Checking for multiple conditions
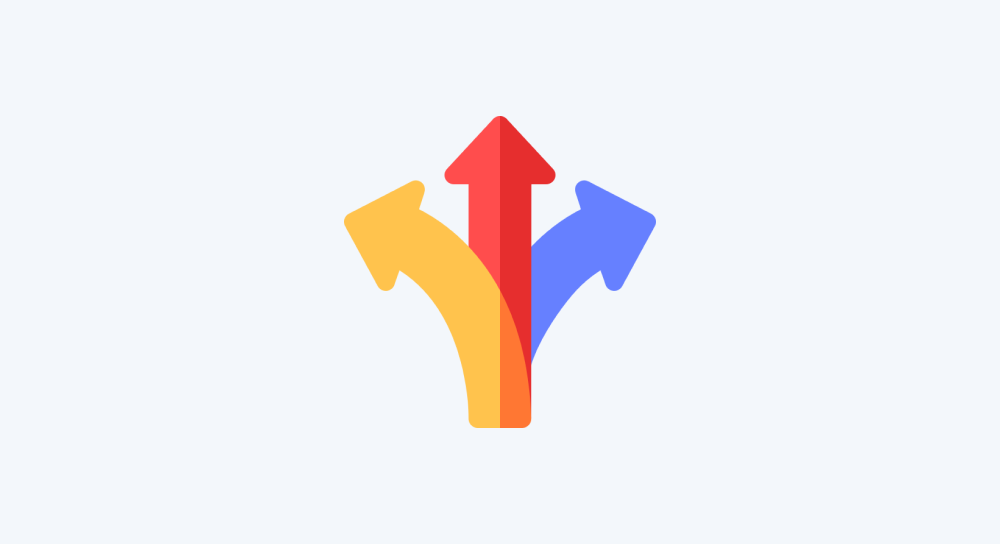
During the last couple of exercises, we explored the scenarios where we checked for a single condition and then made a decision based on it.
if(condition_is_true){
//Code to execute if the condition is true
} else {
//Code to execute if the condition is false
}
For example, in the Show/Hide password project, we checked whether the "Show password" field was turned on. And then:
- If the "Show password" field is turned on, we reveal the password.
- Or else we concealed the password.
if(showPasswordField.checked){
//If it is checked, show the password
} else {
//Hide password
}
Similarly, in the Subscribe / Unsubscribe exercise, when the user clicked on the Subscribe button, we checked the current text of the button. And then:
- If the current button text was "Subscribe", we changed it to "Unsubscribe".
- Or else we changed it to "Subscribe".
if(buttonText === "Subscribe"){
//Change it to "Unsubscribe"
} else {
//Change it back to "Subscribe"
}
But what if you want to check for multiple conditions before making a decision?
For example, let's say you run a website where you must show a recipe based on the time available to cook.
- If the time available is 60 minutes, you want to show the "Grilled Chicken" recipe.
- Else, if the time available is less than 30 minutes, you want to show the "Vegetable Stir Fry" recipe.
- Else, if the available time is less than 15 minutes, you want to show the "Quick Salad" recipe.
- Else, if we don't know the time available, then show all the above recipes.
How do we achieve this?
This is where the "Else If" statement comes in.
What is an "Else If" Statement?
The "Else If" statement is used as an extension to the "If" statement when we want to check multiple conditions sequentially.
Here is the syntax of the "If/Else" statement combined with the "Else If" statement:
if (condition_1){
// Code to execute if condition_1 is true
} else if (condition_2){
// Code to execute if condition_1 is false and condition_2 is true
} else {
// code to execute if all the above conditions are false
}
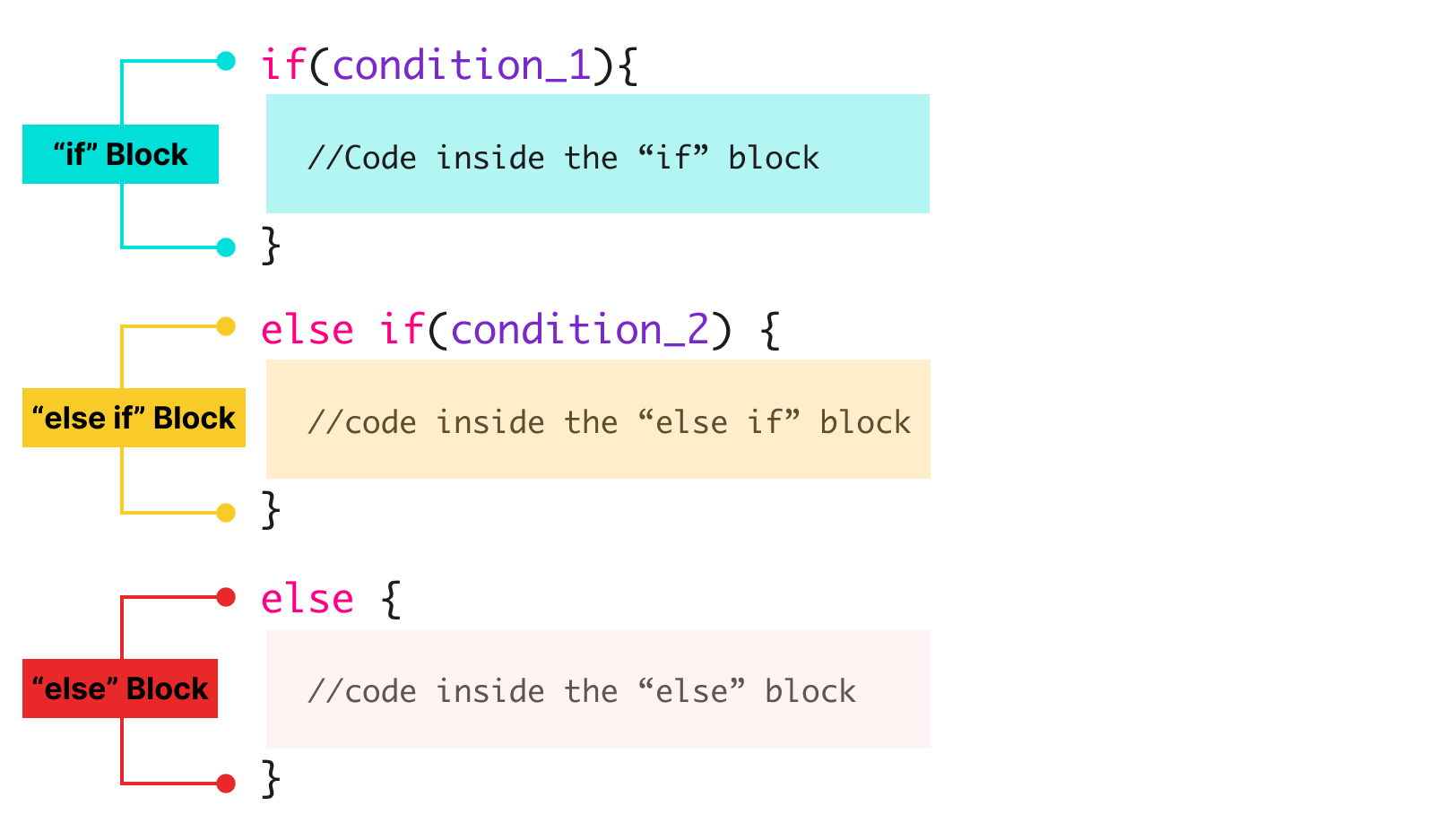
If you notice the above syntax, we are checking for two conditions subsequently:
- Inside the
if
statement - Inside the
else if
statement
And only if both of them are false, we are resorting to the else
statement and executing the code inside it.
Here is what's happening with the flow of the code:
1) As usual, we are first checking if the condition_1
is true. If it is true, then the Javascript engine will ignore the "else if" and "else" statements altogether:
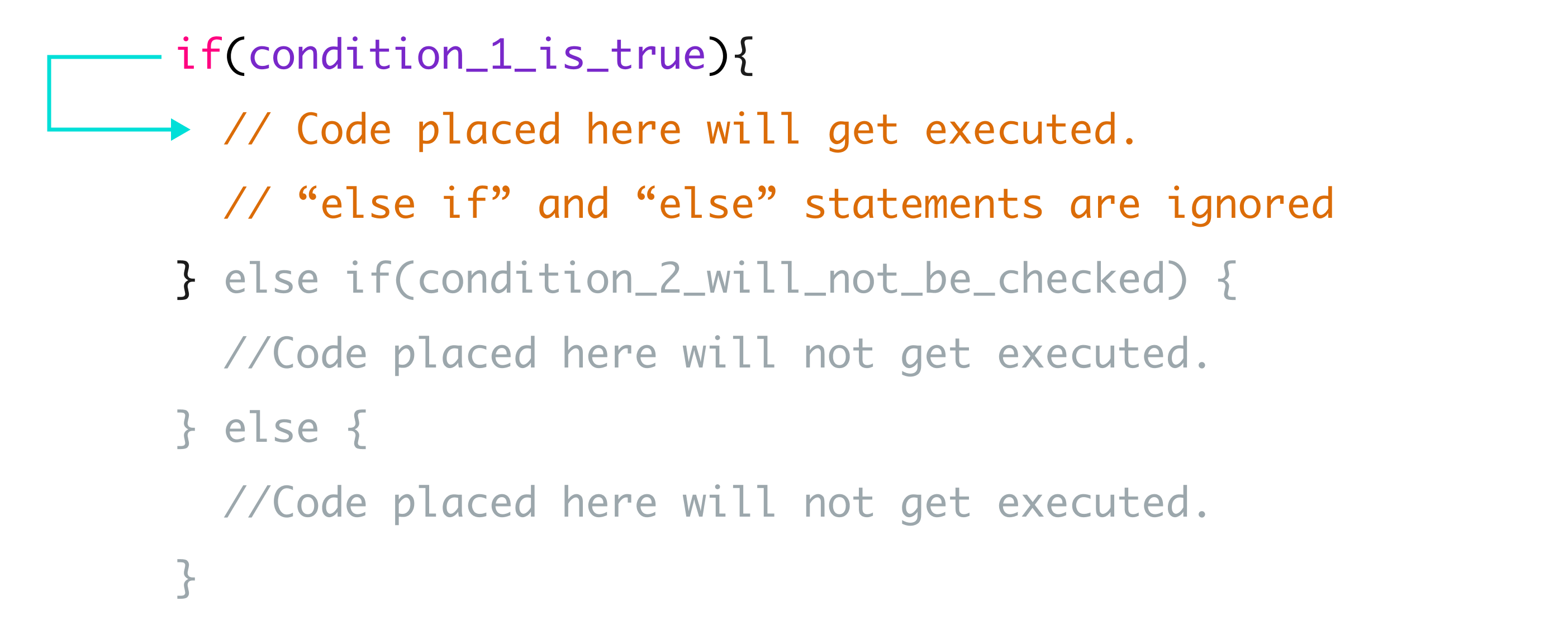
This also means that the condition_2
will not be checked at all.
2) But if the condition_1
is false, then instead of executing the code inside the "else" block directly, this time condition_2
will be checked whether it is true or not:
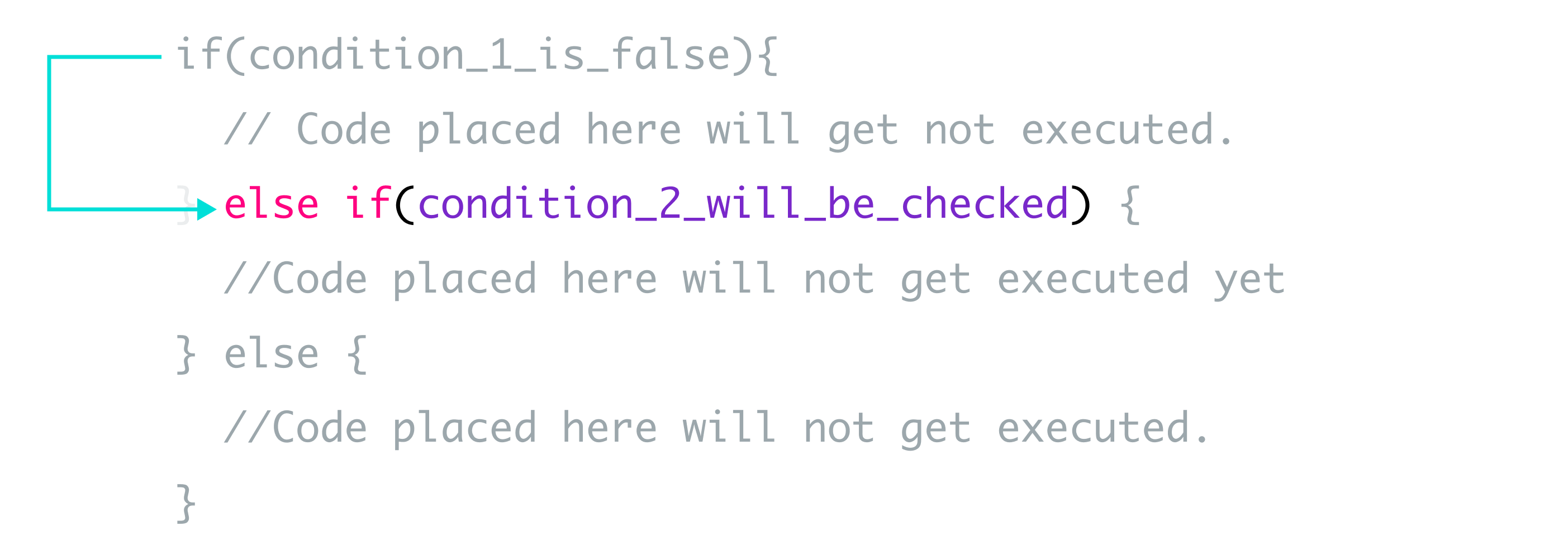
If the condition_2
is true, then the code inside the else if
block is executed:
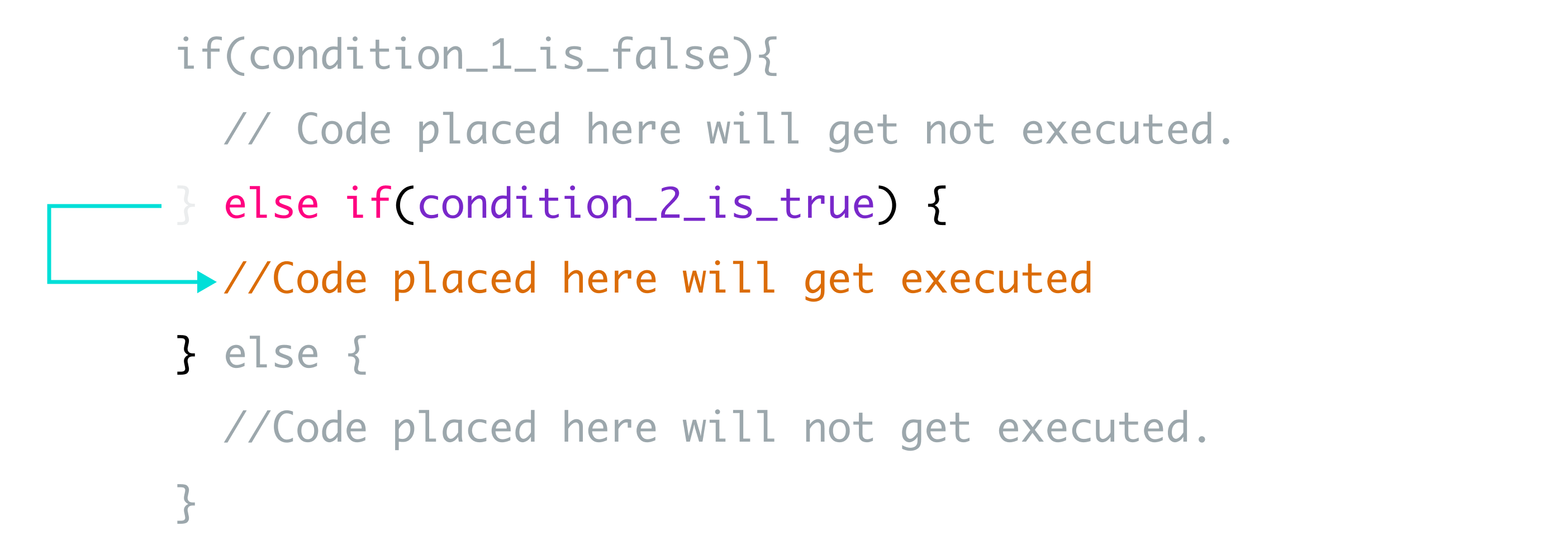
3) But if the condition_2
is also false, then the code inside the else
block will get executed automatically:
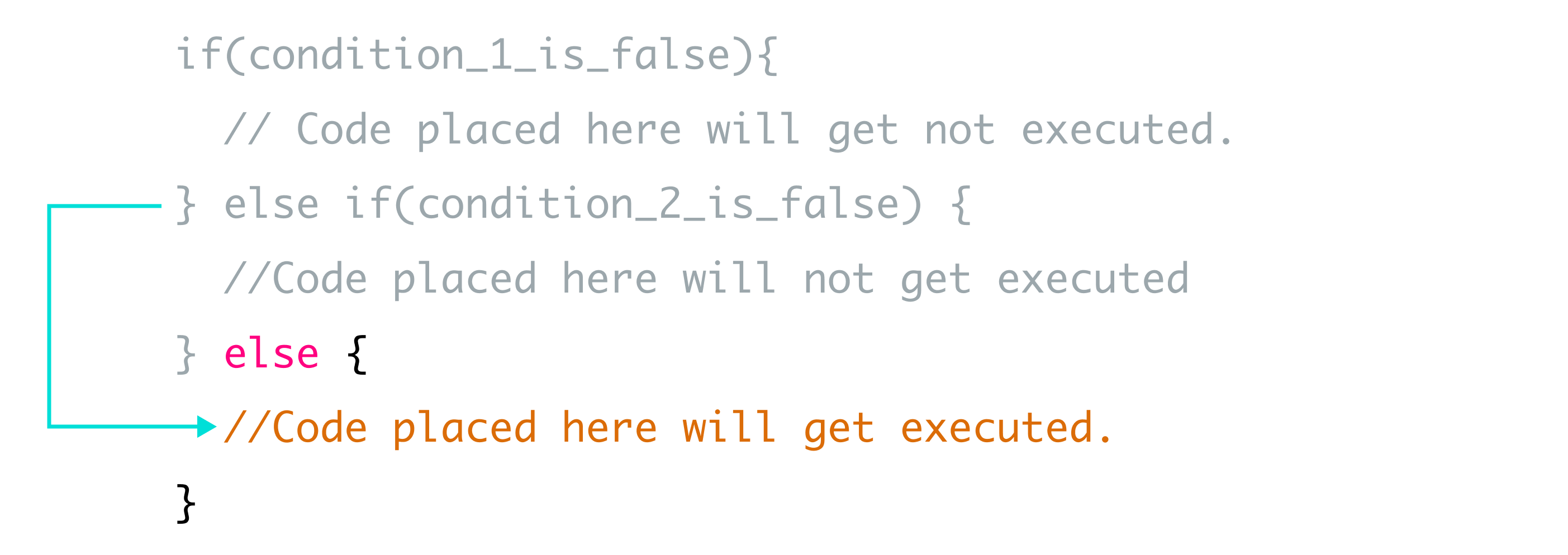
Simply put, the else
block will get executed only if all the other conditions are false.
Easy, right?
You can have an unlimited number of "else if" statements
That means you can check for any number of subsequent conditions after the "if" statement:
if(condition_1){
// Code to execute if condition_1 is true
} else if(condition_2){
// Code to execute if condition_1 is false and condition_2 is true
} else if(condition_3) {
// Code to execute if condition_1 and condition_2 are false and condition_3 is true
} else if(condition_4){
// Code to execute if condition_1, condition_2, and condition_3 are false and condition_4 is true
} else {
// Code to execute if condition_1, condition_2, condition_3, and condition_4 are false
}
Read the comments to understand what's happening.
Nice, right?
This will give us the ultimate control over the decisions our code can make and write more robust components.
In the next lesson, we will take a look at a couple of practical examples for using the "Else If" statement.