Accessing array items automatically using the "for" loop and it's counter
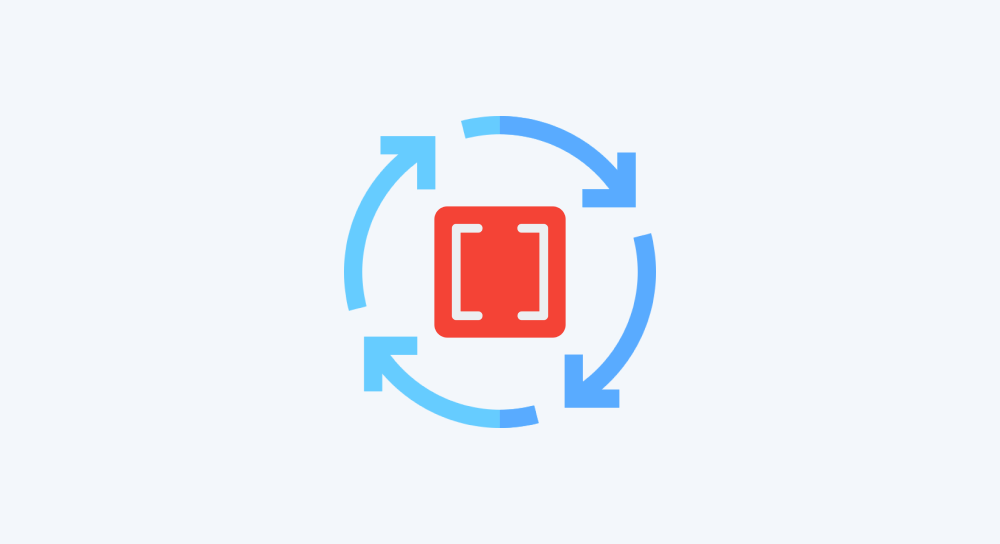
If you remember, we access the items of an array using their index value:
let unsafePasswords = [
"123456",
"password",
"admin",
"qwerty123",
"password123"
];
console.log(unsafePasswords[0]); // "123456"
console.log(unsafePasswords[1]); // "password"
console.log(unsafePasswords[2]); // "admin"
console.log(unsafePasswords[3]); // "qwerty123"
console.log(unsafePasswords[4]); // "password123"
console.log("Stop using these passwords!");
In the above snippet, we are outputting all the items inside the unsafePasswords
array into the browser console.
And we are outputting the items one by one manually.
But we can automate it using a "for" loop statement and its counter mechanism:
let unsafePasswords = [
"123456",
"password",
"admin",
"qwerty123",
"password123"
];
for(let counter = 0; counter < 5; counter++ ){
console.log(unsafePasswords[counter]);
}
console.warn("Stop using these passwords!");
This results in the following output if you open up the browser console:
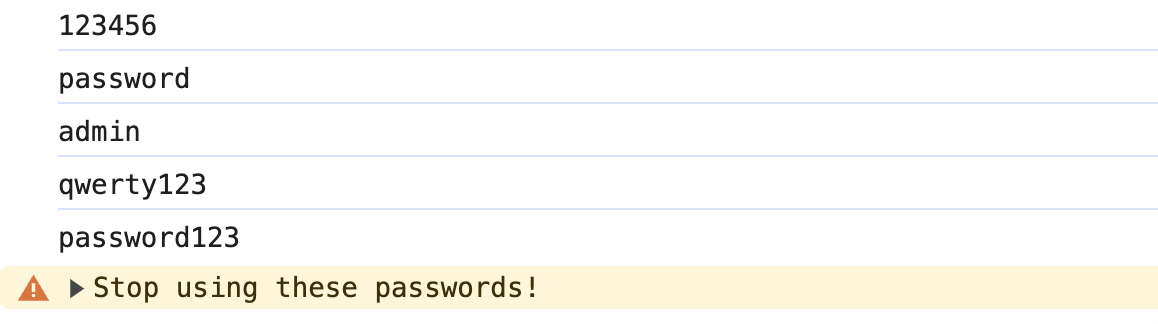
Now, let's go through the above code step by step so that you can clearly understand what's happening.
For starters...
When we are trying to access items of an array automatically using a loop statement, first, we have to figure out the count of the number of items inside an array.
This is easy.
let unsafePasswords = [
"123456",
"password",
"admin",
"qwerty123",
"password123"
];
Now tell me something: how many items are stored inside the unsafePasswords
array?
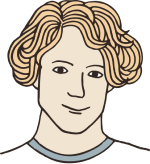
Yeah, easy. Five items!
Correct.
The reason why we need this count is that it will help us set up the conditional statement of the "for" loop.
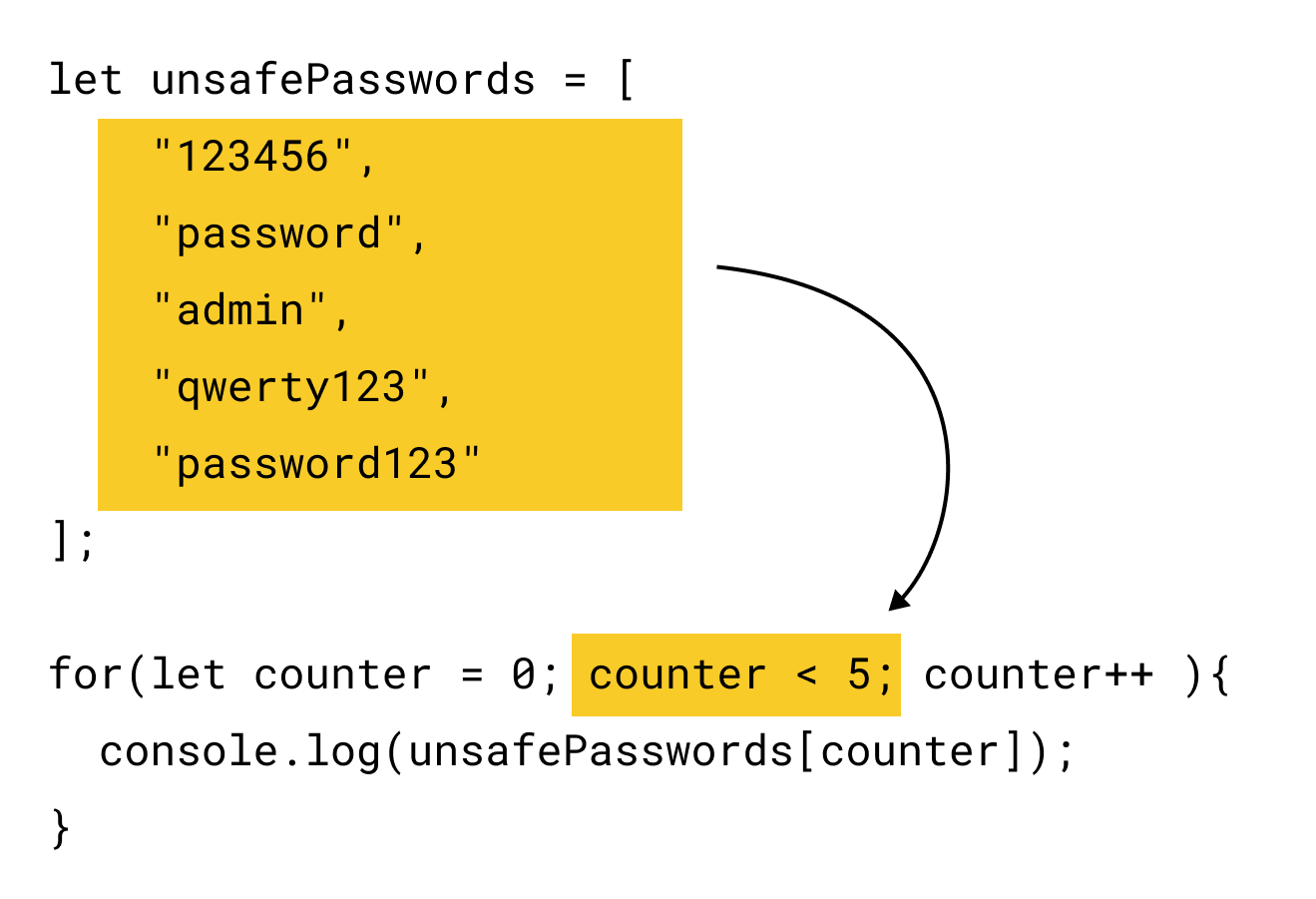
If you remember, the conditional statement will help us decide how many times the code inside the loop must run.
In our case, because the number of items inside the unsafePasswords
array is five, we only want the loop to run five times.
Not more and not less because running the loop five times is enough to output all five items into the browser console.
So, getting the count of items in an array is important.
Another important thing...
When working with arrays and "for" loops, remember that arrays start their indexing at 0.
That means the first element has an index of 0
, the second at 1, and so on.

So, to access all elements in an array using a loop, you need to start the counter at 0.
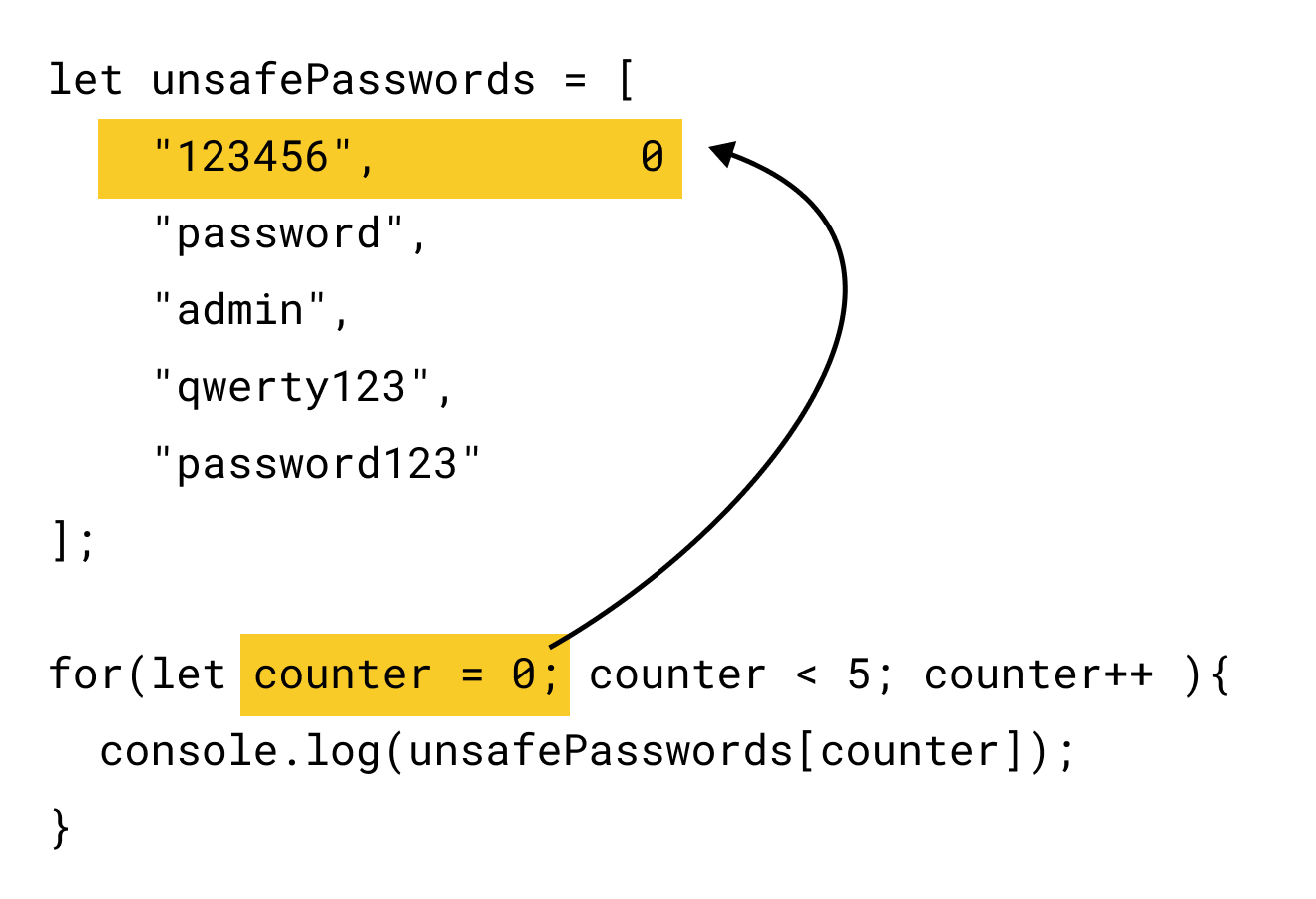
This will make sure that you don't miss the first item in an array.
If you start at 1, you'll miss the first item of the array that is located at index 0, like skipping the first person in a line!
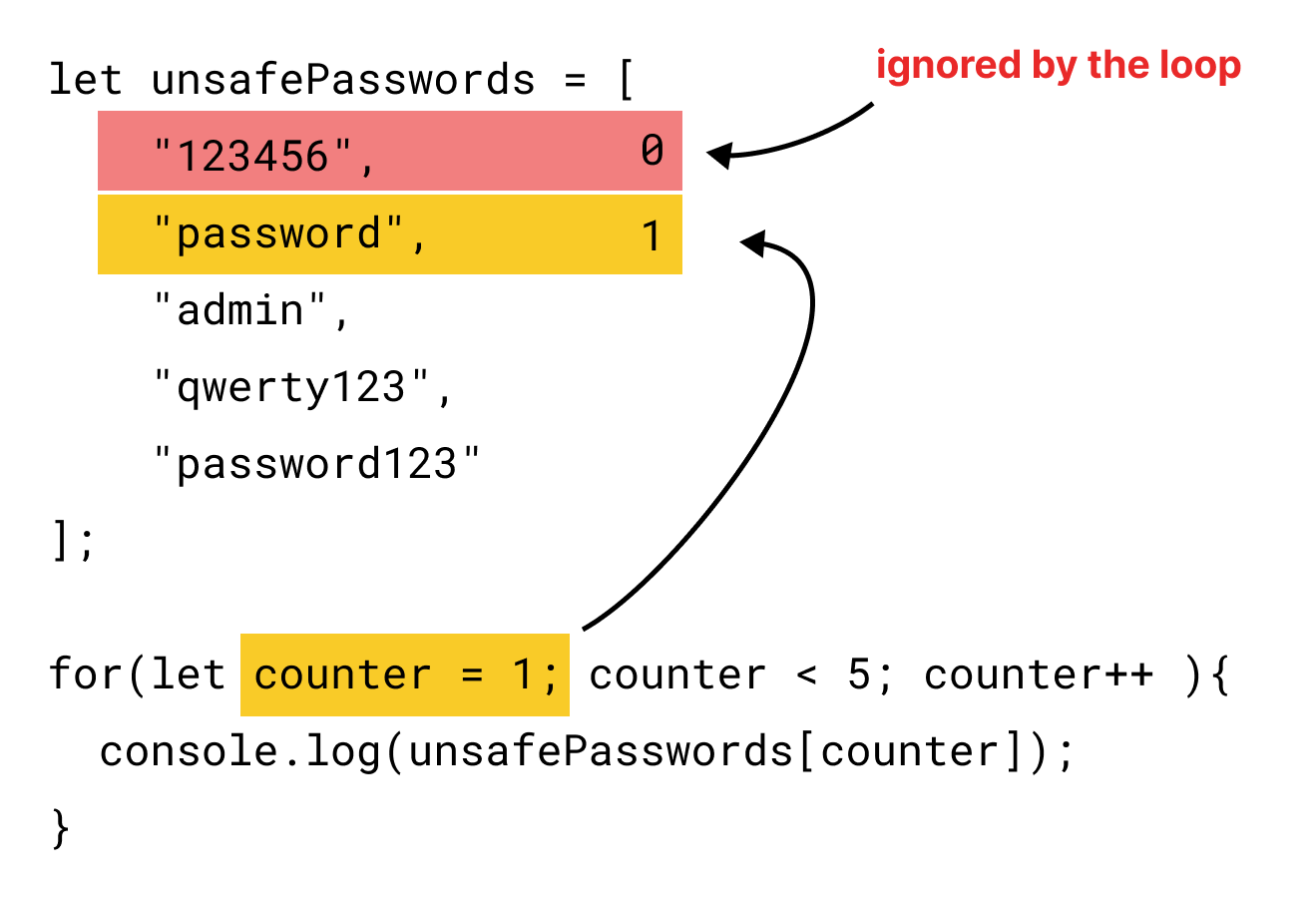
Also, if you notice, the conditional statement says that the counter must be less than 5:
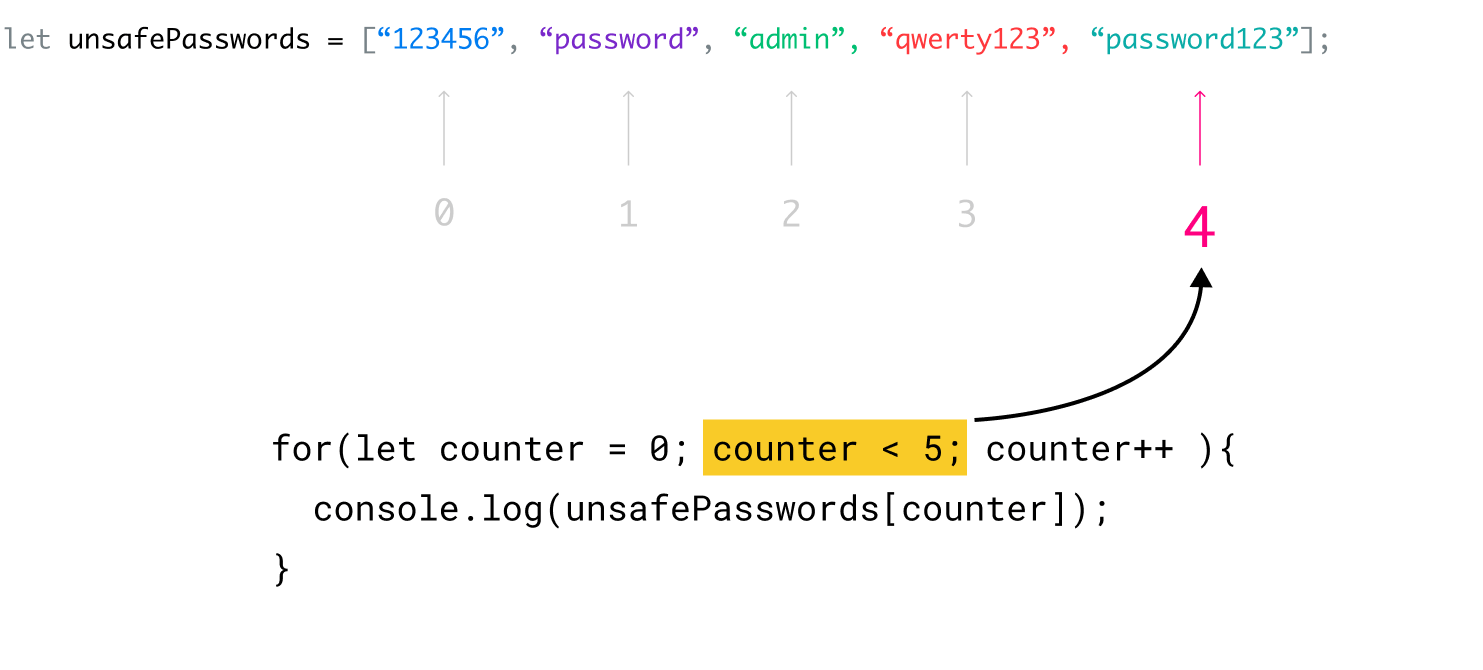
The counter < 5
condition might seem like it should run the loop only four times (0, 1, 2, 3).
But because we are starting the counter at 0
, the loop completes five iterations when the counter reaches four: 0, 1, 2, 3, 4.
This ensures all elements in the unsafePasswords
array are accessed and printed.
Why is this important?
In real-world scenarios, like checking for weak passwords, accessing all elements is important.
Imagine missing a weak password just because the loop skipped the first element due to incorrect indexing :D
Anyway, now that we understand the not-so-straight-forward parts of our task at hand...
Let's go through the code below step by step so that you can clearly understand what's happening inside every iteration.
let unsafePasswords = [
"123456",
"password",
"admin",
"qwerty123",
"password123"
];
for(let counter = 0; counter < 5; counter++ ){
console.log(unsafePasswords[counter]);
}
console.warn("Stop using these passwords!");
Before the loop begins:
We have created an array named unsafePasswords
, and it contains five weak passwords:
let unsafePasswords = [
"123456",
"password",
"admin",
"qwerty123",
"password123"
];
And then the loop begins:
for(let counter = 0; counter < 5; counter++ ){
console.log(unsafePasswords[counter]);
}
Iteration 1, Step 1: Set up the counter
To set up the counter, a variable named counter
is declared within the for
loop and initialized to 0
.
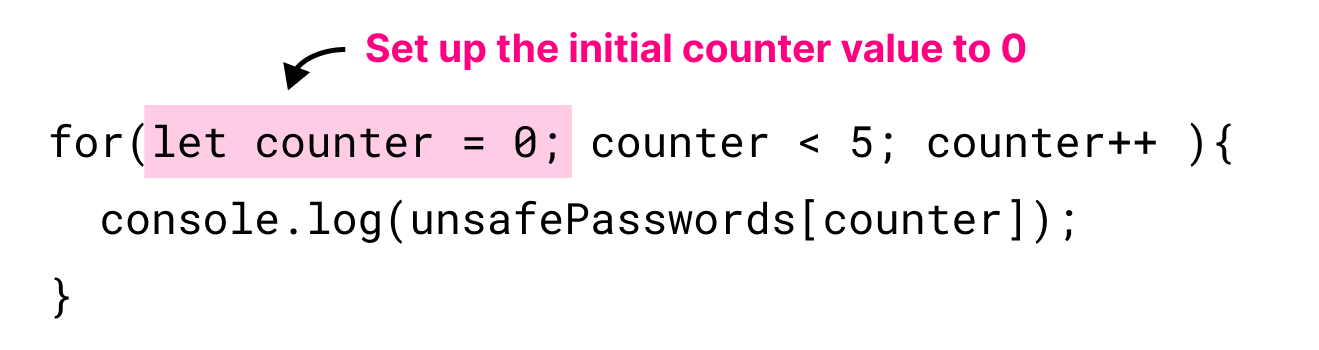
This is important because arrays are zero-indexed, meaning the first item has an index of 0
.
Iteration 1, Step 2: Checking the condition
Next, the loop mechanism will shift its focus to the condition statement.
The condition counter < 5
is evaluated.
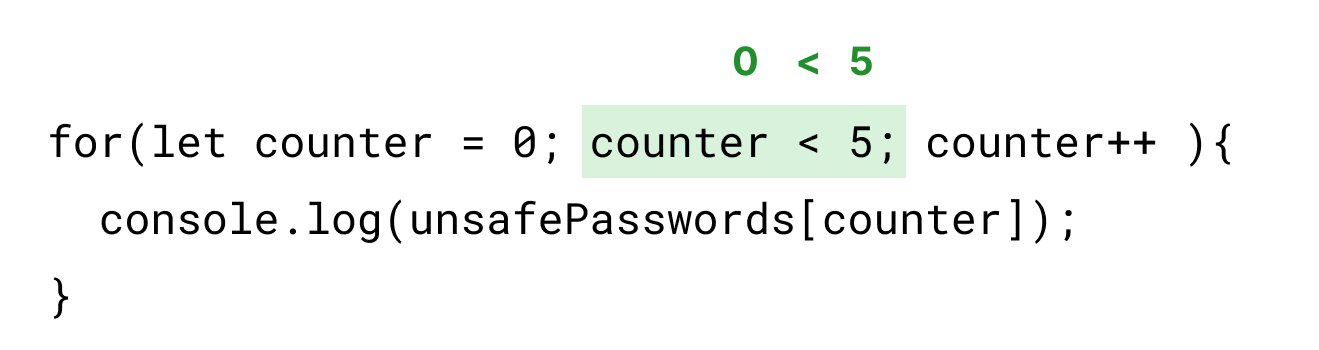
Since counter
is currently 0
(less than 5), the condition is true, and the loop proceeds to execute the code inside its body.
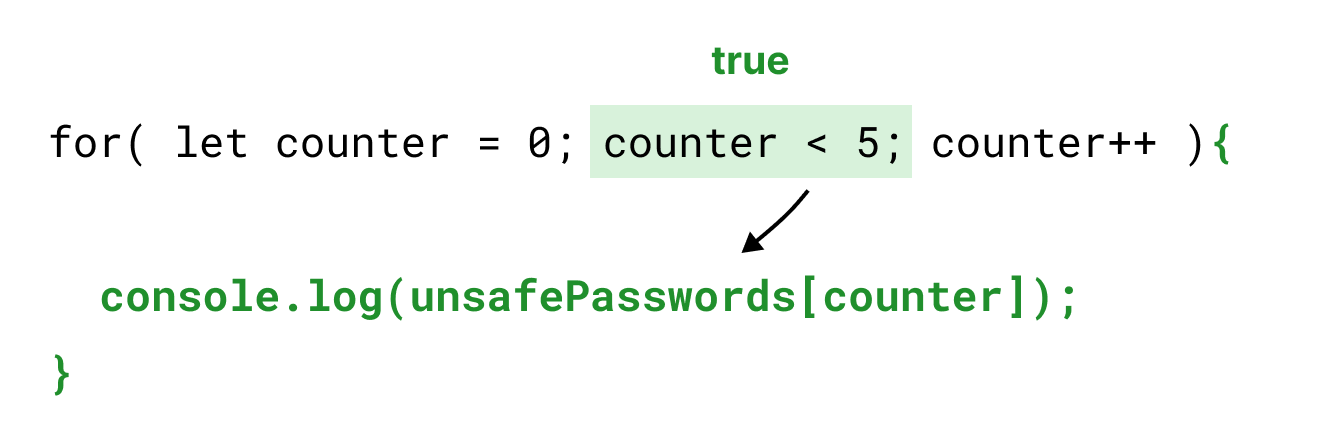
And inside the loop body, the console.log(unsafePasswords[counter])
statement is doing two things:
1) Array Access
unsafePasswords[counter];
During the first iteration, the value of the counter is zero, right?
So, technically, unsafePasswords[counter]
translates to:
unsafePasswords[0];
Simply put, the unsafePasswords[counter]
statement uses the current value of counter
(which is 0
in this iteration) as an index to access the first item in the unsafePasswords
array:
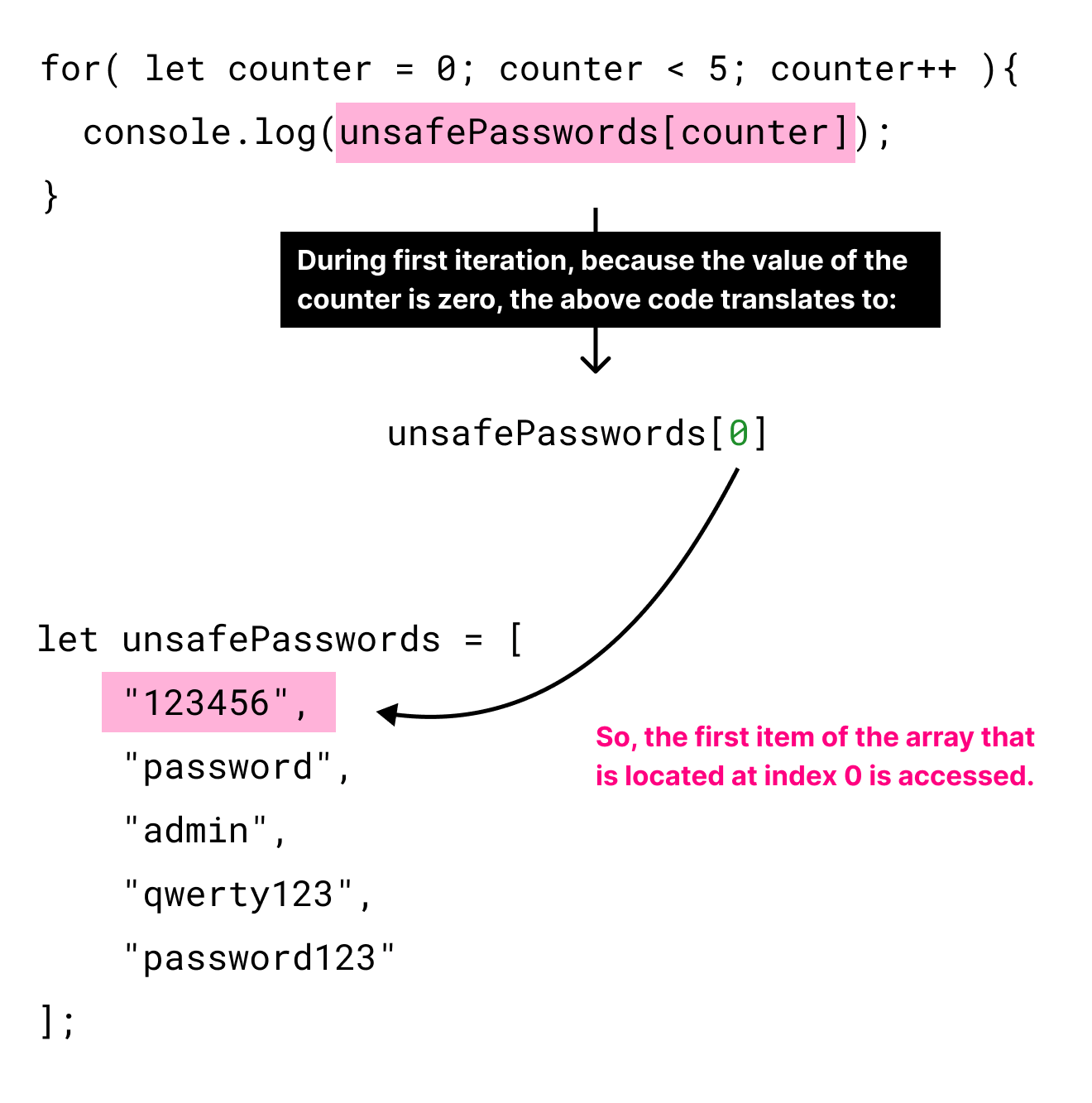
Remember, arrays are zero-indexed, so "123456" is at index 0.
2) Printing: The value retrieved from the array ("123456") is then printed to the browser console.
Iteration 1, Step 3: Updating the counter
Once all the code inside the loop body is executed, it technically means the first iteration is over.
So, the loop mechanism shifts its focus to updating the counter:
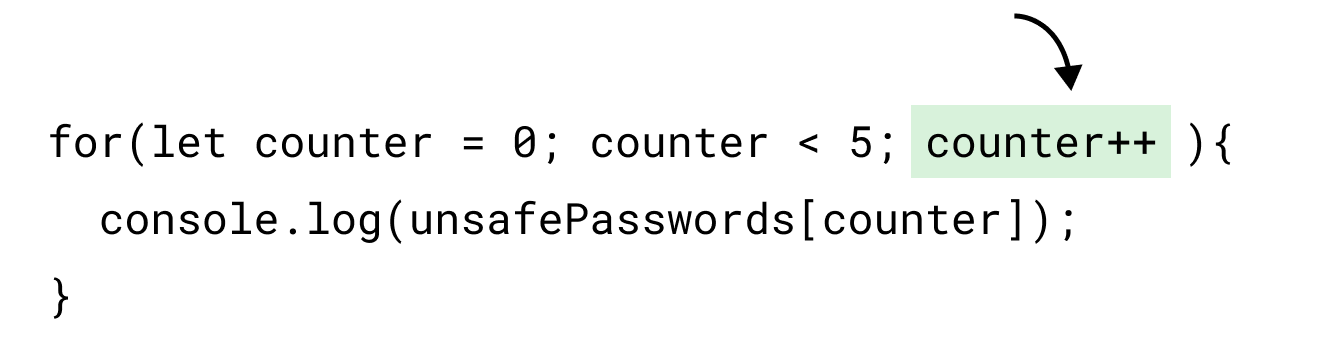
The counter++
statement increments the value of the counter
variable by 1.
In our case, the current value stored inside counter
variable is 0
right?
So, executing counter++
will update the current counter value to 1
.
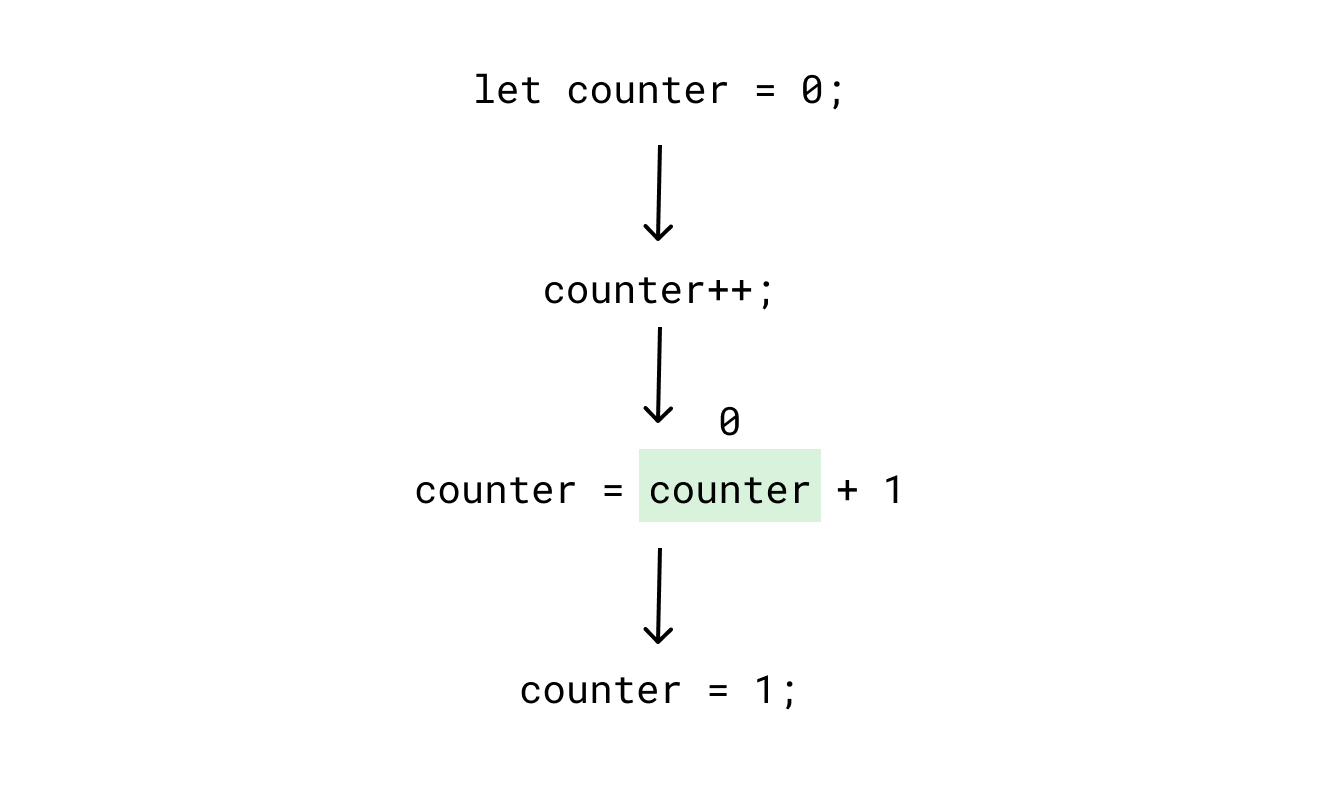
Because of this, the value stored inside the counter
variable is now 1
.
This also means our loop counter is now updated to 1
.
The loop now moves on to its second iteration.
Iteration 2, Step 1: Checking the condition
During the second iteration, the loop mechanism will ignore the counter set-up part because it was done during the first iteration.
In fact, it will be ignored for the rest of the iterations because the counter
variable declaration only happens during the first iteration:
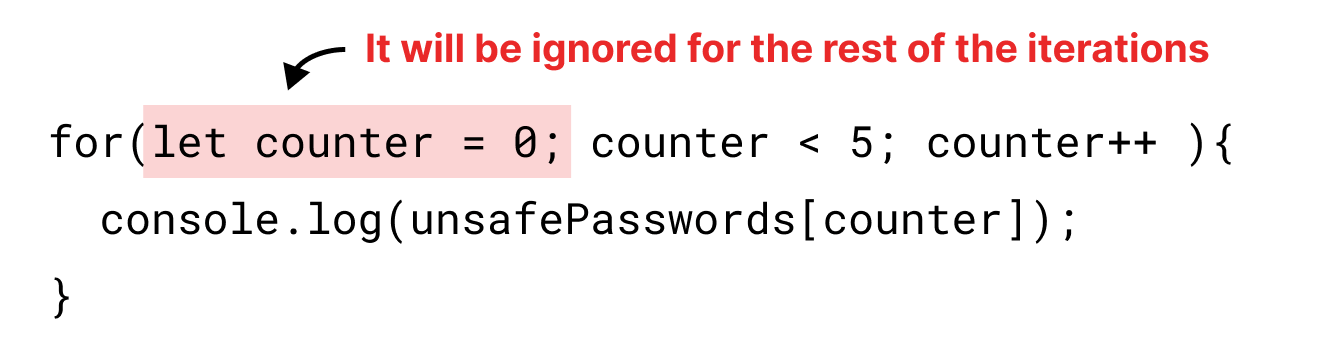
And the loop mechanism directly jumps to the conditional statement again:
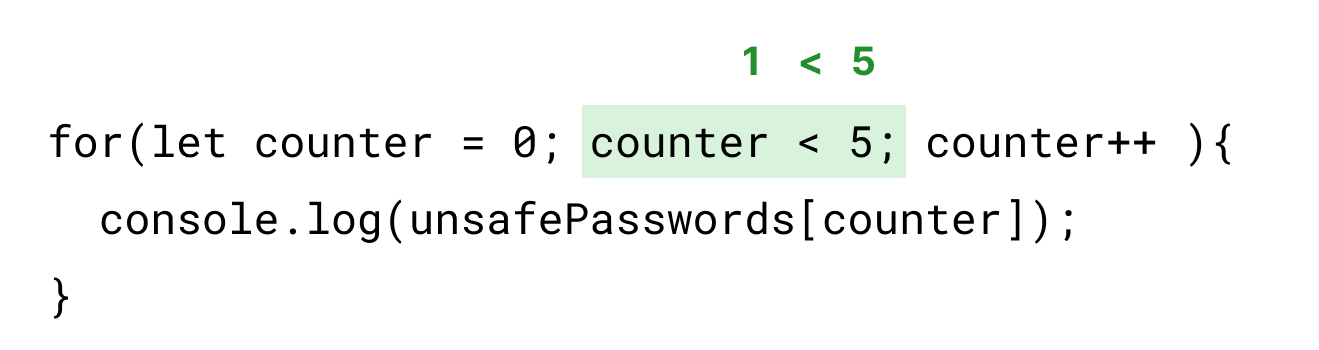
Because we have incremented the counter during the previous iteration, the current value of the counter is 1
, right?
Is 1
less than 5
?
True, it is.
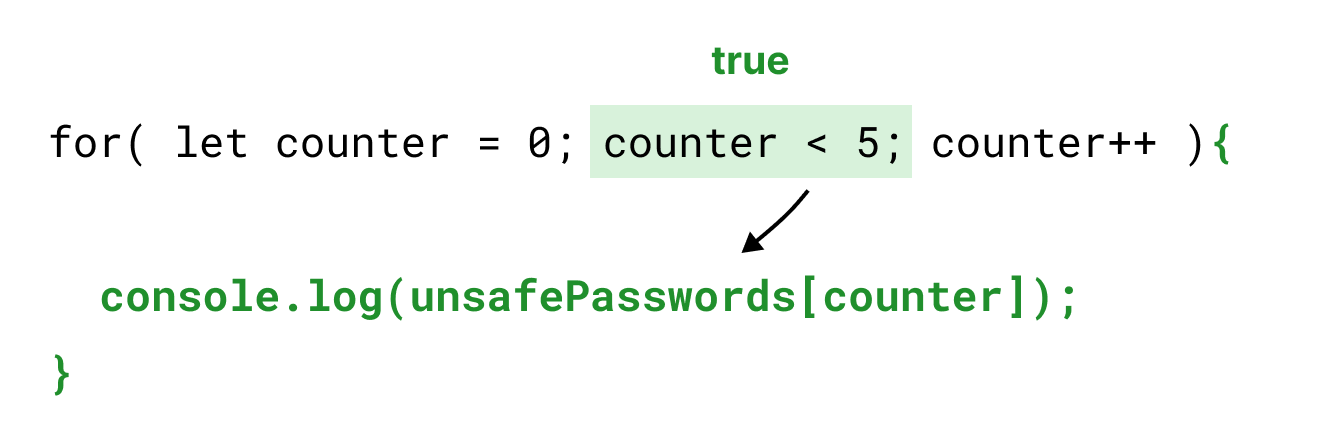
So the condition statement resolves to true, and the code inside the loop body will get executed for the second time.
And inside the loop body, the console.log(unsafePasswords[counter])
statement is doing two things again:
1) Array Access
unsafePasswords[counter];
During the second iteration, the value of the counter is 1
, right?
So, technically, unsafePasswords[counter]
translates to:
unsafePasswords[1];
Simply put, the unsafePasswords[counter]
statement uses the current value of counter
(which is 1
in this iteration) as an index to access the second item in the unsafePasswords
array:
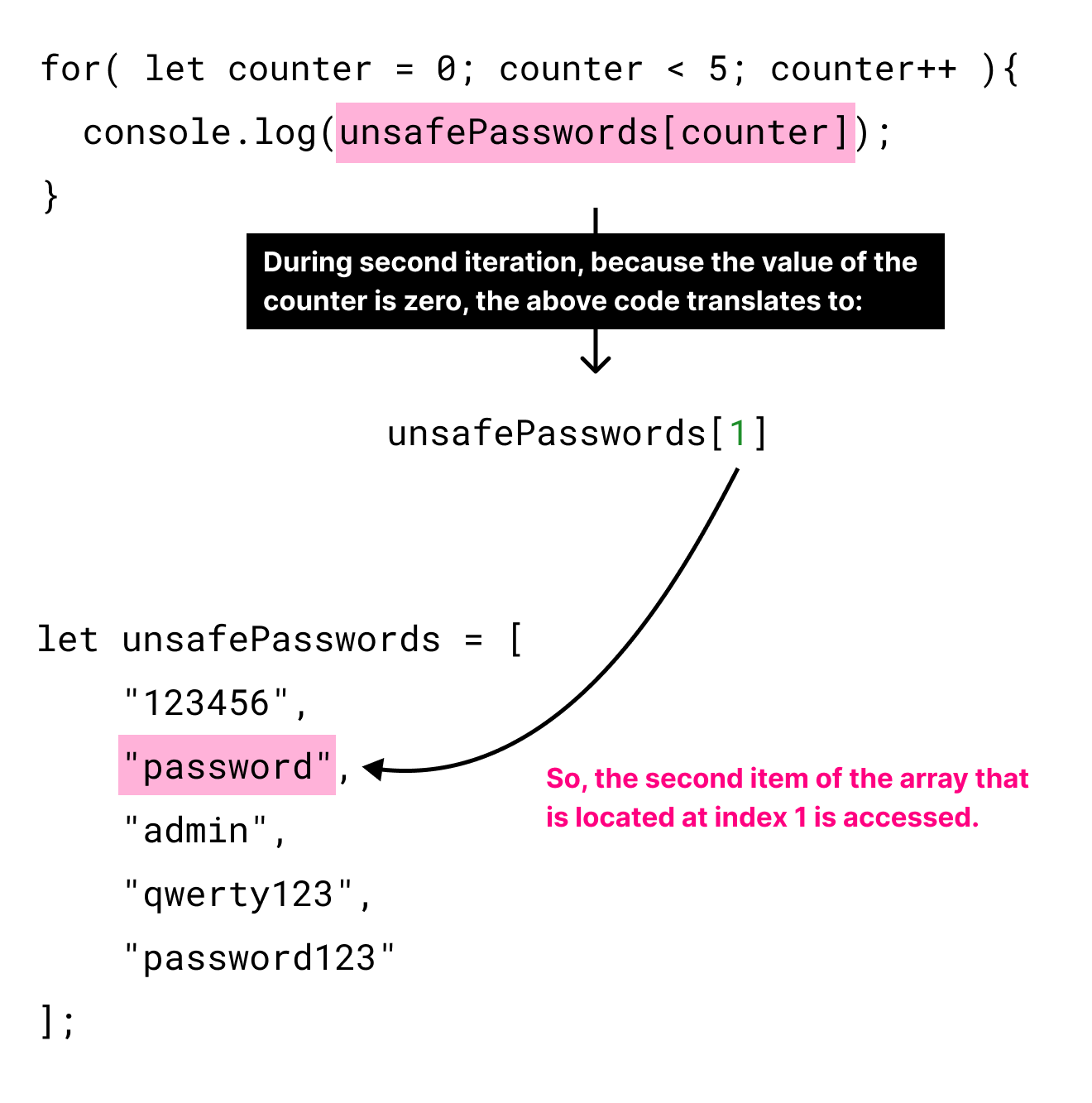
Remember, arrays are zero-indexed, so "password" is at index 1.
2) Printing: The value retrieved from the array ("password") is then printed to the browser console.
The same process repeats for the rest of the iterations, but with counter increasing, allowing access to different elements in the array.
In other words:
- Iteration 3 will access "admin" at index 2
- Iteration 4 will access "qwerty123" at index 3
- Finally, iteration 5 will access "password123" at index 4
At iteration 5 (last loop cycle), the loop is now completed and results in the following output inside the console:
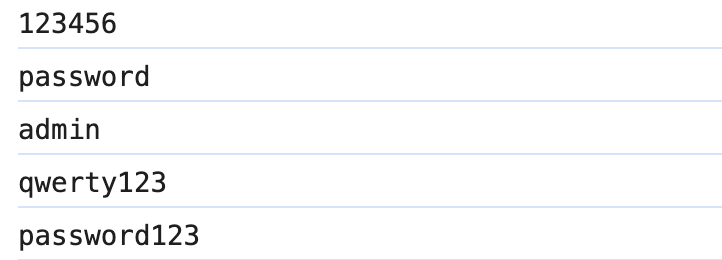
The loop now tries to move on to its sixth iteration and checks whether the current counter value is less than five.
Iteration 6, Step 1: Checking the condition
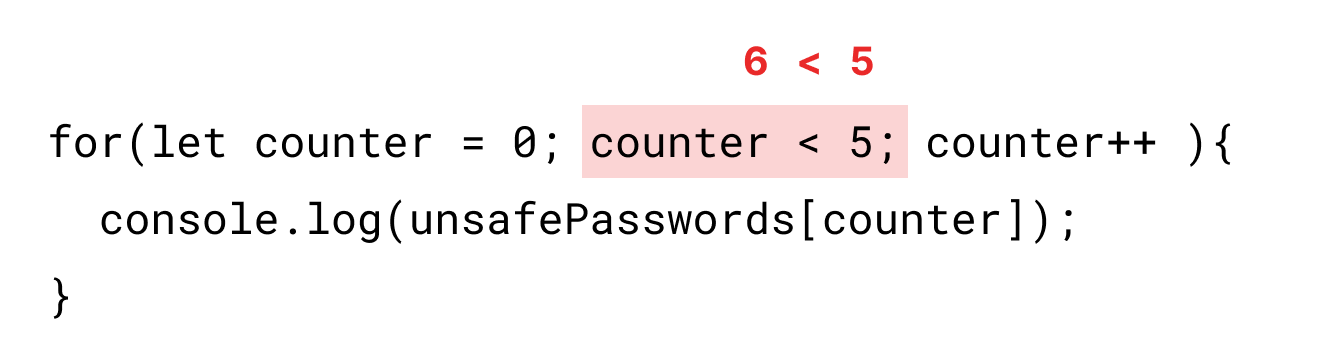
Because we have incremented the counter during the previous iteration, the current value of the counter is 6
, right?
Is 6
less than or equal to 5
?
Nope! False!
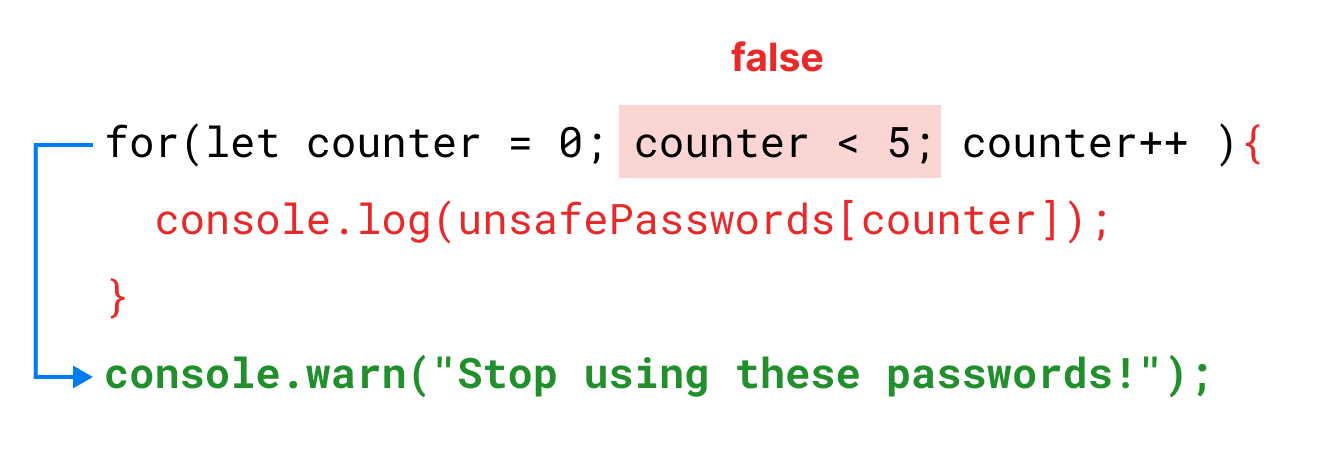
So, the condition statement resolves to false, and the code flow exits the "for" loop altogether when the condition statement returns false and starts executing the remaining code that comes after the for loop.
Currently, there is only one console.log
call after coming out of the loop:
console.warn("Stop using these passwords!");
So it will get executed.
Because of this, in the browser console, once all the array items have been logged, you'll see the message "Stop using these passwords!":
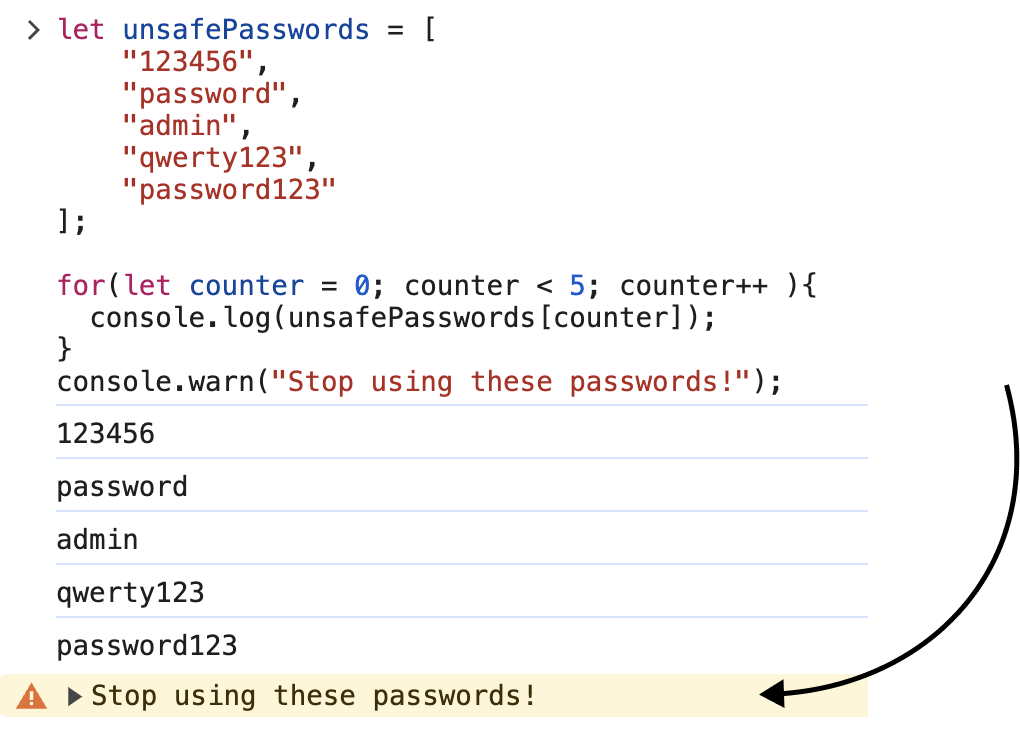
That's all.
That's how you can automatically access all the items of an array using a "for" loop.
But there is one problem in our loop implementation and we will learn about that in the next lesson.