Objects can have methods
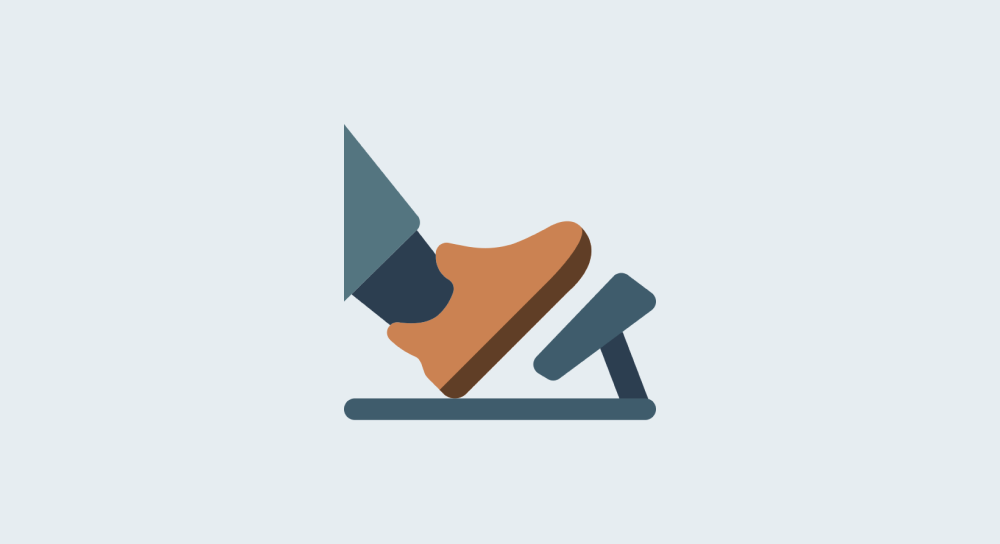
In a previous lesson, we learned that an object can have properties.
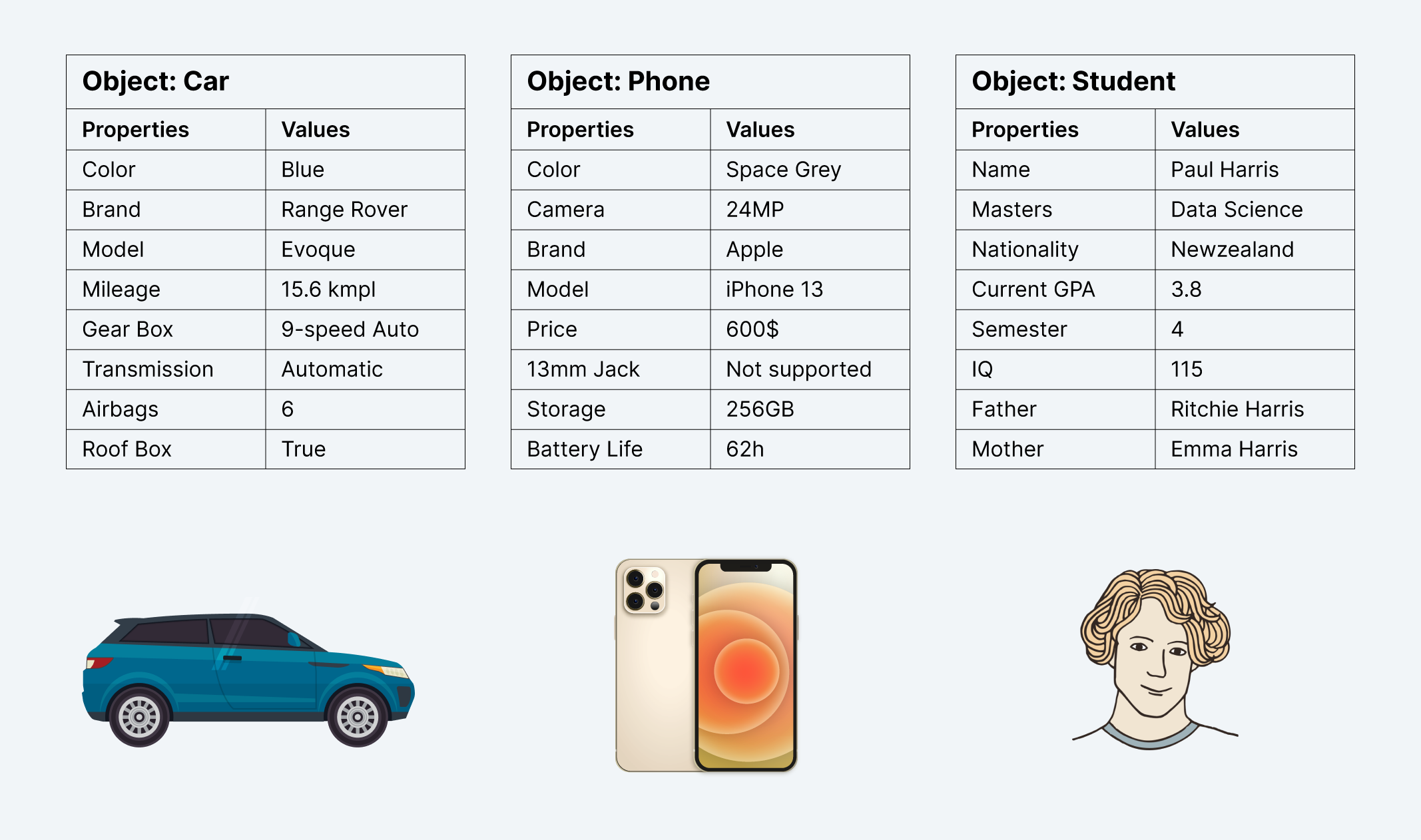
Similarly, objects can also have methods.
And methods are nothing but actions that can be performed on objects.
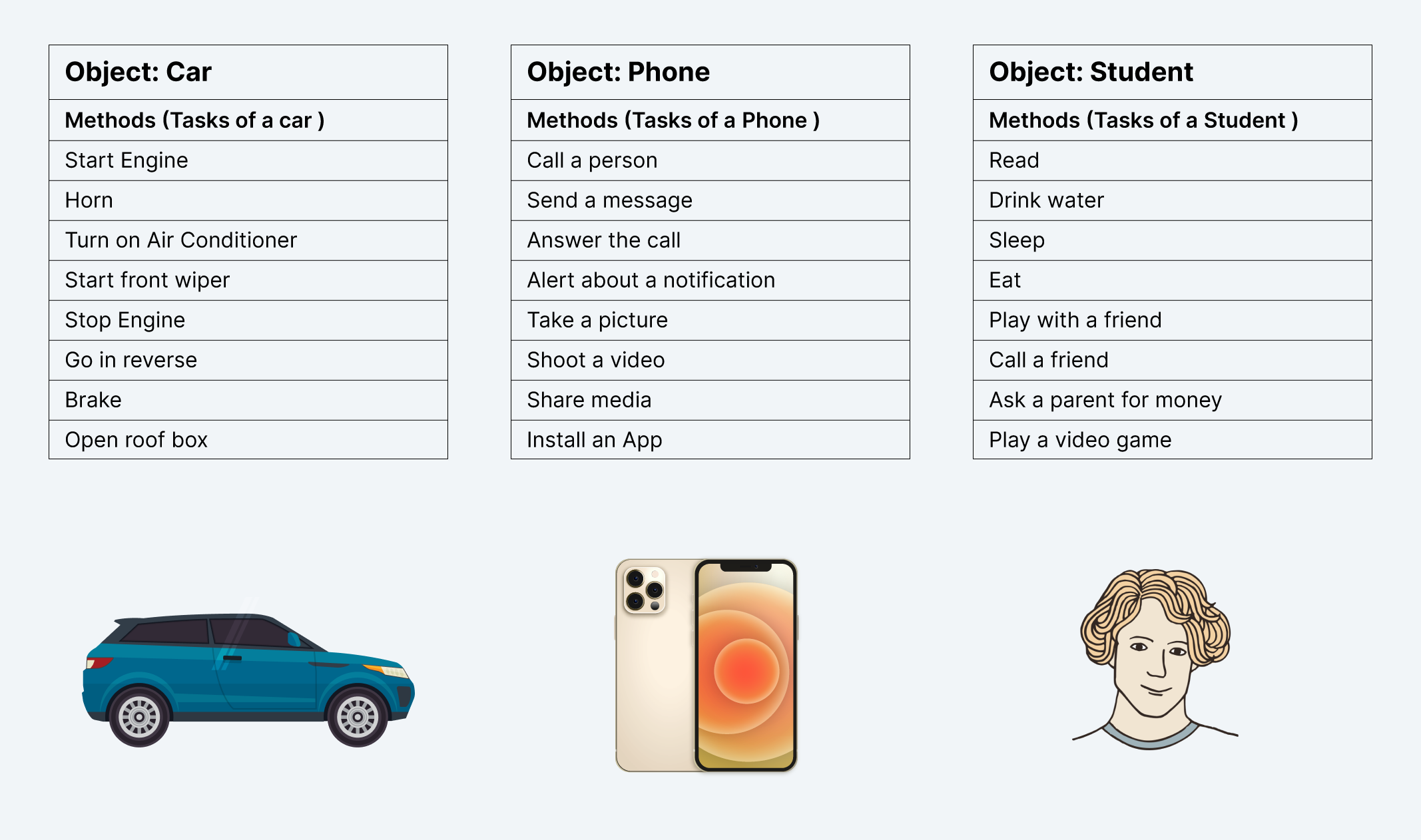
For example, if we take the car as an object, one of the actions we can perform on a car is starting its engine.
Similarly, one of the actions we can perform on a phone is calling someone.
One last example.
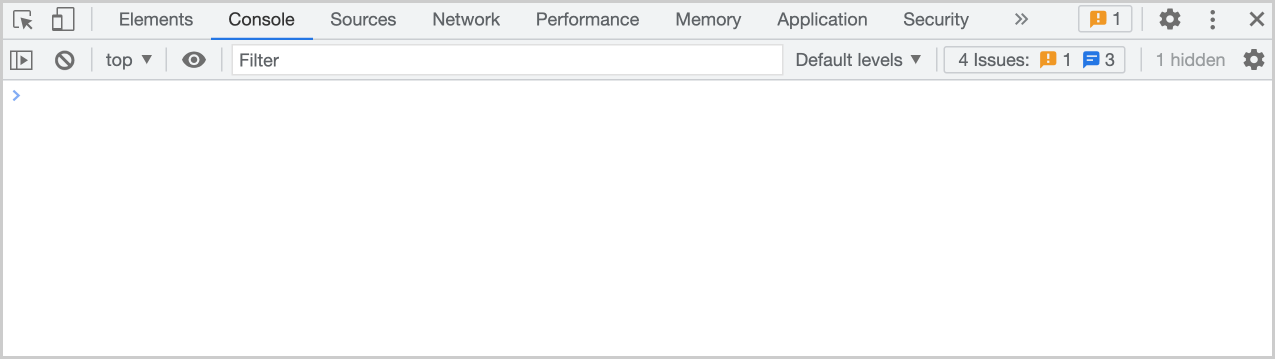
Let's imagine the Browser Console as an object.
One of the actions we can perform on the Console object is logging a message inside it for debugging purposes.
Actually, we don't have to imagine :D
The Browser Console is directly available to us as an in-built object in Javascript.
So, let's try to understand the concept of methods with the help of the Console object.
The Console object
We have been using the Console in the browser since the beginning of this course.
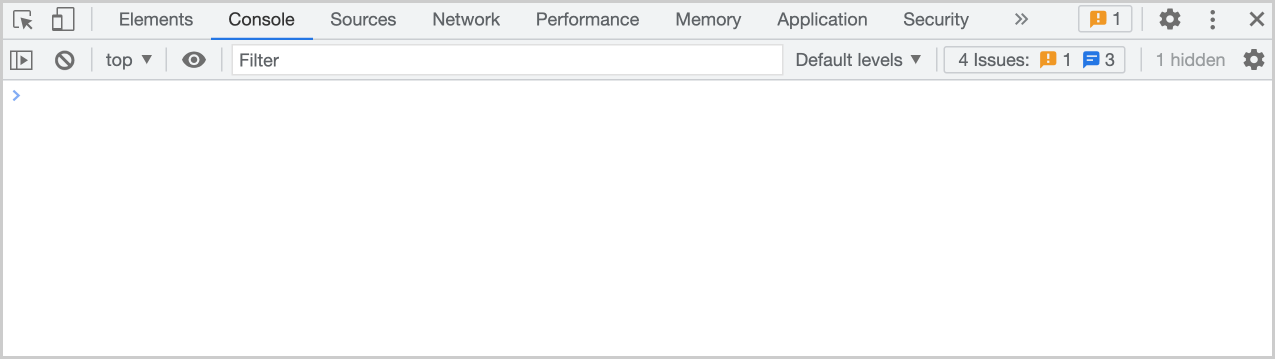
The good news is that Javascript's console
object will help us interact with the Browser Console through the code.
And the console
object comes packed with a lot of predefined methods.
So, we can use those methods to perform actions on the Browser Console.
Anyway, this is better shown than explained.
We can use the console
object in all three places where Javascript code can be executed:
- Inside the
<script>
tag of an HTML document. - Inside a file with
.js
extension. For example, themain.js
file. - Inside the Browser Console
This is fantastic because we can take our debugging to the next level.
For this lesson, let's use the Browser Console to try out the console
object.
So, open up the Browser Console and follow along with me.
Next, as I said before, one of the actions we can perform on the Console is logging a message inside it.
And for that, the console
object provides us with a log
method.
Now, let's see how to use the log
method.
So, how to use a method in Javascript?
A method is nothing but a property of an object.
In other words, the log
method is a property of the console
object.
So we can access the log
method using the Object dot notation:
console.log;

And bang! What are we seeing?
Don't worry.
What we are seeing is the function definition of log
method.
A function definition simply contains a set of instructions for performing the action.
For example, the function definition of the log
method contains instructions for logging a message inside the Browser Console.
But why are we seeing a function definition?
Normally, when we try to access a property of an object inside the Console, we will see the data stored inside that property:
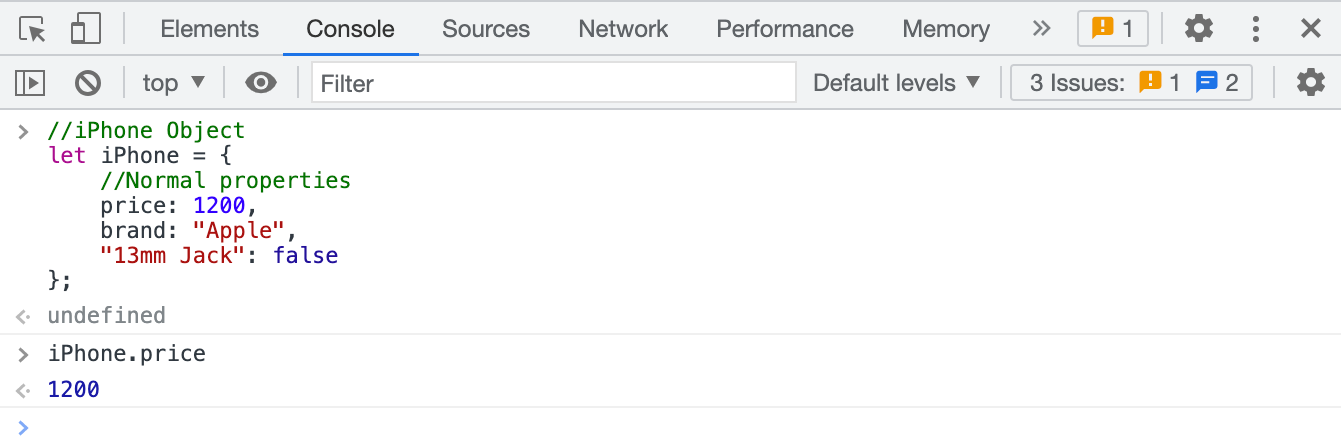
This tells us that the log
is not an ordinary property of the console
object.
Simply put, the log
method is storing a function
instead of a piece of data.

Don't try to understand the above code. It is not necessary as of now.
I am gonna cut to the chase.
Here is another secret about methods in Javascript.
Methods are functions.
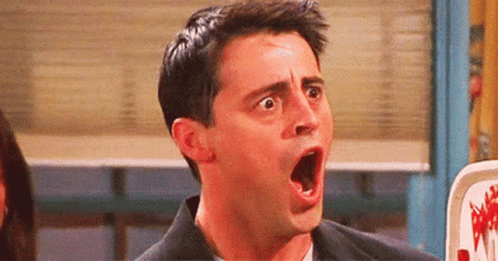
The log
method is nothing but a function like alert
, prompt
, etc.
So, we have to use the log
the method in the same way we use functions like alert
, prompt
, etc.
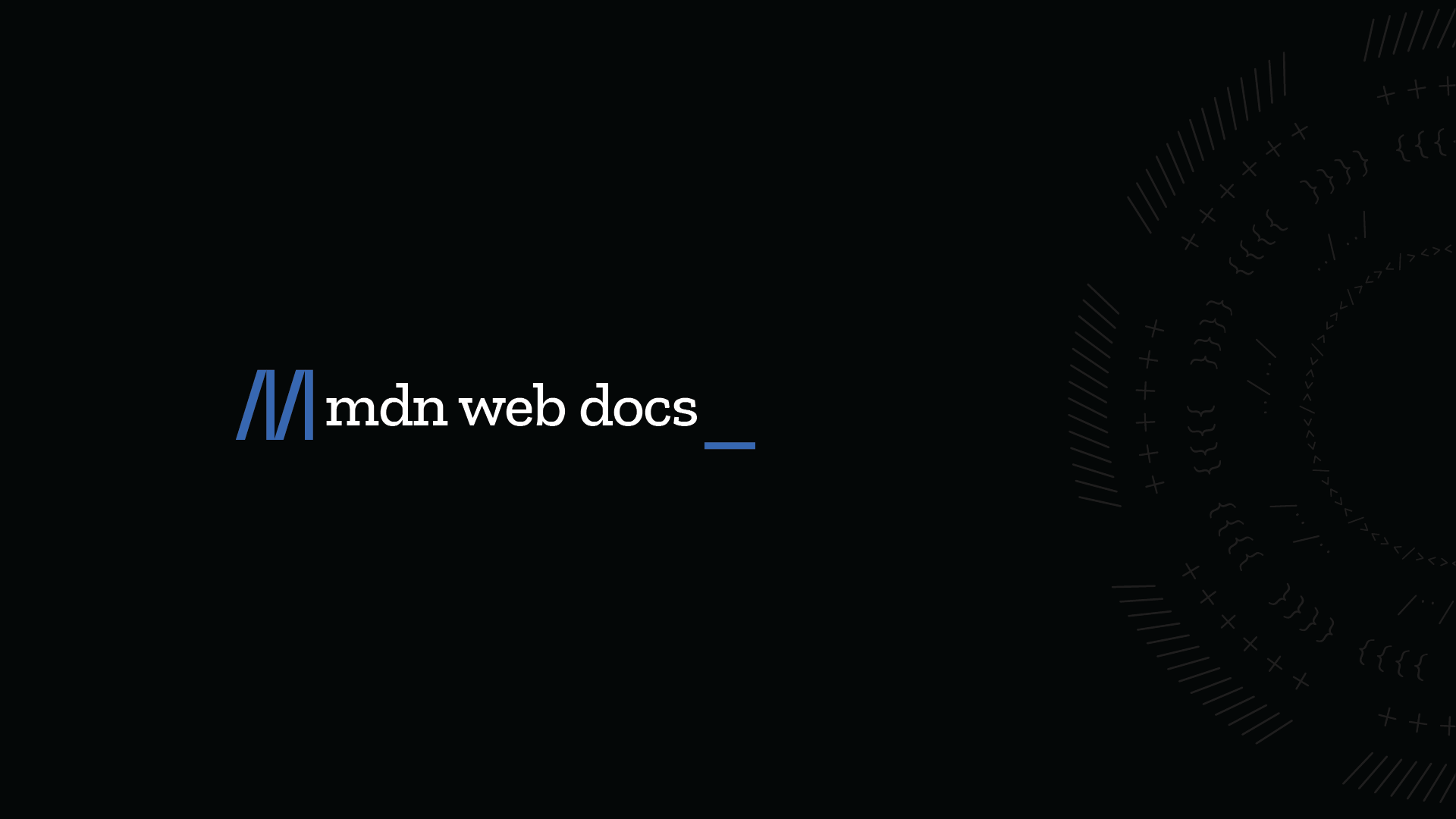
Simply put, we have to use the parentheses in order to execute the log
method and provide the message input in between the parentheses.
console.log("Hey developer! Cheers to objects and methods.");
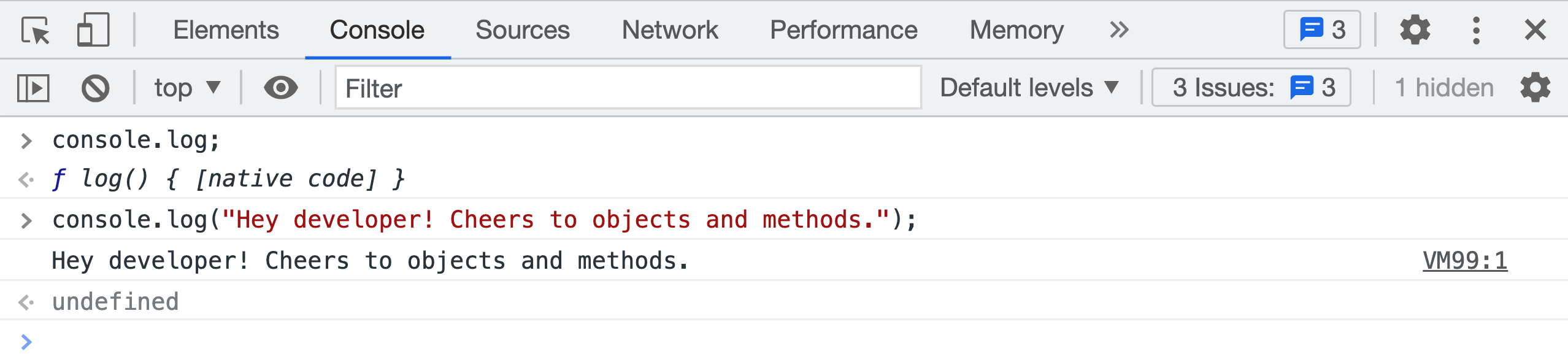
What we are seeing now is the result of the execution of instructions inside the log
method.
In simpler words, we are seeing our message logged inside the Console.
Anyway, let's quickly perform another action on the Browser Console.
I want to clear the existing code inside the Console using the console.clear()
method.
Actually, the console
object is more useful than what we just saw.
In the next lesson, we will see what other actions we can perform on the Console.