Anonymous functions 101
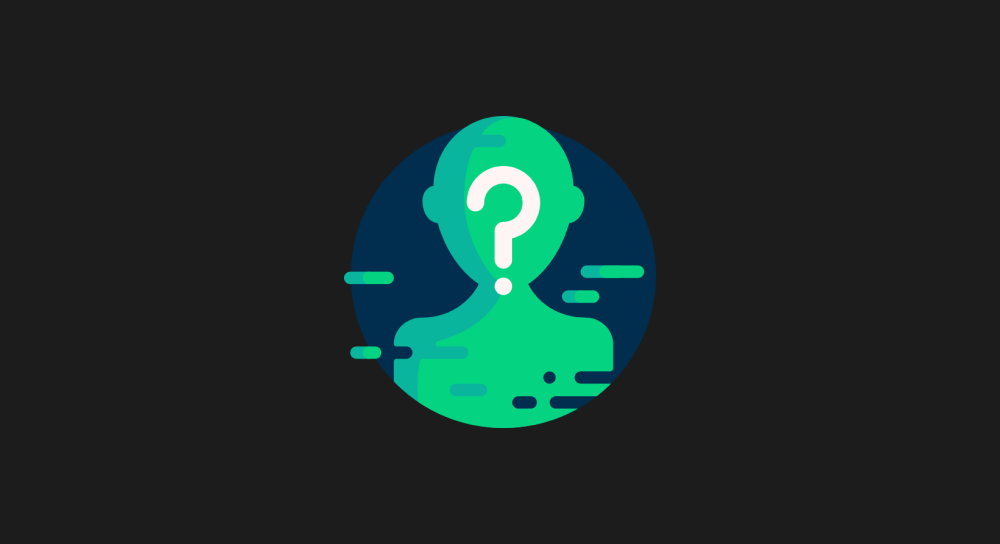
There are two types of functions in Javascript:
- Custom-defined functions - such as,
showModal
,hideModal
, etc. - In-built functions - such as,
alert
,prompt
,print
, etc.
And within custom-defined functions, there are three types:
- Named functions
- Anonymous functions
- Arrow functions
We already know how to define a named function:
function showModal(){
let discountModal = document.querySelector("#surprise-discount-modal");
discountModal.style.display = "block";
}
Named function definition
So, in this lesson, we will learn about Anonymous functions and their role in writing clean javascript code.
So, what is an anonymous function?
It is nothing but a function definition with no name.
For example:

If you notice, we didn't provide a name while defining the function.
There is no way to call it directly
And because an anonymous function doesn't have a name, "we" can not call it directly.
For example, let's just say we have a named function definition called, showModal
:
function showModal(){
let discountModal = document.querySelector("#surprise-discount-modal");
discountModal.style.display = "block";
}
Because it has a name, "we" can directly call it:
showModal();
But if we transform the showModal
function into an anonymous function:
function(){
let discountModal = document.querySelector("#surprise-discount-modal");
discountModal.style.display = "block";
}
There is no way we can call it directly because it doesn't have a name.
Having said that, everything else is the same:
- Anonymous functions can accept input parameters
- They can return a value
- And they are the same as named functions in all other aspects
To summarize
There are two differences between a named function and an anonymous function:
- An anonymous function can not have a name
- So, we can not call it directly.
But what is the use of anonymous functions if they can not be called directly?
Well...
We usually create anonymous functions to use as event handlers
If you remember, we don't call an event handler directly.
The browser will call it for you when an associated event is generated.
For example, here is the code we have written for showing the modal when a button is clicked:
function showModal(){
let discountModal = document.querySelector("#surprise-discount-modal");
discountModal.style.display = "block";
}
let showModalButton = document.querySelector(".show-modal-btn");
showModalButton.addEventListener("click", showModal);
Here, we provided the showModal
function as the event handler.
But we are not calling the showModal
function directly, right?
The browser is calling it for us when the button is clicked.
So, event handlers are the perfect use case for using anonymous functions in javascript because the browser can still call them when needed.
How to use an anonymous function as an event handler?
I would recommend following with me to grasp this concept easily.
So, open up the "Project: Discount Modal" project in your code editor.
Anyway, so far, while working with event listeners, we have only provided the name of the function as the event handler and declared the function separately:
function showModal(){
let discountModal = document.querySelector("#surprise-discount-modal");
discountModal.style.display = "block";
}
let showModalButton = document.querySelector(".show-modal-btn");
showModalButton.addEventListener("click", showModal);
Here, we are just providing the showModal
name as a reference to the actual function definition.
But, for an event handler, you can also provide the function definition directly:
showModalButton.addEventListener("click", function showModal(){
let discountModal = document.querySelector("#surprise-discount-modal");
discountModal.style.display = "block";
});
In the above code, we replaced the name showModal
with the actual function definition directly.
And this works just fine too.
Come on, test it out.
The modal functionality should still work just fine.
Now comes the interesting question.
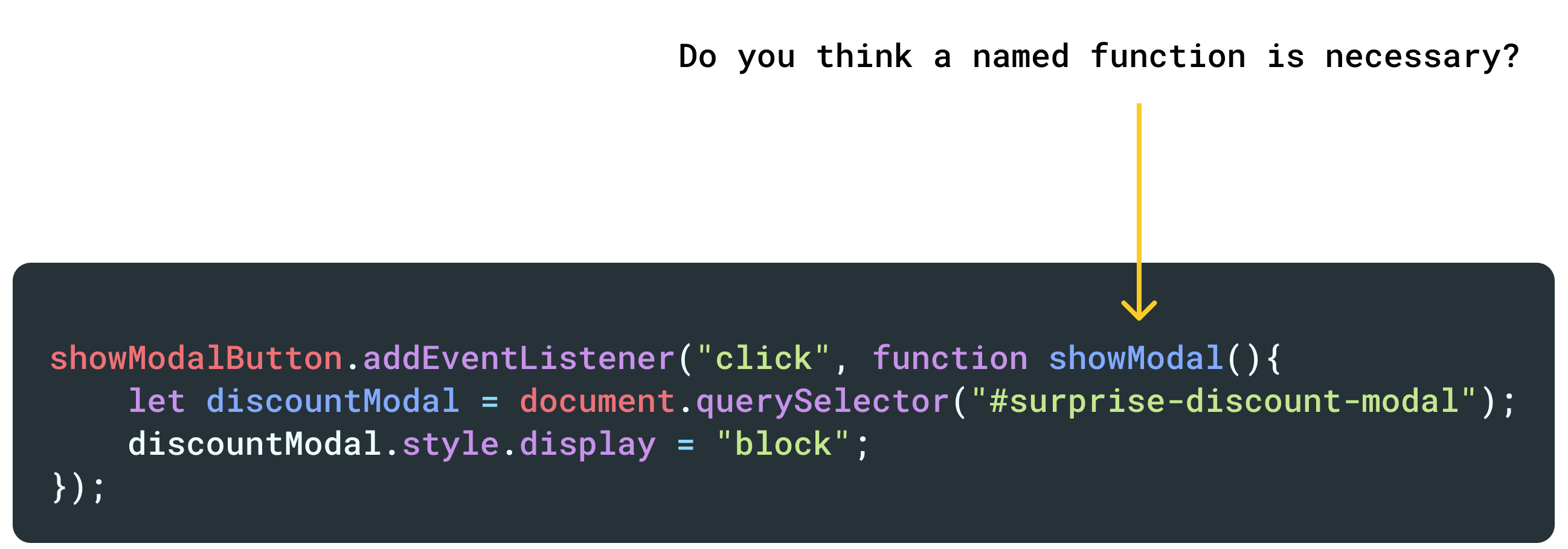
Because we are not calling the showModal
function directly and the browser calls it for us automatically, do you think it is necessary to use a named function for the event handler?
The answer is a big fat NO.
We can just use an anonymous function instead:
showModalButton.addEventListener("click", function(){
let discountModal = document.querySelector("#surprise-discount-modal");
discountModal.style.display = "block";
});
And the functionality should still work just fine.
The comparison
Now tell me something.
This is the code for implementing the modal functionality and it is using the named functions for event handlers:
/* Functionality for revealing the modal */
function showModal(){
let discountModal = document.querySelector("#surprise-discount-modal");
discountModal.style.display = "block";
}
let showModalButton = document.querySelector(".show-modal-btn");
showModalButton.addEventListener("click", showModal);
/* Functionality for hiding the modal */
function hideModal(){
let discountModal = document.querySelector("#surprise-discount-modal");
discountModal.style.display = "none";
}
let hideModalButton = document.querySelector(".hide-modal-btn");
hideModalButton.addEventListener("click", hideModal);
Now, here is how the same implementation looks with anonymous functions:
/* Functionality for revealing the modal */
let showModalButton = document.querySelector(".show-modal-btn");
showModalButton.addEventListener("click", function(){
let discountModal = document.querySelector("#surprise-discount-modal");
discountModal.style.display = "block";
});
/* Functionality for hiding the modal */
let hideModalButton = document.querySelector(".hide-modal-btn");
hideModalButton.addEventListener("click", function(){
let discountModal = document.querySelector("#surprise-discount-modal");
discountModal.style.display = "none";
});
Which one is easier to understand and read?
If you ask me, I would say the version with anonymous functions because:
- It clearly tells me which function is getting called when the event is generated on a particular event listener.
- We don't have to think about using a function before defining it because we doing that on the fly.
- It keeps our code lean and extra neat.
Having said that, it is mostly a personal preference in my opinion.
So, if you feel that named functions are better for event handlers, feel free to use them. There is nothing wrong with it.
But from here onwards, I will be using anonymous functions a lot.
Anyway, that's all you need to know about anonymous functions for now.
In the next lesson, we will test your knowledge by performing a small exercise.