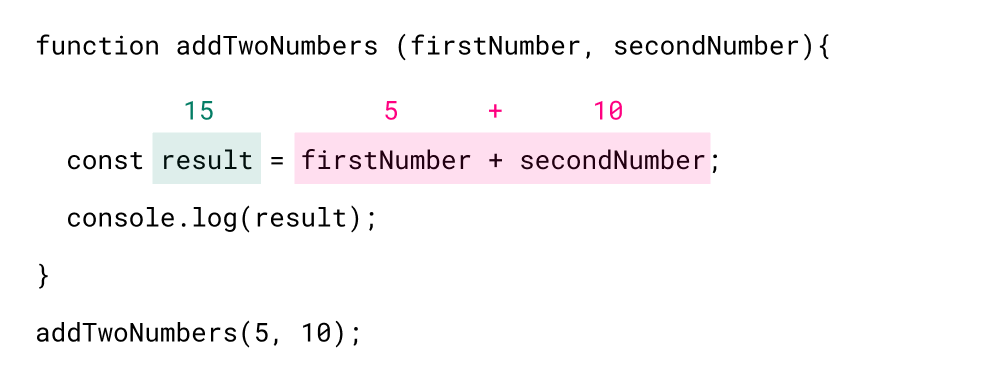
8) Finally, we are logging the result
(15) to the browser console:
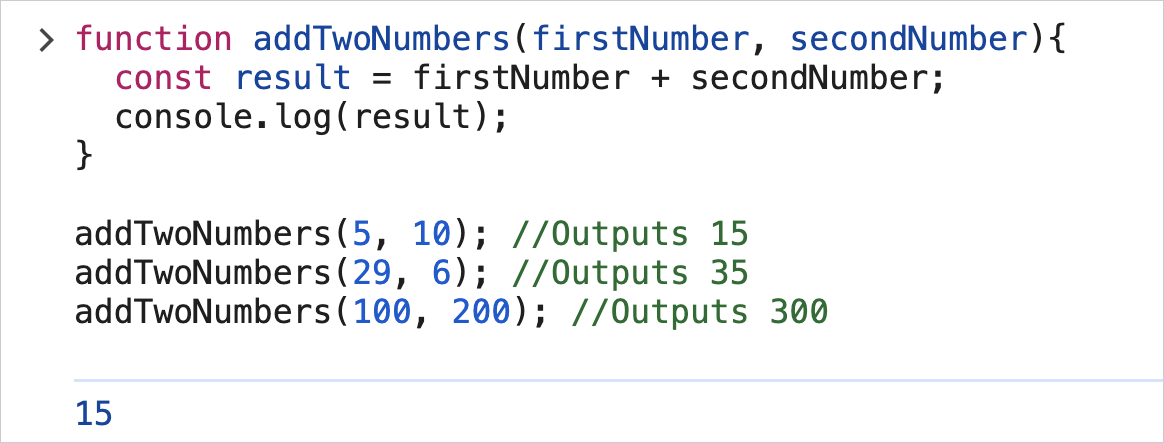
9) After logging the result, there is no more code to execute inside the addTwoNumbers
function, so the Javascript engine will come out of the function body and see the next function call:
addTwoNumbers(29, 6);
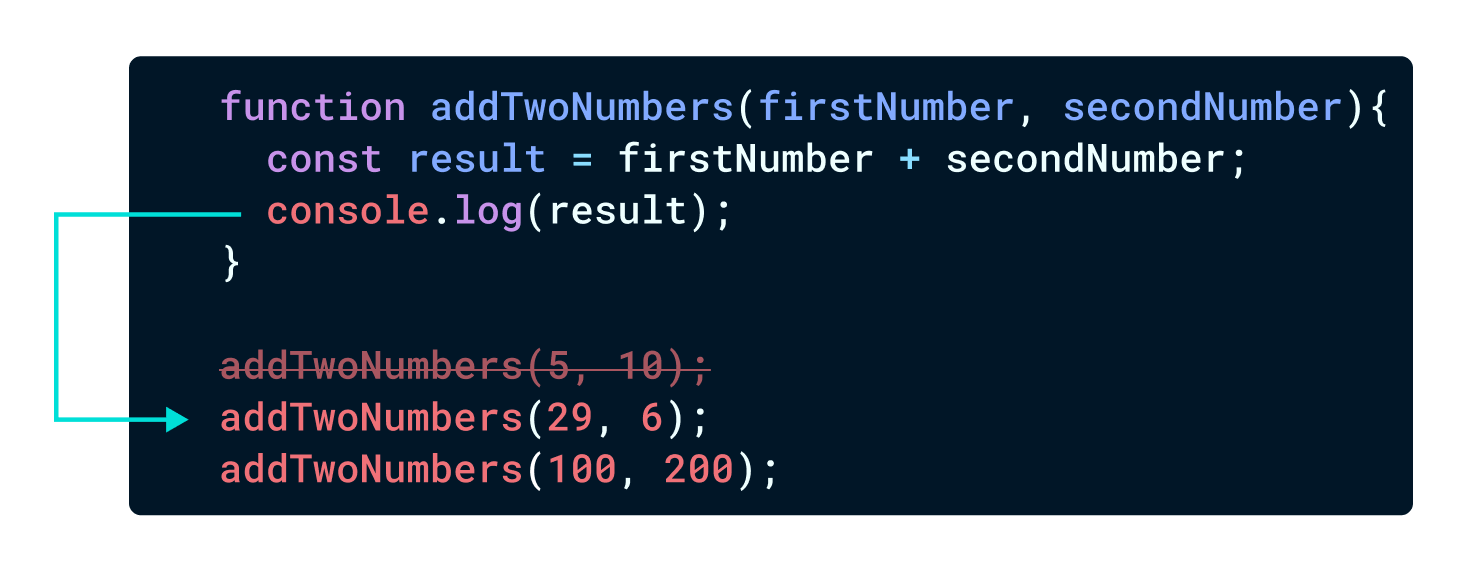
As usual, it sees the second call to the addTwoNumbers
function and prepares itself to execute the code inside the addTwoNumbers
function definition, again.
Simply put, the whole process repeats again.
10) Just to be on the same page, during the preparation, the Javascript engine realizes that we are calling the addTwoNumbers
function by passing it two arguments: 29, 6
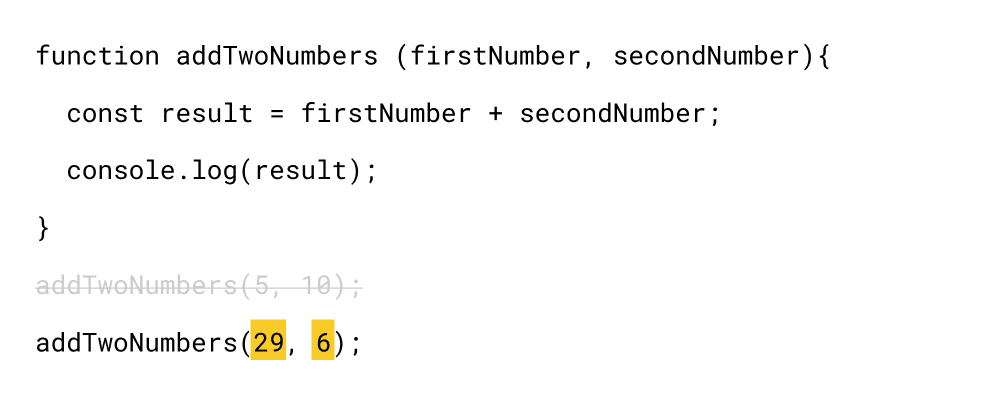
If you notice, this time, we are passing two totally different numbers from the previous time.
11) So, before executing the code inside the function body, these arguments will be mapped to the input parameter variables from the function definition:
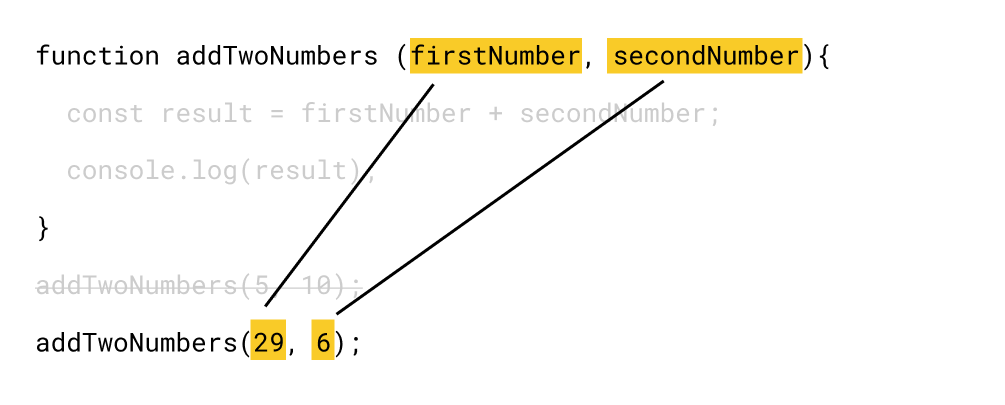
12) The arguments will be mapped to input parameters in sequential order, no matter what the variable names are:
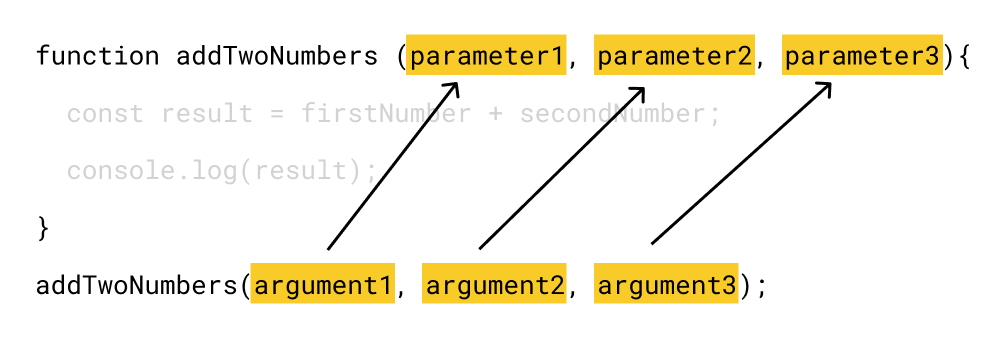
So, in our case:
A) The first argument 29
is mapped to the firstNumber
placeholder variable
B) The second argument 6
is mapped to the secondNumber
placeholder variable
Anyway, after the mapping is done, the firstNumber
variable contains the value 29
and the secondNumber
variable contains 6
:
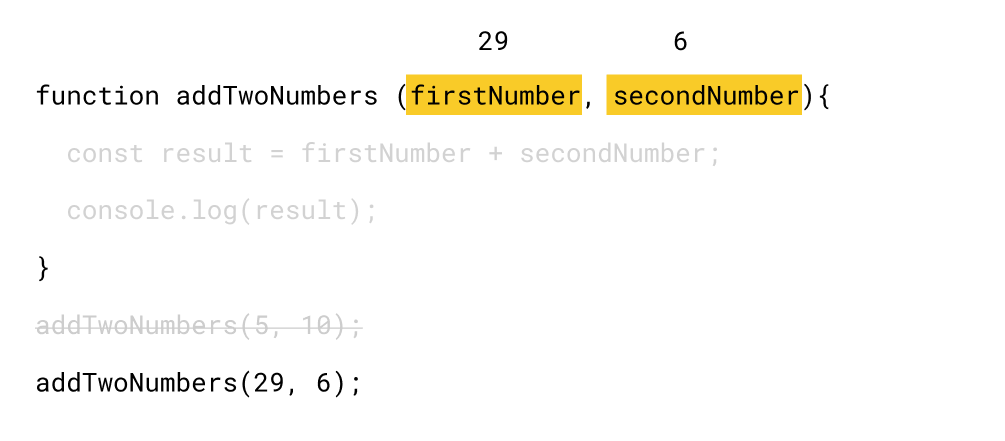
They change every time based on the input arguments we supply, which makes the function reusable for various calculations.
13) Next, the code inside the function body gets executed line by line:
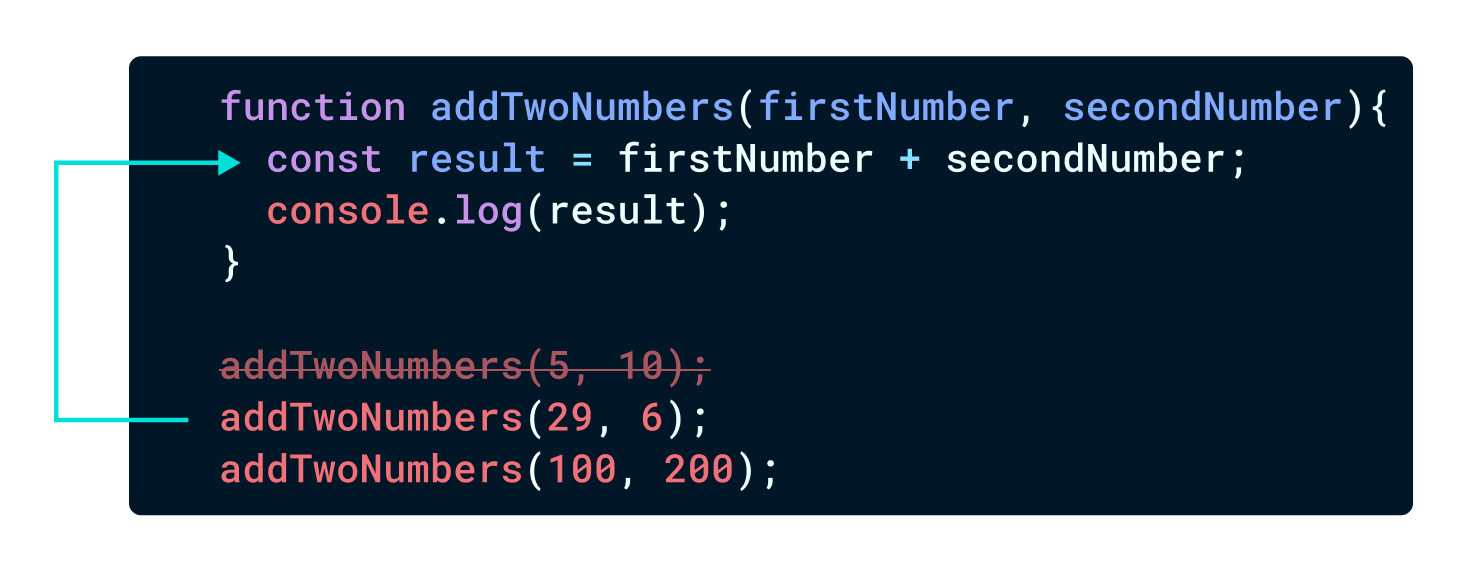
14) The first line inside the function body is adding the two numbers by utilizing the input parameter variable names:
const result = firstNumber + secondNumber;
Nothing fancy is happening here.
We are adding the value stored inside the firstNumber
(29) with the secondNumber
(6) and storing the calculation result 25
inside the result
variable.
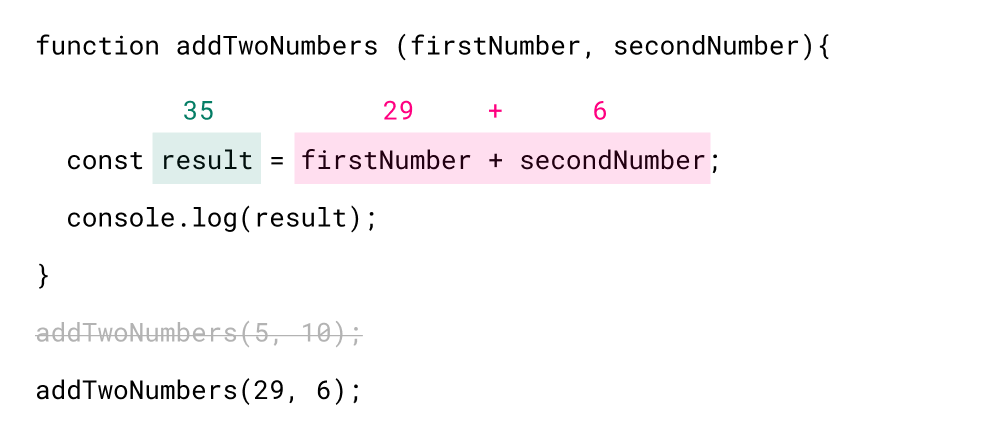
15) Finally, we are logging the result
(35) to the browser console:
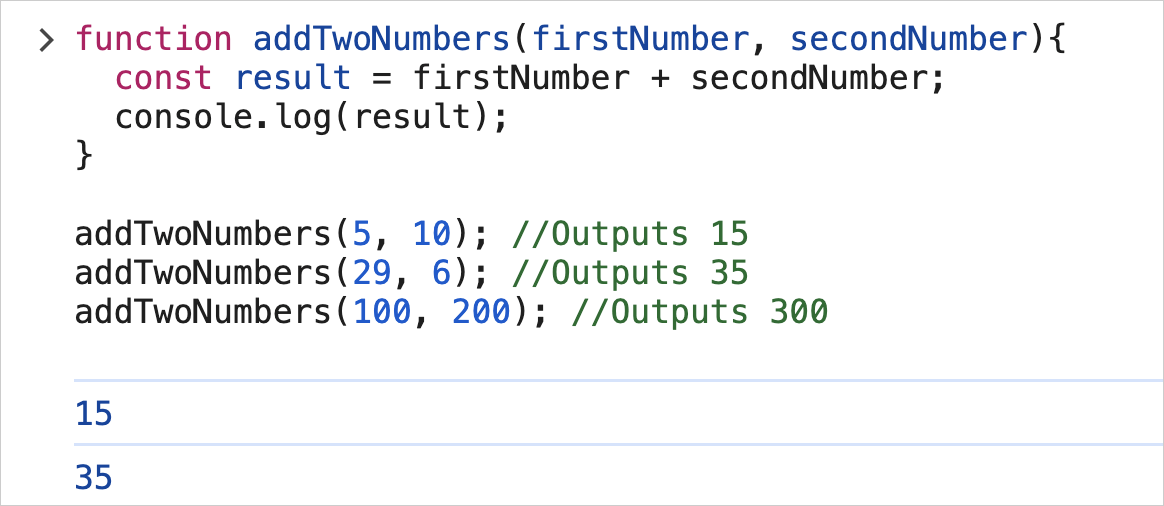
16) After logging the result, there is no more code to execute inside the addTwoNumbers
function, so the Javascript engine will come out of the function body and see the next function call (the last call):
addTwoNumbers(100, 200);
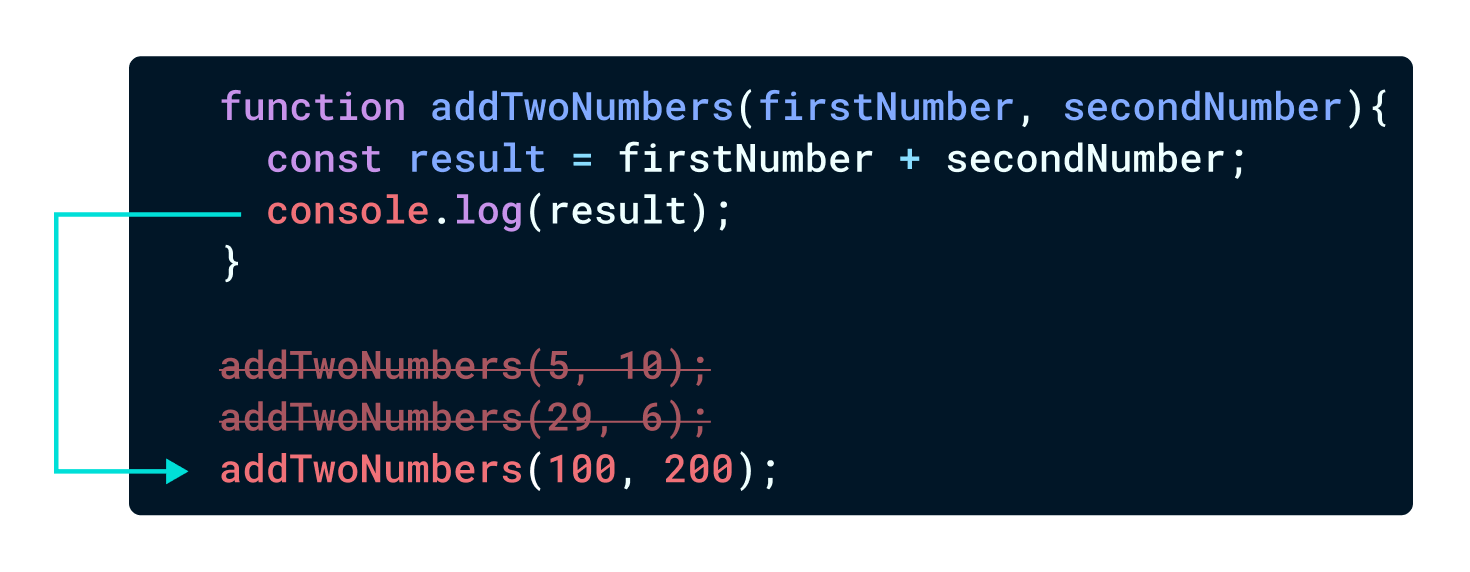
17) And the whole process repeats again, resulting in the final output of 300 to the browser console:
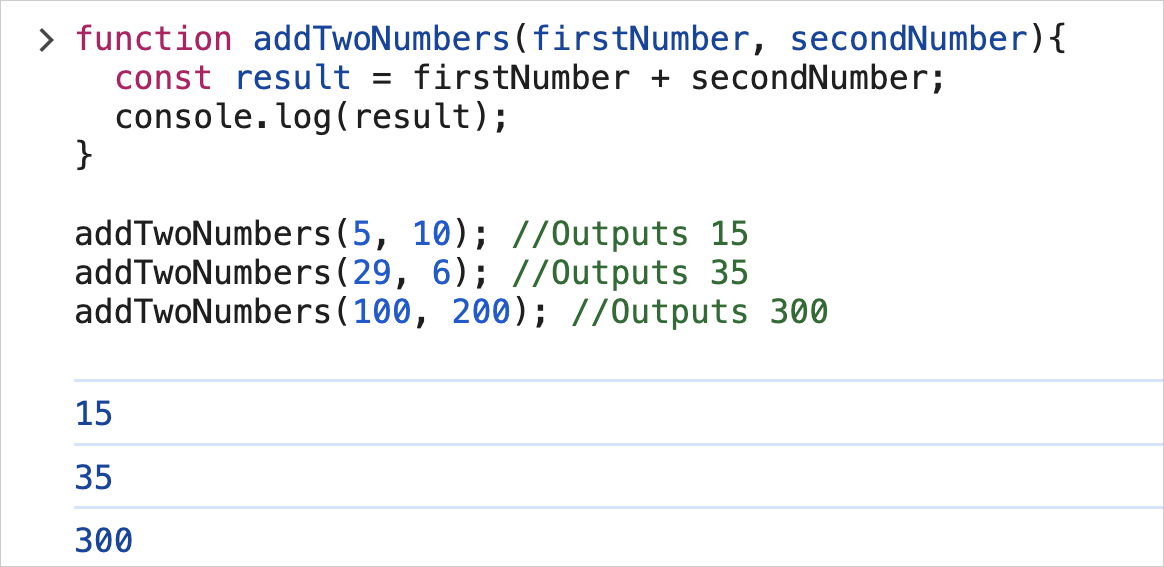
That's all.
That's how custom functions with input parameters work.
We will work on a practical custom function when working on the "Percentage Calculator" project.
But before that, I want you to understand how the passing of parameters works for callback functions.